个人私活做了一个关于博物馆相关网站和管理后台,可以中英文切换,架构springBoot+mysql+layui
项目部分截图
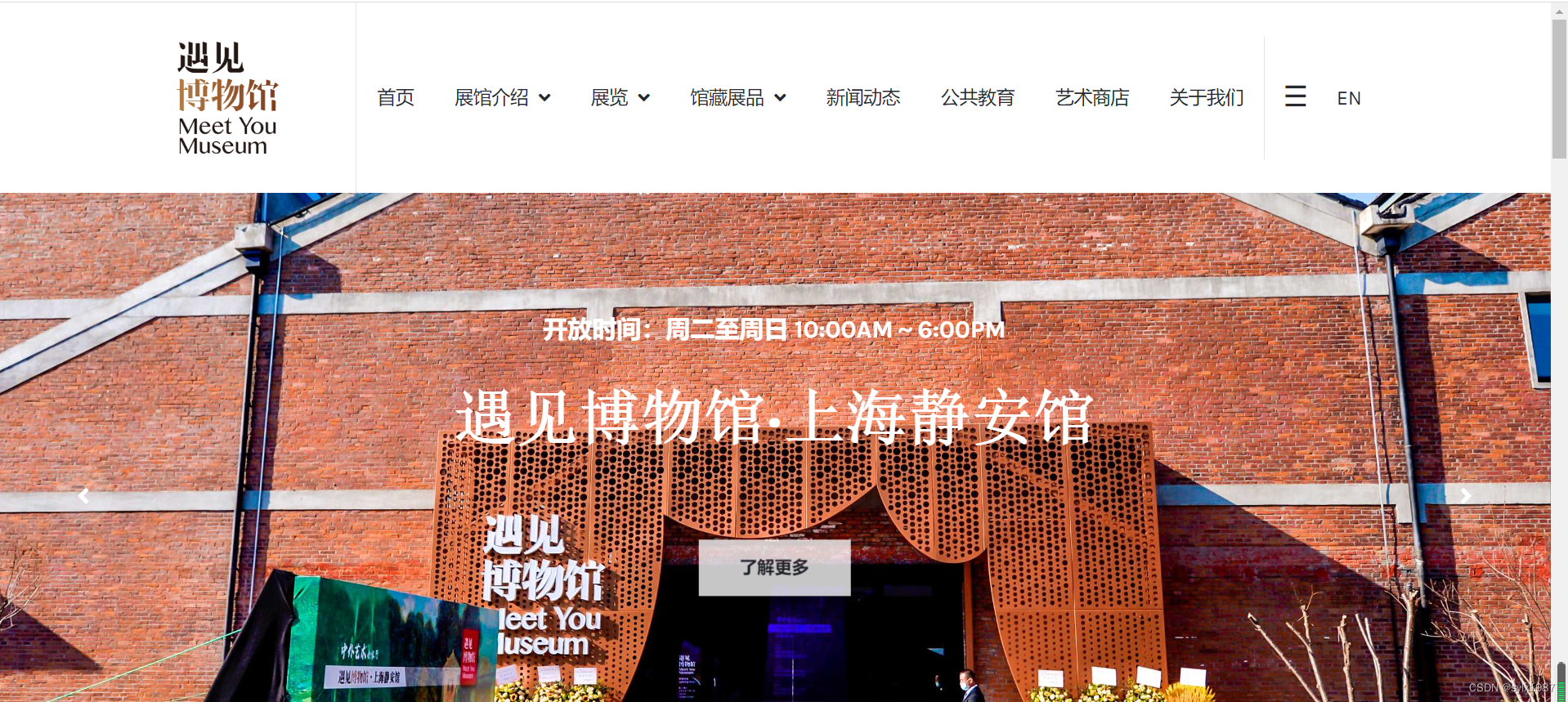
?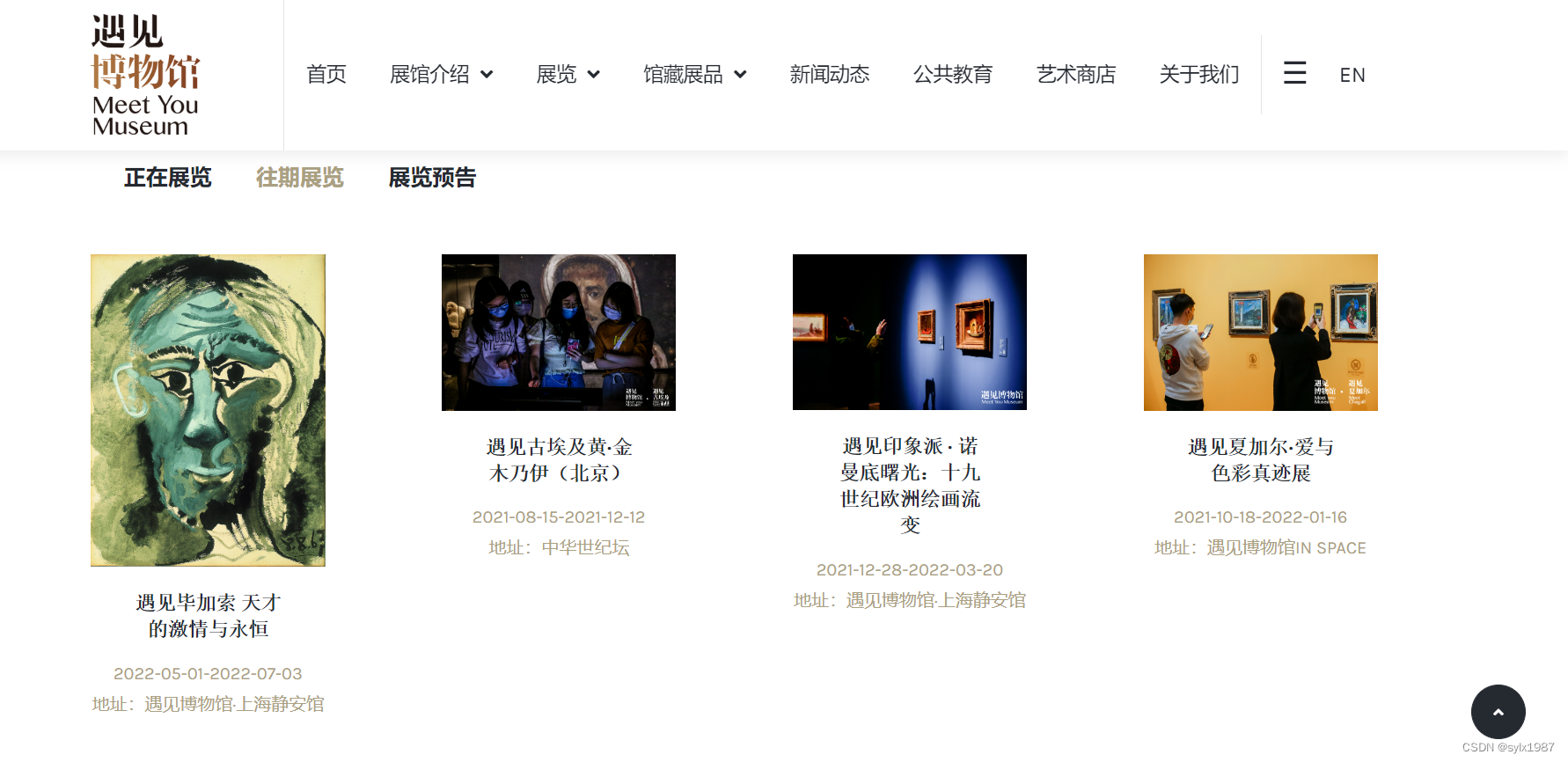
、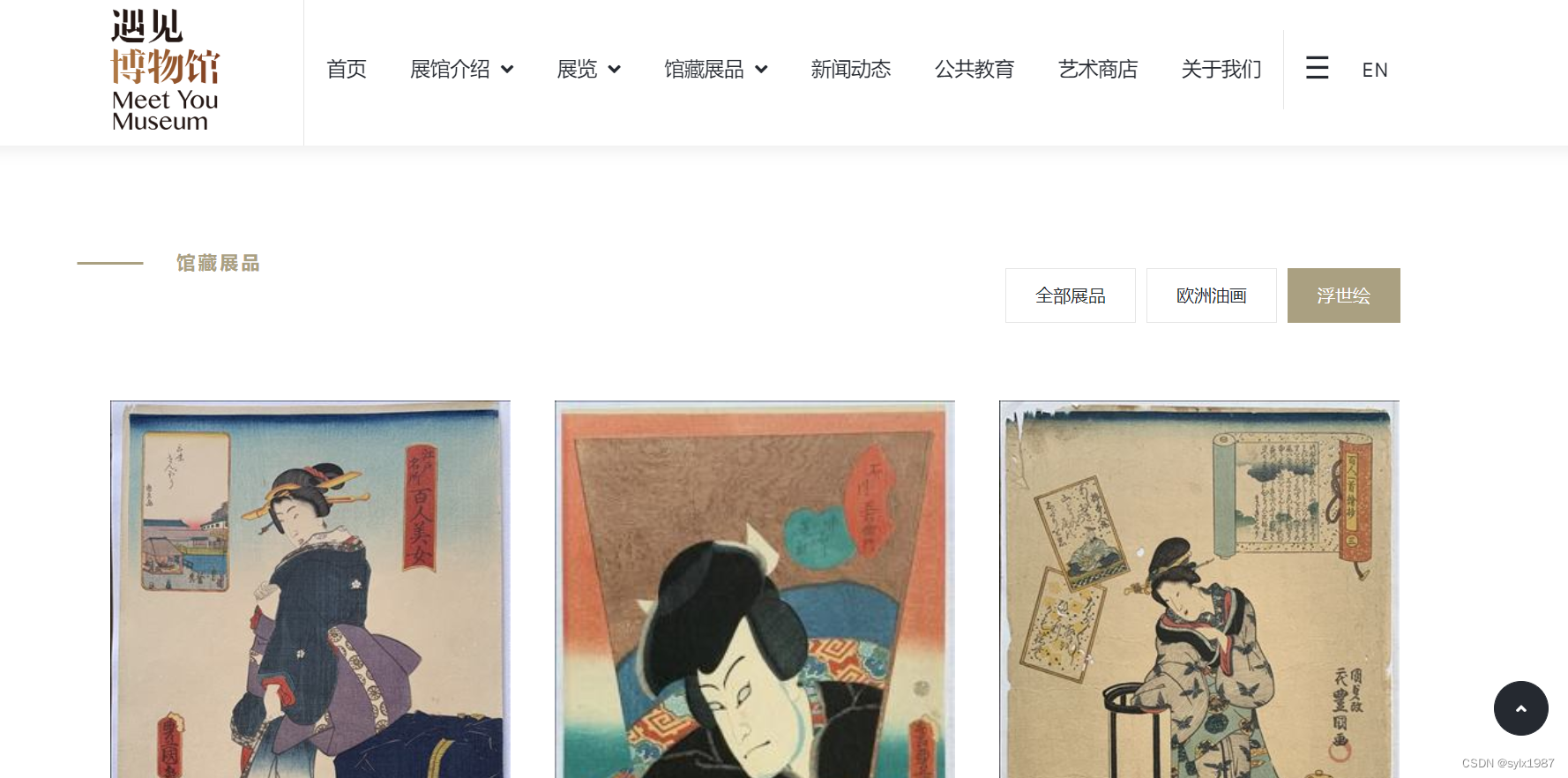
?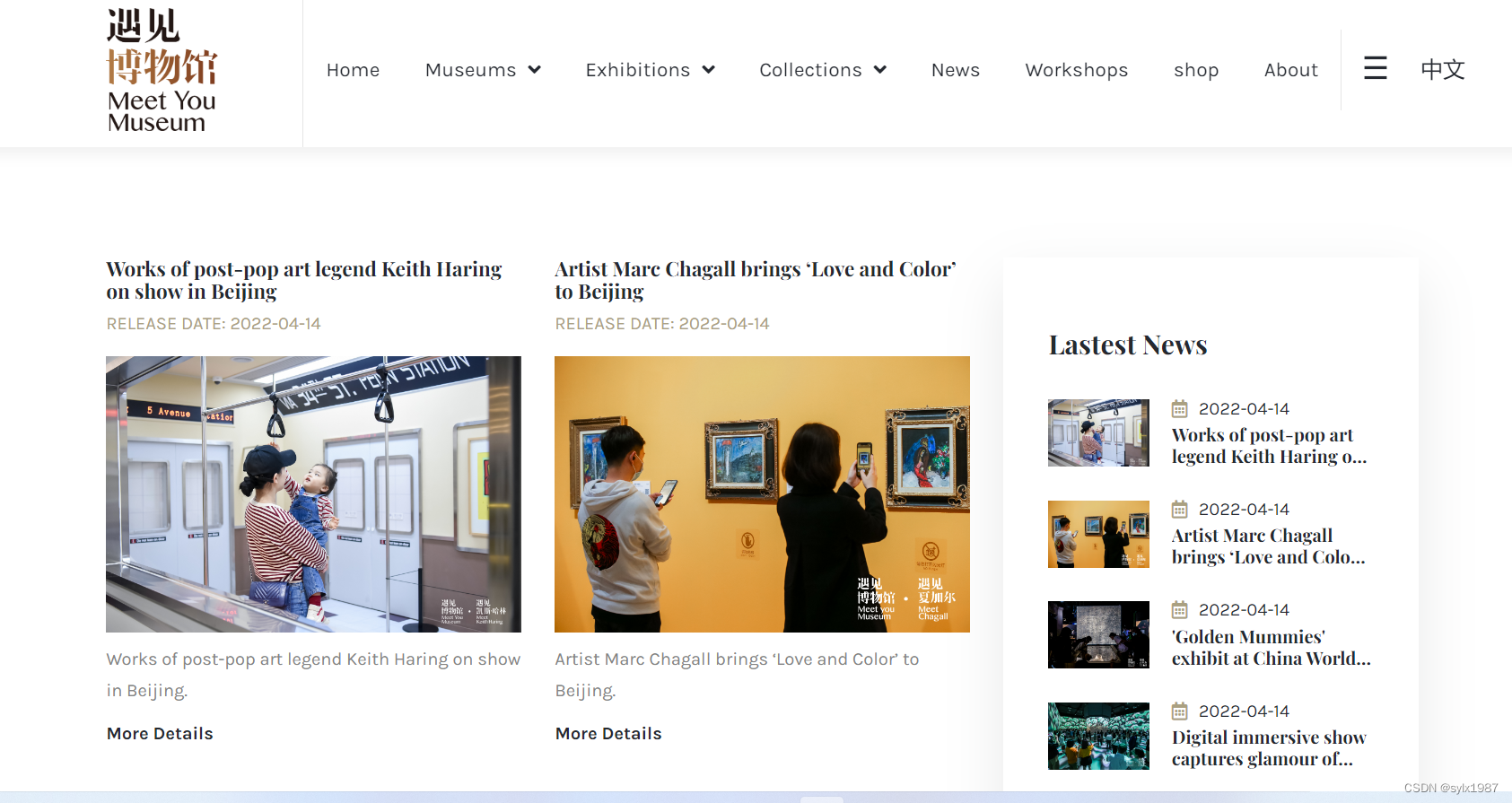
后台管理部分截图
?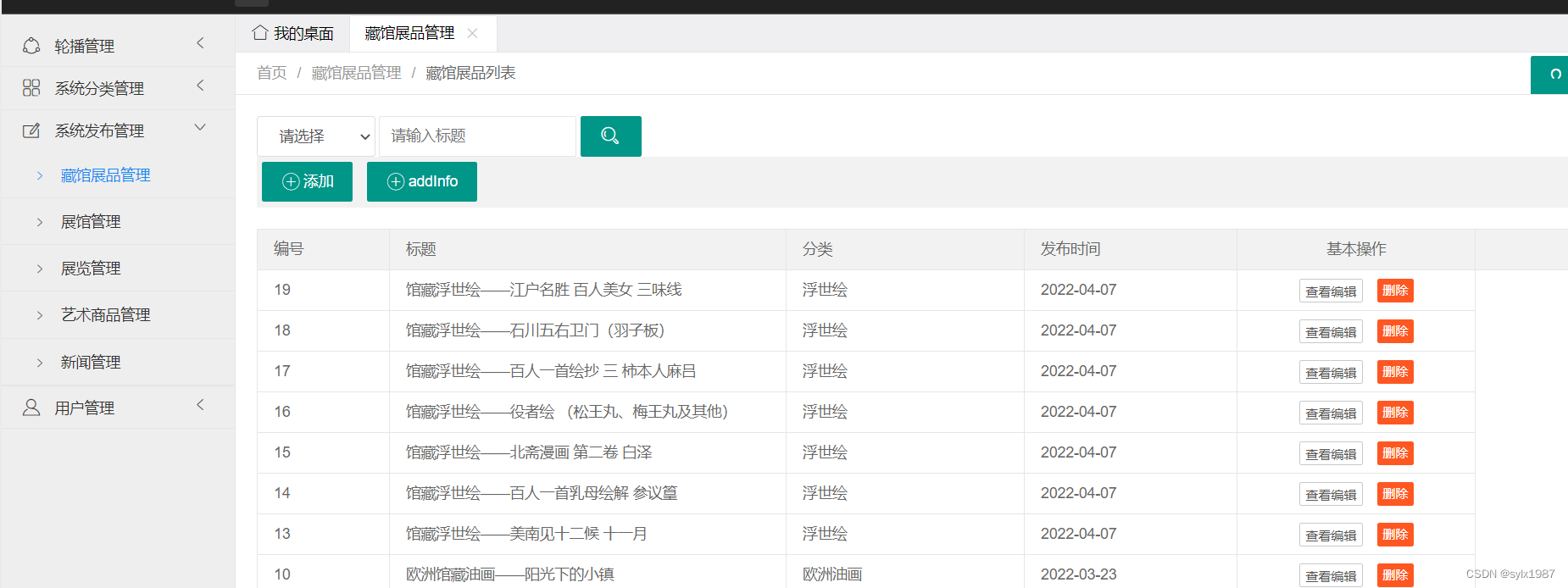
?
?
代码架构
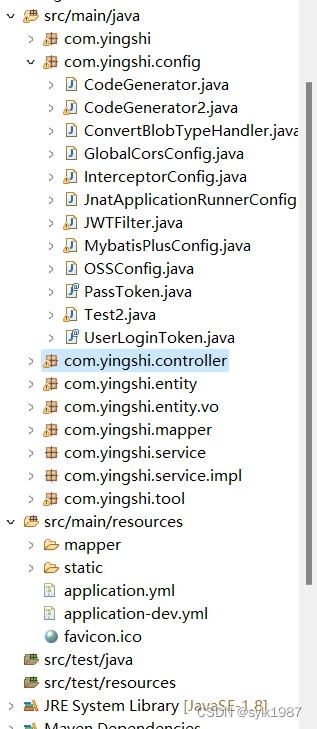
?部分代码,代码里有自己封装的代码生成工具,可快速做基本代码构架
package com.yingshi.controller;
import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; import java.util.HashMap; import java.util.List; import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; import com.baomidou.mybatisplus.core.metadata.IPage; import com.baomidou.mybatisplus.extension.plugins.pagination.Page; import com.yingshi.entity.Exhibition; import com.yingshi.entity.vo.ExhibitionVo; import com.yingshi.mapper.ExhibitionMapper;
/** ?* <p> ?* ?不要枯燥的重复工作,让更多的时间去创造价值!---4c4为你腾出更多的时间。 ?* </p> ?* ?* @author www.4c4.cn ?* @since Fri Mar 04 11:29:17 CST 2022 ?*/ @RestController @RequestMapping("/exhibition") public class ExhibitionController { ?? ?@Autowired ?? ?ExhibitionMapper exhibitionMapper; ?? ? ?? ? ?? ?/** ?? ? * 保存操作 ?? ? * @return ?? ? */ ?? ?@RequestMapping("/v1/save") ?? ?public Map<String, Object> save(Exhibition exhibition){ ?? ??? ? //SimpleDateFormat ?format = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss"); ?? ??? ?if(exhibition.getStates() == null) { ?? ??? ??? ?exhibition.setStates(1); ?? ??? ? } ?? ??? ? exhibition.setCdate(new Date()); ?? ??? ? Map<String, Object> map = new HashMap<String, Object>(); ?? ??? ? exhibitionMapper.insert(exhibition); ?? ??? ? map.put("code", 0); ?? ??? ? return map; ?? ?} ?? ? ?? ?/** ?? ? * 分页查询 ?? ? * @param page ?? ? * @param limit ?? ? * @return ?? ? */ ?? ?@RequestMapping("/v1/getAll") ?? ?public Map<String, Object> getAll(Long page,Long limit,String title,String type,Integer state){ ?? ??? ?Map<String, Object> map = new HashMap<String, Object>(); ?? ??? ?if(page == null && limit == null){ ?? ??? ??? ?map.put("msg","参数有误"); ?? ??? ??? ?map.put("code",1); ?? ??? ??? ?return map; ?? ??? ?} ?? ??? ?Date date = new Date(); ?? ??? ?SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); ?? ??? ?String format = sdf.format(date); ?? ??? ?QueryWrapper<ExhibitionVo> queryWrapper = ?new QueryWrapper<>(); ?? ??? ?if(title != null && title.length() > 0) { ?? ??? ??? ?queryWrapper.like("title", title); ?? ??? ?} ?? ??? ?if(type != null && type.length() > 0) { ?? ??? ??? ?queryWrapper.eq("e.type", type); ?? ??? ?} ?? ??? ?if(state != null ) { ?? ??? ??? ?queryWrapper.eq("e.states", state); ?? ??? ?} ?? ??? ??? ? ?? ??? ??? ? ?? ??? ??? ?/* ?? ??? ??? ? * if("1".equals(state)) {//预告 queryWrapper.lambda() ?? ??? ??? ? * .ge(Exhibition::getStime,format); ?? ??? ??? ? *? ?? ??? ??? ? * } if("2".equals(state)) {//正在 queryWrapper.lambda() ?? ??? ??? ? * .le(Exhibition::getStime,format) .ge(Exhibition::getEtime,format); ?? ??? ??? ? *? ?? ??? ??? ? * } if("3".equals(state)) { queryWrapper.lambda() ?? ??? ??? ? * //.le(Exhibition::getEtime,format) .le(Exhibition::getEtime,format); } ?? ??? ??? ? */ ?? ??? ? ?? ??? ? ?? ??? ?queryWrapper.select().orderByDesc("cdate"); ?? ??? ?Page<ExhibitionVo> pageObject = new Page<>(page , limit); ?? ??? ?IPage<ExhibitionVo> exhibitionPage = exhibitionMapper.findByPage(pageObject, queryWrapper); ?? ??? ?map.put("code", 0); ?? ??? ?map.put("msg",""); ?? ??? ?map.put("count",exhibitionPage.getTotal() ); ?? ??? ?map.put("newdate",format ); ?? ??? ?map.put("count",exhibitionPage.getTotal() ); ?? ??? ?map.put("data", exhibitionPage.getRecords()); ?? ??? ?return map; ?? ?} ?? ? ?? ? ?? ? ?? ?/** ?? ? * 根据id更新数据 ?? ? * @param name ?? ? * @param id ?? ? * @return ?? ? */ ?? ?@RequestMapping("/v1/edit") ?? ?public Map<String, Object> edit(Exhibition exhibition){ ?? ??? ?Map<String, Object> map = new HashMap<String, Object>(); ?? ??? ?exhibitionMapper.updateById(exhibition); ?? ??? ?map.put("code", 0); ?? ??? ?map.put("msg","更新成功"); ?? ??? ?return map;?? ??? ?? ?? ?} ?? ?@RequestMapping("/v1/test") ?? ?public Map<String, Object> test(ExhibitionVo exhibition,Long page,Long limit,String title,String type){ ?? ??? ?Map<String, Object> map = new HashMap<String, Object>(); ?? ??? ?QueryWrapper<ExhibitionVo> queryWrapper = ?new QueryWrapper<>(); ?? ??? ? ?? ??? ?if(title != null && title.length() > 0) { ?? ??? ??? ?queryWrapper.like("title", title); ?? ??? ?} ?? ??? ?if(type != null && type.length() > 0) { ?? ??? ??? ?queryWrapper.eq("type", type); ?? ??? ?} ?? ??? ?queryWrapper.select().orderByDesc("cdate"); ?? ??? ?Page<ExhibitionVo> pageObject = new Page<>(page , limit); ?? ??? ?IPage<ExhibitionVo> exhibitionPage = exhibitionMapper.findByPage(pageObject, queryWrapper); ?? ??? ?map.put("code", 0); ?? ??? ?map.put("msg",""); ?? ??? ?map.put("count",exhibitionPage.getTotal() ); ?? ??? ?map.put("count",exhibitionPage.getTotal() ); ?? ??? ?map.put("data", exhibitionPage.getRecords()); ?? ??? ? ?? ??? ?return map;?? ??? ?? ?? ?} ?? ? ?? ?/** ?? ? * 根据id删除数据 ?? ? * @param id ?? ? * @return ?? ? */ ?? ?@RequestMapping("/v1/dele") ?? ?public Map<String, Object> dele(Integer id){ ?? ??? ?Map<String, Object> map = new HashMap<String, Object>(); ?? ??? ?if(id == null){ ?? ??? ??? ?map.put("msg","参数有误"); ?? ??? ??? ?map.put("code",1); ?? ??? ??? ?return map; ?? ??? ?} ?? ??? ?exhibitionMapper.deleteById(id); ?? ??? ?map.put("code", 0); ?? ??? ?map.put("msg","删除成功"); ?? ??? ?return map; ?? ?} ?? ? ?? ?/** ?? ? * 根据id查询数据 ?? ? * @param id ?? ? * @return ?? ? */ ?? ?@RequestMapping("/v1/getById") ?? ?public Map<String, Object> getById(Integer id){ ?? ??? ?Map<String, Object> map = new HashMap<String, Object>(); ?? ??? ?if(id == null){ ?? ??? ??? ?map.put("msg","参数有误"); ?? ??? ??? ?map.put("code",1); ?? ??? ??? ?return map; ?? ??? ?} ?? ??? ?Exhibition entity = exhibitionMapper.selectById(id); ?? ??? ?map.put("code", 0); ?? ??? ?map.put("data",entity); ?? ??? ?return map;?? ? ?? ?} } ?
源码下载地址
?
|