前言
提示:以下是本篇文章正文内容,下面案例可供参考
一、封装
1、布局
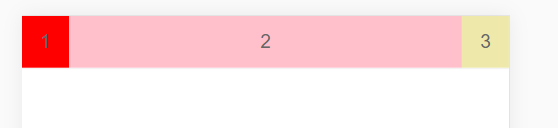
- 左右两侧我们可以用来放置图标之类的
- 中间部分我们用来保存内容
2、实现
- 直接在slot 上是不可以书写样式的
- 将slot 放入div 中,然后书写大体的布局样式
<template>
<div class="nar-bar">
<div class="left"><slot name="left"></slot></div>
<div class="center"><slot name="center"></slot></div>
<div class="right"><slot name="right"></slot></div>
</div>
</template>
<script>
export default {
};
</script>
<style scoped>
.nar-bar {
display: flex;
line-height: 44px;
z-index: 100;
text-align: center;
box-shadow: 0 1px 1px rgba(100, 100, 100, 0.1);
}
.left,.right {
width: 40px;
}
.center {
flex: 1;
}
</style>
二、使用
1.引入并设置内容+简单布局
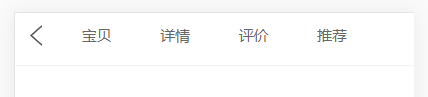
<template>
<nav-bar>
<div slot="left" class="left">
<img src="@/assets/images/common/back.svg" alt="" />
</div>
<div slot="center" class="center">
<div v-for="(item, index) in titles" :key="index">
{{ item }}
</div>
</div>
</nav-bar>
</template>
<script>
import NavBar from "components/common/navbar/NavBar";
export default {
components: {
NavBar
},
data() {
return {
titles: ["宝贝", "详情", "评价", "推荐"]
};
},
methods: {}
};
</script>
<style scoped>
.left img {
margin-top: 10px;
}
.center {
display: flex;
justify-content: space-around;
align-items: center;
font-size: 14px;
}
.active {
color: red;
}
</style>
2.添加效果,点击变色,返回上一级
- 1、添加点击事件 并传入参数(index)
- 2、定义一个变量 currentIndex 表示默认显示
- 3、点击后,获取索引 index 并 赋值 给currentIndex (此时 index == currentI 为 true )
- 4、使用class样式绑定对象写法 并将 其中值的true、false 判断交给 index == currentI
- 5、给left 中的元素添加点击事件,返回上一级
<template>
<nav-bar>
<div slot="left" class="left">
<img src="@/assets/images/common/back.svg" alt="" @click="goback" />
</div>
<div slot="center" class="center">
<div
v-for="(item, index) in titles"
:key="index"
@click="clickred(index)"
:class="{ active: index === currentIndex }"
>
{{ item }}
</div>
</div>
</nav-bar>
</template>
<script>
import NavBar from "components/common/navbar/NavBar";
export default {
components: {
NavBar
},
data() {
return {
titles: ["宝贝", "详情", "评价", "推荐"],
currentIndex: 0
};
},
methods: {
clickred(index) {
this.currentIndex = index;
},
goback() {
this.$router.go(-1);
}
}
};
</script>
<style scoped>
.left img {
margin-top: 10px;
}
.center {
display: flex;
justify-content: space-around;
align-items: center;
font-size: 14px;
}
.active {
color: red;
}
</style>
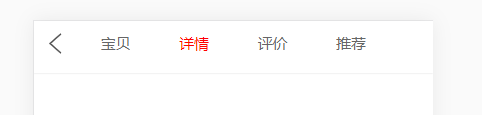
|