Ribbon案例
需要准备几个节点,上面部署服务C
可以复制服务A 修改成Service-C9004 Service-C9005 注意pom.xml application.yml 请求中的数据
修改项目的pom.xml
准备服务C
Service-C9004
》1:修改服务端口,使用服务名Service-C
server:
port: 9004
spring:
application:
name: Service-C
eureka:
instance:
prefer-ip-address: true #使用ip地址注册
instance-id: ${spring.cloud.client.ip-address}:${server.port} #向注册中心中注册服务id
lease-renewal-interval-in-seconds: 5 #向注册中心中注册服务id
lease-expiration-duration-in-seconds: 10 #续约到期的时间
client:
service-url:
defaultZone: http://localhost:9000/eureka/,http://localhost:8000/eureka/
》2:准备Controller
@RestController
@RequestMapping("/person")
public class PersonController {
@RequestMapping(method = RequestMethod.GET,value = "/find/{id}")
public Person find(@PathVariable Long id){
Person person = new Person();
person.setId(id);
person.setName("我是服务A的根据id查询Person信息 负载均衡9004");
return person;
}
}
测试 http://localhost:9004/person/find/1
Service-C9005
参考上面 http://localhost:9005/person/find/1
编写消费
Cosumer-C9003
》1:增加Controller 必须使用服务名称,才可以进行负载均衡的算法选择,否则就是直接访问
@RestController
@RequestMapping("/useServiceC")
public class UseServiceCController {
@Autowired
private RestTemplate restTemplate ;
@RequestMapping(method = RequestMethod.GET,value = "/get/{id}")
public Person getByID(@PathVariable Long id){
//当前的方法要去消息服务C
//http://localhost:9004/person/find/1
//http://localhost:9005/person/find/1
//必须使用服务名称,才可以进行负载均衡的算法选择,否则就是直接访问
Person person = restTemplate.getForObject("http://Service-C/person/find/"+id,Person.class);
return person;
}
}
》2:配置负载均衡@LoadBalanced
@SpringBootApplication
//激活eurekaClient
@EnableEurekaClient
//@EnableDiscoveryClient
public class C9003 {
//创建RestTemplate,由@Bean将实例放到spring容器里面
@Bean
@LoadBalanced
RestTemplate restTemplate(){
RestTemplate r = new RestTemplate();
return r;
}
public static void main(String[] args) {
SpringApplication.run(C9003.class,args);
}
}
》3:测试http://localhost:9003/useServiceC/get/1 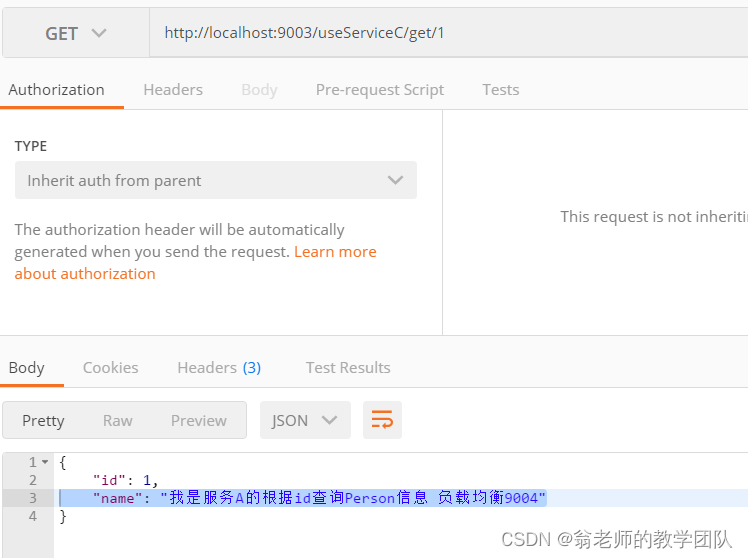 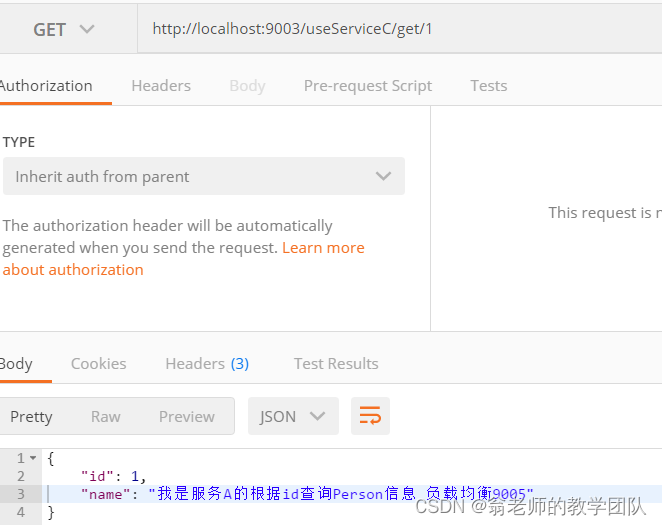
默认使用轮询算法
案例:负载均衡算法
(4)负载均衡策略
Ribbon内置了多种负载均衡策略,内部负责复杂均衡的顶级接口为
com.netflix.loadbalancer.IRule ,实现方式如下
com.netflix.loadbalancer.RoundRobinRule :以轮询的方式进行负载均衡。
com.netflix.loadbalancer.RandomRule :随机策略
com.netflix.loadbalancer.RetryRule :重试策略。
com.netflix.loadbalancer.WeightedResponseTimeRule :权重策略。会计算每个服务的权重,越高的被调用的可能性越大。
com.netflix.loadbalancer.BestAvailableRule :最佳策略。遍历所有的服务实例,过滤掉故障实例,并返回请求数最小的实例返回。
com.netflix.loadbalancer.AvailabilityFilteringRule :可用过滤策略。过滤掉故障和请求数超过阈值的服务实例,再从剩下的实力中轮询调用。
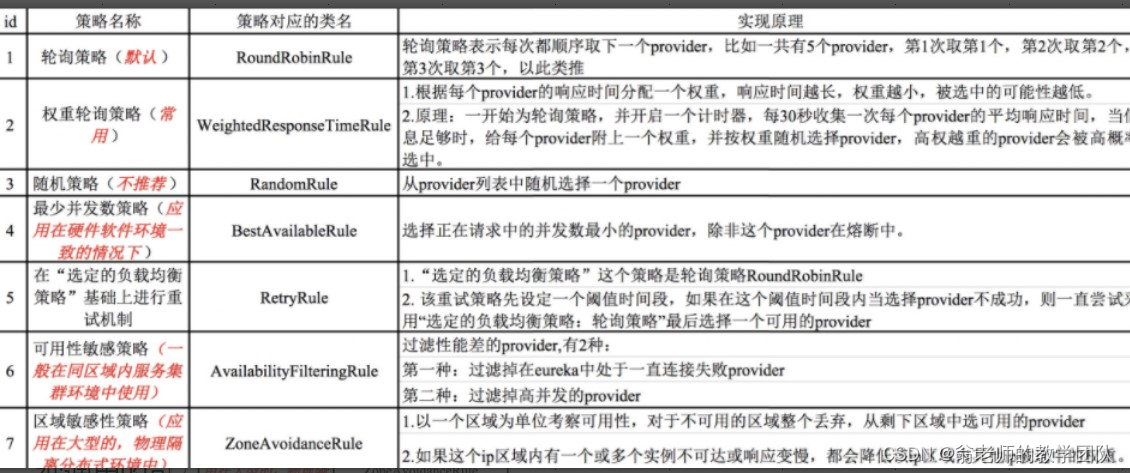
在消费端进行配置
Service-C:
ribbon:
NFLoadBalancerRuleClassName: com.netflix.loadbalancer.RandomRule
案例:重试配置
1 pom.xml添加依赖
<dependency>
<groupId>org.springframework.retry</groupId>
<artifactId>spring-retry</artifactId>
</dependency>
2 applicaiton.yml配置
Service-C:
ribbon:
NFLoadBalancerRuleClassName: com.netflix.loadbalancer.RandomRule
ConnectTimeout: 250 # Ribbon的连接超时时间
ReadTimeout: 1000 # Ribbon的数据读取超时时间
OkToRetryOnAllOperations: true # 是否对所有操作都进行重试
MaxAutoRetriesNextServer: 1 # 切换实例的重试次数
MaxAutoRetries: 1 # 对当前实例的重试次数
Feign案例
测试下ServiceA服务是否正常
测试下 服务A接口是否正常
项目名:Service-A9001 http://localhost:9001/person/find/1
Feign入门案例
》1:导入依赖
<!--springcloud整合的openFeign-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
》2:配置调用接口
/**
* FeignClient声明需要调用的微服务名称
* name服务提供者的名称
*/
//1:指定服务名,不使用ip,端口
@FeignClient(name="Service-A9001")
public interface PersonServiceAFeign {
@RequestMapping(method = RequestMethod.GET,value = "person/find/{id}")
public Person findById(@PathVariable Long id);
}
//2:当前只是一个接口,由框架实现本接口
》3:在启动类上激活Feign
//激活Feign
@EnableFeignClients
public class ServiceBApplication
》4:通过自动的接口调用远程微服务
@RestController
@RequestMapping("/useFeign")
public class UseFeignController {
@Autowired
PersonServiceAFeign feign;
@RequestMapping(method = RequestMethod.GET,value = "/get/{id}")
public Person getByID(@PathVariable Long id){
Person person = feign.findById(id);
return person;
}
}
Feign负载均衡
默认开启,使用轮询
访问服务C,没有添加额外设置 如果服务名下面有多个相同的服务,feign可以按算法来访问。
//1:指定服务名,不使用ip,端口
@FeignClient(name="Service-C")
public interface PersonServiceCFeign {
@RequestMapping(method = RequestMethod.GET,value = "person/find/{id}")
public Person findById(@PathVariable Long id);
}
//2:当前只是一个接口,由框架实现本接口
Feign数据压缩
feign:
compression:
request:
enabled: true # 开启请求压缩
response:
enabled: true # 开启响应压缩
同时,我们也可以对请求的数据类型,以及触发压缩的大小下限进行设置:
feign:
compression:
request:
enabled: true # 开启请求压缩
mime-types: text/html,application/xml,application/json # 设置压缩的数据类型
min-request-size: 2048 # 设置触发压缩的大小下限
日志级别
添加日志配置
#配置feign日志的输出
#日志配置
#NONE : 不输出日志(高)
#BASIC: 适用于生产环境追踪问题
#HEADERS : 在BASIC的基础上,记录请求和响应头信息
#FULL : 记录所有
feign:
client:
config:
Service-C:
loggerLevel: FULL
compression:
request:
enabled: true # 开启请求压缩
mime-types: text/html,application/xml,application/json # 设置压缩的数据类型
min-request-size: 2048 # 设置触发压缩的大小下限
logging:
level:
com.dev1.feign.PersonServiceCFeign: debug
|