springboot 版本 2.7.3
1、依赖导入
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
</dependency>
因为springBoot做了jar包版本管理,所以不需要引入version标签
2、application.yaml配置
spring.mvc.converters.preferred-json-mapper: gson
3、GsonConfig
@Configuration
public class GsonConfig {
@Bean
public GsonBuilderCustomizer customizer() {
return b -> b
.setLongSerializationPolicy(LongSerializationPolicy.STRING)
.registerTypeAdapter(LocalDate.class, new LocalDateAdapter())
.registerTypeAdapter(LocalDateTime.class, new LocalDateTimeAdapter())
.setDateFormat("yyyy-MM-dd HH:mm:ss")
.serializeNulls()
.create();
}
public static class LocalDateAdapter extends TypeAdapter<LocalDate> {
@Override
public void write(final JsonWriter jsonWriter, final LocalDate localDate) throws IOException {
if (localDate == null) {
jsonWriter.nullValue();
} else {
jsonWriter.value(localDate.toString());
}
}
@Override
public LocalDate read(final JsonReader jsonReader) throws IOException {
if (jsonReader.peek() == JsonToken.NULL) {
jsonReader.nextNull();
return null;
} else {
return LocalDate.parse(jsonReader.nextString());
}
}
}
public static class LocalDateTimeAdapter extends TypeAdapter<LocalDateTime> {
@Override
public void write(final JsonWriter jsonWriter, final LocalDateTime localDate) throws IOException {
if (localDate == null) {
jsonWriter.nullValue();
} else {
jsonWriter.value(localDate.format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss")));
}
}
@Override
public LocalDateTime read(final JsonReader jsonReader) throws IOException {
if (jsonReader.peek() == JsonToken.NULL) {
jsonReader.nextNull();
return null;
} else {
return LocalDateTime.parse(jsonReader.nextString(), DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"));
}
}
}
}
4、测试
@Data
public class Book {
private Long id;
private String name;
private LocalDate publishDate;
private LocalDateTime createTime;
}
@RestController
@RequestMapping("/book")
public class IndexController {
@PostMapping
public Book save(@RequestBody Book book) {
return book;
}
@GetMapping
public Book get() {
Book book = new Book();
book.setId(1L);
book.setName("tcoding");
book.setCreateTime(LocalDateTime.now());
book.setPublishDate(LocalDate.now());
return book;
}
}
curl 127.0.0.1:8080/book
{"id":"1","name":"tcoding","publishDate":"2022-09-10","createTime":"2022-09-10 15:32:17"}%
curl -X POST 127.0.0.1:8080/book --header 'Content-Type: application/json' --data-raw '{"id":"1","name":"tcoding","publishDate":"2022-09-10","createTime":"2022-09-10 15:32:17"}'
{"id":"1","name":"tcoding","publishDate":"2022-09-10","createTime":"2022-09-10 15:32:17"}%
5、 SpringBoot Gson自动配置流程
GsonAutoConfiguration
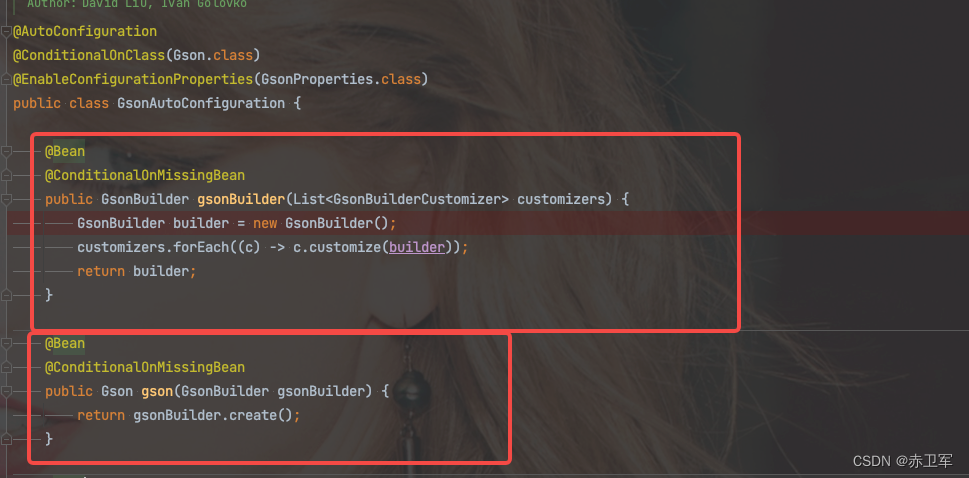
上面GsonConfig配置类里面
@Bean
public GsonBuilderCustomizer customizer() {
return b -> b
.setLongSerializationPolicy(LongSerializationPolicy.STRING)
.registerTypeAdapter(LocalDate.class, new LocalDateAdapter())
.registerTypeAdapter(LocalDateTime.class, new LocalDateTimeAdapter())
.setDateFormat("yyyy-MM-dd HH:mm:ss")
.serializeNulls()
.create();
}
是为了注入到GsonBuilder
根据 GsonBuilder生成Gson
GsonHttpMessageConvertersConfiguration
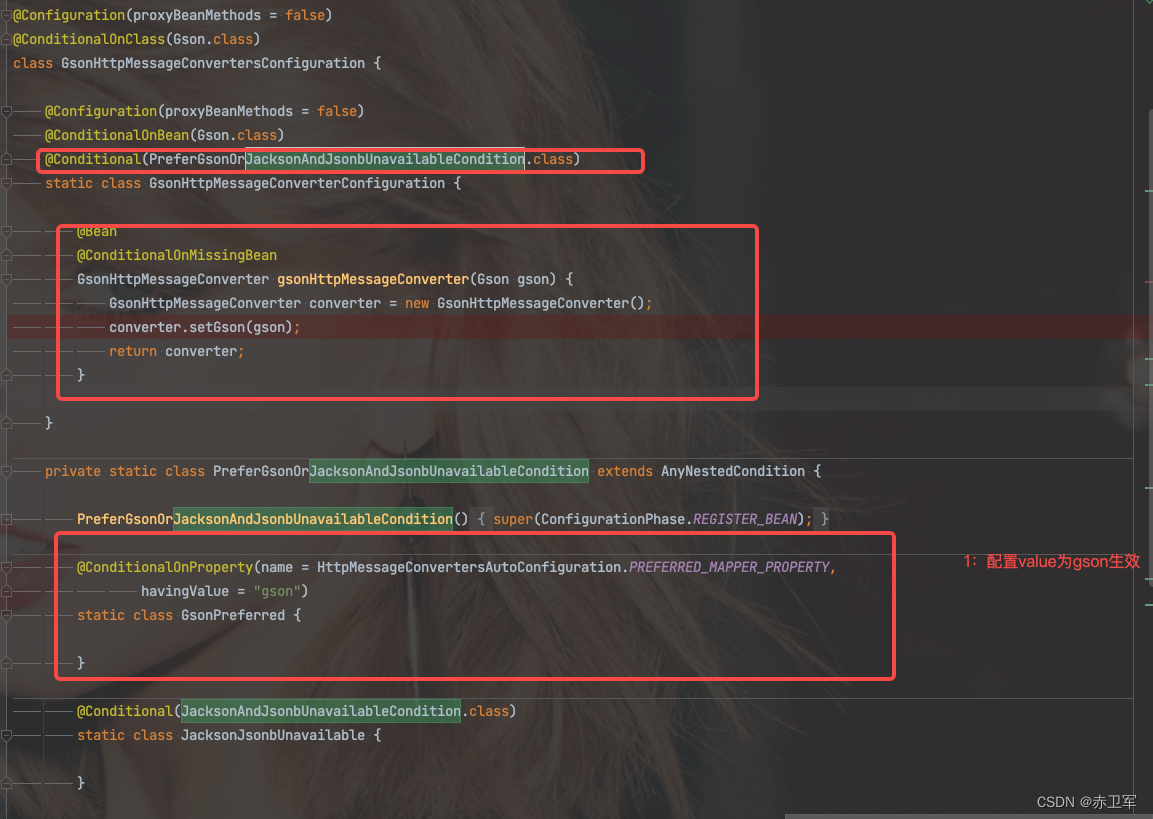  yaml配置 spring.mvc.converters.preferred-json-mapper的值为gson 则自动生成 GsonHttpMessageConverter bean
HttpMessageConvertersAutoConfiguration
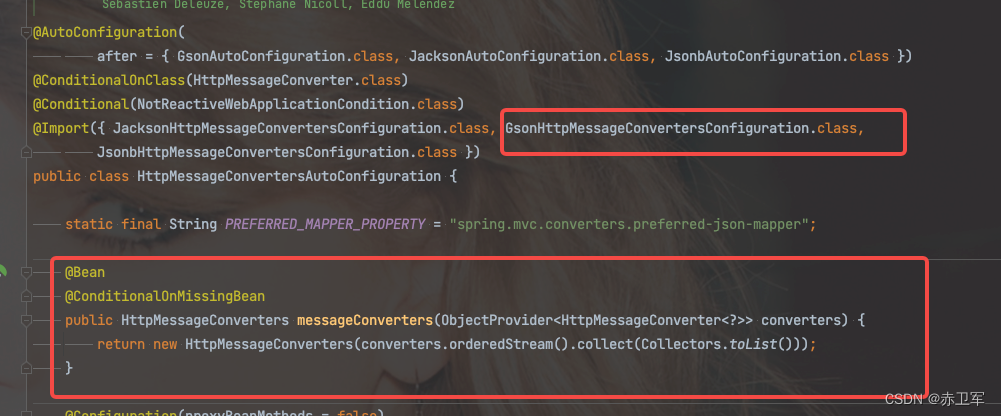
WebMvcAutoConfigurationAdapter
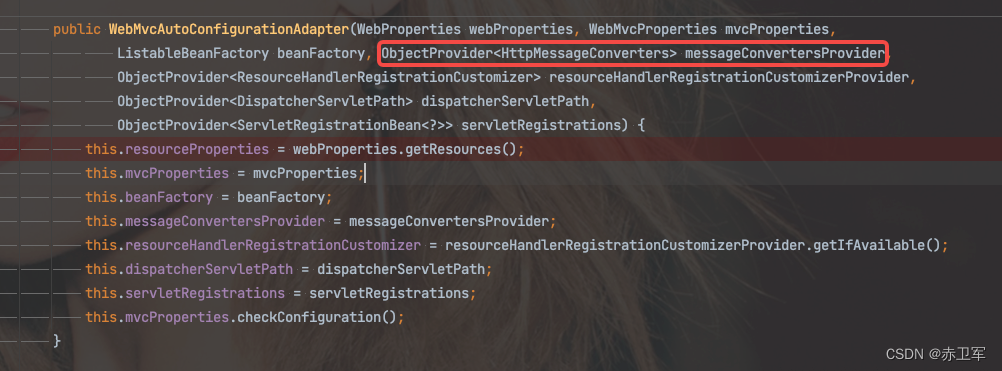
源码地址 https://github.com/googalAmbition/hello-spring-boot/tree/main/31-gson
|