目录
一.前言
二.基本整合方式
思路梳理
Spring和MyBatis的整合步骤
三.Spring整合MyBatis
1.目录轮廓
2.使用Navicat在数据库中创建
3.添加项目所需的maven依赖
编写
pom.xml
实体类Student
StudentDao接口
StudentDao.Mapper映射文件
MyBatis.xml核心配置文件
service接口
service实现类
jdbc.properties外部文件
Spring核心配置文件
四.测试方法
1.1测试
1.2测试
1.3测试
五.总结
一.前言
将 MyBatis 与 Spring 进行整合,主要解决的问题就是将 SqlSessionFactory 对象交由 Spring 来管理。所以,该整合,只需要将 SqlSessionFactory 的对象生成器 SqlSessionFactoryBean 注册在 Spring 容器中,再将其注入给 Dao 的实现类即可完成整合。实现 Spring 与 MyBatis 的整合常用的方式:扫描的 Mapper 动态代理 Spring 像插线板一样,mybatis 框架是插头,可以容易的组合到一起。插线板 spring 插上 mybatis,两个框架就是一个整体。?
使用mybatis,需要创建mybatis框架中的某些对象,使用这些对象,就可以使用mybatis提供的功能了。
对于mybatis执行sql语句,需要用到的对象有:
- SqlSessionFactory对象,只有创建了SqlSessionFactory对象,才能调用openSession()方法得到SqlSession对象。
- dao接口的代理对象,例如StudentDao接口,需要的代理对象为:SqlSeesion.getMapper(StudentDao.class)。
- 数据源DataSource对象,使用一个更强大、功能更多的连接池对象代替mybatis自己的PooledDataSource。
二.基本整合方式
思路梳理
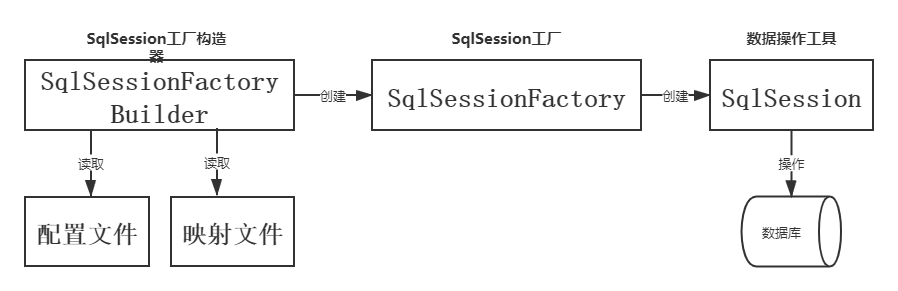
?以上流程可以全部移交给Spring来处理 读取配置文件、组件的创建、组件之间的依赖关系以及整个框架的生命周期都由Spring容器统一管理
Spring和MyBatis的整合步骤
- 整合所需的依赖及配置
- 通过Spring配置文件配置数据源
- 通过Spring配置文件创建SqlSessionFactory
- 通过SqlSessionTemplate操作数据库
提示
使用SqlSessionDaoSupport简化编码
使用Spring配置数据源时,首先选择一种具体的数据源实现技术
三.Spring整合MyBatis
1.目录轮廓
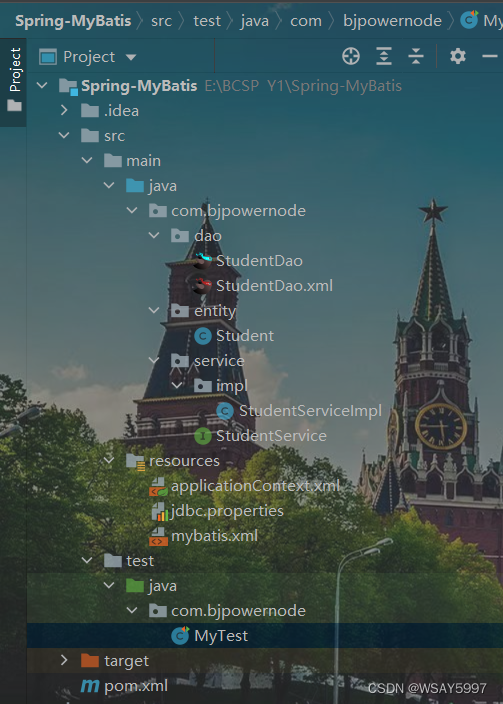
数据库:ssm
数据表:student2?
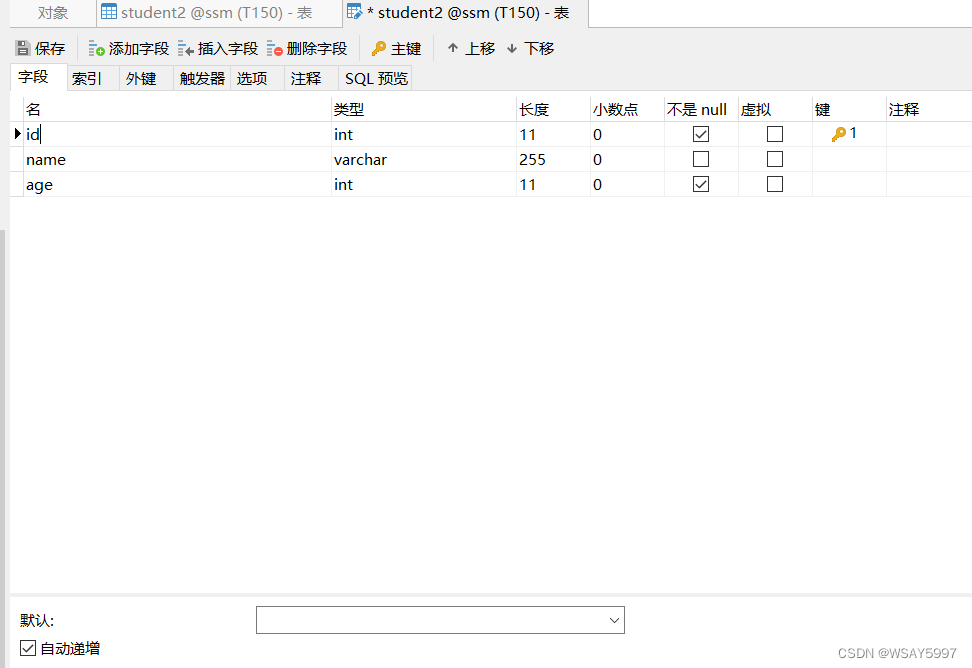
?ID设置了自动递增,并且主键
3.添加项目所需的maven依赖
编写
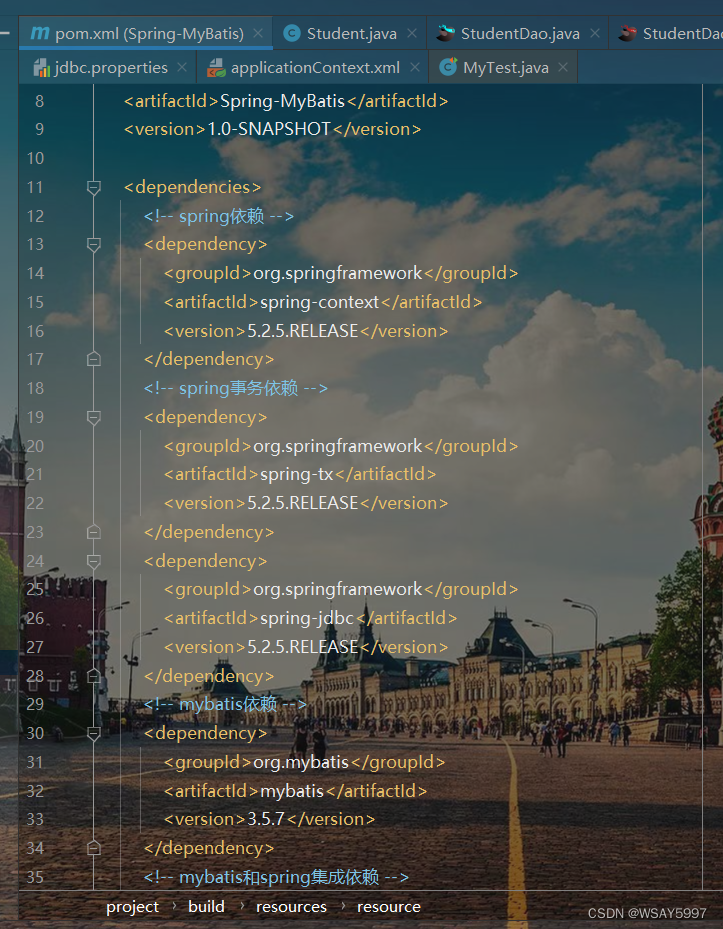
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>Spring-MyBatis</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<!-- spring依赖 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<!-- spring事务依赖 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<!-- mybatis依赖 -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.7</version>
</dependency>
<!-- mybatis和spring集成依赖 -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.3.1</version>
</dependency>
<!-- mysql驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.13</version>
</dependency>
<!-- 阿里的连接池 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.6</version>
</dependency>
<!-- 单元测试 -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<resources>
<resource>
<directory>src/main/java</directory> <!--所在的目录-->
<includes> <!--包括目录下的.properties,.xml 文件都会扫描到-->
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
</build>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
</project>
实体类Student
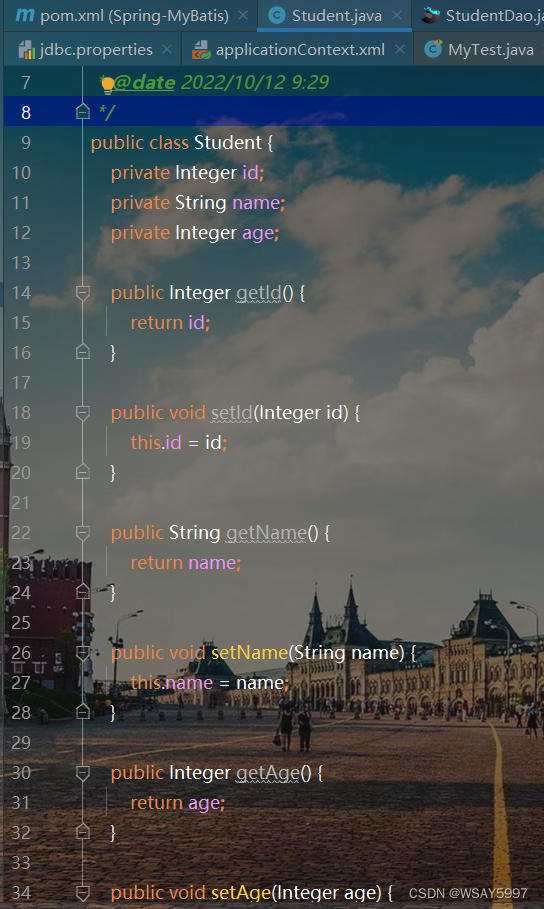
代码?
public class Student {
private Integer id;
private String name;
private Integer age;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", age=" + age +
'}';
}
}
StudentDao接口
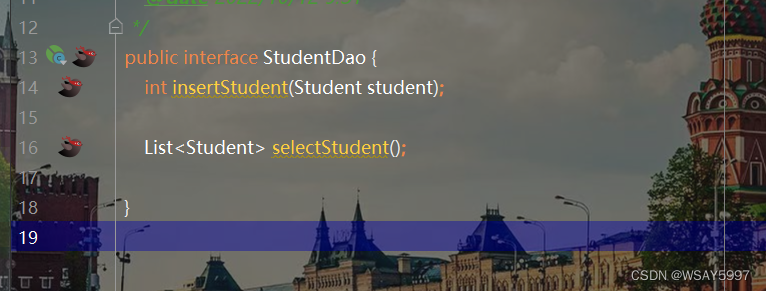
?代码
public interface StudentDao {
int insertStudent(Student student);
List<Student> selectStudent();
}
StudentDao.Mapper映射文件
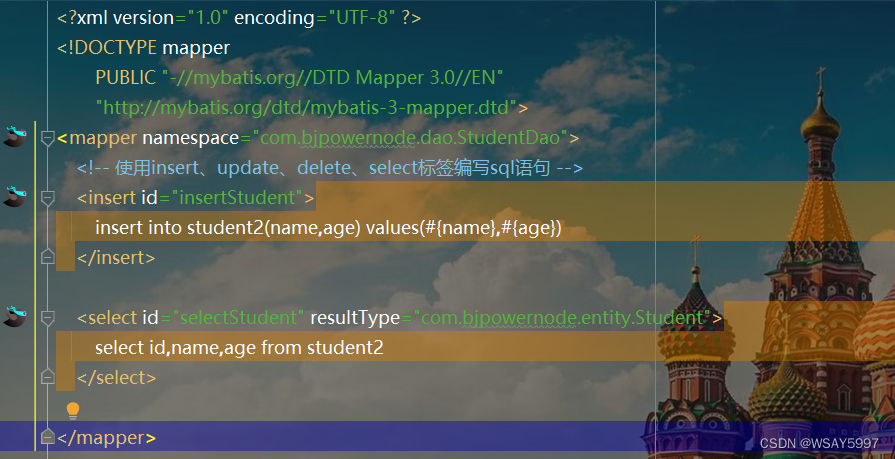
代码?
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.bjpowernode.dao.StudentDao">
<!-- 使用insert、update、delete、select标签编写sql语句 -->
<insert id="insertStudent">
insert into student2(name,age) values(#{name},#{age})
</insert>
<select id="selectStudent" resultType="com.bjpowernode.entity.Student">
select id,name,age from student2
</select>
</mapper>
MyBatis.xml核心配置文件
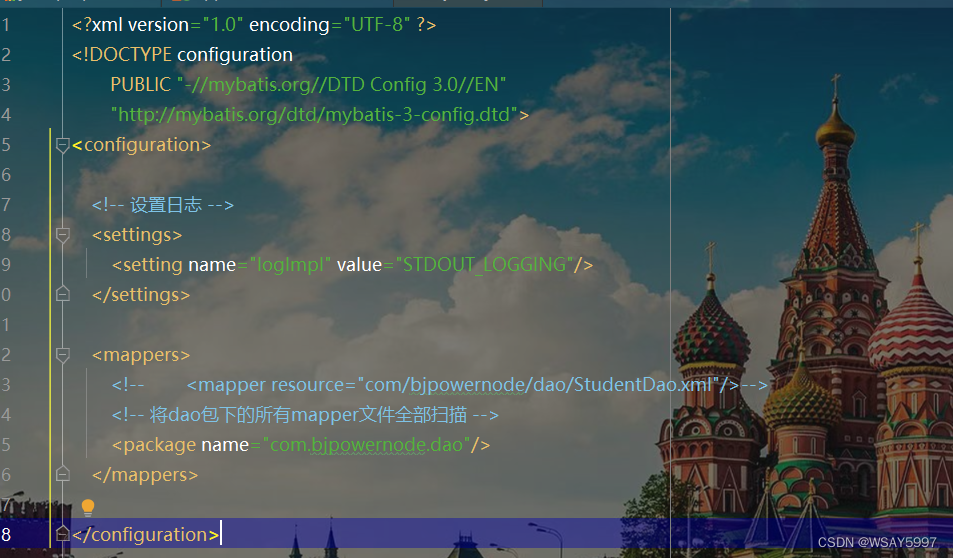
mybatis.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!-- 设置日志 -->
<settings>
<setting name="logImpl" value="STDOUT_LOGGING"/>
</settings>
<mappers>
<!-- <mapper resource="com/bjpowernode/dao/StudentDao.xml"/>-->
<!-- 将dao包下的所有mapper文件全部扫描 -->
<package name="com.bjpowernode.dao"/>
</mappers>
</configuration>
service接口
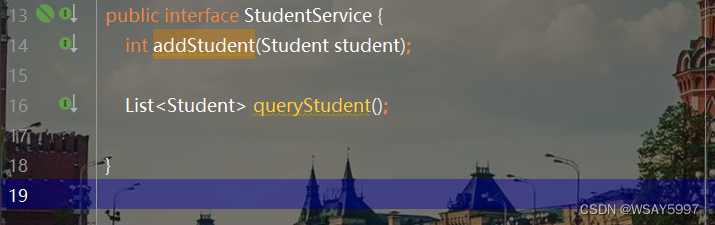
?代码
public interface StudentService {
int addStudent(Student student);
List<Student> queryStudent();
}
service实现类
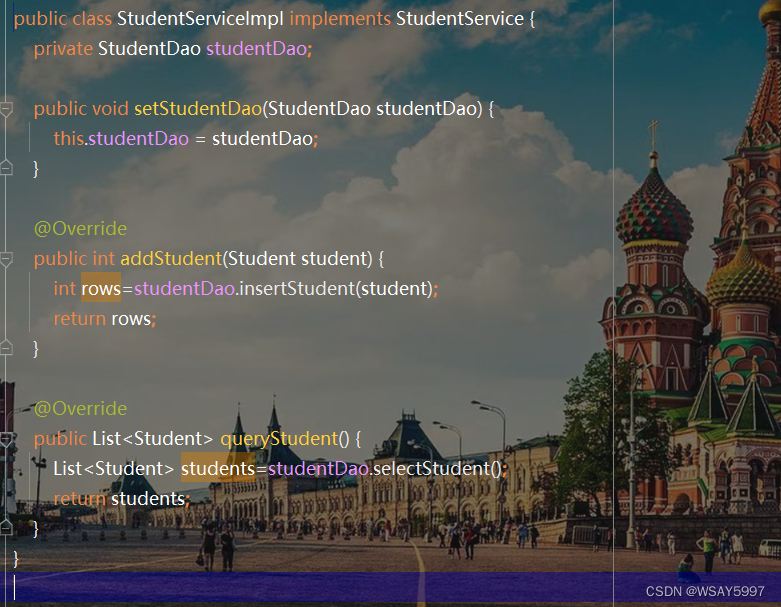
代码?
public class StudentServiceImpl implements StudentService {
private StudentDao studentDao;
public void setStudentDao(StudentDao studentDao) {
this.studentDao = studentDao;
}
@Override
public int addStudent(Student student) {
int rows=studentDao.insertStudent(student);
return rows;
}
@Override
public List<Student> queryStudent() {
List<Student> students=studentDao.selectStudent();
return students;
}
}
jdbc.properties外部文件
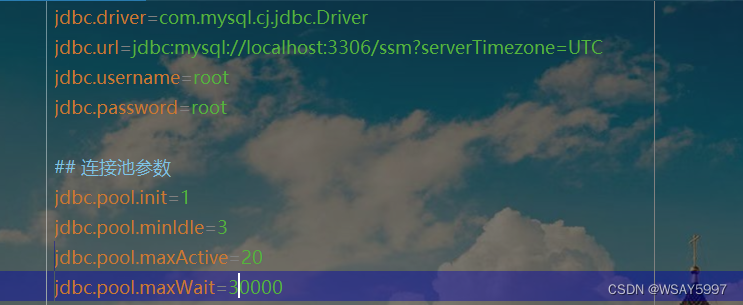
代码?
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/ssm?serverTimezone=UTC
jdbc.username=root
jdbc.password=root
## 连接池参数
jdbc.pool.init=1
jdbc.pool.minIdle=3
jdbc.pool.maxActive=20
jdbc.pool.maxWait=30000
Spring核心配置文件
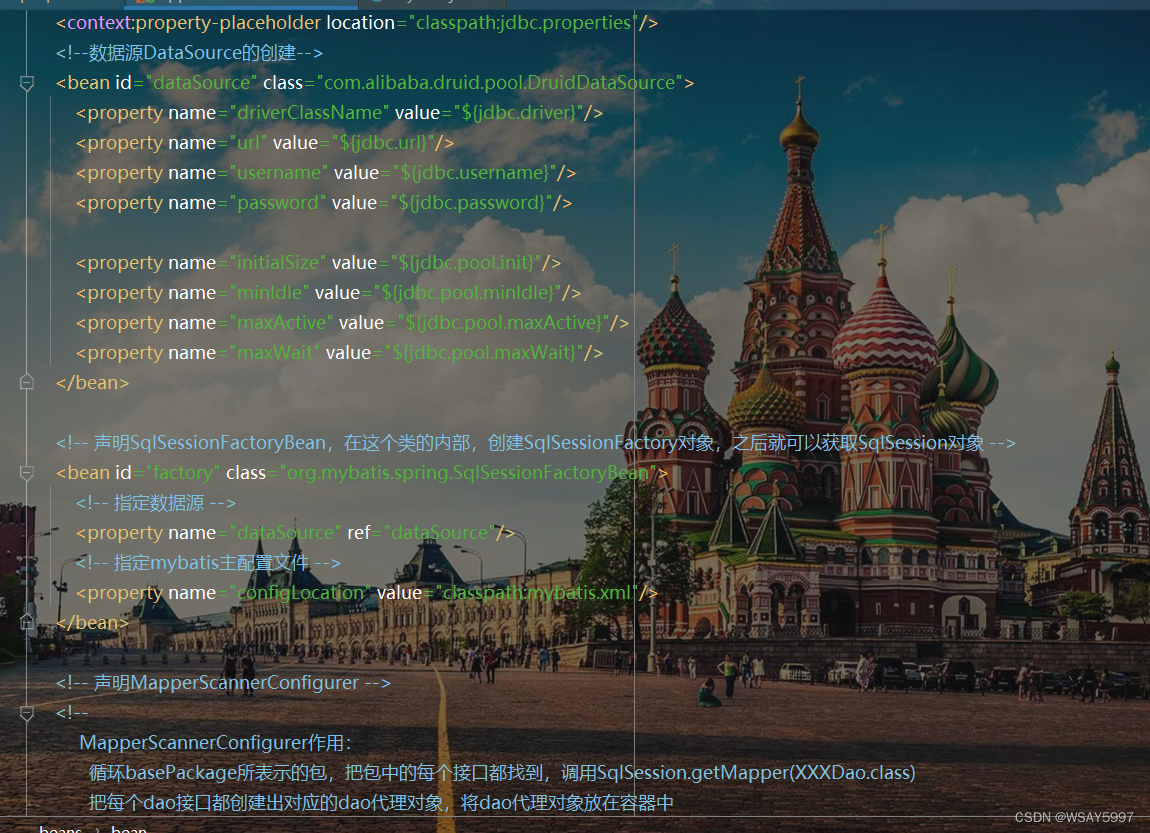
applicationContext.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd">
<!--加载jdbc.properties文件-->
<context:property-placeholder location="classpath:jdbc.properties"/>
<!--数据源DataSource的创建-->
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="${jdbc.driver}"/>
<property name="url" value="${jdbc.url}"/>
<property name="username" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
<property name="initialSize" value="${jdbc.pool.init}"/>
<property name="minIdle" value="${jdbc.pool.minIdle}"/>
<property name="maxActive" value="${jdbc.pool.maxActive}"/>
<property name="maxWait" value="${jdbc.pool.maxWait}"/>
</bean>
<!-- 声明SqlSessionFactoryBean,在这个类的内部,创建SqlSessionFactory对象,之后就可以获取SqlSession对象 -->
<bean id="factory" class="org.mybatis.spring.SqlSessionFactoryBean">
<!-- 指定数据源 -->
<property name="dataSource" ref="dataSource"/>
<!-- 指定mybatis主配置文件 -->
<property name="configLocation" value="classpath:mybatis.xml"/>
</bean>
<!-- 声明MapperScannerConfigurer -->
<!--
MapperScannerConfigurer作用:
循环basePackage所表示的包,把包中的每个接口都找到,调用SqlSession.getMapper(XXXDao.class)
把每个dao接口都创建出对应的dao代理对象,将dao代理对象放在容器中
对于StudentDao接口,其代理对象为 studentDao
-->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<!-- 指定SqlSessionFactory对象的名称 -->
<property name="sqlSessionFactoryBeanName" value="factory"/>
<!-- 指定基本包,dao接口所在的包名 -->
<property name="basePackage" value="com.bjpowernode.dao"/>
</bean>
<!-- 声明service -->
<bean id="studentService" class="com.bjpowernode.service.impl.StudentServiceImpl">
<property name="studentDao" ref="studentDao"/>
</bean>
</beans>
四.测试方法
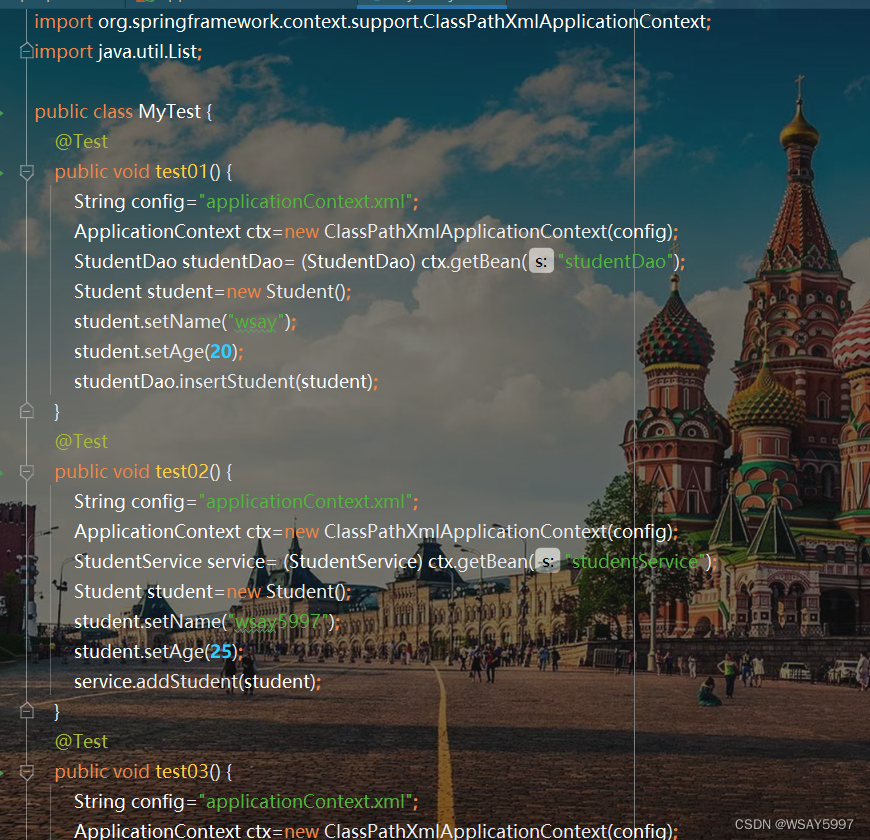
1.1测试
@Test
public void test01() {
String config="applicationContext.xml";
ApplicationContext ctx=new ClassPathXmlApplicationContext(config);
StudentDao studentDao= (StudentDao) ctx.getBean("studentDao");
Student student=new Student();
student.setName("wsay");
student.setAge(20);
studentDao.insertStudent(student);
}
?结果
1.2测试
@Test
public void test02() {
String config="applicationContext.xml";
ApplicationContext ctx=new ClassPathXmlApplicationContext(config);
StudentService service= (StudentService) ctx.getBean("studentService");
Student student=new Student();
student.setName("wsay5997");
student.setAge(25);
service.addStudent(student);
}
?结果
1.3测试
@Test
public void test03() {
String config="applicationContext.xml";
ApplicationContext ctx=new ClassPathXmlApplicationContext(config);
StudentService service= (StudentService) ctx.getBean("studentService");
List<Student> students=service.queryStudent();
for (Student stu : students) {
System.out.println("stu = " + stu);
}
}
结果?
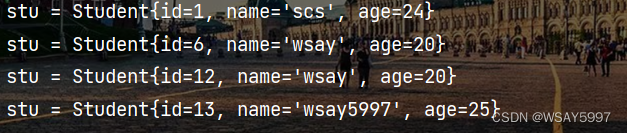
五.总结
spring集成mybatis实现步骤: 1.使用mysql数据库创建表 2.创建maven项目 3.加入依赖
mybatis和spring整合的时候,事务是自动提交的。 4.创建实体类Student 5.创建dao接口和mapper文件 6.编写mybatis主配置文件 7.创建service接口和它的实现类 8.创建spring的配置文件
9.创建jdbc.properties外部文件
10.测试
以上就是Spring整合MyBatis的内容。下一篇博文:Spring配置扩展
|