(1)在spring4之后,要使用注解开发,必须要保证aop的包导入了
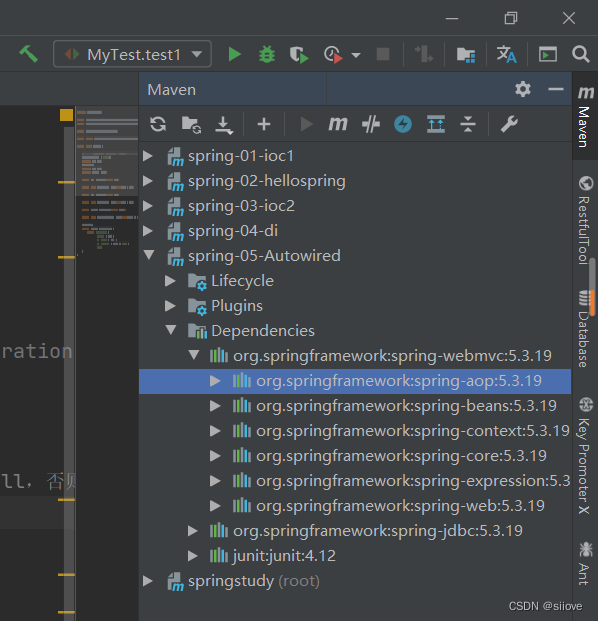
?
使用注解需要导入context约束,增加注解的支持!
精简版:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
<context:annotation-config/>
</beans>
扩展版:?
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
<context:annotation-config/>
<!--
指定要扫描的包,这个包下的注解就会生效
-->
<context:component-scan base-package="com.gt.pojo"/>
</beans>
(2)bean
?绿色的叶子表示放入组件当中!
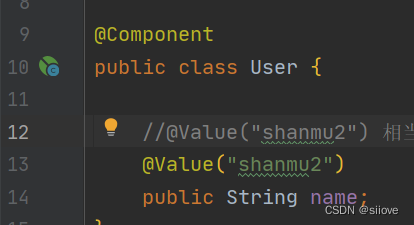
?
?User
package com.gt.pojo;
import org.springframework.stereotype.Component;
//@Component 等价于<bean id="user" class="com.gt.pojo.User"
//@Component 组件
@Component
public class User {
public String name = "山姆";
}
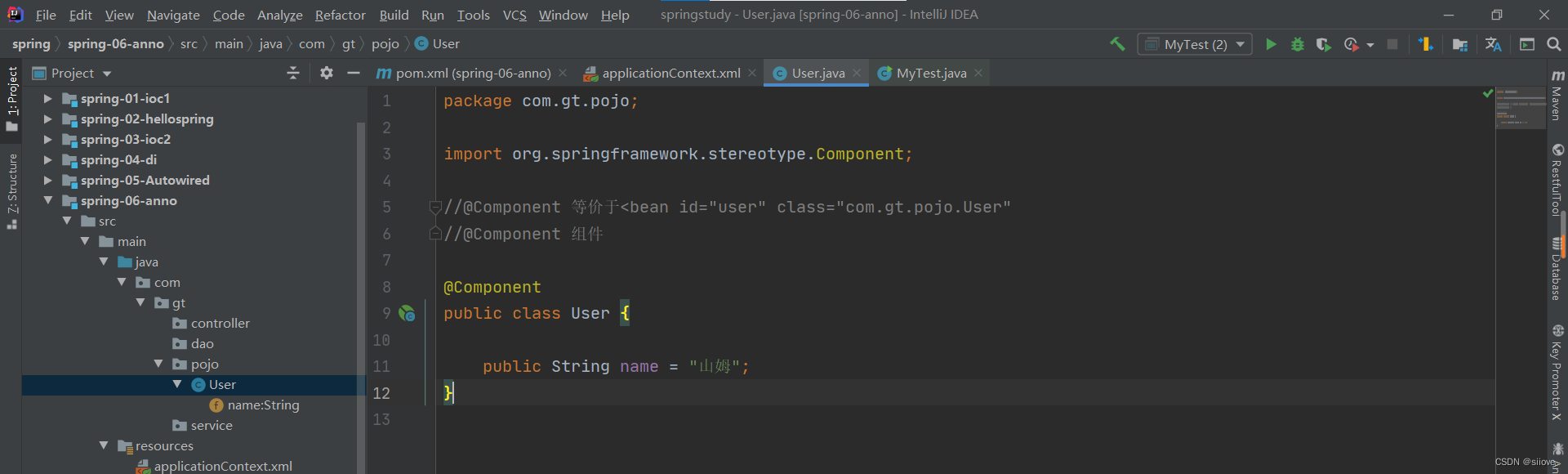
MyTest
import com.gt.pojo.User;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MyTest {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
//getBean括号里面的东西就是默认类的小写
User user = context.getBean("user",User.class);
System.out.println(user.name);
}
}
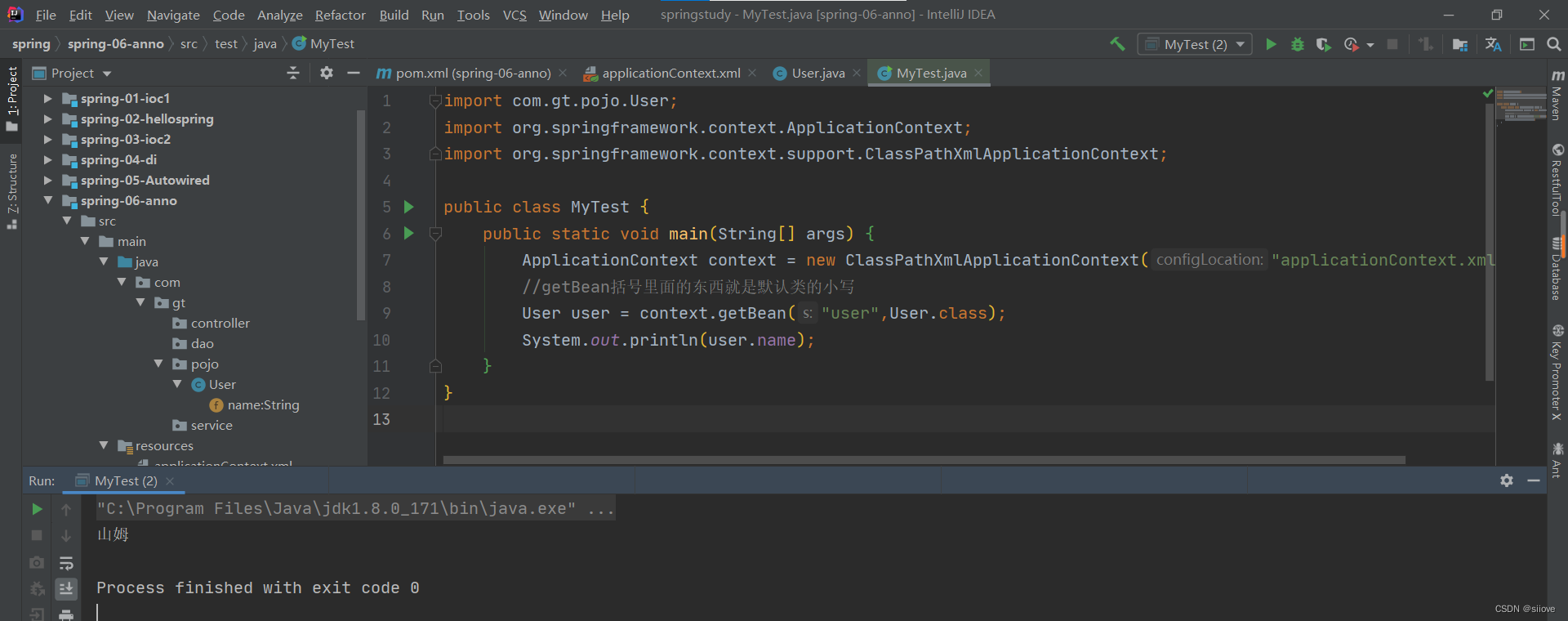
?
(3)属性如何注入
- @Component 等价于<bean id="user" class="com.gt.pojo.User"
- @Component 就是组件
- @Value("shanmu2") 相当于在beans.xml中<property name="name" value="shanmu2"/>
?
User
package com.gt.pojo;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
//@Component 等价于<bean id="user" class="com.gt.pojo.User"
//@Component 组件
@Component
public class User {
//@Value("shanmu2") 相当于在beans.xml中<property name="name" value="shanmu2"/>
public String name;
@Value("shanmu2")
public void setName(String name) {
this.name = name;
}
}
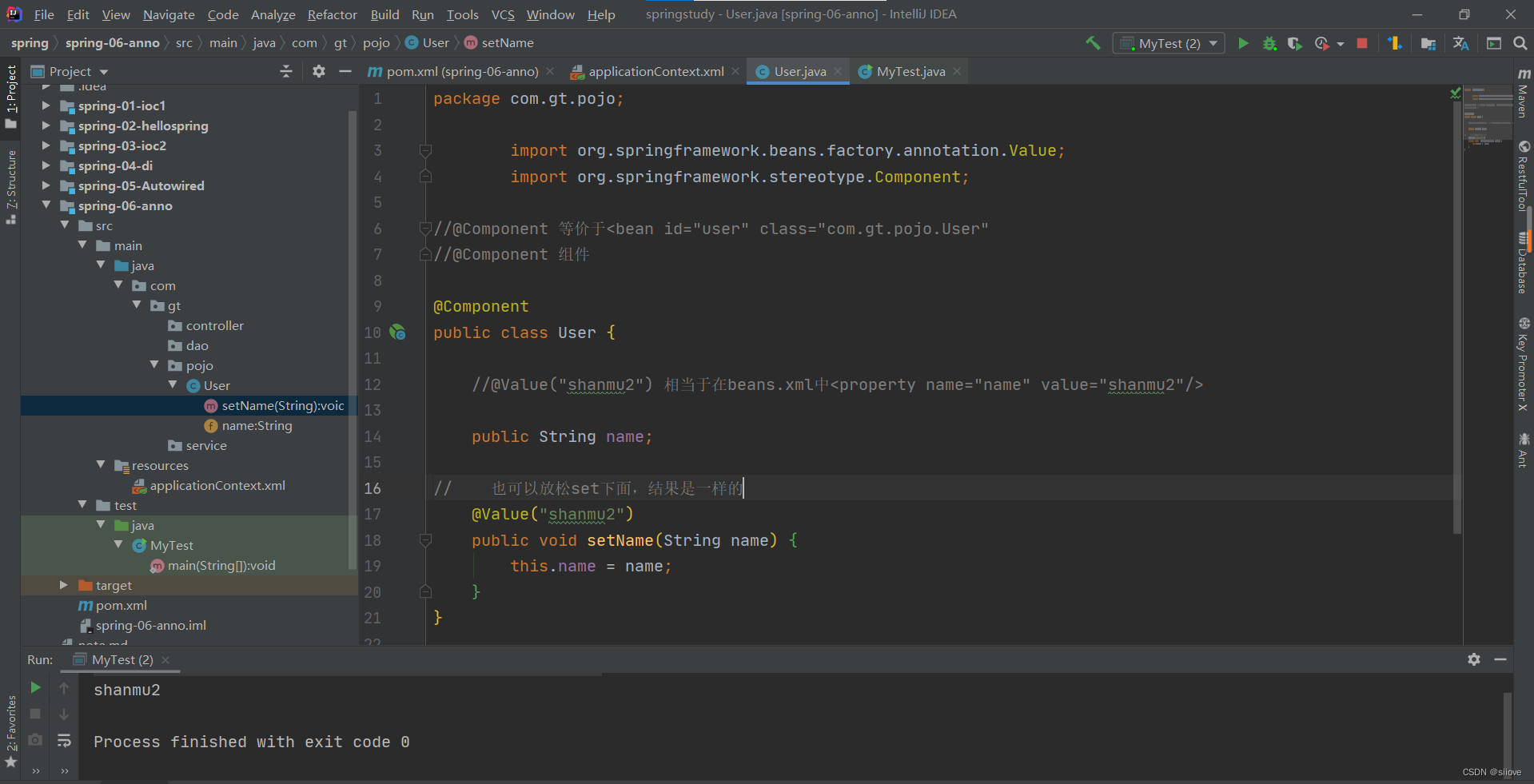
?
?扩展(Web):
1、衍生的注解
@Component有几个衍生注解,我们在web开发中,会按照mvc三层架构分层
?
2、自动装配
- @Autowired:自动装配通过类型。名字
- @Qualifier:如果Autowired不能唯一自动装配上属性,则需要通过@Qualifier(value='xxx')
- @Resource:自动装配通过名字。类型
- @Component 等价于<bean id="user" class="com.gt.pojo.User"/>
- @Scope("prototype") 这个等价于作用域
- @Value("山姆02")? * 相当于?<bean id="user" class="com.gt.pojo.User"><property name="name" value="山姆02"/>?</bean>
3、作用域
/**
* @Component 等价于<bean id="user" class="com.gt.pojo.User"/>
*
*/
@Component
// @Scope("prototype") 这个等价于作用域
@Scope("prototype")
public class User {
/**
* 相当于
* <bean id="user" class="com.gt.pojo.User">
* <property name="name" value="山姆02"/>
* </bean>
*/
// @Value("山姆02")
public String name;
@Value("shanmu02")
public void setName(String name) {
this.name = name;
}
}
小结
xml与注解:
-
xml更加万能,适用于任何场合,维护简单方便 -
注解不是自己的类使用不了,维护相对复杂
xml与注解最佳实践:
<context:annotation-config/>
<!--
指定要扫描的包,这个包下的注解就会生效
-->
<context:component-scan base-package="com.gt"/>
|