一、RestTemplate 概述
在实际开发中需要调用第三方接口数据,或者是调用另外一个服务接口时,我们可以使用spring 框架提供的 RestTemplate 类可用于在应用中调用 rest 服务,它简化了与 http 服务的通信方式,统一了 restful 的标准,封装了 http 链接, 我们只需要传入 url 及返回值类型即可。
二、使用步骤
2.1 添加依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.2.9.RELEASE</version>
</dependency>
2.2 添加配置类
将RestTemplate 交给spring容器管理,方便直接使用
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.client.RestTemplate;
@Configuration
public class RestTemplateConfig {
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
2.3 使用案例
@RestController
@RequestMapping("/consumer/depart")
public class DepartController {
@Autowired
private RestTemplate restTemplate;
private static final String SERVICE_PROVIDER = "http://localhost:8081";
@PostMapping("/save")
public boolean saveHandle(@RequestBody DepartVO depart) {
String url = SERVICE_PROVIDER + "/provider/depart/save";
return restTemplate.postForObject(url, depart, Boolean.class);
}
@DeleteMapping("/del/{id}")
public void deleteHandle(@PathVariable("id") int id) {
String url = SERVICE_PROVIDER + "/provider/depart/del/" + id;
restTemplate.delete(url);
}
@PutMapping("/update")
public void updateHandle(@RequestBody DepartVO depart) {
String url = SERVICE_PROVIDER + "/provider/depart/update";
restTemplate.put(url, depart, Boolean.class);
}
@GetMapping("/get/{id}")
public DepartVO getHandle(@PathVariable("id") int id) {
String url = SERVICE_PROVIDER + "/provider/depart/get/" + id;
return restTemplate.getForObject(url, DepartVO.class);
}
@GetMapping("/list")
public List<DepartVO> listHandle() {
String url = SERVICE_PROVIDER + "/provider/depart/list/";
return restTemplate.getForObject(url, List.class);
}
}
2.4 测试
消费者调用http服务接口  服务端接收到请求 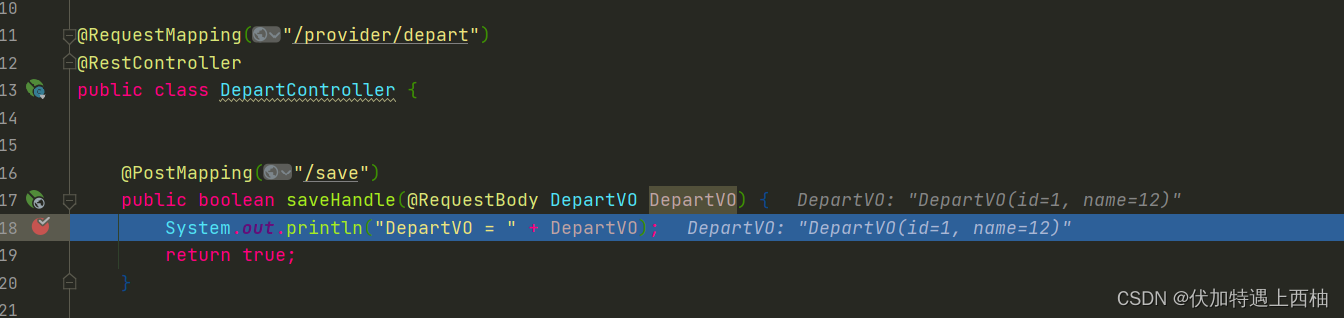
本文只是简单记录一下,无过多深入拓展
一文吃透接口调用神器RestTemplate RestTemplate全网最强总结(永久更新)
|