学习视频指路–>【狂神说Java】一小时掌握Swagger
Swagger的作用
1、通过Swagger给一些比较难理解的属性或者接口增加注释信息 2、接口文档实时更新 3、可以在线测试
1、创建springboot-web项目 2、导入相关依赖–>maven仓库查询相关依赖
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
3、配置Swagger 1>创建Swagger的配置类,开启 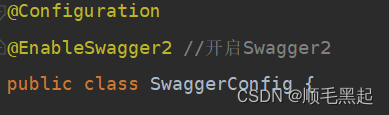
Swagger的bean实例Docket;
package com.tiki.swagger.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
import java.util.ArrayList;
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket docket(){
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo());
}
private ApiInfo apiInfo(){
Contact contact = new Contact("TiTi","https://www.csdn.net/","12345@123.com");
return new ApiInfo(
"Swagger的Api文档",
"对Api文档的描述",
"1.0",
"https://www.csdn.net/",
contact,
"Apache 2.0",
"http://www.apache.org/licenses/LICENSE-2.0",
new ArrayList()
);
}
}
Swagger配置扫描接口
Docket.select()
@Bean
public Docket docket(){
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.basePackage("com.tiki.swagger.controller"))
.paths(PathSelectors.ant("/titi/**"))
.build();
}
配置是否启动Swagger
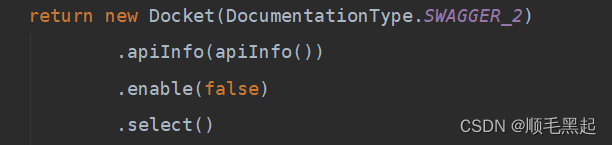
指定在哪个环境中使用Swagger(开发、测试、发布)
1、先模拟配置两个配置类,一个是开发的,一个是发布的,指定各自的端口号
application-dev.yml
server:
port: 8081
application-pro.yml
server:
port: 8082
在application.yml中指定激活的开发环境
spring:
profiles:
active: dev
2、在SwaggerConfig类中
@Bean
public Docket docket(Environment environment){
Profiles profiles=Profiles.of("dev","test");
boolean flag = environment.acceptsProfiles(profiles);
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.enable(flag)
.select()
.apis(RequestHandlerSelectors.basePackage("com.tiki.swagger.controller"))
.build();
}
配置API文档的分组
.groupName("TiKi")
配置多个分组,使用多个Docket实例即可
@Bean
public Docket docket1(){
return new Docket(DocumentationType.SWAGGER_2).groupName("A");
}
@Bean
public Docket docket2(){
return new Docket(DocumentationType.SWAGGER_2).groupName("B");
}
@Bean
public Docket docket3(){
return new Docket(DocumentationType.SWAGGER_2).groupName("C");
}
实体类的API注解
@ApiModel("用户实体类")
public class User {
@ApiModelProperty("用户名")
public String username;
@ApiModelProperty("密码")
public String password;
}
控制类的API注解
@RestController
public class HelloController {
@GetMapping(value = "/hello")
public String hello(){
return "hello";
}
@PostMapping(value = "/user")
public User user(){
return new User();
}
@ApiOperation("Hello控制类")
@GetMapping(value = "/hello2")
public String hello2(@ApiParam("用户名") String username){
return "hello"+username;
}
@ApiOperation("Post测试类")
@PostMapping(value = "/postt")
public User postt(@ApiParam("用户") User user){
return user;
}
}
|