icon: edit date: 2022-08-08 category:
整合Mybatis
导入依赖
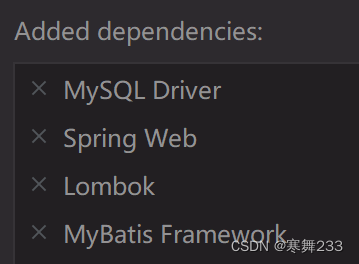
sql文件
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
DROP TABLE IF EXISTS `student`;
CREATE TABLE `student` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_general_ci DEFAULT NULL,
`sex` varchar(25) CHARACTER SET utf8mb4 COLLATE utf8mb4_general_ci DEFAULT NULL,
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 3 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_general_ci ROW_FORMAT = Dynamic;
INSERT INTO `student` VALUES (1, '张三', '男');
INSERT INTO `student` VALUES (2, '李四', '女');
SET FOREIGN_KEY_CHECKS = 1;
idea连接数据库
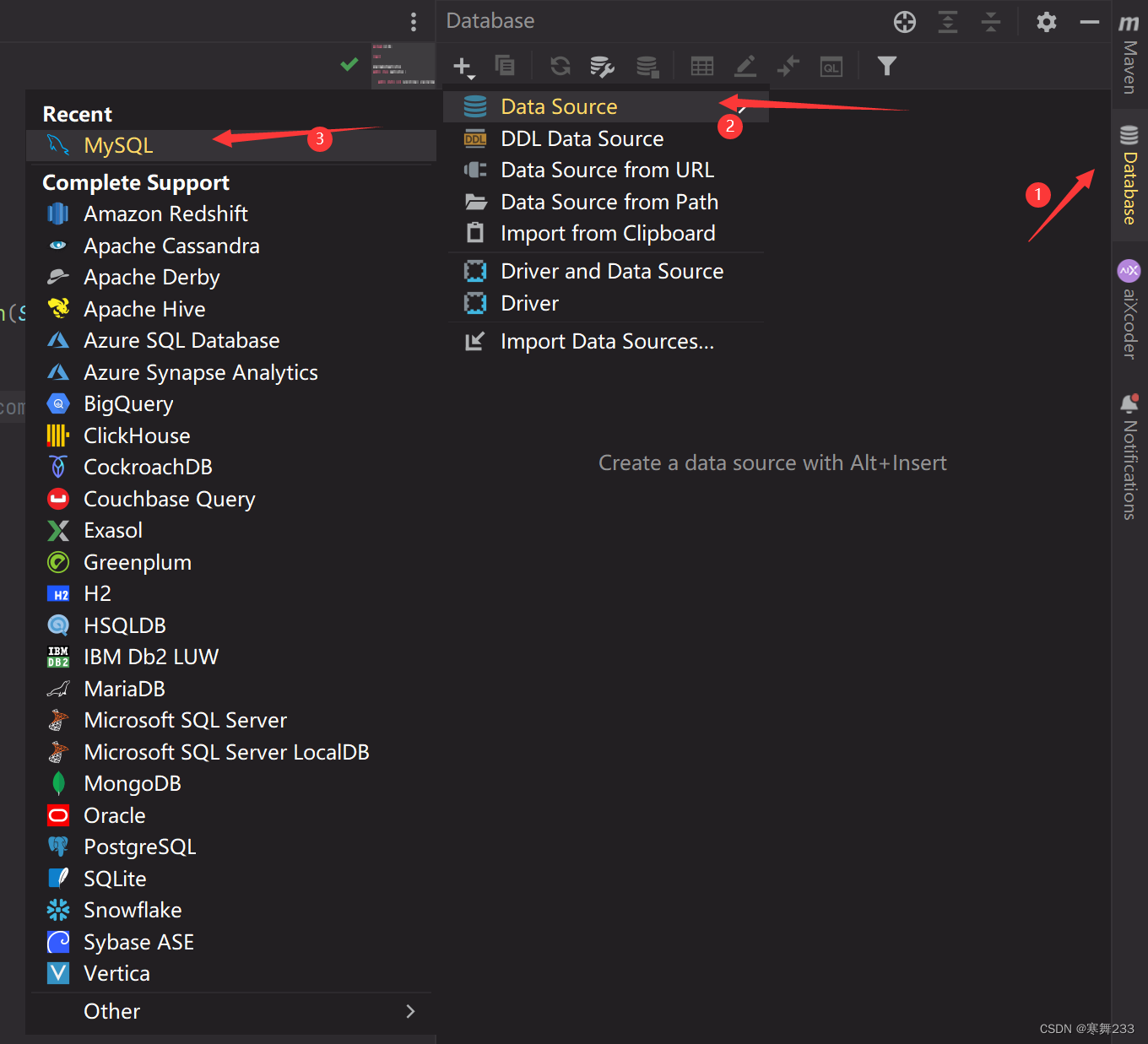
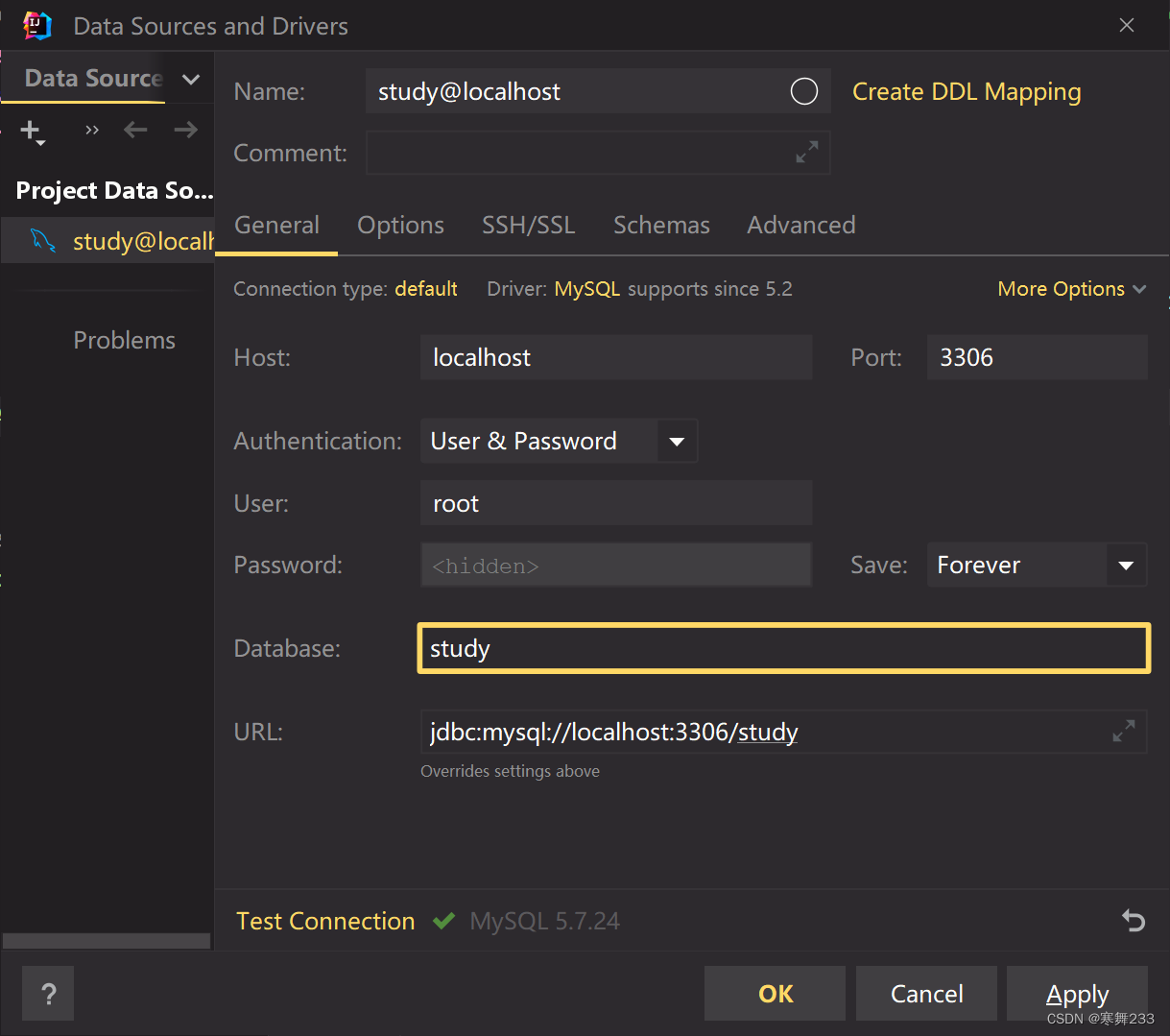
配置文件中配置数据库
application.yml
spring:
datasource:
username: root
password: root
url: jdbc:mysql://localhost:3306/study?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8&useSSL=false
driver-class-name: com.mysql.cj.jdbc.Driver
1.入门案例
1.1.创建实体类
entity.Student
@Data
public class Student {
private String name;
private String sex;
}
1.2.创建mapper
mapper.StudentMapper
@Mapper
public interface StudentMapper {
@Select("select * from student where id = #{id}")
Student selectStudent(int id);
}
1.3.编写controller
@RestController
@RequestMapping("/student")
public class MainController {
@Resource
private StudentMapper studentMapper;
@GetMapping("/{id}")
public Student index(@PathVariable int id) {
return studentMapper.selectStudent(id);
}
}
1.4.测试接口
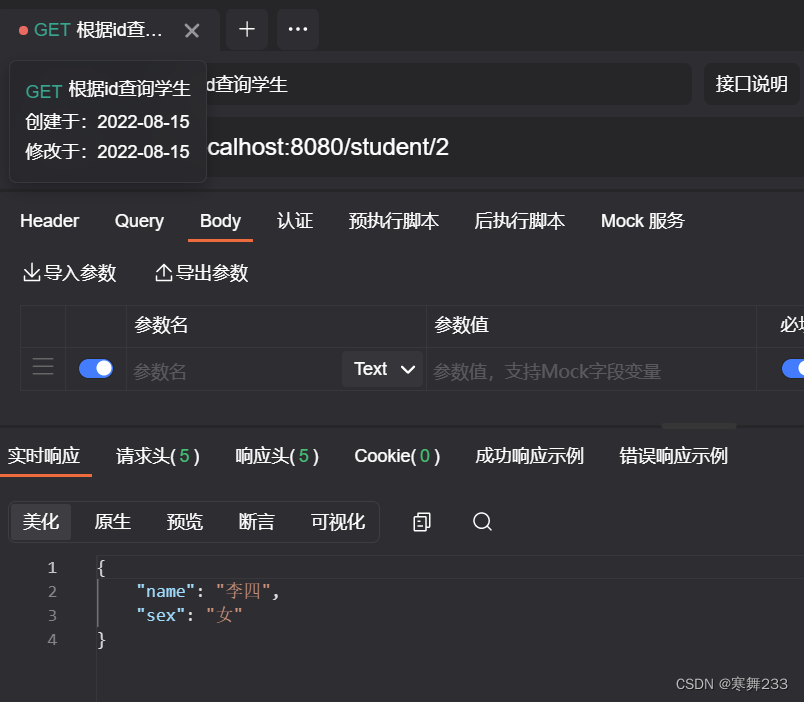
2. yaml
什么是yaml,和properties有什么区别?
- YAML(YAML Ain’t Markup Language),一种数据序列化格式
- 优点:
- 容易阅读
- 容易与脚本语言交互
- 以数据为核心,重数据轻格式
- YAML文件扩展名
2.1 yaml语法规则
- 大小写敏感
- 属性层级关系使用多行描述,每行结尾使用冒号结束
- 使用缩进表示层级关系,同层级左侧对齐,只允许使用空格(不允许使用Tab键)
- 属性值前面添加空格(属性名与属性值之间使用冒号+空格作为分隔)
- #表示注释
- 核心规则:数据前面要加空格与冒号隔开
2.2 yaml数组数据
- 数组数据在数据书写位置的下方使用减号作为数据开始符号,每行书写一个数据,减号与数据间空格分隔
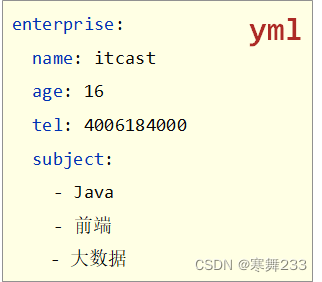
2.3 yaml数据读取
- 使用@Value读取单个数据,属性名引用方式:${一级属性名.二级属性名……}
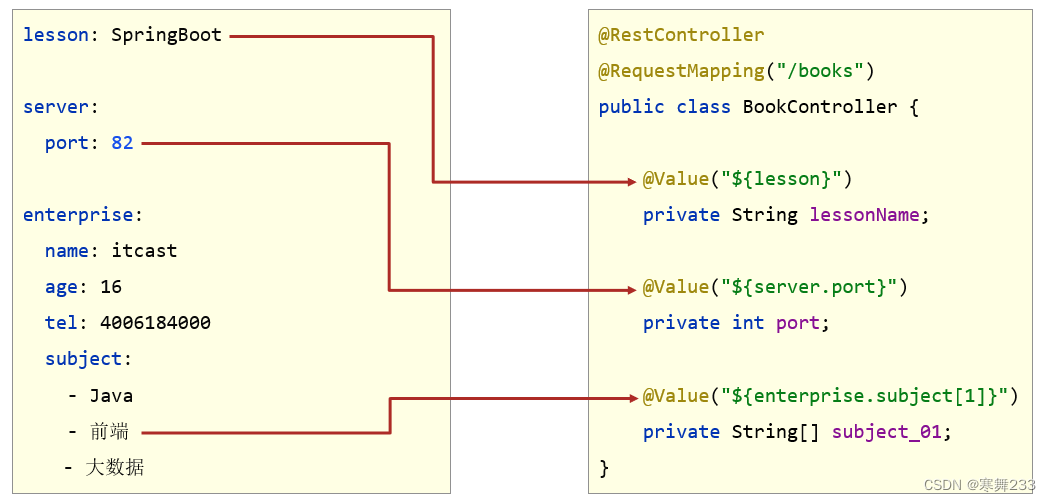
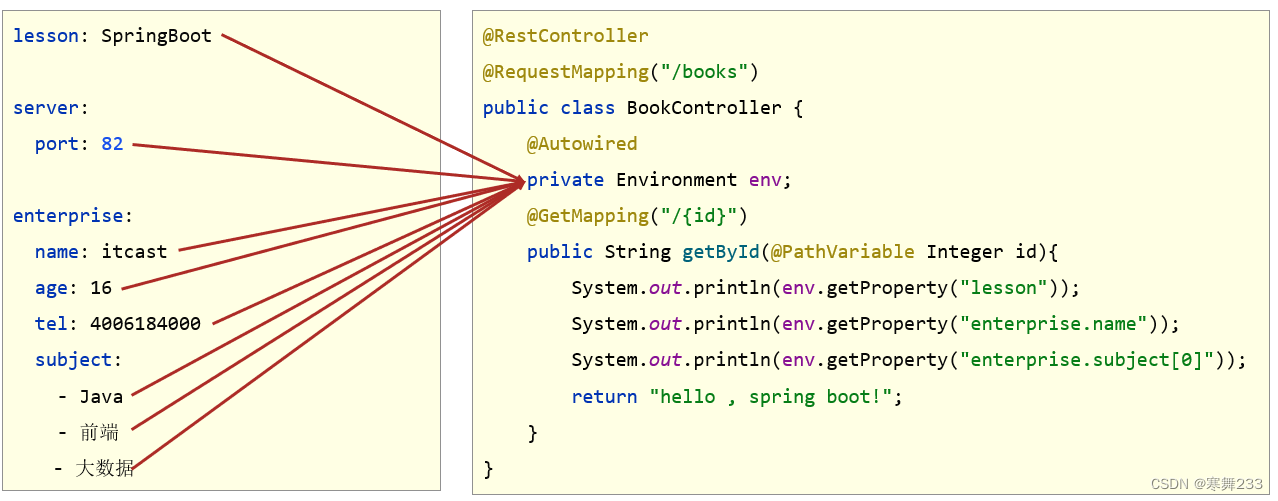
public class Enterprise {
private String name;
private Integer age;
private String tel;
private String[] subject;
}
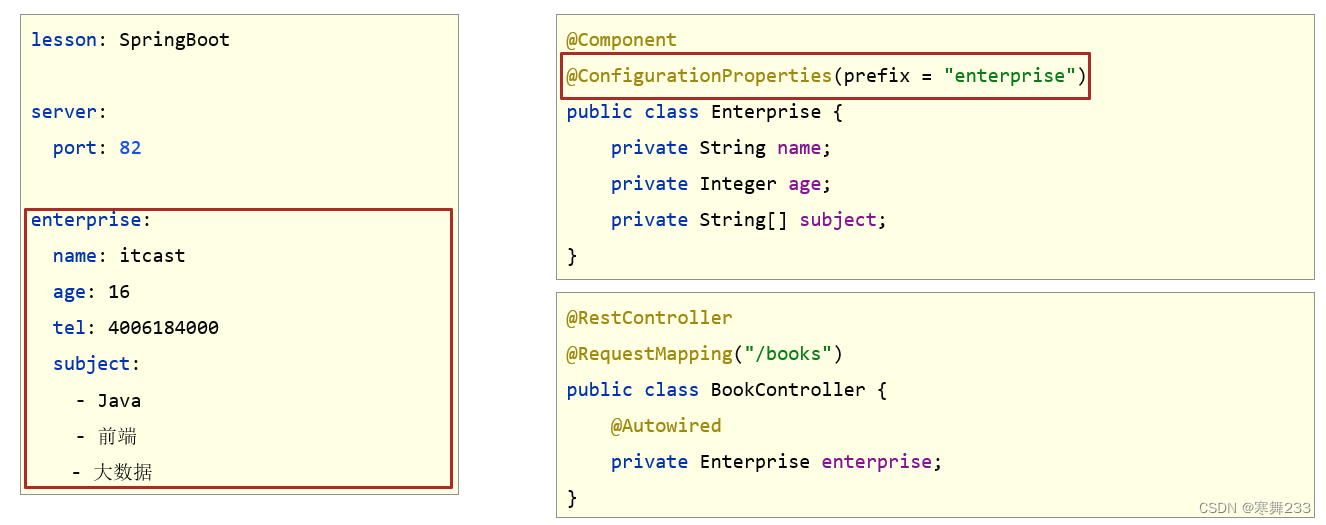

<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
3.1 多环境启动配置
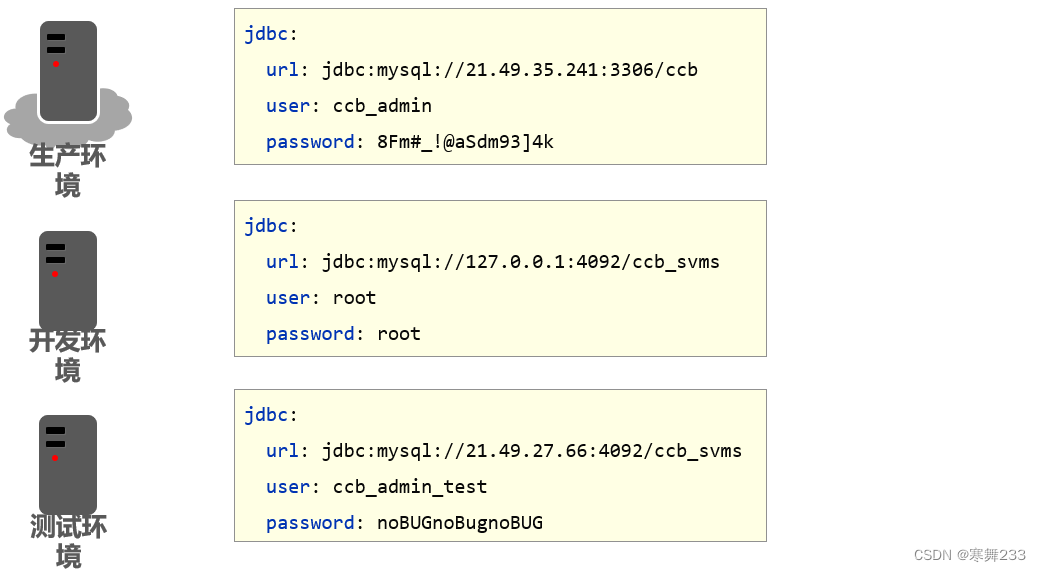 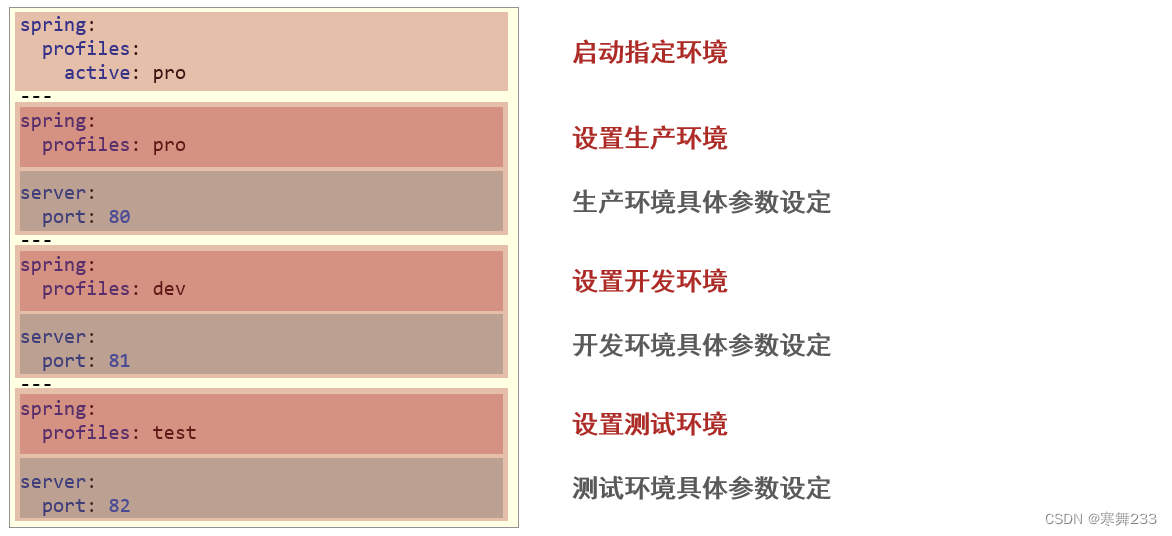 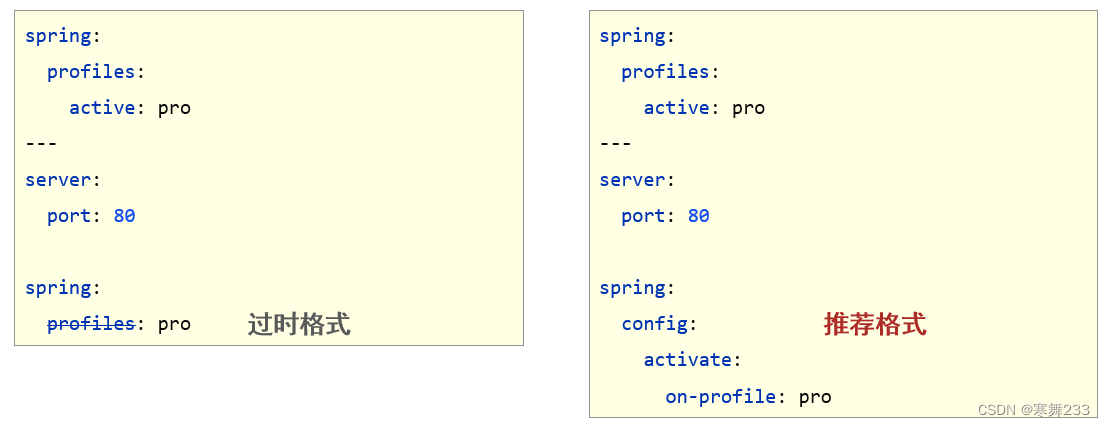
#主启动配置文件 application.properties
spring.profiles.active=pro
#环境分类配置文件 application-pro.properties
server.port=80
#环境分类配置文件 application-dev.properties
server.port=81
#环境分类配置文件application-test.properties
server.port=82
3.2 多环境启动命令格式
java –jar springboot.jar --spring.profiles.active=test
java –jar springboot.jar --server.port=88
java –jar springboot.jar --server.port=88 --spring.profiles.active=test
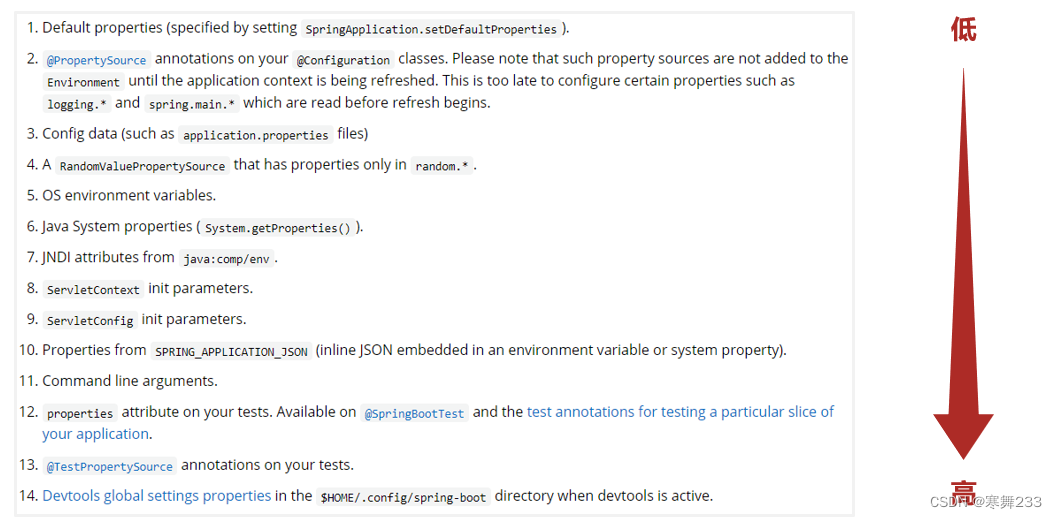
3.3 多环境开发控制
Maven与SpringBoot多环境兼容(步骤)
①:Maven中设置多环境属性
<profiles>
<profile>
<id>dev_env</id>
<properties>
<profile.active>dev</profile.active>
</properties>
<activation>
<activeByDefault>true</activeByDefault>
</activation>
</profile>
<profile>
<id>pro_env</id>
<properties>
<profile.active>pro</profile.active>
</properties>
</profile>
<profile>
<id>test_env</id>
<properties>
<profile.active>test</profile.active>
</properties>
</profile>
</profiles>
②:SpringBoot中引用Maven属性
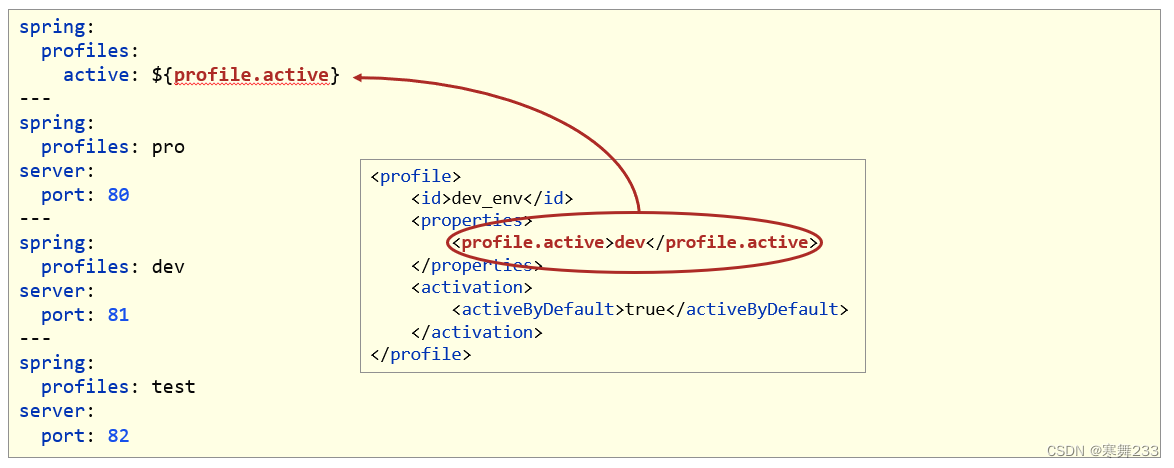
③:执行Maven打包指令
- Maven指令执行完毕后,生成了对应的包,其中类参与编译,但是配置文件并没有编译,而是复制到包中

- 解决思路:对于源码中非java类的操作要求加载Maven对应的属性,解析${}占位符
④:对资源文件开启对默认占位符的解析
<build>
<plugins>
<plugin>
<artifactId>maven-resources-plugin</artifactId>
<configuration>
<encoding>utf-8</encoding>
<useDefaultDelimiters>true</useDefaultDelimiters>
</configuration>
</plugin>
</plugins>
</build>

4.整合druid
Druid是一个关系型数据库连接池,是阿里巴巴的一个开源项目,地址:https://github.com/alibaba/druid。Druid不但提供连接池的功能,还提供监控功能,可以实时查看数据库连接池和SQL查询的工作情况。
常见问题 · alibaba/druid Wiki (github.com)

springBoot默认使用hikari数据源
4.1 添加依赖
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.2.11</version>
</dependency>
4.2. 基础使用
spring:
datasource:
type: com.alibaba.druid.pool.DruidDataSource
druid:
username: root
password: root
url: jdbc:mysql://localhost:3306/study?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8&useSSL=false
driver-class-name: com.mysql.cj.jdbc.Driver

4.3 自定义配置
spring:
datasource:
type: com.alibaba.druid.pool.DruidDataSource
druid:
username: root
password: root
url: jdbc:mysql://localhost:3306/study?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8&useSSL=false
driver-class-name: com.mysql.cj.jdbc.Driver
initial-size: 5
min-idle: 10
max-active: 20
max-wait: 60000
min-evictable-idle-time-millis: 300000
time-between-eviction-runs-millis: 60000
filters: stat,wall
validation-query: SELECT 1
test-on-borrow: true
test-on-return: true
test-while-idle: true
stat-view-servlet:
enabled: true
allow: 127.0.0.1
login-username: admin
login-password: admin
filter:
stat:
enabled: true
log-slow-sql: true
slow-sql-millis: 5000
merge-sql: false
访问: http://localhost:8080/druid/index.html 登录查看数据源
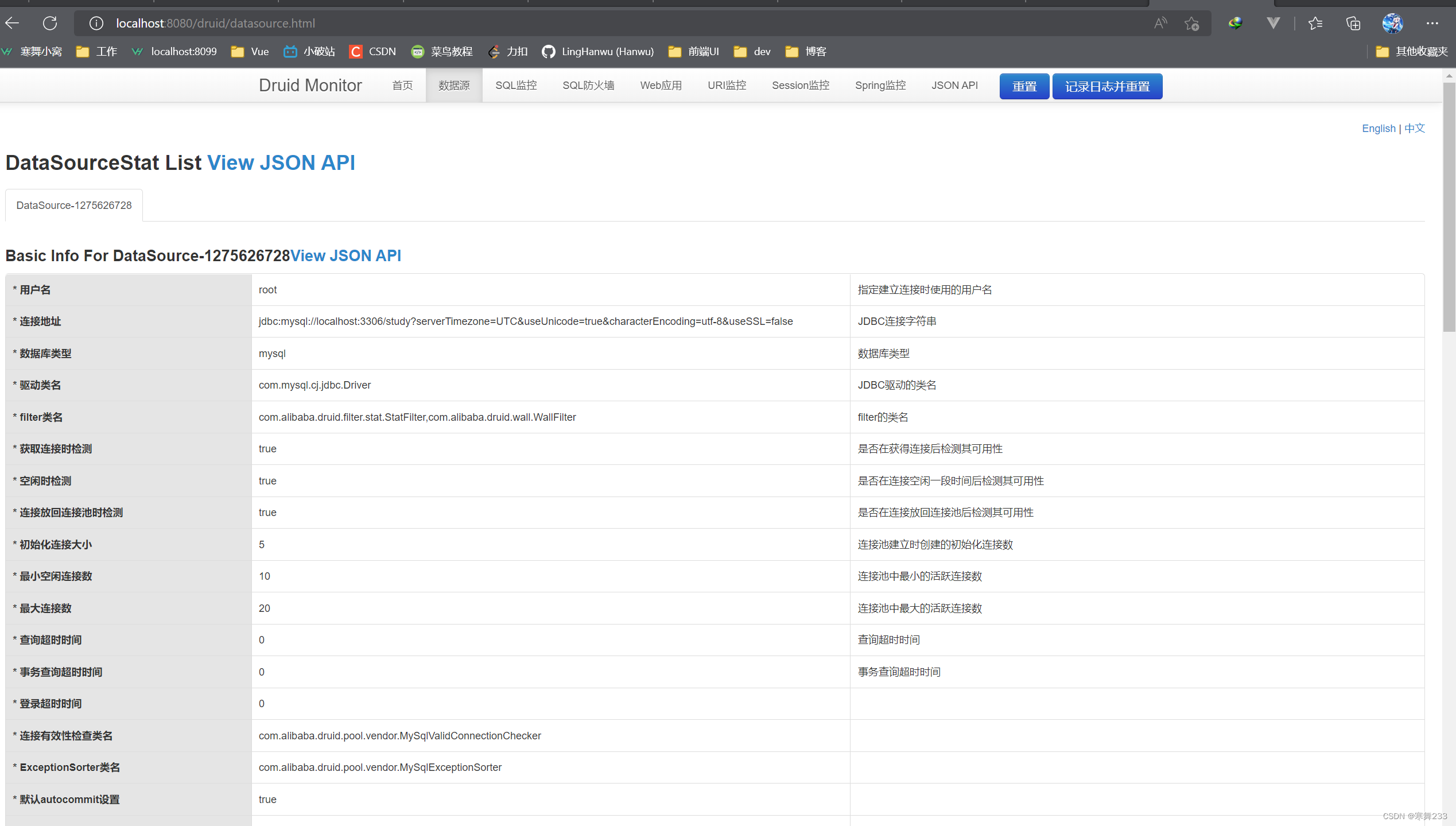
查看sql监控

|