开发工具:IDEA
服务器:Tomcat9.0, jdk1.8
项目构建:maven
数据库:mysql5.7
系统分前后台,采用前后端分离
前端技术:vue+elementUI等框架实现
服务端技术:springboot+jpa
项目功能描述:
1.登录、注册、首页
2.用户管理:添加用户、修改用户、删除用户、查询用户、分配角色等功能
3.权限管理:
(1)角色列表:添加、修改、删除、分配权限
(2)权限列表
4.文件管理
(1)文件上传
(2)文件列表:分享文件、详情、下载文件、删除文件
5.个人中心:修改个人信息和修改密码
6.退出登录
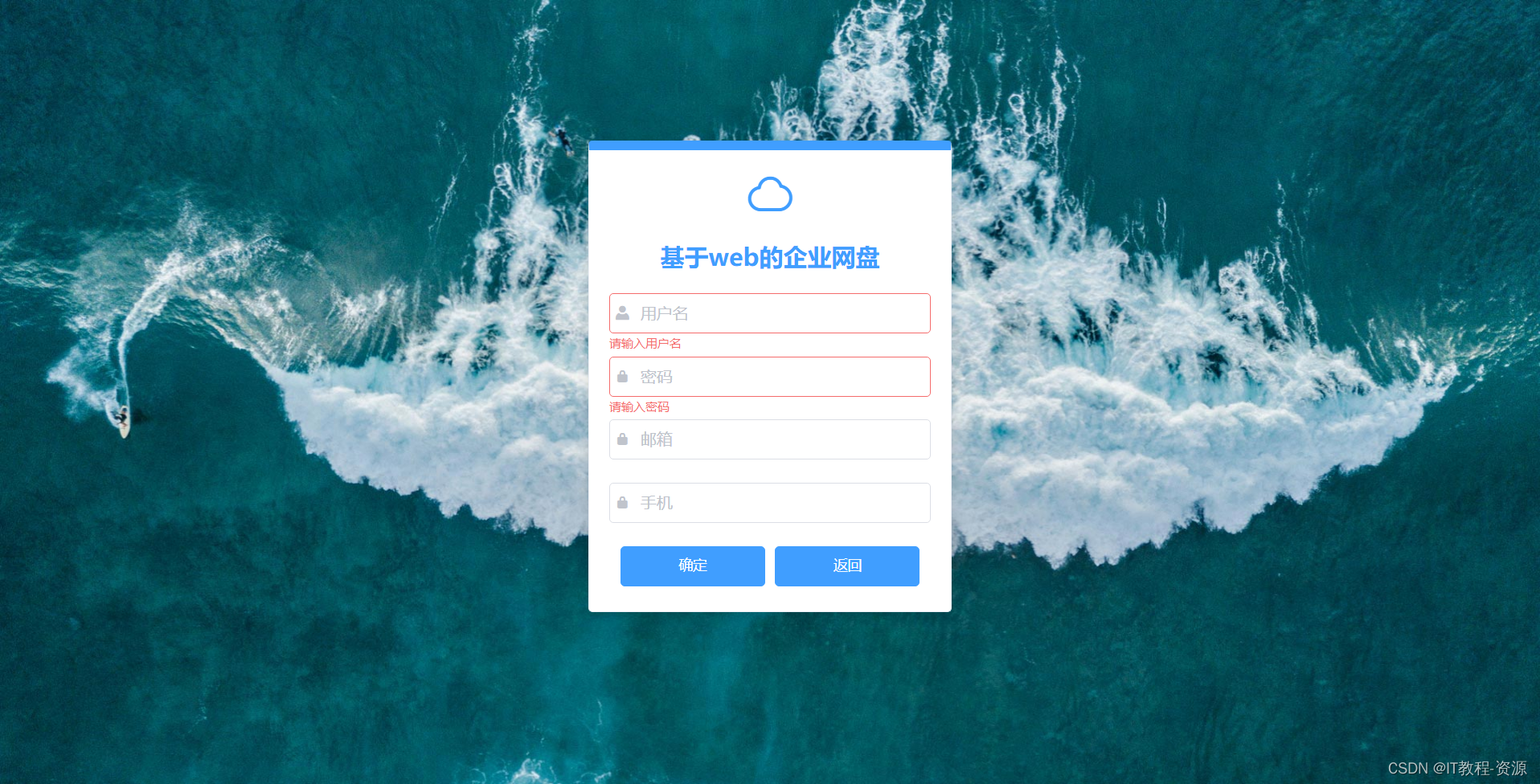 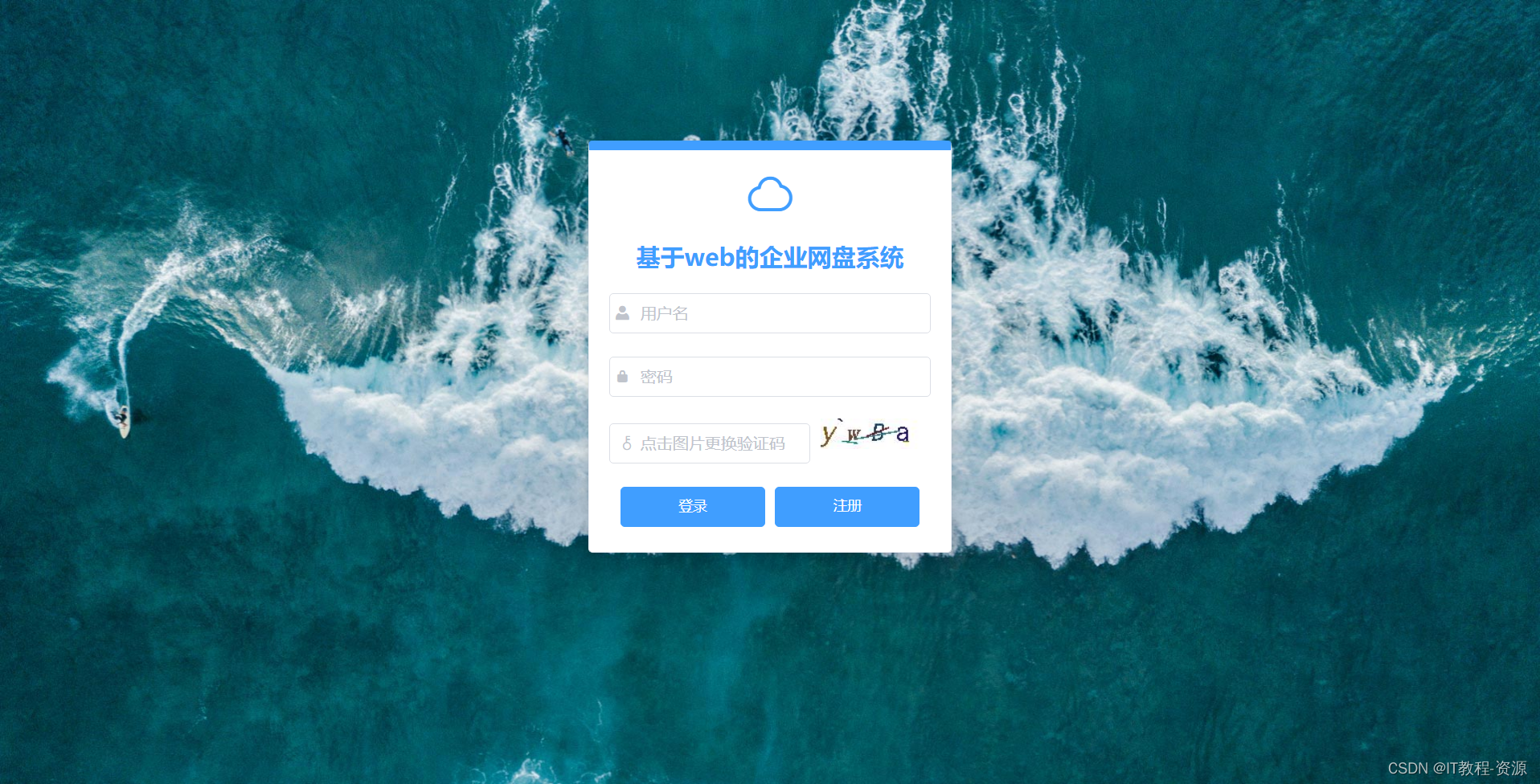 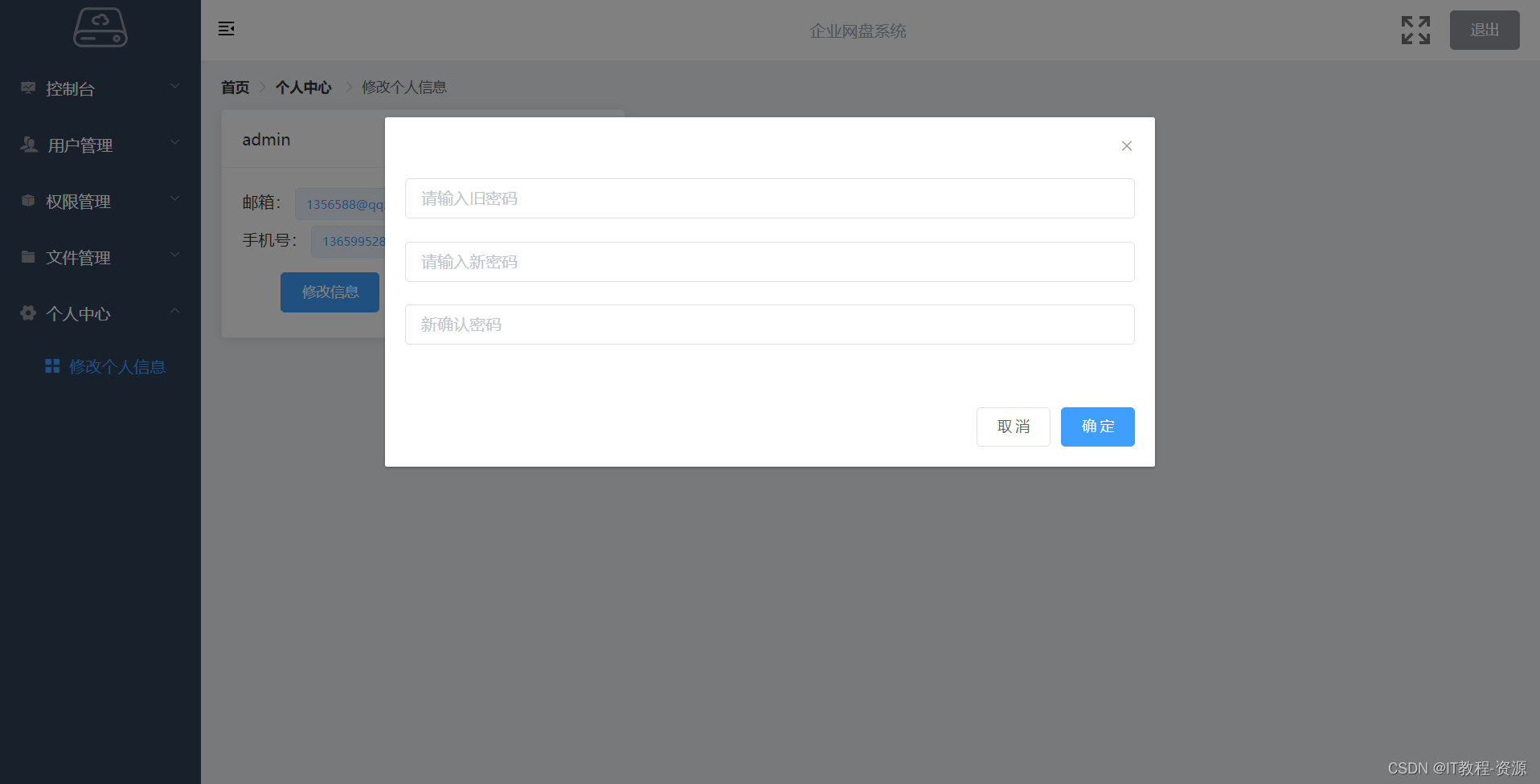 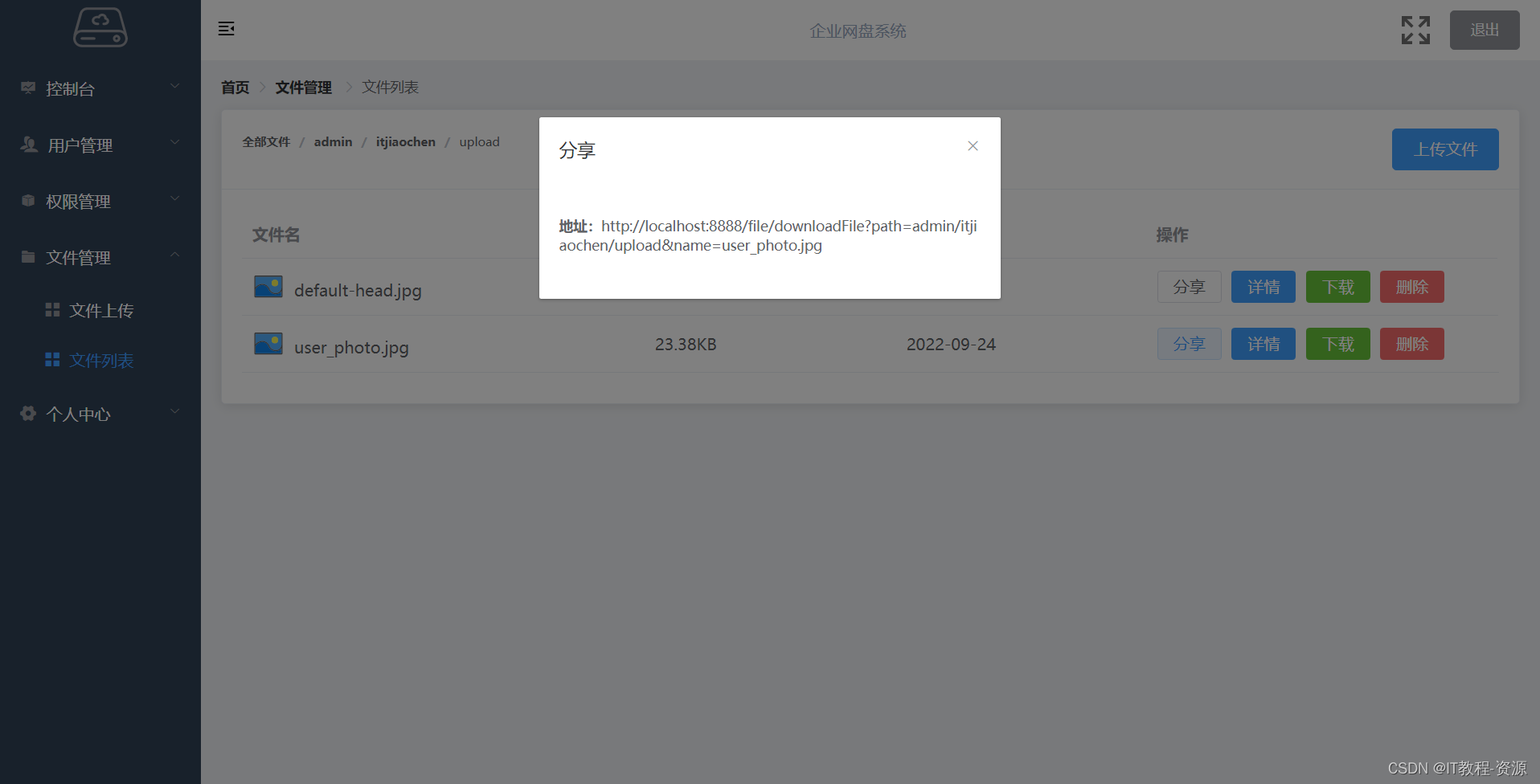 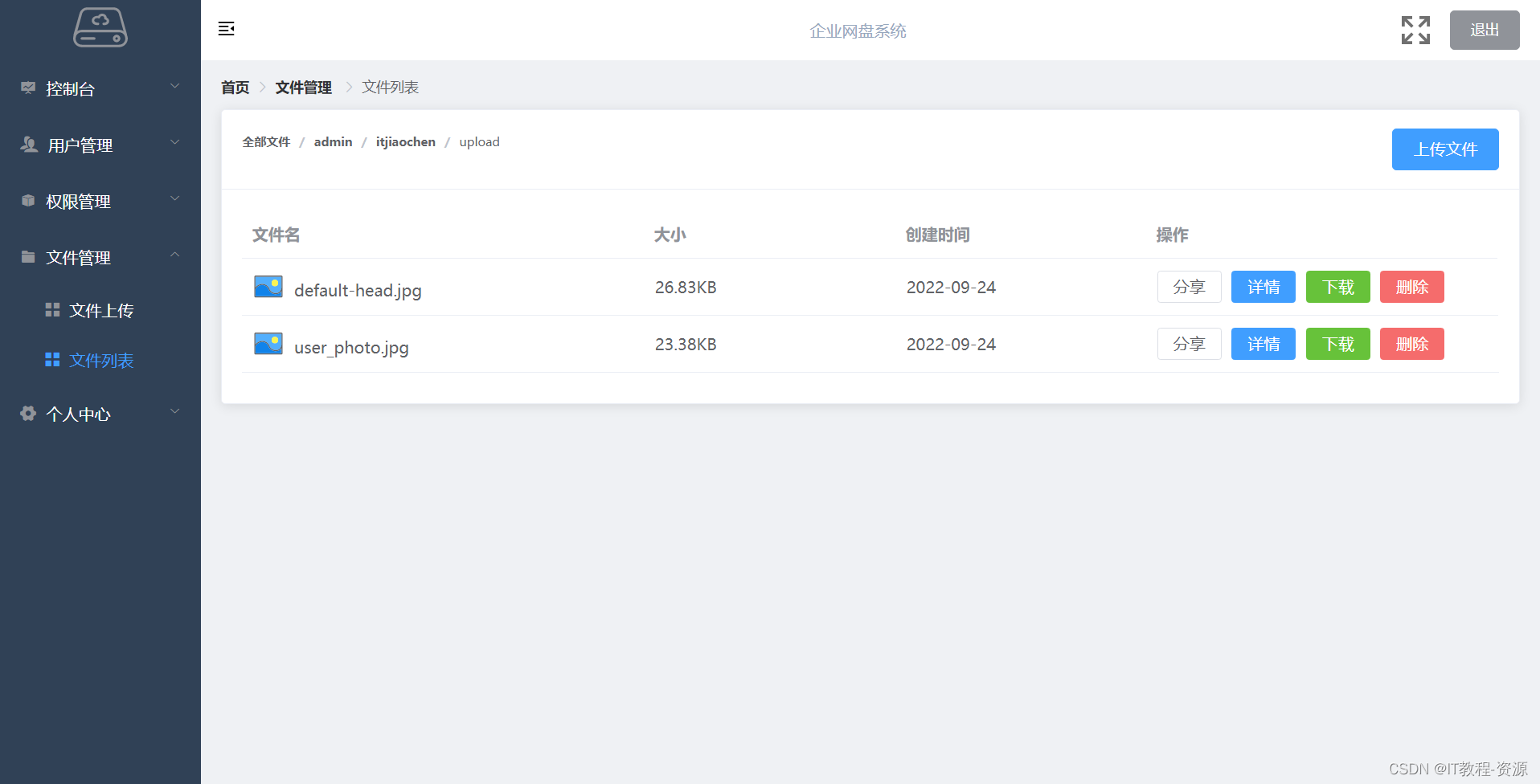 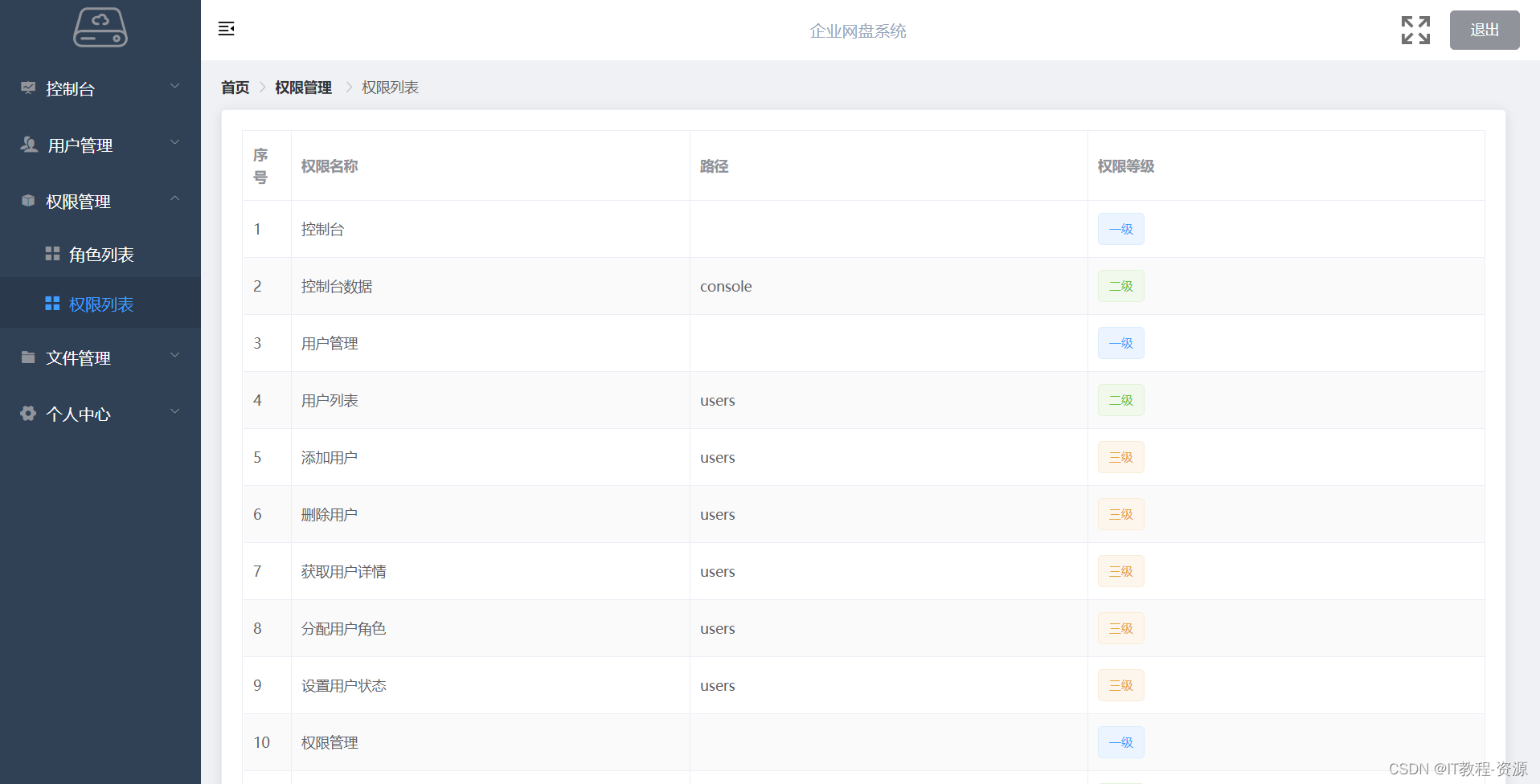 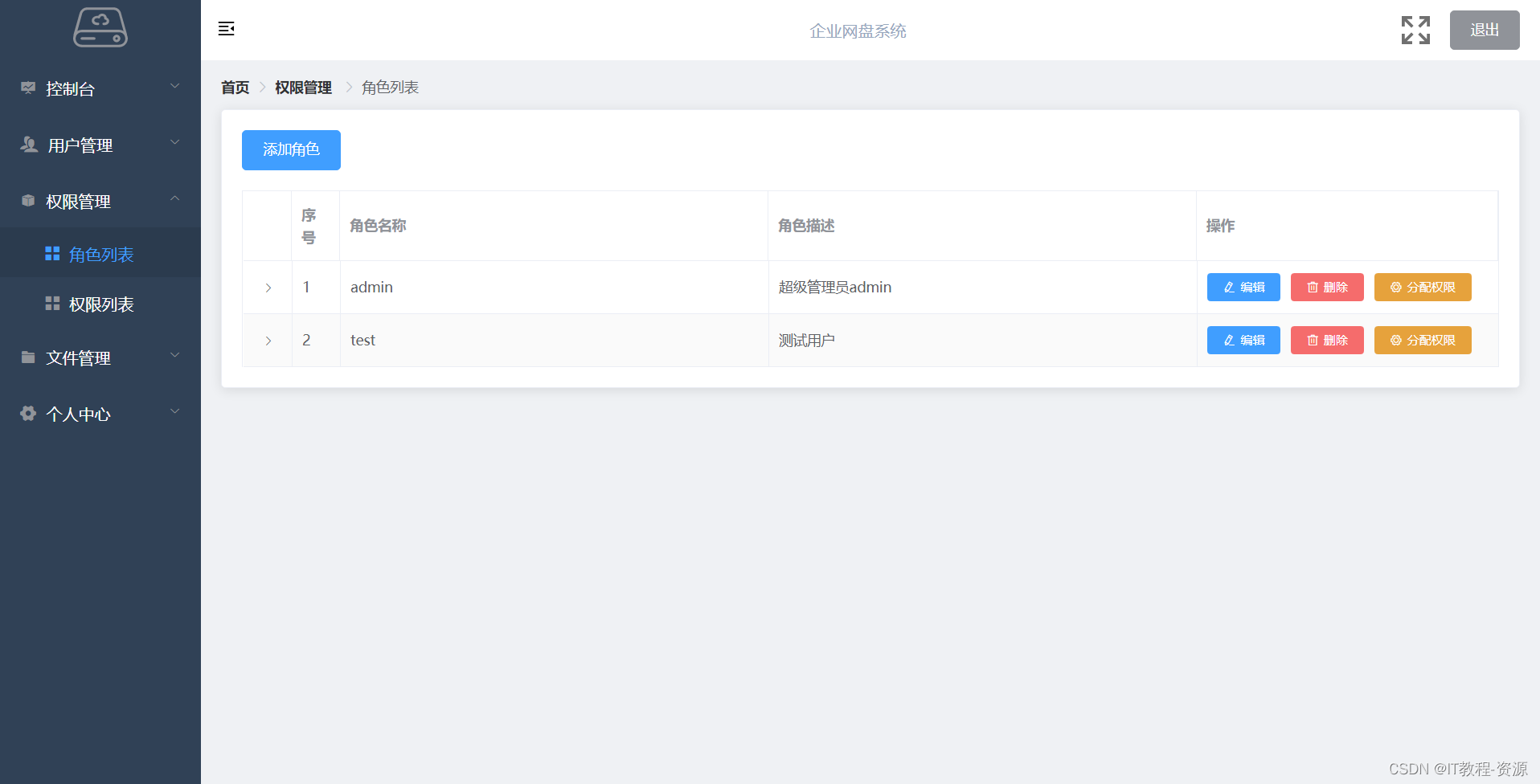 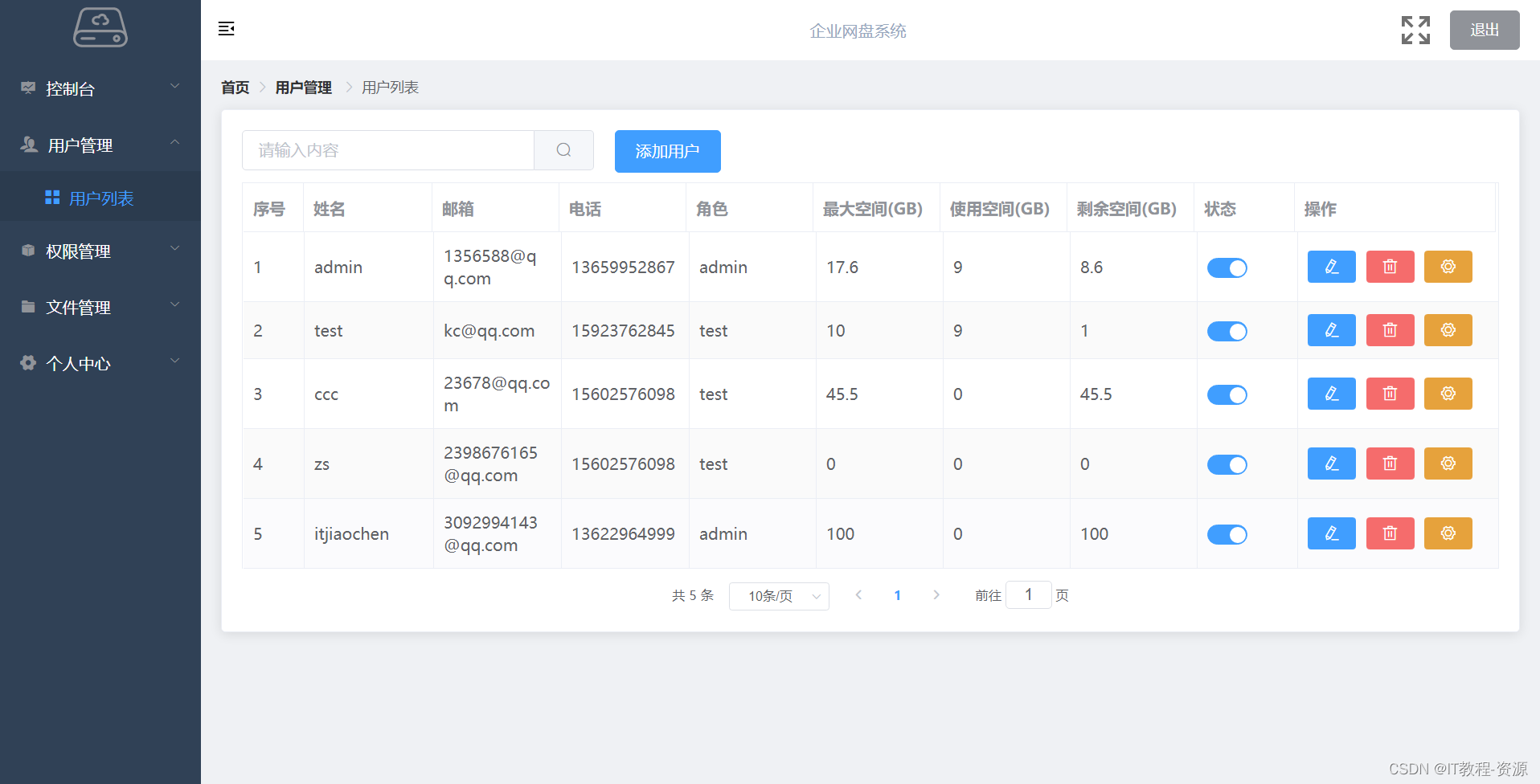 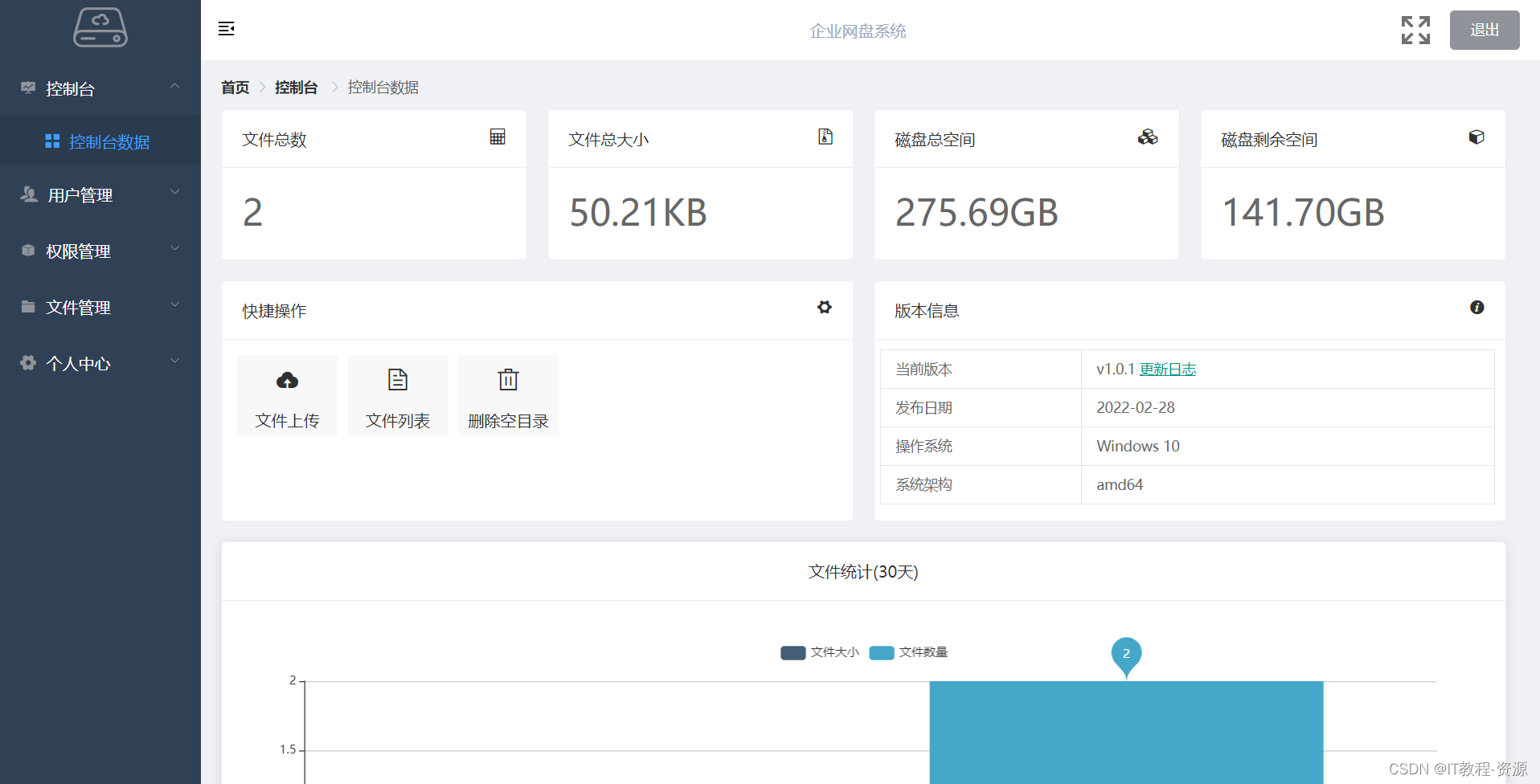 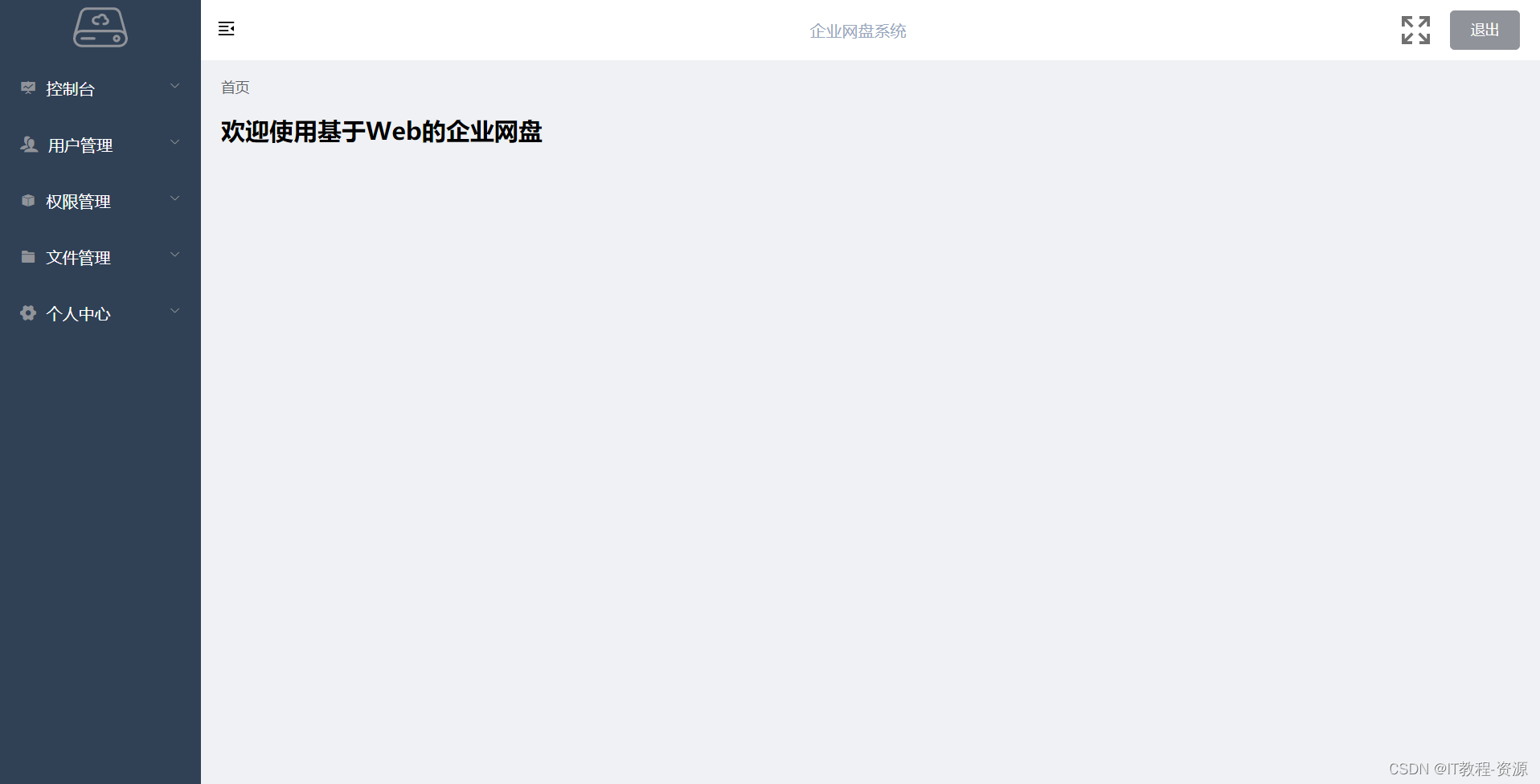
package com.kcqnly.application.controller;
import com.kcqnly.application.common.Result; import com.kcqnly.application.config.VerificationCode; import com.kcqnly.application.entity.Permission; import com.kcqnly.application.model.MenuParam; import com.kcqnly.application.service.PermissionService; import com.kcqnly.application.service.RoleService; import com.kcqnly.application.service.UserService; import com.kcqnly.application.utils.JwtTokenUtil; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.HttpSession; import java.awt.image.BufferedImage; import java.io.IOException; import java.util.ArrayList; import java.util.List;
@RestController public class LoginController {
@Autowired
private JwtTokenUtil jwtTokenUtil;
@Autowired
private UserService userService;
@Autowired
private RoleService roleService;
@Autowired
private PermissionService permissionService;
@GetMapping("/verifyCode")
public void verifyCode(HttpServletRequest request, HttpServletResponse resp) throws IOException {
VerificationCode code = new VerificationCode();
BufferedImage image = code.getImage();
String text = code.getText();
HttpSession session = request.getSession(true);
session.setAttribute("verify_code", text);
VerificationCode.output(image, resp.getOutputStream());
}
@GetMapping("/menus")
public Result getMenuList(HttpServletRequest request) {
String token = request.getHeader("Authorization").replace("Bearer ", "");
String username = jwtTokenUtil.getUsernameFromToken(token);
List<Permission> parent = roleService.getParentPermission(userService.getUserRole(username));
List<MenuParam> menuParamList = new ArrayList<>();
for (Permission permission : parent) {
menuParamList.add(new MenuParam(permission));
}
for (MenuParam menuParam : menuParamList) {
List<MenuParam> childList = new ArrayList<>();
for (Permission permission : permissionService.getTwoLevelChild(menuParam.getId())) {
childList.add(new MenuParam(permission));
}
menuParam.setChildren(childList);
}
return Result.ok("成功",menuParamList);
}
}
package com.kcqnly.application.controller;
import cn.hutool.json.JSONObject;
import cn.hutool.json.JSONUtil;
import com.kcqnly.application.common.Result;
import com.kcqnly.application.service.FileService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.net.URL;
import java.net.URLEncoder;
@RestController
public class FileController {
@Autowired
private FileService fileService;
@Value("${fileServer.url}")
private String url;
@Value("${fileServer.showUrl}")
private String showUrl;
@Value("${fileServer.groupName}")
private String groupName;
@GetMapping("/file/getParentFile")
@PreAuthorize("hasAuthority('文件列表')")
public Result getParentFile() {
return Result.ok(fileService.getParentFile(groupName, url));
}
@GetMapping("/file/getDirFile")
@PreAuthorize("hasAuthority('文件列表')")
public Result getDirFile(@RequestParam("dir") String dir) {
return Result.ok( fileService.getDirFile(showUrl, url, dir));
}
@DeleteMapping("/file/delete/{md5}")
@PreAuthorize("hasAuthority('删除文件')")
public Result deleteFile(@PathVariable String md5) {
if (fileService.deleteFile(url, md5)) {
return new Result(Result.AJAX_SUCCESS,"删除成功");
}
return new Result(Result.AJAX_ERROR, "删除失败");
}
@PostMapping("/file/details")
@PreAuthorize("hasAuthority('文件列表')")
public Result details( String md5) {
return fileService.details(url, md5);
}
@GetMapping("/file/downloadFile")
public void downloadFile(String path,String name, HttpServletResponse response) {
response.setHeader("Content-Disposition", "attachment;filename=" + name);
response.setContentType("application/octet-stream");
BufferedInputStream in = null;
try {
URL url1 = new URL(url + "/" + path + "/" + name.replaceAll(" ","%20"));
in = new BufferedInputStream(url1.openStream());
response.reset();
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode(name, "UTF-8"));
int i;
while ((i = in.read()) != -1) {
response.getOutputStream().write(i);
}
response.getOutputStream().close();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (in != null) {
in.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
|