?? ??? ?创建容器: ?? ??? ??? ?方式一:类路径加载配置文件 ?? ??? ??? ?
ApplicationContext applicationContext = new
ClassPathXmlApplicationContext("applicationContext.xml");
?? ??? ??? ?方式二:文件路径加载配置文件 ?? ??? ???
?ApplicationContext applicationContext = new FileSystemXmlApplicationContext("D:\\applicationContext.xml");
?? ??? ??? ?方式三:加载多个配置文件 ?? ??? ??? ?
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("bean1.xml","bean2.xml");
?? ??? ?获取bean: ?? ??? ??? ?方式一:使用bean名称获取 ?? ??? ??? ?
BookDao bookDao = (BookDao)applicationContext.getBean("bookDao");
?? ??? ??? ?方式一:使用bean名称获取并指定类型 ?? ??? ??? ?
BookDao bookDao = applicationContext.getBean("bookDao",BookDao.class);
?? ??? ??? ?方式一:使用bean类型获取 ?? ??? ??? ?
BookDao bookDao = applicationContext.getBean(BookDao.class);
?? ??? ?注解开发定义bean ?? ??? ??? ?使用@Component定义bean ?? ??? ???
?@Component("userDao")
?? ??? ??? ?public class BookDaoImpl implements BookDao {
?? ??? ??? ??? ?@Override
?? ??? ??? ??? ?public void save(){
?? ??? ??? ??? ??? ?System.out.println("book dao save ...");
?? ??? ??? ??? ?}
?? ??? ??? ?}
?? ??? ??? ?核心配置文件中通过组件扫描加载bean ?? ??? ? ?
<context:component-scan base-package="com.sx"/>
?? ??? ? ? Spring提供@Component注解的三个衍生注解 ?? ??? ? ? @Controller:用于定义表现层的bean ?? ??? ? ? @service:用于业务层bean定义 ?? ??? ? ? @Repository:用于数据层bean定义 ?? ?Spring3.0升级了纯注解开发模式,使用Java类替代配置文件 ?? ??? ?Java类代替spring核心注解 ?? ??? ???
?//定义当前类为配置类 ?@Configuration d代表xml的一个模板
?? ??? ??? ?@Configuration
?? ??? ??? ?//开启扫描
?? ??? ??? ?@ComponentScan("com.sx")
?? ??? ??? ?public class springConfig {
?? ??? ??? ?}
?? ??? ??? ?@ComponentScan注解用于设定扫描路径,此注解只能添加一次,多个数据请求用数组格式
?? ??? ??? ?@ComponentScan({"com.sx","com.sx1"})
?? ??? ? //初始化Spring容器ApplicationContext,加载配置文件
?? ??? ?// ? ? ? ?ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
? ? ? ? //加载配置类的格式初始
? ? ? ? ApplicationContext ctx= new AnnotationConfigApplicationContext(springConfig.class);
? ?? ??? ? ?? ?? ?依赖注入之自动装配 ?? ??? ?使用@Autowired注解开启自动装配模式(按类型) ?? ??? ?
@Service
?? ??? ?public class BookSeriveImpl implements BookSerive {
?? ??? ??? ??? ?//设置当前属性注入(引用)
?? ??? ??? ?@Autowired
?? ??? ??? ?private BookDao bookDao;
?? ??? ??? ?//可以删除set方法
?? ??? ??? ?//public BookSeriveImpl(BookDao bookDao) {
?? ??? ??? ?// ?this.bookDao = bookDao;
?? ??? ??? ?//}
?? ??? ?
?? ??? ??? ?@Override
?? ??? ??? ?public ?void save(){
?? ??? ??? ??? ?System.out.println("book service save...");
?? ??? ??? ??? ?bookDao.save();
?? ??? ??? ?}
?? ??? ?}
?? ??? ? 注意:1.自动装配基于反射设计创建对象并暴力反射对应属性为私有属性初始化数据,由此无需提供setter方法 ?? ??? ? 2.自动装配建议使用无参构造方法创建对象(默认),如果不提供对应的构造方法,请提供唯一的构造方法 ?? ??? ?使用@Qualifier("***")注解开启指定名称装配 ?? ???
?@Service
?? ??? ?public class BookSeriveImpl implements BookSerive {
?? ??? ??? ??? ?//设置当前属性注入(引用)
?? ??? ??? ?@Autowired
?? ??? ??? ?//使用注解设置注入的bean的id
?? ??? ??? ?@Qualifier("dao")
?? ??? ??? ?private BookDao bookDao;
?? ??? ?}
?? ??? ?注意:@Qualifier注解无法单独使用,必须配合@Autowired注解使用 ?? ??? ?使用@Value实现简单类型注入 ?? ??? ?
@Repository("bookDao")
?? ??? ?public class BookDaoImpl BookDao(){
?? ??? ??? ?@Value("100")
?? ??? ??? ?private String num;
?? ??? ?}
?? ??? ? ?? ??? ?使用@PropertySource注解加载properties文件 ?? ???
?//定义当前类为配置类 ?@Configuration d代表xml的一个模板
?? ??? ?@Configuration
?? ??? ?//开启扫描
?? ??? ?@ComponentScan("com.sx")
?? ??? ?@PropertySource("classpath:jdbc.properties")
?? ??? ?public class SpringConfg{
?? ??? ?}
?? ??? ?注意:路径仅支持单一文件配置,多文件请属于数组格式配置,不允许使用通配符*
这是自己一个小Demo 运行结果
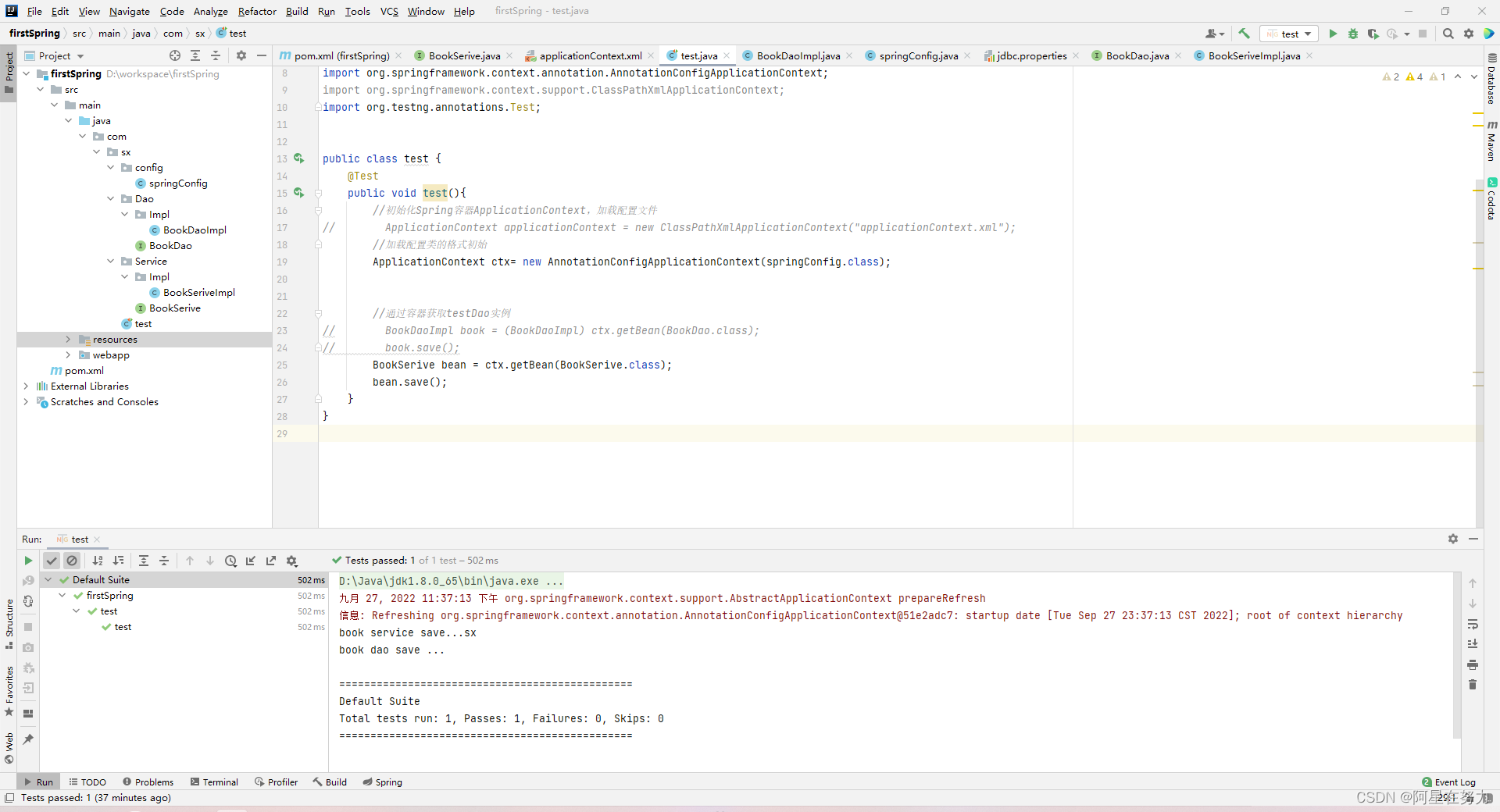
?config包下代码
package com.sx.config;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
//定义当前类为配置类 @Configuration d代表xml的一个模板
@Configuration
//开启扫描
@ComponentScan("com.sx")
//加载外部properties文件
@PropertySource("jdbc.properties")
public class springConfig {
}
BookDaoImpl下代码
package com.sx.Dao.Impl;
import com.sx.Dao.BookDao;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Repository;
//1.将要定义的bean所在的类上添加@Component,相当于是 <bean id="userDao" class="com.sx.Dao.Impl.BookDaoImpl"/>
//@Component("userDao")
@Repository("dao")
public class BookDaoImpl implements BookDao {
@Override
public void save(){
System.out.println("book dao save ...");
}
}
BookDao代码
package com.sx.Dao;
import org.springframework.stereotype.Repository;
public interface BookDao {
public void save();
}
BookServiceImpl下代码
package com.sx.Service.Impl;
import com.sx.Dao.BookDao;
import com.sx.Service.BookSerive;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
@Service
public class BookSeriveImpl implements BookSerive {
//设置当前属性注入(引用)
@Autowired
//使用注解设置注入的bean的id
@Qualifier("dao")
private BookDao bookDao;
@Autowired
@Value("${name}")
private String name;
//可以删除set方法
//public BookSeriveImpl(BookDao bookDao) {
// this.bookDao = bookDao;
//}
@Override
public void save(){
System.out.println("book service save..."+name);
bookDao.save();
}
}
BookService下代码
package com.sx.Service;
import com.sx.Dao.BookDao;
public interface BookSerive {
public void save();
}
test测试类代码
package com.sx;
import com.sx.Dao.BookDao;
import com.sx.Dao.Impl.BookDaoImpl;
import com.sx.Service.BookSerive;
import com.sx.config.springConfig;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import org.testng.annotations.Test;
public class test {
@Test
public void test(){
//初始化Spring容器ApplicationContext,加载配置文件
// ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
//加载配置类的格式初始
ApplicationContext ctx= new AnnotationConfigApplicationContext(springConfig.class);
//通过容器获取testDao实例
// BookDaoImpl book = (BookDaoImpl) ctx.getBean(BookDao.class);
// book.save();
BookSerive bean = ctx.getBean(BookSerive.class);
bean.save();
}
}
jdbc.properties下 就只有一个对name赋值
name=sx
applicationContext.xml文件可要不可要
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation=
"http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<!-- 将对象的创建权交由spring管理-->
<!-- <bean id="userDao" class="com.sx.Dao.Impl.BookDaoImpl"/>-->
<!-- 2.在容器中发现bean,挨个文件遍历 称为扫描-->
<!-- <context:component-scan base-package="com.sx"/>-->
</beans>
|