1. IOC的概念和原理
1. 什么是IOC
- 控制反转,把对象创建和对象之间的调用过程,交给Spring管理
- 使用IOC目的:为了耦合度降低
- 入门案例就是IOC实现
2. IOC底层原理
技术:xml + 工厂模式 + 反射
举个栗子:两个方法之间的调用
传统模式 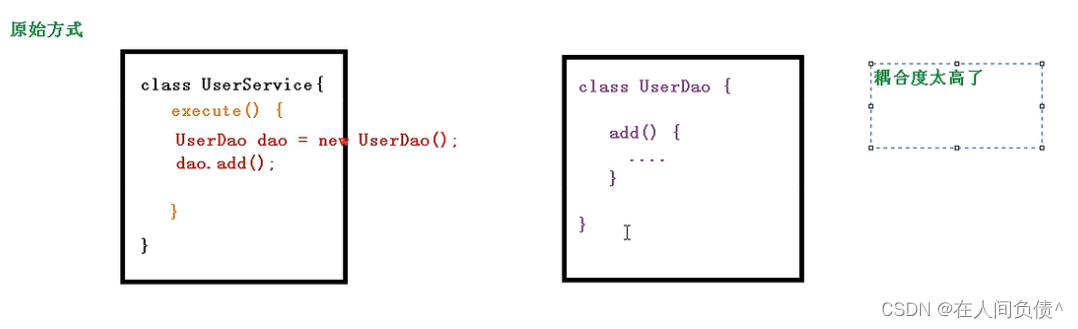
工厂模式 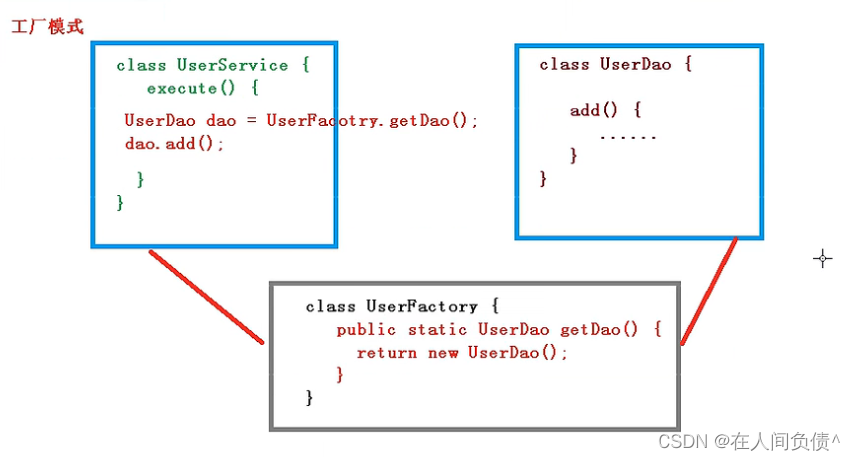
IOC过程 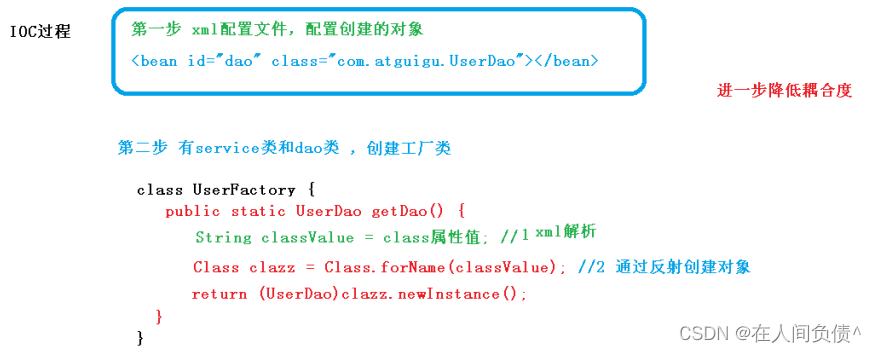
2. IOC的BeanFactory接口
-
IOC 思想基于 IOC 容器完成,IOC 容器底层就是对象工厂 -
Spring 提供了 IOC 容器实现两种方式:(两个接口)
- BeanFactor:IOC 容器基本实现,是 Spring 内部的使用接口,一般不提供开发人员进行使用(加载配置文件的时候,不会创建对象,在获取对象(使用)才会创建对象)
- ApplicationContext:BeanFactory 接口的子接口,比 BeanFactory 提供了更多更强大的功能,一般由开发人员进行使用(加载配置文件时候,就会创建对象)
-
ApplicationContext 接口有实现类 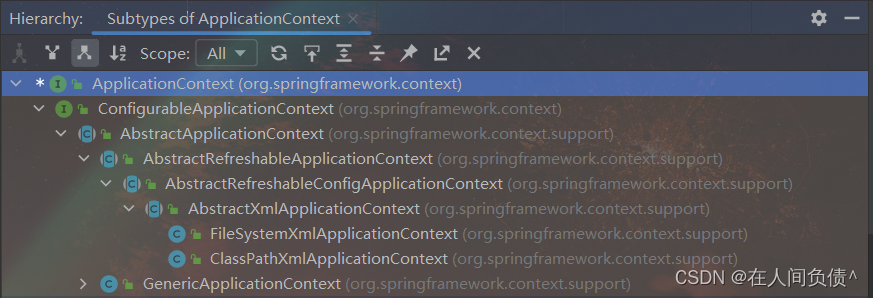
3. IOC操作Bean管理(概念)
1. 什么是Bean管理
Bean 管理指的是两个操作:
- Spring 创建对象
- Spring 注入属性
2. Bean管理操作有两种方式
- 基于 xml 配置文件方式实现
- 基于注解方式实现
4. IOC操作Bean管理(基于xml方式)
1. 基于xml方式创建对象
<bean id="user" class="com.fickler.spring5.User"></bean>
-
在 spring 配置文件中,使用 bean 标签,标签里面添加对应属性,就可以实现对象创建 -
在 bean 标签有很多属性,介绍常用属性
- id 属性:唯一标识(别名)
- class 属性:创建对象所在位置的全路径
-
创建对象时候,默认也是执行无参数构造方法完成对象创建
2. 基于xml方式注入属性
DI:依赖注入,就是注入属性(要在创建对象的基础之上注入属性)
3. 第一种注入方式:使用set方法进行注入
- 创建类,定义属性和对应的 set 方法
package com.fickler.spring5;
public class Book {
private String name;
private String author;
public void setName(String name) {
this.name = name;
}
public void setAuthor(String author) {
this.author = author;
}
public void testDemo(){
System.out.println(name + "::" + author);
}
}
- 在 spring 配置文件配置对象创建,配置属性注入
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="book" class="com.fickler.spring5.Book">
<property name="name" value="易筋经"></property>
<property name="author" value="达摩老祖"></property>
</bean>
</beans>
- 测试输出
package com.fickler.spring5.testdemo;
import com.fickler.spring5.Book;
import com.fickler.spring5.User;
import org.junit.jupiter.api.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class TestSpring5 {
@Test
public void testAdd(){
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("bean1.xml");
Book book = applicationContext.getBean("book", Book.class);
System.out.println(book);
book.testDemo();
}
}
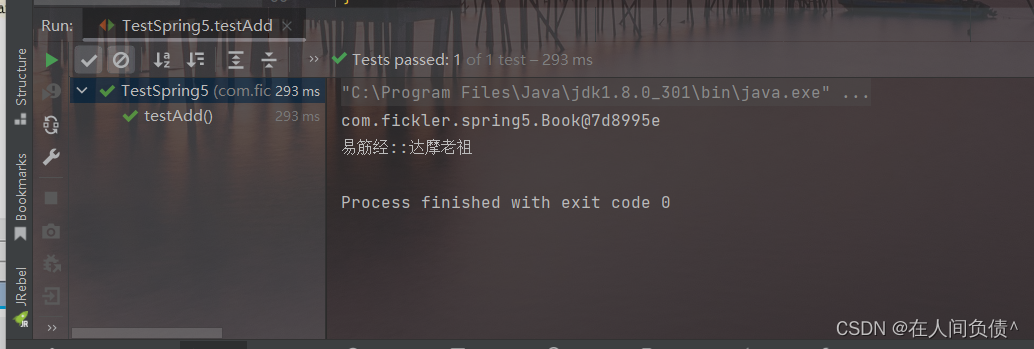
4. 第二种注入方式:使用有参数构造进行注入
- 创建类,定义属性,创建属性对应有参数构造方法
package com.fickler.spring5;
public class Orders {
private String name;
private String address;
public Orders(String name, String address) {
this.name = name;
this.address = address;
}
public void ordersTest(){
System.out.println(name + "::" + address);
}
}
- 在 spring 配置文件中进行配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="orders" class="com.fickler.spring5.Orders">
<constructor-arg name="name" value="电脑"></constructor-arg>
<constructor-arg name="address" value="中国"></constructor-arg>
</bean>
</beans>
- 测试输出
package com.fickler.spring5.testdemo;
import com.fickler.spring5.Book;
import com.fickler.spring5.Orders;
import com.fickler.spring5.User;
import org.junit.jupiter.api.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class TestSpring5 {
@Test
public void testAdd(){
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("bean1.xml");
Orders orders = applicationContext.getBean("orders", Orders.class);
System.out.println(orders);
orders.ordersTest();
}
}
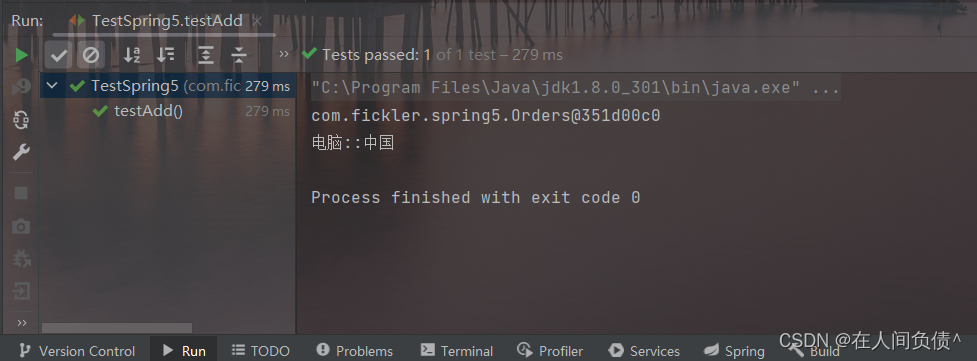
5. p名称空间注入(了解)
使用 p 名称空间注入,可以简化基于 xml 配置方式
- 添加 p 名称空间在配置文件中
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
- 进行属性注入,在 bean 标签里面进行操作
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="book" class="com.fickler.spring5.Book" p:name="九阳神功" p:author="无名氏"></bean>
</beans>
- 测试输出
package com.fickler.spring5.testdemo;
import com.fickler.spring5.Book;
import com.fickler.spring5.Orders;
import com.fickler.spring5.User;
import org.junit.jupiter.api.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class TestSpring5 {
@Test
public void testAdd(){
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("bean1.xml");
Book book = applicationContext.getBean("book", Book.class);
System.out.println(book);
book.testDemo();
}
}
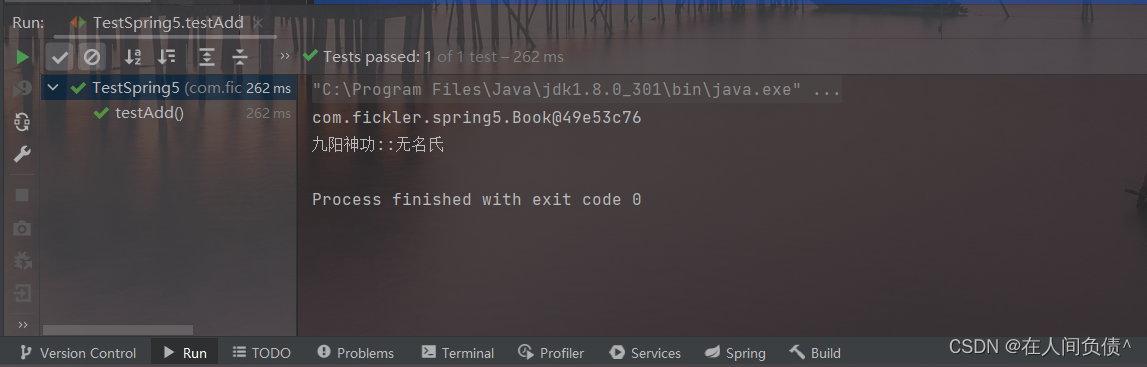
|