1、jquery和js对象的转换
让我们先看看它们的区别吧!
let oLi = document.querySelectorAll('li');
let $oLi = $('li');

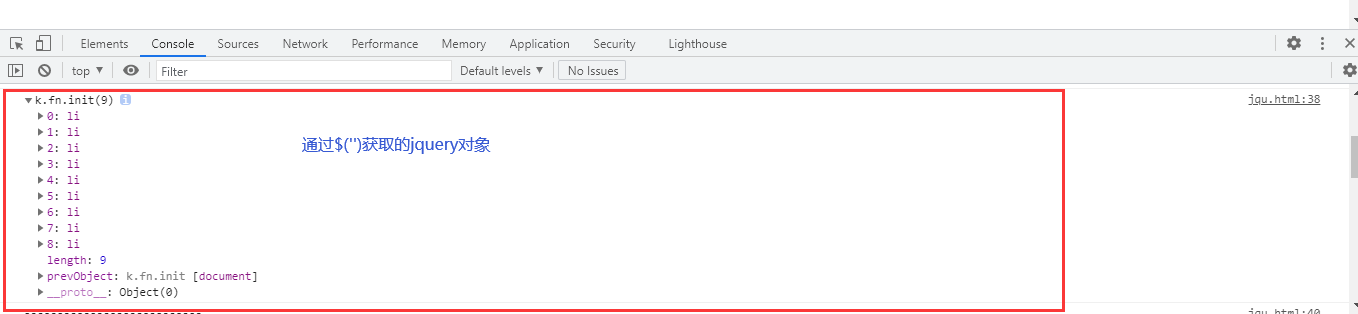 可以看出,它们的结果很相似,但分别属于js对象和jquery对象,那怎么转化呢?
console.log($(oLi));
console.log($oLi[0]);
console.log($oLi[1]);
console.log($oLi[3]);
2、创建元素
$(标签)
$('<p>创建的p标签</p>');
$('<p></p>').html('创建的p');
3、插入元素
ele.after(); 从元素后面插入 ele.insertAfter(); 从元素后面插入 ele.before(); 从元素前面插入 ele.insertBefore(); 从元素前面插入
$('#box').after($p);
$p.insertAfter($('#box'));
$p.insertBefore($('#box'));
$('#box').before($p);
ele.append(); 内部尾部追加 ele.appendTo() 内部尾部追加 ele.prependTo() 内部前面追加 ele.prepend(); 内部前面追加
$('#box').append($p);
$p.appendTo($('#box'))
$p.prependTo($('#box'))
$('#box').prepend($p);
4、元素替换
ele1.replaceWith(ele2); ele1替换为ele2 ele2.replaceAll(ele1);
$('#box').replaceWith($p);
$p.replaceAll($('#box'));
5、元素删除
ele.empty() 仅清空子代元素 ele.remove() 清空本身以及子代元素
$(odiv).empty();
$(odiv).remove();
6、元素的尺寸
innerWidth():内部宽(可视宽) width+padding outerWidth():外部宽(占位宽) width+padding+border outerWidth(true):外部宽(占位宽) width+padding+border+margin
let $Div = $('div');
$Div.height(200);
$Div.width(200)
$Div.css('border', '5px red solid');
$Div.css('padding', '5px');
$Div.css('margin', '5px');
console.log($Div.innerWidth());
console.log($Div.innerHeight());
console.log($Div.outerHeight());
console.log($Div.outerWidth(true));
7、元素的位置
offset(): 获取相对于页面的left和top(对象) offset({‘left’:100,‘top’:100}): 设置相对于页面的left和top(对象) postion(): 获取相对于具有定位的父级元素的left和top(对象)
console.log($Div.offset());
$Div.offset({
'left': '400',
'top': '300',
})
console.log($Div.offset());
8、页面滚出的距离
$(window).scrollTop() 获取页面滚动出去的高度 $(window).scrollTop(数值) 设置页面滚动出去的高度
$('body').css('height', '10000px');
window.onscroll = function () {
console.log($(window).scrollTop());
console.log($(window).scrollLeft());
}
9、元素的事件绑定
元素.on(事件类型,事件处理函数) 元素.事件类型(事件处理函数) 括号里可以传参 元素.hover(事件处理函数1,事件处理函数2)
$div.on('click', function () {
$(this).css('width','100px');
$(this).css('height','100px');
})
$div.click(function () {
$(this).css('width','100px');
$(this).css('height','100px');
})
$div.click({msg:"haha",info:'信息'},function (e) {
console.log(e.data);
})
$div.hover(function(){
$(this).css('background','green')
},function(){
$(this).css('background','yellow')
})
10、jquery实现事件委托
$('父元素').on('click','子元素',function(e){
var ev = e|| window.event;
var t = ev.srcElement||ev.target;
})
11、显示与隐藏
show() 显示 hide() 隐藏
$('.btn1').on('click',function(){
$('div').show(1000,'linear',function(){
alert('执行完毕')
});
})
$('.btn2').on('click',function(){
$('div').hide(500);
})
12、淡入淡出
$('.btn1').on('click',function(){
$('div').fadeIn();
})
$('.btn2').on('click',function(){
$('div').fadeOut();
})
经典案例:jquery实现轮播图淡入淡出
let arr = ['red','pink','blue','gray','green'];
$('ul>li').each(function(index,item){
console.log(index);
$(item).css('background',arr[index])
})
$('ol>li').on('click',function(){
$(this).addClass('l1').siblings('li').removeClass('l1');
$('ul>li').eq($(this).index()).fadeIn().siblings('li').fadeOut();
})
13、jquery实现动画
$('ul').animate({
'left': -nindex * $('ul>li').innerWidth() + 'px',
})
经典案例:jquery实现元素的创建,追加,遍历,删除
let resu;
window.onload = function () {
resu = getLocal('score');
for (let i = 0; i < resu.length; i++) {
creat(resu[i]['姓名'], resu[i]['科目'], resu[i]['分数']);
$('.a1').on('click', function () {
$(this).parent().parent().remove();
})
}
}
function creat(name, sub, mark) {
let $Td1 = $('<td></td>').html(name);
let $Td2 = $('<td></td>').html(sub);
let $Td3 = $('<td></td>').html(mark);
let $Td4 = $('<td></td>').html('<a class="a1">删除</a>')
let $Tr = $('<tr></tr>');
$Tr.append($Td1);
$Tr.append($Td2);
$Tr.append($Td3);
$Tr.append($Td4);
$Tr.appendTo($('tbody'));
}
|