结合vuex实现自定义路由回退按钮
简洁版,只提供思路,代码简单,追求看懂。
使用vuex创建数组,存储每次路由跳转的path,模拟路由历史记录的push,pop,replace等操作,回退按钮根据此数组来实现相关逻辑。
以下为路由表代码
export default new Router({
routes: [
{path:"/main",name:"main",component:Main},
{path:"/details",name:"details",component:Details},
{path:"/profile",name:"profile",component:Profile},
{path:"/",redirect:"/main"},
],
mode:'history'
})
三个简单的页面 分别为首页main 个人页profile 详情页details 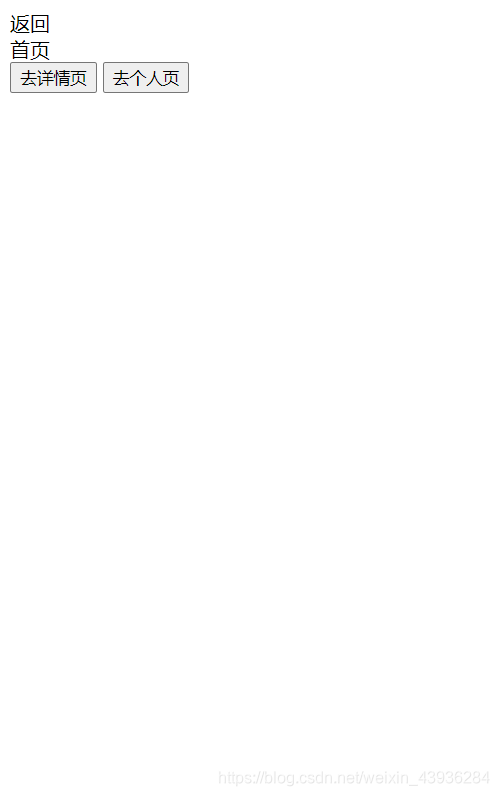
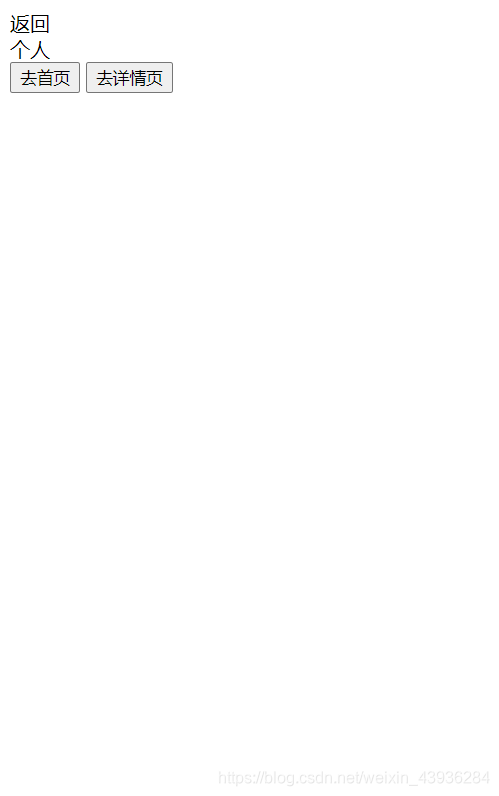 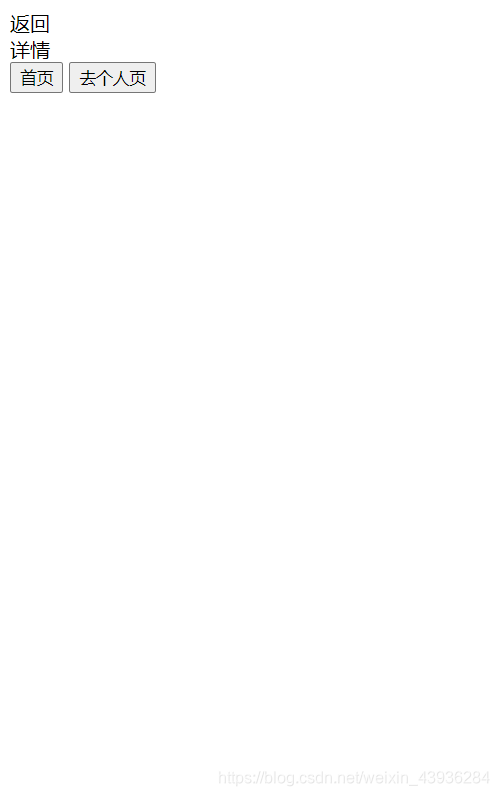 去详情页,个人页为push方式跳转,去首页为replace 返回二字可点击进行回退功能
首页 个人页 详情页 代码如下
methods: {
go(path) {
this.$store.commit("changeOprate",{type:'push'})
this.$router.push({
name: path,
});
},
},
methods: {
go(path) {
if (path == "main") {
this.$store.commit("changeOprate", { type: "replace" });
this.$store.commit("popRouterArr", {index:1})
this.$router.replace({
name: path,
});
} else {
this.$store.commit("changeOprate", { type: "push" });
this.$router.push({
name: path,
});
}
},
},
go(path) {
if (path == "main") {
this.$store.commit("changeOprate", { type: "replace" });
this.$store.commit("popRouterArr", {index:1})
this.$router.replace({
name: path,
});
} else {
this.$store.commit("changeOprate", { type: "push" });
this.$router.push({
name: path,
});
}
},
vue store代码:
import Vuex from "vuex"
Vue.use(Vuex)
export default new Vuex.Store({
state:{routerArr:[],routerOprate:''}, //记录存储路由的数组 以及跳转方式的记录
mutations:{
//添加一条记录
pushRouterArr(state,{path}) {
state.routerArr.push(path)
},
//替换末尾的一条记录
replaceRouterArr(state,{path}) {
state.routerArr[state.routerArr.length-1] = path
},
//删除最后一条记录
popOneRouterArr(state) {
state.routerArr.length = state.routerArr.length-1
},
//当数组中出现重复path值时 ,回退按钮只根据第一次path值出现的位置进行回退,回退成功后需要以当前位置截取整个数组
popRouterArr(state,{index}) {
// if (param.index > 0) {return}
state.routerArr.length = index
},
changeOprate(state,{type}){
state.routerOprate = type
}
},
getters: {
getRouterArr(state) {
return state.routerArr
},
getRouterOprate(state) {
return state.routerOprate
},
}
})
回退按钮放在app.vue中
<template>
<div id="app">
<header @click="back">返回</header>
<router-view />
</div>
</template>
在app.vue中对route进行监听
watch: {
$route(to, from) {
let len = this.getRouterArr.length;
if (len === 0) { //加载页面后第一次进行路由跳转时
if (this.getRouterOprate === "push") {
//向数组中添加两条记录
let path = from.path;
this.pushRouterArr({ path });
path = to.path;
this.pushRouterArr({ path });
} else if (this.getRouterOprate === "replace") {
let path = to.path;
this.pushRouterArr({ path });
}
} else {
if (
//处理浏览器返回按钮
from.path === this.getRouterArr[len - 1] &&
to.path === this.getRouterArr[len - 2] &&
this.getRouterOprate !== "push" &&
this.getRouterOprate !== "replace"
) {
console.log(123456567756);
this.popOneRouterArr();
return;
}
if (this.getRouterOprate === "back") {
// 此间处理回退事件
let adaptResult = {};
this.getRouterArr.forEach((item, index, list) => {
if (to.path === item) {
adaptResult.index = index - list.length + 1;
return;
}
});
let index = adaptResult.index;
this.popRouterArray({ index });
} else if (this.getRouterOprate === "replace") {
this.changeOprate({ type: "" });
let path = to.path;
this.replaceRouterArr({ path });
} else if (this.getRouterOprate === "push") {
this.changeOprate({ type: "" });
let path = to.path;
this.pushRouterArr({ path });
}
}
console.log(this.getRouterArr);
},
},
computed: {
...mapGetters(["getRouterArr", "getRouterOprate"]),
},
methods: {
back() {
//路有记录为1或0时不进行回退
if (this.getRouterArr.length <= 1) {
return;
} else {
//根据数组计算回退位置location 同时计算数组截断位置
let x = this.getRouterArr[this.getRouterArr.length - 1];
console.log(x);
let location = 1;
let index = 0;
for (let i = 0; i < this.getRouterArr.length; i++) {
if (x == this.getRouterArr[i]) {
location = this.getRouterArr.length - i;
index = i;
break
}
}
this.$router.go(-location);
//进行数组的截取操作
if (index > 0) {
this.popRouterArr({ index });
} else {
this.popOneRouterArr();
}
}
},
...mapMutations([
"popOneRouterArr",
"pushRouterArr",
"replaceRouterArr",
"popRouterArr",
"changeOprate",
]),
},
|