创建springboot工程
Web->Spring Web SQL->MyBatis Framework SQL->MySQL Driver
建表
CREATE TABLE product(id INT PRIMARY KEY AUTO_INCREMENT,title VARCHAR(50),
price DOUBLE(10,2),num INT);
连接数据库
spring.datasource.url=jdbc:mysql://localhost:3306/empdb?characterEncoding=utf8&serverTimezone=Asia/Shanghai&useSSL=false
spring.datasource.username=root
spring.datasource.password=123456
前端页面
主页index.html
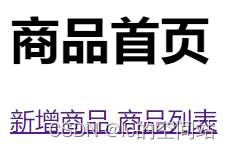
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<h1>商品首页</h1>
<a href="/insert.html">新增商品</a>
<a href="/list.html">商品列表</a>
</div>
</body>
</html>
商品新增insert.html

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<h1>商品首页</h1>
<input type="text" v-model="p.title" placeholder="请输入商品商品标题">
<input type="text" v-model="p.price" placeholder="请输入商品价格">
<input type="text" v-model="p.num" placeholder="库存数量">
<input type="button" value="提交" @click="insert()">
</div>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script src="https://cdn.staticfile.org/vue/2.2.2/vue.min.js"></script>
<script>
let v = new Vue({
el: "body>div",
data: {
p:{
title:"",
price:"",
num:""
}
},
methods:{
insert(){
axios.post("/insert",v.p).then(function (response) {
alert("添加成功!");
location.href="/";
})
}
}
})
</script>
</body>
</html>
商品列表
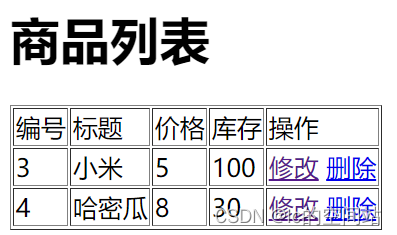
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<h1>商品列表</h1>
<table border="1">
<tr>
<td>编号</td>
<td>标题</td>
<td>价格</td>
<td>库存</td>
<td>操作</td>
</tr>
<tr v-for="p in arr">
<td>{{p.id}}</td>
<td>{{p.title}}</td>
<td>{{p.price}}</td>
<td>{{p.num}}</td>
<td>
<a :href="'/update.html?id='+p.id" >修改</a>
<a href="javascript:void(0)" @click="del(p.id)" >删除</a>
</td>
</tr>
</table>
</div>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script src="https://cdn.staticfile.org/vue/2.2.2/vue.min.js"></script>
<script>
let v = new Vue({
el: "body>div",
data: {
arr:[]
},
methods:{
del(id){
if(confirm("确认删除吗?")){
axios.get("/deleteById?id="+id).then(function (response) {
alert("删除成功!");
location.reload();
})
}
}
},
created:function () {
axios.get("/select").then(function (response) {
v.arr=response.data;
})
}
})
</script>
</body>
</html>
商品修改

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<h1>修改页面</h1>
<input type="text" v-model="p.id" placeholder="id" readonly>
<input type="text" v-model="p.title" placeholder="标题">
<input type="text" v-model="p.price" placeholder="价格">
<input type="text" v-model="p.num" placeholder="库存">
<input type="button" value="修改" @click="update()">
</div>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script src="https://cdn.staticfile.org/vue/2.2.2/vue.min.js"></script>
<script>
let v = new Vue({
el: "body>div",
data: {
p:{
id:"",
title:"",
price:"",
num:""
}
},
methods:{
update(){
axios.post("/update",v.p).then(function (response) {
alert("修改成功!");
location.href="list.html";
})
}
},
created:function () {
axios.get("/selectById"+location.search).then(function (response) {
v.p=response.data;
})
}
})
</script>
</body>
</html>
后端代码
实体类entity
public class Product {
private Integer id;
private String title;
private Double price;
private Integer num;
@Override
public String toString() {
return "Product{" +
"id=" + id +
", title='" + title + '\'' +
", price=" + price +
", num=" + num +
'}';
}
}
controller
@RestController
public class ProductController {
@Autowired(required = false)
ProductMapper mapper;
@RequestMapping("/insert")
public void insert(@RequestBody Product product){
System.out.println("product = " + product);
mapper.insert(product);
}
@RequestMapping("/select")
public List<Product> select(){
return mapper.select();
}
@RequestMapping("/deleteById")
public void delete(int id){
System.out.println("id = " + id);
mapper.delete(id);
}
@RequestMapping("/selectById")
public Product selectById(int id){
return mapper.selectById(id);
}
@RequestMapping("/update")
public void update(@RequestBody Product product){
mapper.update(product);
}
}
mapper
@Mapper
public interface ProductMapper {
@Insert("insert into product values(null,#{title},#{price},#{num})")
void insert(Product product);
@Select("select * from product")
List<Product> select();
@Delete("delete from product where id=#{id}")
void delete(int id);
@Select("select * from product where id=#{id}")
Product selectById(int id);
@Update("update product set title=#{title},price=#{price},num=#{num} where id=#{id}")
void update(Product product);
}
|