第一步
axios插件的安装
npm install axios
第二步
在main.js里引入axios,这里引入即为全局引入
import axios from "axios"
Vue.prototype.$http = axios
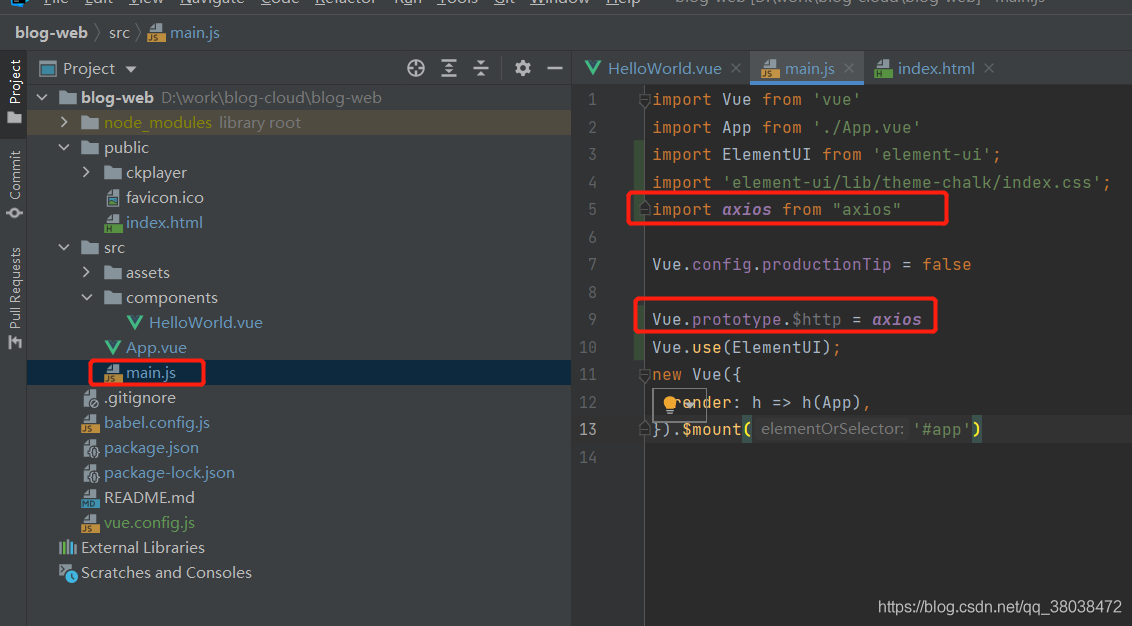
在main.js里引入之后,既可以在vue组件中使用 例:HelloWord.vue页面
<el-button type="primary" @click="btnClick">主要按钮</el-button>
btnClick() {
let url1 = 'https://api.coindesk.com/v1/bpi/currentprice.json';
let url2 = 'http://localhost:9020/test';
this.$http .get(url1).then(response => {
console.log(response);
})
}
初步实现了数据请求,但此时请求url2还存在着跨域请求问题;
第三步
注释: 既然使用axios直接进行跨域访问不可行,我们就需要配置代理了。代理可以解决的原因:因为客户端请求服务端的数据是存在跨域问题的,而服务器和服务器之间可以相互请求数据,是没有跨域的概念(如果服务器没有设置禁止跨域的权限问题),也就是说,我们可以配置一个代理的服务器可以请求另一个服务器中的数据,然后把请求出来的数据返回到我们的代理服务器中,代理服务器再返回数据给我们的客户端,这样我们就可以实现跨域访问数据。
准备工作:安装所需中间件和插件等,比如axios 配置代理,对vue.config.js文件中的proxy属性,作如下处理:
module.exports = {
devServer: {
open: true,
host: '0.0.0.0', // 前端IP地址
port: 8080, // 前端端口号
https: false,
// 配置不同的后台API地址
proxy: {
'/api': { // 请求的时候用这个/api就可以,无需在加协议、IP和端口; 例: http://localhost:9011/test请求时,只需使用/api/test即可
target: `http://localhost:9011`, // 接口服务IP地址
ws: true,
changOrigin: true,//允许跨域
pathRewrite: {
'^/api': '/'
}
}
}
}
}
第四步
在具体使用axios的地方,修改url如下即可(添加“/api”前缀)
btnClick() {
let url1 = 'https://api.coindesk.com/v1/bpi/currentprice.json';
let url2 = '/api/test';
this.$http .get(url2).then(response => {
console.log(response);
})
}
|