官网 https://spring.io/projects/spring-data
来看一张全景图:
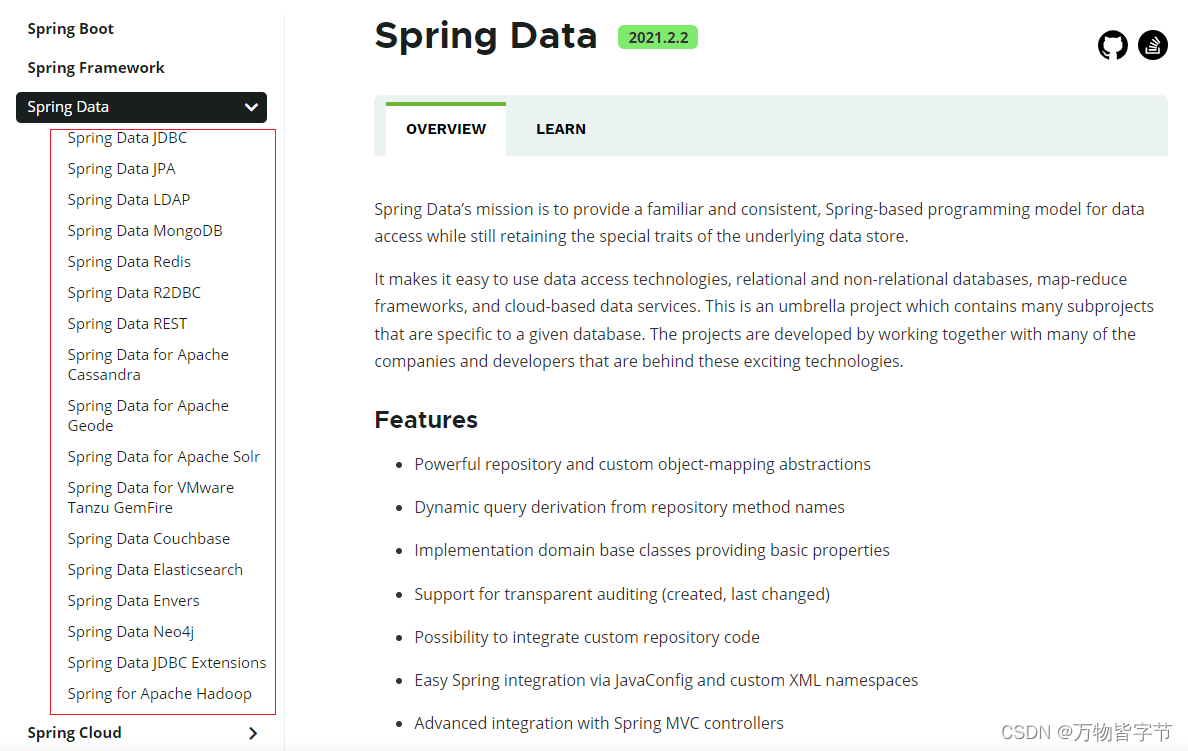
本文涉及框架版本说明:
组件 | 版本 |
---|
es服务版本 | 7.10.1 | spring-boot 版本 | 2.3.12-RELEASE |
文章涉及代码已上传到 GITEE https://gitee.com/aqu415/demo/tree/master/spring-data
spring-data-elasticsearch
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>spring-data</artifactId>
<groupId>com.uu</groupId>
<version>1.0.0</version>
<relativePath>../pom.xml</relativePath>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>spring-data-es-demo</artifactId>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<!-- Spring Boot Elasticsearch 依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
</dependencies>
</project>
spring:
elasticsearch:
rest:
uris: http://192.168.126.105:9200
package com.xx.entity;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
@Data
@Document(indexName = "info")
@Builder
@AllArgsConstructor
@NoArgsConstructor
public class Info implements java.io.Serializable {
@Id
@Field(type = FieldType.Long, store = true)
private long id;
@Field(type = FieldType.Text, store = true, analyzer = "ik_smart")
private String title;
@Field(type = FieldType.Text, store = true, analyzer = "ik_smart")
private String content;
}
package com.xx.repository;
import com.xx.entity.Info;
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface InfoRepository extends ElasticsearchRepository<Info, Long> {
}
package com.xx;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ESApplication {
public static void main(String[] args) {
SpringApplication.run(ESApplication.class, args);
}
}
package com.xx;
import com.xx.entity.Info;
import com.xx.repository.InfoRepository;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import javax.annotation.Resource;
import java.util.Arrays;
import java.util.UUID;
@RunWith(SpringRunner.class)
@SpringBootTest(classes = ESApplication.class)
public class ESTest {
@Resource
private InfoRepository infoRepository;
@Test
public void batchSaveTest() {
Info info = Info.builder().id(System.currentTimeMillis()).content(UUID.randomUUID().toString()).title(UUID.randomUUID().toString()).build();
Iterable<Info> infos = infoRepository.saveAll(Arrays.asList(info));
System.out.println(infos);
}
@Test
public void indexTest() {
Iterable<Info> all = this.infoRepository.findAll();
System.out.println(all);
}
}
- 测试结果
写入 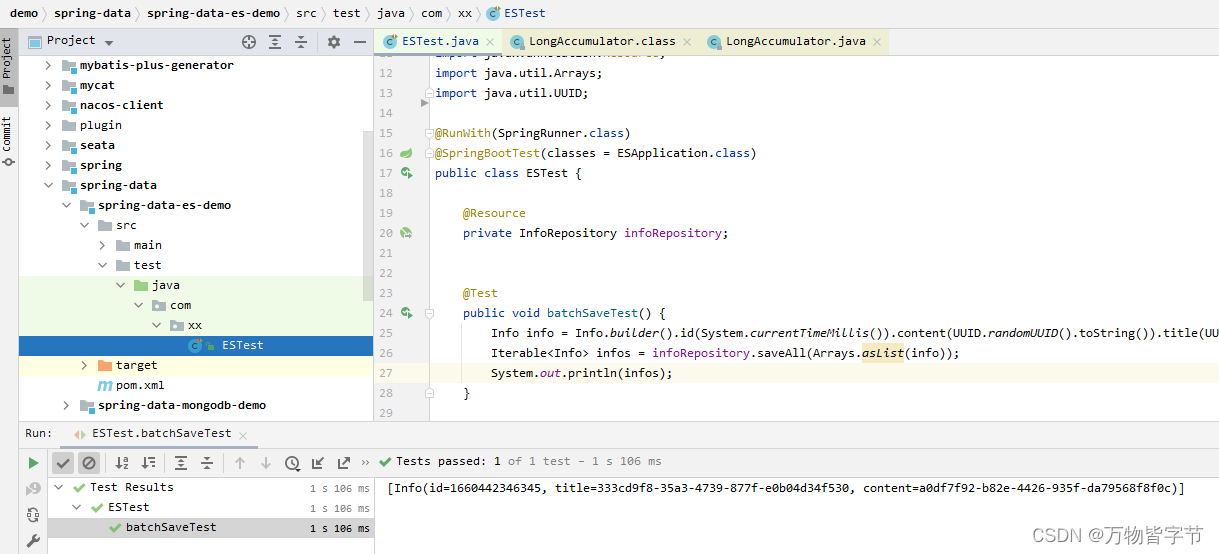
查询 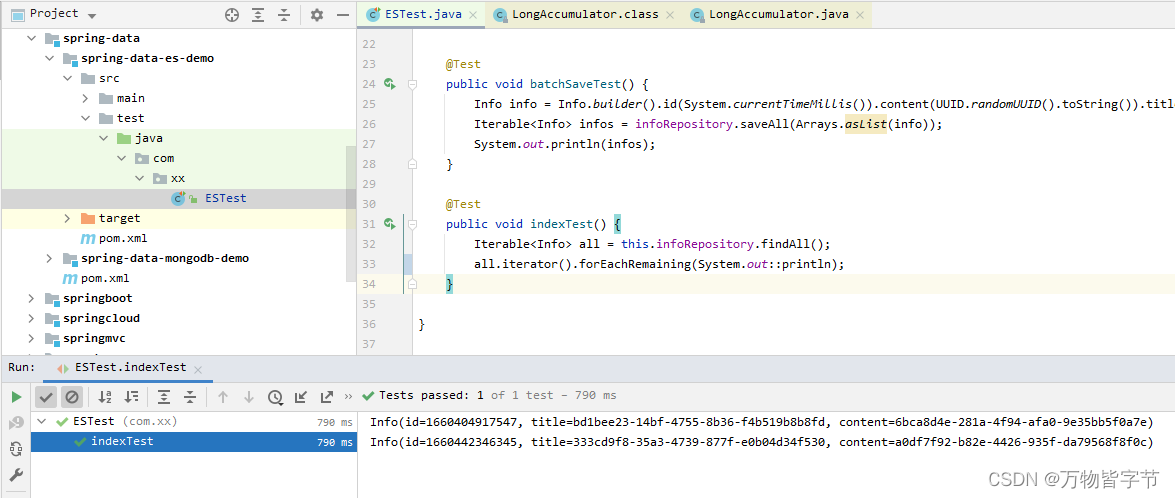
spring-data-mongodb
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>spring-data</artifactId>
<groupId>com.uu</groupId>
<version>1.0.0</version>
<relativePath>../pom.xml</relativePath>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>spring-data-mongodb-demo</artifactId>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
</dependencies>
</project>
spring:
application:
name: mongo
data:
mongodb:
uri: mongodb://127.0.0.1:27017/test
package com.xx.entity;
import lombok.Builder;
import lombok.Data;
import lombok.ToString;
import org.springframework.data.mongodb.core.index.Indexed;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection = "test")
@Data
@ToString
@Builder
public class People implements java.io.Serializable {
@Indexed
private String name;
@Indexed
private int age;
}
package com.xx.service;
import com.xx.entity.People;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.List;
@Service
public class PeopleServie {
@Resource
private MongoTemplate mongoTemplate;
public List<People> getAll() {
return mongoTemplate.findAll(People.class);
}
public void batchSave(People people){
mongoTemplate.save(people);
}
}
package com.xx;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MongoApp {
public static void main(String[] args) {
SpringApplication.run(MongoApp.class);
}
}
package java.com.xx;//package com.xx.test;
//
import com.xx.MongoApp;
import com.xx.entity.People;
import com.xx.service.PeopleServie;
import lombok.extern.slf4j.Slf4j;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import javax.annotation.Resource;
@RunWith(SpringRunner.class)
@SpringBootTest(classes = MongoApp.class)
@Slf4j
public class MongoTest {
@Resource
private PeopleServie peopleServie;
@Test
public void getAllTest() {
log.info("" + peopleServie.getAll());
}
@Test
public void batchSaveTest() {
for (int i = 0; i < 10000; i++) {
this.peopleServie.batchSave(People.builder().name(String.valueOf(i)).age(i).build());
}
log.info("save over");
}
}
文章涉及代码已上传到 GITEE https://gitee.com/aqu415/demo/tree/master/spring-data
|