MyBatis-Plus
MyBatis-Plus官网链接:https://baomidou.com/
1. ORM介绍
- ORM (Object Relational Mapping,
对象关系映射 )是为了解决面向对象与关系数据库存在的互不匹配现象的一种技术。 - ORM通过使用描述对象和数据库之间映射的元数据将程序中的对象自动持久化到关系数据库中。
- ORM框架的本质是简化编程中操作数据库的编码。
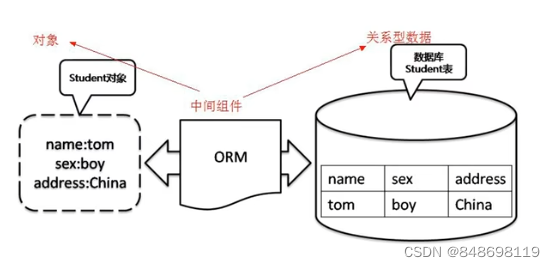
2. MyBatis-Plus介绍
- MyBatis是一款优秀的数据持久层ORM框架,被广泛地应用于应用系统。
- MyBatis能够非常灵活地实现动态SQL,可以使用XML或注解来配置和映射原生信息,能够轻松地将Java的POJO(Plain Ordinary Java Object,普通的Java对象)与数据库中的表和字段进行映射关联。
- MyBatis-Plus是一个
MyBatis的增强工具 ,在MyBatis的基础上做了增强,简化了开发。
2.1 引入依赖
<dependencies>
<!--SpringBoot框架web项目起步依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--mybatisPlus集成SpringBoot起步依赖-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.3.1</version>
</dependency>
<!--MySQL 驱动依赖-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!--druid 数据连接池-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.1.20</version>
</dependency>
</dependencies>
2.2 核心配置文件
#连接数据库
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/study?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=Asia/Shanghai
spring.datasource.username=root
spring.datasource.password=123456
#数据源配置 ———— druid
spring.datasource.type=com.alibaba.druid.pool.DruidDataSource
# 配置日志输出格式——————打印SQL日志
mybatis-plus.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
2.3 核心启动类上添加@MapperScan注解
@MapperScan("com.guo.springboot.mapper") 扫描器,指定扫描哪个包 指定扫描配置文件的包路径
3. MyBatis-Plus CRUD操作
3.1 映射接口——用注解实现CRUD
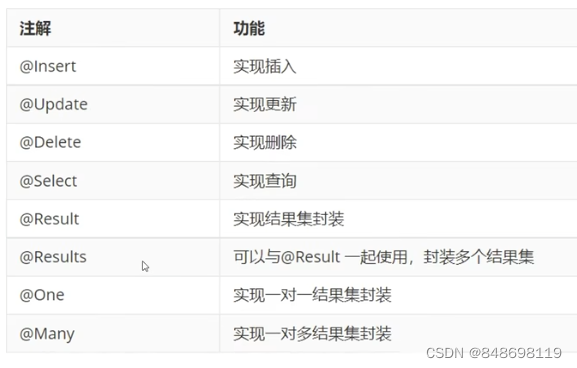 映射接口
package com.guo.springboot.mapper;
import com.guo.springboot.entity.User;
import org.apache.ibatis.annotations.*;
import java.util.List;
@Mapper
public interface UserMapper {
@Select("select * from user")
List<User> queryAllUser();
@Insert("Insert into user values (#{id}, #{name}, #{password}) ")
int insert(User user);
@Update("update user set name=#{name}, password=#{password} where id=#{id}")
int update(User user);
@Delete("delete from user where id=#{id}")
int delete(int id);
}
3.2 映射接口——继承BaseMapper<>实现CRUD
package com.guo.springboot.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.guo.springboot.entity.User;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface UserMapper extends BaseMapper<User> {
}
官网CRUD接口链接:https://baomidou.com/pages/49cc81/#update-3
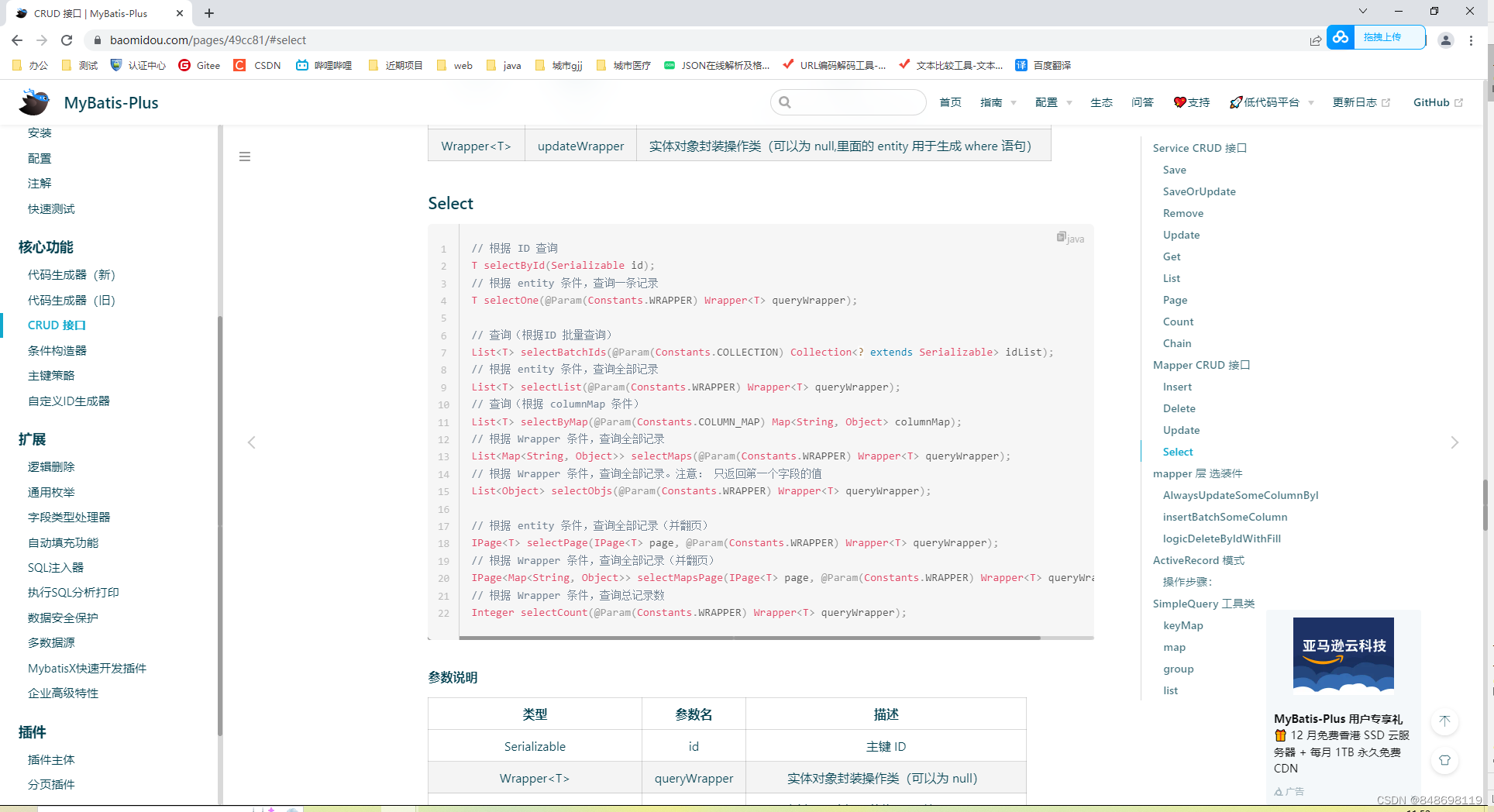
|