介绍:
kaptcha是Google提供的一个图形验证码插件,有了它,你可以通过简单的配置生成各种样式的验证码。
1:SpringBoot引入kaptcha的依赖
<dependency>
<groupId>com.github.penggle</groupId>
<artifactId>kaptcha</artifactId>
<version>2.3.2</version>
</dependency>
2:编写kaptcha配置类
package simulationvirtual.VirtualExperiment.config;
import com.google.code.kaptcha.Producer;
import com.google.code.kaptcha.impl.DefaultKaptcha;
import com.google.code.kaptcha.util.Config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.Properties;
@Configuration
public class KaptchaConfig {
/**
* Kaptcha图形验证码工具配置类
* @author: Xiongch
* @param:
* @return: com.google.code.kaptcha.Producer
* @date: 2022/9/9 15:47
*/
@Bean
public Producer kaptchaProducer() {
// 实例一个DefaultKaptcha
DefaultKaptcha defaultKaptcha = new DefaultKaptcha();
// 创建配置对象
Properties properties = new Properties();
// 设置边框
properties.setProperty("kaptcha.border", "yes");
// 设置颜色
properties.setProperty("kaptcha.border.color", "105,179,90");
// 设置字体颜色
properties.setProperty("kaptcha.textproducer.font.color", "blue");
// 设置宽度
properties.setProperty("kaptcha.image.width", "125");
// 高度
properties.setProperty("kaptcha.image.height", "50");
// 设置session.key
properties.setProperty("kaptcha.session.key", "code");
// 设置文本长度
properties.setProperty("kaptcha.textproducer.char.length", "4");
// 设置字体
properties.setProperty("kaptcha.textproducer.font.names", "宋体,楷体,微软雅黑");
// 将以上属性设置为实例一个DefaultKaptcha的属性
Config config = new Config(properties);
defaultKaptcha.setConfig(config);
// 将defaultKaptcha返回
return defaultKaptcha;
}
}
2.1:Kaptcha详细配置
Kaptcha详细配置表
序号 | 属性名 | 描述 | 示例 |
---|
1 | kaptcha.width | 验证码宽度 | 200 | 2 | kaptcha.height | 验证码高度 | 50 | 3 | kaptcha.border.enabled | 是否显示边框 | false | 4 | kaptcha.border.color | 边框颜色 | black | 5 | kaptcha.border.thickness | 边框厚度 | 2 | 6 | kaptcha.content.length | 验证码文本长度 | 5 | 7 | kaptcha.content.source | 文本源 | abcde2345678gfynmnpwx | 8 | kaptcha.content.space | 文本间隔 | 2 | 9 | kaptcha.font.name | 字体名称 | Arial | 10 | kaptcha.font.size | 字体大小 | 40 | 11 | kaptcha.font.color | 字体颜色 | black | 12 | kaptcha.background-color.from | 背景颜色(开始渐变色) | lightGray | 13 | kaptcha.background-color.to | 背景颜色(结束渐变色 | white |
3:编写Controller,实现验证码请求接口
package simulationvirtual.VirtualExperiment.controller.tools;
import com.google.code.kaptcha.Producer;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.util.FastByteArrayOutputStream;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import simulationvirtual.VirtualExperiment.util.AjaxResult;
import simulationvirtual.VirtualExperiment.util.Base64;
import simulationvirtual.VirtualExperiment.util.UUIDutil;
import javax.imageio.ImageIO;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
@RestController
public class KaptchaController {
@Autowired
private RedisTemplate<String,Object> redisTemplate;
@Autowired
private Producer kaptchaProduer;
/**
* 生成图形验证码
* @author: Xiongch
* @param:null
* @return:Ajax_Result(统一返回工具)
* @date: 2022/9/9 15:47
*/
@GetMapping("/kaptcha")
public AjaxResult getKaptcha(HttpServletResponse response, HttpSession session){
AjaxResult Ajax_Result = AjaxResult.success();
String imagecode = kaptchaProduer.createText();
// 生成图片
BufferedImage image = kaptchaProduer.createImage(imagecode);
// 将验证码存入Session
session.setAttribute("kaptcha",imagecode);
//将图片输出给浏览器
String uuid = UUIDutil.getUUID();//uuid-->验证码唯一标识
FastByteArrayOutputStream os = new FastByteArrayOutputStream();
try {
response.setContentType("image/png");
ImageIO.write(image,"png",os);
//验证码实现redis缓存,过期时间2分钟
session.setAttribute("uuid",imagecode);
redisTemplate.opsForValue().set(uuid,imagecode,2, TimeUnit.MINUTES);
} catch (IOException e) {
return AjaxResult.error(e.getMessage());
}
Ajax_Result.put("uuid",uuid);
Ajax_Result.put("img", Base64.encode(os.toByteArray()));
return Ajax_Result;
}
}
4:vue前端展示代码
<div class="login-code">
<img :src="codeUrl" @click="getCode" class="login-code-img"/>
</div>
?4.1:点击刷新方法
methods: {
getCode() {
this.$axios({
method:'GET',
url:"/kaptcha",
headers: {
"Content-Type": "application/json"
}
}).then(res=>{
this.codeUrl = "data:image/gif;base64," + res.data.img;
})
},
5:效果如下
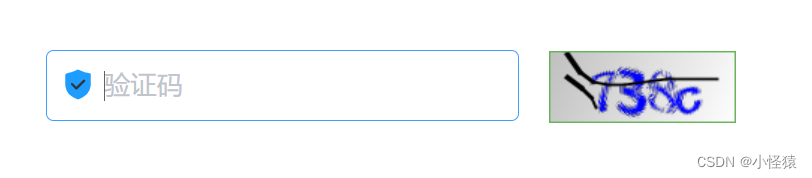
|