SpringBoot是由Privota团队提供的全新框架,其设计目的是用来简化Spring应用的初始搭建及开发过程 sping程序缺点
Springboot程序优点
- 自动配置
- 起步依赖(简化依赖配置)
- 辅助功能(内置服务器)
starter
- springboot中常见项目名称,定义了当前项目使用的所有项目坐标,以达到减少依赖配置的目的
parent
- 所有SpringBoot项目要继承的项目,定义了若干个坐标版本号(依赖管理,而非依赖),以达到减少依赖冲突的目的
实际开发
- 使用任意坐标时,仅书写GAV中的G和A,v由SpringBoot提供
- 如果发送坐标错误,在指定version(要小心版本冲突)
yaml
YAML(YAML Ain’t Markup Language) 一种数据序列化格式
- 优点:
容易阅读 容易与脚本语言交互 以数据为核心,重数据轻格式 - YAML文件扩展名
主流 .yml .yaml 语法规则 大小写铭感 属性层级关系使用多行描述,每行结尾使用冒号结束 使用缩进表示层级关系,同层级左侧对齐,只允许使用空格 属性值前面添加空格(属性名与属性之间使用冒号+空格作为分隔)
yaml读取数据
lesson: SpringBoot
server:
port: 80
enterprise:
name: itcast
age: 16
tel: 187909090
subject:
- java
- spring
- mvc
package com.shanks.controller;
import com.shanks.domain.Enterprise;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.env.Environment;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.sql.SQLOutput;
@RestController
@RequestMapping("/books")
public class BookController {
@Value("${lesson}")
private String lesson;
@Value("${server.port}")
private Integer port;
@Value("${enterprise.subject[0]}")
private String subject_00;
@Autowired
private Environment environment;
@Autowired
private Enterprise enterprise;
@GetMapping("/{id}")
public String getById(@PathVariable Integer id){
System.out.println(lesson);
System.out.println(port);
System.out.println(subject_00);
System.out.println("--=-=-=-===============-=-=-=-");
System.out.println(environment.getProperty("lesson"));
System.out.println(environment.getProperty("server.port"));
System.out.println(environment.getProperty("enterprise.age"));
System.out.println(environment.getProperty("enterprise.subject[1]"));
System.out.println("----------------");
System.out.println(enterprise);
return "hello springboot! ";
}
}
package com.shanks.domain;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
import java.util.Arrays;
@Component
@ConfigurationProperties(prefix = "enterprise")
public class Enterprise {
private String name;
private Integer age;
private String tel;
private String[] subject;
@Override
public String toString() {
return "Enterprise{" +
"name='" + name + '\'' +
", age=" + age +
", tel='" + tel + '\'' +
", subject=" + Arrays.toString(subject) +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
public String[] getSubject() {
return subject;
}
public void setSubject(String[] subject) {
this.subject = subject;
}
}
解决
自定义对象封装数据警告 加上依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
mybatisplus
- 无侵入:只做增强不做改变,不会对现有工程产生影响
- 强大的CURD操作:内置通用Mapper,少配置即可实现单表的CURD
- 支持lambda: 编写查询条件无需担心字段写错
- 支持主键自动生成
- 内置分页插件
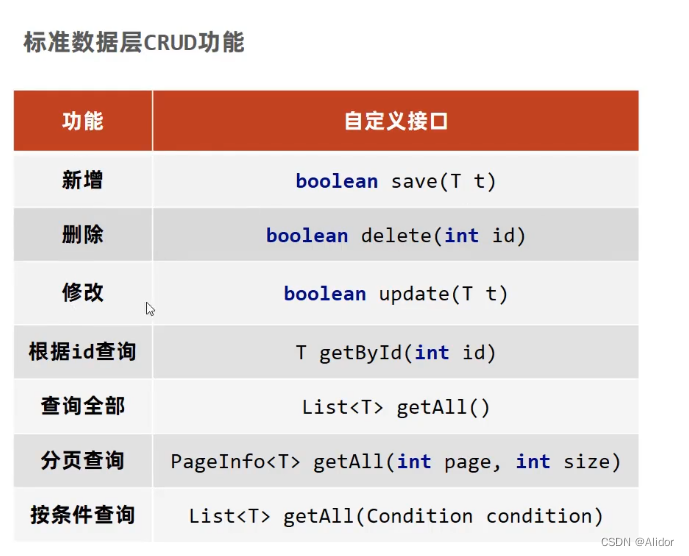 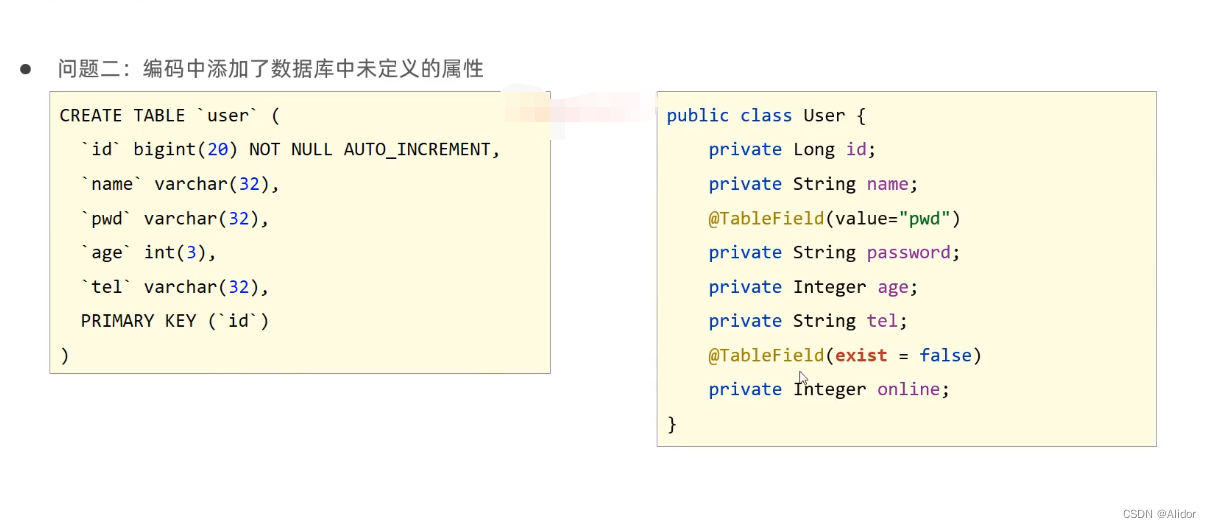 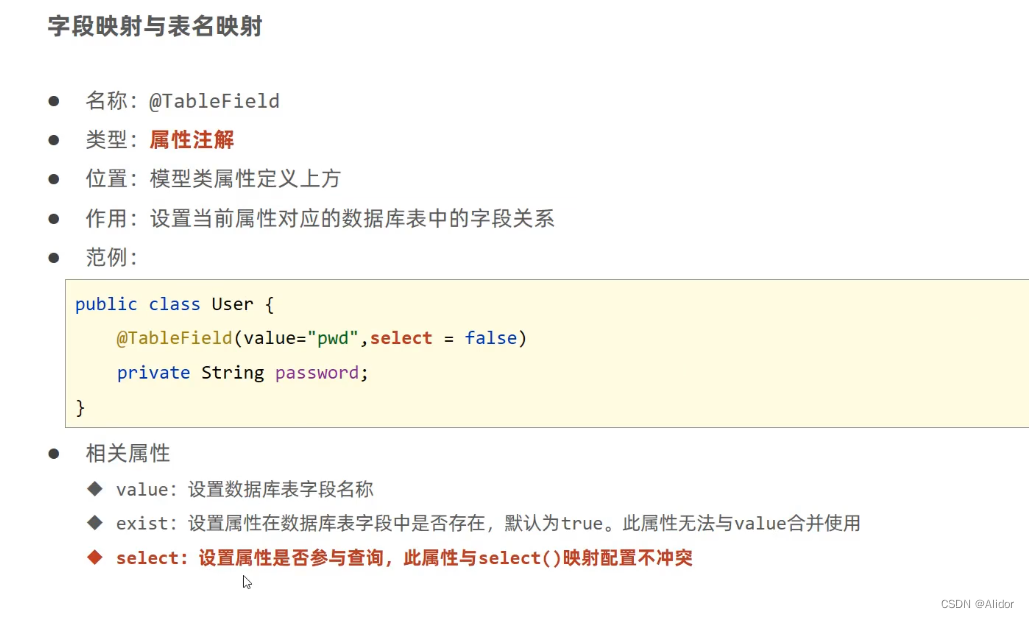 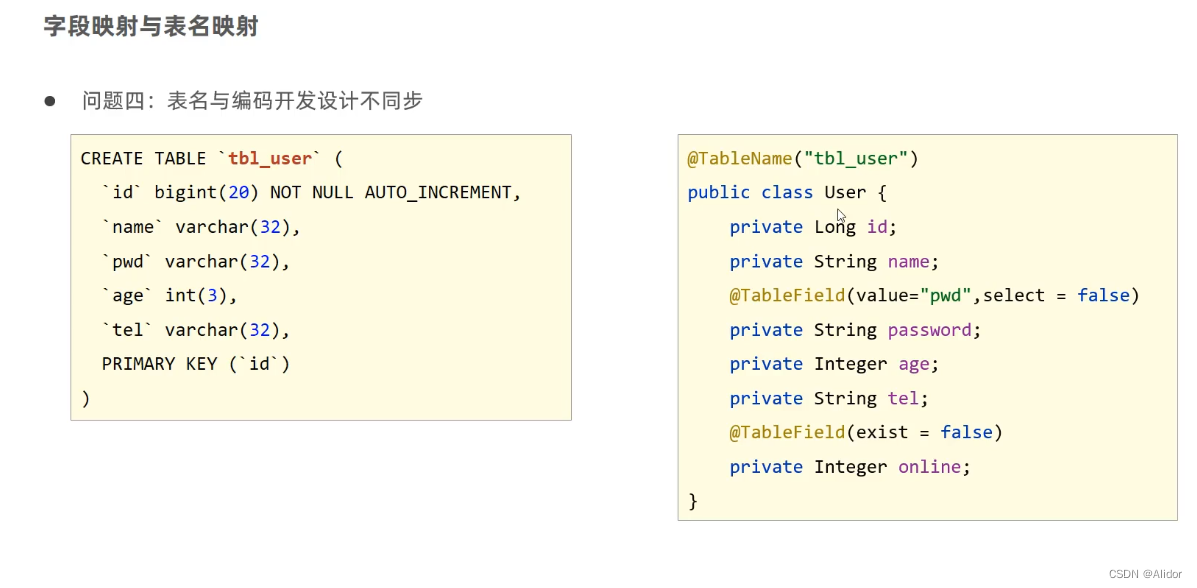 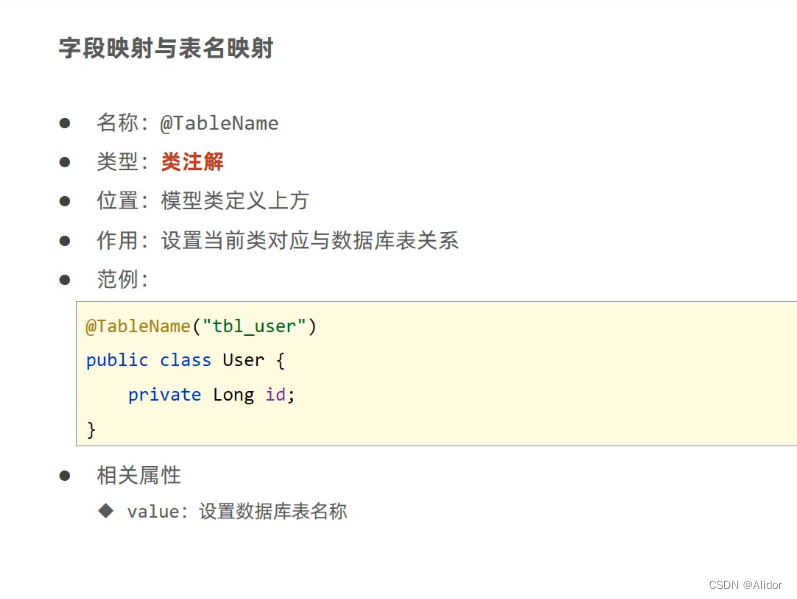 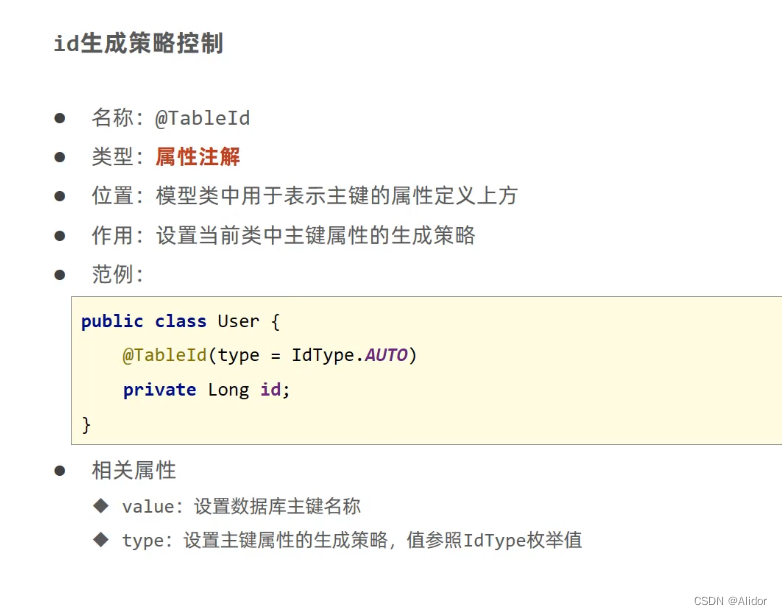 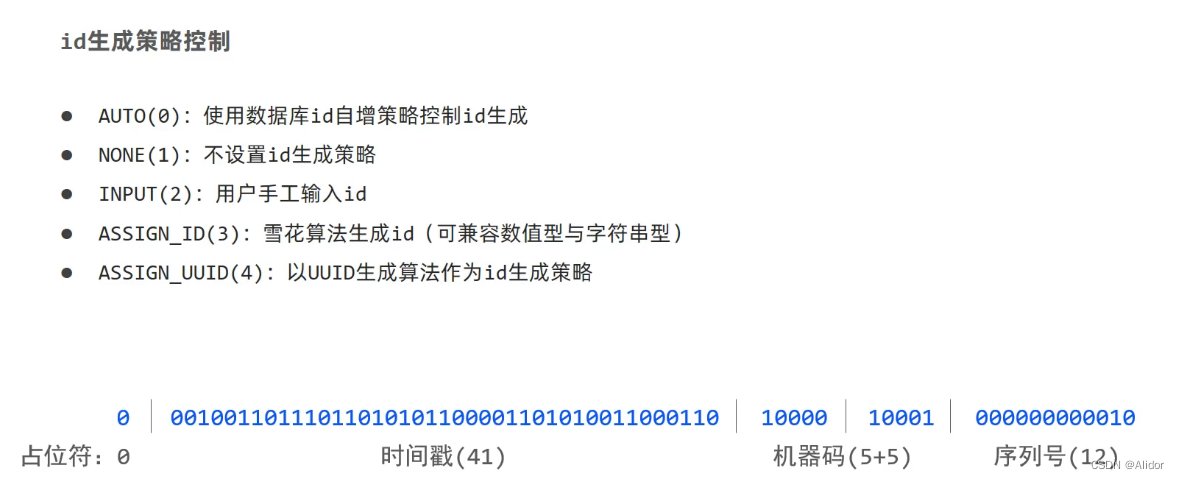 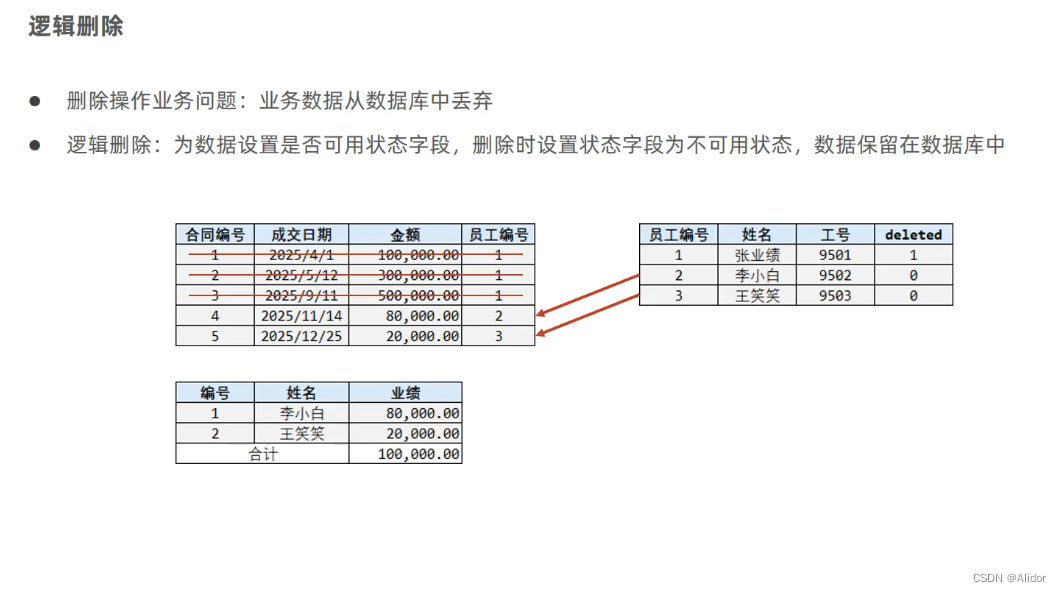
代码生成器
package com.shanks;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.generator.AutoGenerator;
import com.baomidou.mybatisplus.generator.config.DataSourceConfig;
import com.baomidou.mybatisplus.generator.config.GlobalConfig;
import com.baomidou.mybatisplus.generator.config.PackageConfig;
import com.baomidou.mybatisplus.generator.config.StrategyConfig;
public class CodeGenerator {
public static void main(String[] args) {
AutoGenerator autoGenerator = new AutoGenerator();
DataSourceConfig dataSource = new DataSourceConfig();
dataSource.setDriverName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/mybatispuls?serverTimezone=UTC");
dataSource.setUsername("root");
dataSource.setPassword("123456");
autoGenerator.setDataSource(dataSource);
GlobalConfig globalConfig = new GlobalConfig();
globalConfig.setOutputDir(System.getProperty("user.dir")+"/mybatisplus_generate/src/main/java");
globalConfig.setOpen(false);
globalConfig.setAuthor("shanks");
globalConfig.setFileOverride(true);
globalConfig.setMapperName("%sDao");
globalConfig.setIdType(IdType.ASSIGN_ID);
autoGenerator.setGlobalConfig(globalConfig);
PackageConfig packageInfo = new PackageConfig();
packageInfo.setParent("com.shanks");
packageInfo.setEntity("domain");
packageInfo.setMapper("dao");
autoGenerator.setPackageInfo(packageInfo);
StrategyConfig strategyConfig = new StrategyConfig();
strategyConfig.setInclude("tb_user");
strategyConfig.setTablePrefix("tb");
strategyConfig.setRestControllerStyle(true);
strategyConfig.setVersionFieldName("version");
strategyConfig.setLogicDeleteFieldName("deleted");
strategyConfig.setEntityLombokModel(true);
autoGenerator.setStrategy(strategyConfig);
autoGenerator.execute();
}
}
|