- 通过idea创建SpringBoot工程(此项目创建前已经配置过了Maven)
配置依赖: 确保pom.xml文件中的<dependencies> </dependencies>如下(版本可以不同): ? <dependencies>
<!-- Spring Boot的基础依赖项 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<!-- Mybatis整合Spring Boot的依赖项 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<!-- MySQL的依赖项 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!-- Lombok的依赖项,主要用于简化POJO类的编写 -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<scope>provided</scope>
</dependency>
<!-- Spring Boot测试的依赖项 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies> 数据库连接配置: # 连接数据库的配置(mall_pms)数据库名 密码和账号请根据自己的数据库
spring.datasource.url=jdbc:mysql://localhost:3306/mall_pms?useUnicode=true&characterEncoding=utf-8&serverTimezone=Asia/Shanghai
spring.datasource.username=root
spring.datasource.password=root ? - 创建实体类
在自己的项目包下 创建 实体类包 比如:entity 这里创建了一个? 处理相册数据的实体类:Album 实体类规范如下: (1)所有属性应该私有化; (2)所有的属性应该有对应的Getter和Setter方法; (3)必须重写equals()和hashCode()方法; (4)必须实现Serializable接口; (5)建议重写toString()方法。 上述规范出了(4)都可以用下述注解实现(添加到实体类上) @Data 在项目中使用Lombok框架,可以实现:添加注解,即可使得Lombok在项目的编译期自动生成一些代码(例如Setter & Getter)
Lombok配置文件见pom.xml,还需要在idea中下载Lombok插件;
Album实体类:
@Data //处理Getter,Setter,toString和equals,hashCode
public class Album implements Serializable {
/**
* 记录id
* */
private Long id;
/**
* 相册名称
* */
private String name;
/**
* 相册简介(描述)
* */
private String description;//描述
/**
* 自定义排序序号
* */
private Integer sort;//分类
/**
* 数据创建时间
* */
private LocalDateTime gmtCreate;//创建时间
/**
* 数据最后的修改时间
* */
private LocalDateTime gmtModified;//修改时间
} - 通过Mybatis实现数据库编程
3.1关于Mybatis框架: (1)Mybatis是目前主流的解决数据库编程相关问题的框架,主要是简化了数据库编程。 ? 体现:只需要定义访问数据的抽象方法,并被配置此方法映射的SQL语句即可。 (2)Mybatis框架的基础依赖项的<artifactId>是:mybatis,但是直接用的话还要其他的大量配置,这里我们可以用mybatis-spring来简化,这样就不需要其他的配置了。
3.2访问数据库的抽象方法 使用Mybatis框架时,访问数据库的抽象方法必须定义在接口中,原因是:Mybatis框架是通过“接口袋里”的设计模式,生成了接口的实现对象! 对于抽象方法所在的接口通常有两种方法处理:(这里我们使用的(2) ) (1)通过在接口上添加 @Mapper注解来实现 生成接口对象; (2)通过配置类添加@MapperScan 注解来指定 数据访问的接口(此方法的优点是相较于? ? ? ? ? ? ?(1)只需要配置一次,再次生成接口时就不需要再添加@Mapper注解,不然没创建一个数数据接口就需要添加一个@Mapper注解) (2.1)配置类的创建: 需要创建在根包下;添加注解@Configuration (称为配置类),再添加@MapperScan指定接口 生成实现对象。如下:
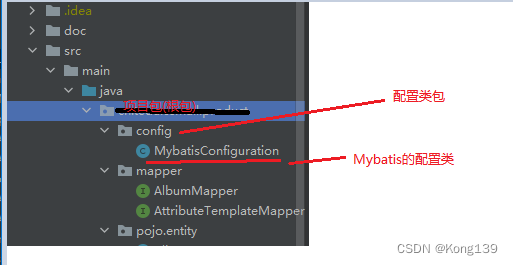 配置类(MybatisConfiguration): @Configuration //使类称为配置类
@MapperScan("XXX(根包全路径).mapper") //此处的mapper是接口包
public class MybatisConfiguration {
}
? ? ? ? ?3.3配置SQL语句 ? ? ? ? (1)通过@Insert等注解添加在 接口的抽象方法上; ?
public interface AlbumMapper {
@Insert("Sql语句")
int insert(Album album);
}
? ? ? ? (2)通过配置对应的XML文件,配置SQL语句(推荐这种方法) ????????
public interface AlbumMapper {
/**
* 插入相册数据
*
* @param album 相册数据
* @return 受影响的行数
*/
int insert(Album album);
}
XML文件中的配置(在resource包下建立mapper(xml包)放XML文件?) ????????
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="XXX(项目包全路径).mapper.AlbumMapper">//mapper(XML文件包)
//AlbumMapper(XML文件名)
<!-- int insert(Album album); -->
<insert id="insert" useGeneratedKeys="true" keyProperty="id">
INSERT INTO pms_album (
name, description, sort
) VALUES (
#{name}, #{description}, #{sort}
)
</insert>
</mapper>
?注意: a.?其他SQL与其类似,其中<select> 需要在标签中必须配置`resultType`或`resultMap`这2个属性中的其中1个,其中resultType=“” 值为抽象方法的返回值; b. 其中 标签中id的值为 抽象方法的名称; c. 根标签必须是<mapper>,根标签必须配置namespace属性,此属性值:接口的**全限定名(包名与类名)**
d. <mapper> 标签内部使用<insert> / <delete> /<update> /<select>来配置增删改查
还要在application.properties配置XML文件位置: ?
# 配置Mybatis的XML文件的位置
mybatis.mapper-locations=classpath:mapper/*.xml
结束!
可以再测试类中进行测试:
@SpringBootTest
public class AlbumMapperTests {
@Autowired
AlbumMapper mapper;
@Test
void testInsert() {
Album album = new Album();
album.setName("测试相册006");
album.setDescription("测试简介006");
album.setSort(99); // 注意:我的表 的取值必须是 [0, 255]
System.out.println("插入数据之前,参数=" + album);
int rows = mapper.insert(album);
System.out.println("插入数据完成,受影响的行数=" + rows);
System.out.println("插入数据之后,参数=" + album);
}
}
|