1、starter与autoconfigure
一个完整的Spring Boot Starter可能包含以下组件:
- autoconfigure模块:包含自动配置的代码
- starter模块:提供对autoconfigure模块的依赖,以及一些其它的依赖
(PS:如果你不需要区分这两个概念的话,也可以将自动配置代码模块与依赖管理模块合并成一个模块) 简而言之,starter应该提供使用该库所需的一切
1.1、命名
- 模块名称不能以spring-boot开头
- 如果你的starter提供了配置keys,那么请确保它们有唯一的命名空间。而且,不要用Spring Boot用到的命名空间(比如:server, management, spring 等等)
举个例子,假设你为“acme”创建了一个starter,那么你的auto-configure模块可以命名为acme-spring-boot-autoconfigure,starter模块可以命名为acme-spring-boot-starter。如果你只有一个模块包含这两部分,那么你可以命名为acme-spring-boot-starter。
1.2、autoconfigure模块
建议在autoconfigure模块中包含下列依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-autoconfigure-processor</artifactId>
<optional>true</optional>
</dependency>
2、示例

2.1、autoconfigure模块
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-autoconfigure</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
</dependencies>
public class HelloService {
private String name;
private Integer age;
private String hometown;
public HelloService(String name, Integer age, String hometown) {
this.name = name;
this.age = age;
this.hometown = hometown;
}
public String sayHello(String name) {
return "Hello, " + name;
}
public String helloWorld() {
return String.format("[name=%s, age=%d, hometown=%s]", this.name, this.age, this.hometown);
}
}
@ConfigurationProperties("my.hello")
@Data
public class HelloProperties {
private String name;
private Integer age;
private String hometown;
}
自动配置类,将HelloService.class Bean注册到Spring容器中
@Configuration
@EnableConfigurationProperties(HelloProperties.class)
public class HelloServiceAutoConfiguration {
private final HelloProperties helloProperties;
public HelloServiceAutoConfiguration(HelloProperties helloProperties) {
this.helloProperties = helloProperties;
}
@Bean
@ConditionalOnMissingBean
public HelloService helloService() {
return new HelloService(this.helloProperties.getName(),
this.helloProperties.getAge(),
this.helloProperties.getHometown());
}
}
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
com.mecuros.springboot.custom.starter.HelloServiceAutoConfiguration
2.2、starter模块
只引入autoconfigure模块,其他不做任何操作(PS:可以合并到一个模块)
2.3、web模块
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.mecuros</groupId>
<artifactId>hello-spring-boot-starter</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
my:
hello: yujianbo
age: 25
hometown: chongqing
@RestController("/demo")
public class HelloController {
@Resource
private HelloService helloService;
@GetMapping("/sayHello/{name}")
public String sayHello(@PathVariable String name){
return helloService.sayHello(name);
}
}
3、验证
3.1、猜想
首先,我们的目的是写一个入门级HelloWorldStarter,最关键的是web模块是否能不能引用到自定义starter,并成功使用。
3.2、结果
启动web模块,然后访问接口: 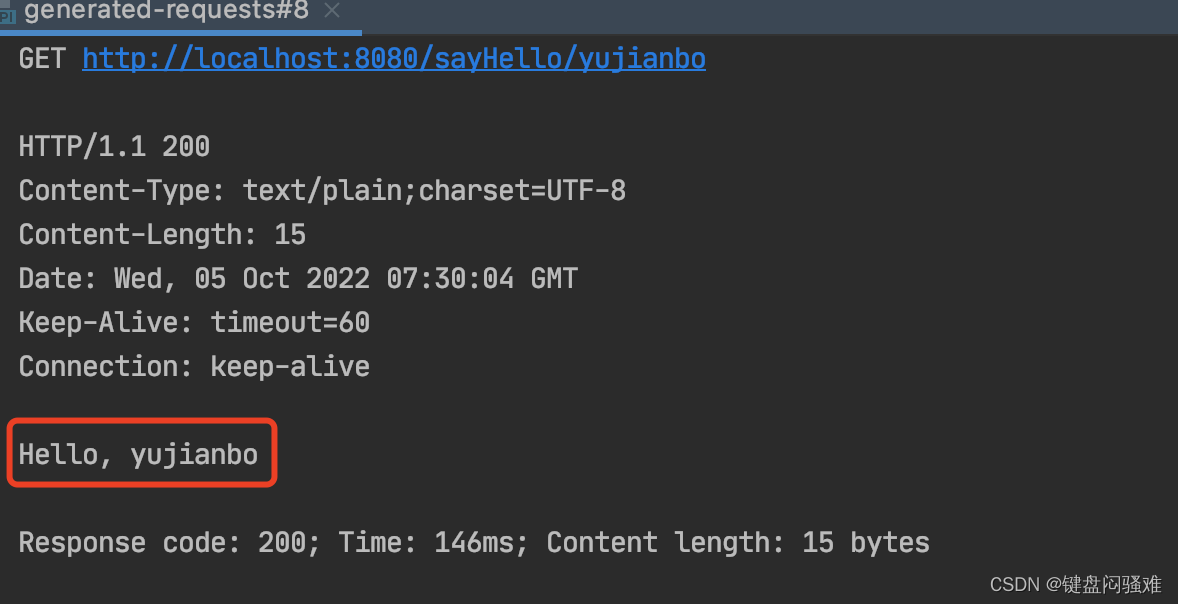
从结果看:自定义starter是学会了
|