创建两个SpringBoot项目,A项目端口号8080;B项目端口号8081。
一、创建配置文件
在A项目当中创建配置文件
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.client.RestTemplate;
@Configuration
public class ApplicationContextConfig {
@Bean
public RestTemplate getRestTemplate() {
return new RestTemplate();
}
}
二、简单GET请求
1、在B项目当中编写一个contoller
@GetMapping("/testList.do")
public List<String> testList()
{
List<String> list = new ArrayList<>();
for (int i = 0; i < 5; i++) {
list.add("测试" + i);
}
return list;
}
2、在A项目当中编写一个controller
@Resource
private RestTemplate restTemplate;
@GetMapping("/test")
public void test()
{
Object forObject = restTemplate.getForObject("http://localhost:8081/testList.do", Object.class);
System.out.println(forObject);
}
3、调用A项目的接口
http://localhost:8080/test
4、输出结果
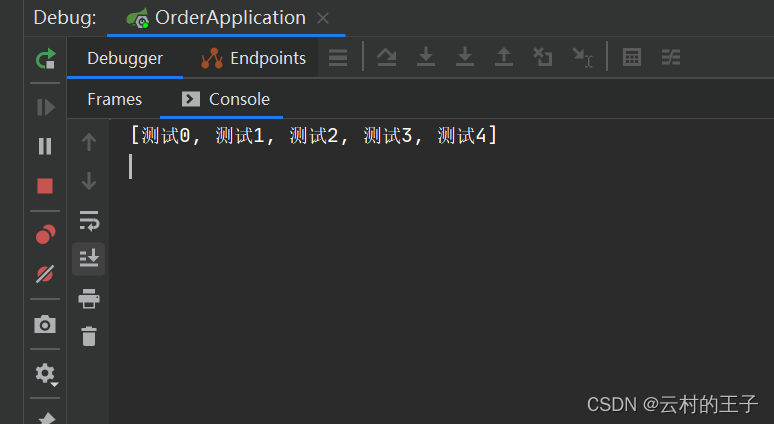
三、带参数的GET请求
操作跟上面的一样,适当修改一样内容。
1、在B项目当中编写一个contoller
@GetMapping("/testList.do")
public List<String> testList(@RequestParam Integer page, @RequestParam Integer pageCount)
{
List<String> list = new ArrayList<>();
String str = "A工程传过来的page参数值为:"+ page +", pageCount参数值为:" + pageCount;
list.add(str);
return list;
}
2、在A项目当中编写一个controller
@Resource
private RestTemplate restTemplate;
@GetMapping("/test")
public void test()
{
Object forObject = restTemplate.getForObject("http://localhost:8081/testList.do?page=1&pageCount=2", Object.class);
System.out.println(forObject.toString());
}
3、还是调用A项目的接口
http://localhost:8080/test
4、输出结果
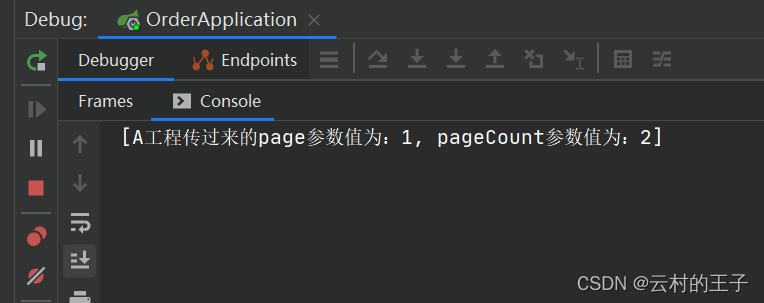
四、POST请求
1、在B项目当中编写一个contoller
@PostMapping("/testAdd.do")
public Employee testAdd(@RequestBody Employee employee)
{
employee.setEmployeeNo("001");
return employee;
}
2、在A项目当中编写一个controller
@Resource
private RestTemplate restTemplate;
@GetMapping("/test")
public void test()
{
Employee employee = new Employee();
employee.setEmployeeName("张三");
Object forObject = restTemplate.postForObject("http://localhost:8081/testAdd.do", employee, Object.class);
System.out.println(forObject);
}
3、还是调用A项目的接口
http://localhost:8080/test
4、输出结果
可以看见在B项目当中set的employeeNo已经返回了。 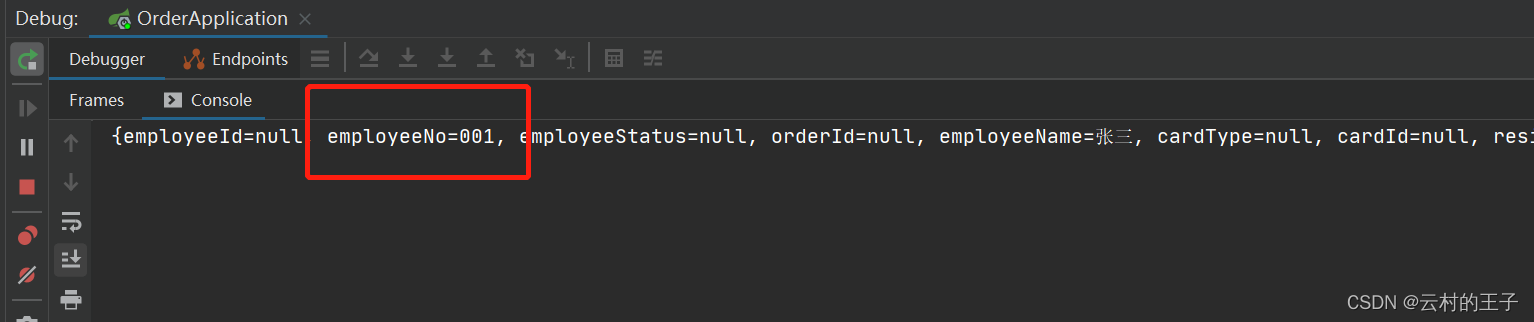
|