vuex的概念
vuex是什么
官方文档:https://vuex.vuejs.org/zh/ Vuex 是一个专为 Vue.js 应用程序开发的状态管理模式。 vuex 是 Vue 配套的 公共数据管理工具,可以方便的实现组件之间数据的共享 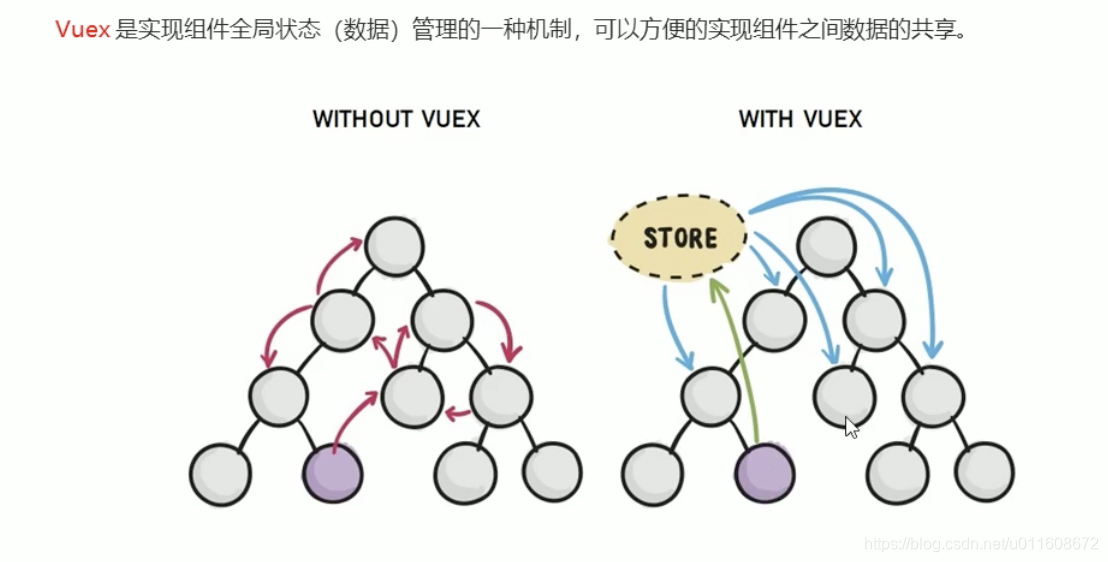
vuex统一管理状态的好处
- 能够再vuex中集中管理共享的数据,易于开发和后期维护
- 能够高效低实现组件之间的数据共享,提高开发效率
- 存储再vuex中的数据都是响应式的,能够实现保持数据与页面的同步
什么样的数据可以存储到vuex中
它可以把一些共享的数据,保存到 vuex 中,方便 整个程序中的任何组件直接获取或修改我们的公共数据;对于私有的数据还是放在data中去
vuex的基本使用
1、安装vuex依赖包
npm install vuex --save
2、导入vuex包
import Vuex from 'vuex'
Vue.use(Vuex)
3、创建store对象
const store = new Vuex.Store({
state:{ count:0 }
})
4、将store对象挂载到vue实例中
new Vue({
el:'#app',
render: h => h(App),
router,
store
})
vuex的核心概念
1、Vuex中的主要核心概念如下:
- State
- Mutation
- Action
- Getter
2、State
State 提供唯一的公共数据源,所有共享的数据都要统一放到Store 的State 中存储
const store = new Vuex.Store({
state:{ count:0 }
})
组件中访问State中数据的第一种方式
this.$store.state.全局数据名称
组件中访问State中数据的第二种方式
// 1、从vuexzhong 中按需导入 mapState 函数
import { mapState } from 'vuex'
通过刚才导入的mapState 函数,将当前组键需要的全局数据,映射为当前组件的computed 计算书籍;
// 2、将全局数据,映射为当前组件的计算属性
computed:{
...mapState(['count'])
}
展示
<template>
<div class="hello">
<h3>当前的最新count值为:{{count}}</h3>
</div>
</template>
<script>
import { mapState } from 'vuex'
export default {
data(){
return{}
},
computed:{
...mapState(['count'])
}
}
</script>
3、Mutation
Mutation 用于变更Store 中的数据
- 只能通过 mutation 变更 Store 数据,不可以直接操作 Store 中的数据
- 通过这种方式虽然操作起来稍微繁琐一点,但是可以集中监控所有数据的变化
const store = new Vuex.Store({
state: {
count:0
},
mutations: {
add(state){
state.count++
},
addN(state, step){
state.count += step
},
reduce(state){
state.count--
},
reduceN(state, step){
state.count -= step
}
}
})
触发 Mutation 的时候传递参数,第一种方式
methods:{
add1(){
this.$store.commit('add')
}
}
使用触发传递参数
const store = new Vuex.Store({
state: {
count:0
},
mutations: {
add(state){
state.count++
},
addN(state, step){
state.count += step
}
}
})
触发
methods:{
add1(){
this.$store.commit('add')
},
add2(){
this.$store.commit('addN', 3)
}
}
触发 Mutation 的第二种方式
// 1、从vuex中按需导入 mapMutations 函数
import { mapMutations } from 'vuex'
通过刚才导入的 mapMutations 函数,将需要的 mutations 函数,映射为当前组件的 methods 方法:
<script>
import { mapMutations, mapState} from 'vuex'
export default {
data(){
return{}
},
computed:{
...mapState(['count'])
},
methods:{
...mapMutations(['reduce', 'reduceN']),
reduce1(){
this.reduce()
},
reduce2(){
this.reduceN(3)
},
}
}
</script>
注意:
不要在mutations函数中,执行异步函数
4、Action
Action用于处理异步任务 如果通过异步操作变更数据,必须通过Action,而不能使用Mutation,但是在Action中还是要通过触发Mutation的方式间接变更数据
export default new Vuex.Store({
state: {
count:0
},
mutations: {
add(state){
state.count++
}
},
actions: {
addAsync(context) {
setTimeout(() => {
context.commit('add')
}, 1000);
}
}
})
触发actions的第一种方式
export default {
methods:{
add3(){
this.$store.dispatch('addAsync')
}
}
}
触发actions 异步任务的时候如何携带参数
export default new Vuex.Store({
state: {
count:0
},
mutations: {
addN(state, step){
state.count += step
},
},
actions: {
addNAsync(context,step) {
setTimeout(() => {
context.commit('addN', step)
}, 1000);
}
}
})
调用
export default {
methods:{
add4(){
this.$store.dispatch('addNAsync', 5)
}
}
}
触发actions的第二种方式
this.$store.dispatch() 是出发 actions 的第一种方式;
触发actions 的第二种方式
1、从vuex中按需到人 mapActions 函数 import { mapActions } from ‘vuex’
通过刚才导入的 mapActions 函数,将需要的 actions 函数,映射为当前组件的 methosd 方法:
2、将指定的actions函数,映射为当前组件的 methods 函数 methods:{ …mapActions([‘reduceAsync’, ‘reduceNAsync’]), }
触发代码
<template>
<div class="hello">
<h3>当前的最新count值为:{{count}}</h3>
<button @click="reduce3">-1 延迟</button>
<button @click="reduceNAsync(5)">-N 延迟</button>
</div>
</template>
<script>
import { mapActions,mapState } from 'vuex'
export default {
data(){
return{ }
},
computed:{
...mapState(['count'])
},
methods:{
...mapActions(['reduceAsync', 'reduceNAsync']),
reduce3(){
this.reduceAsync()
},
}
}
</script>
5、Getter
Getter 用于对 Store 中的数据进行加工处理,形成新的数据
- Getter 可以对 Store 中已有的数据加工处理之后形成新的数据,类似Vue的计算属性
- Store 中数据发生变化,Getter的数据也是会发生变化的
export default new Vuex.Store({
state: {
count:0
},
getters:{
showNum:state => {
return '当前最新的数据市是【' + state.count +'】'
}
},
})
调用 Getter
调用Getter的第一种方式
this.$store.getters.名称
页面html引用代码
<h3>{{ this.$store.getters.showNum }}</h3>
调用Getter的第二种方式
import { mapGetters } from ‘vuex’ computed:{ …mapGetters([‘showNum’]) },
页面html引用代码
<h3>{{ showNum }}</h3>
6、modules
modules是什么
Vuex 可以将每个特定的操作内容分成模块。每个模块都可以包含自己的state、mutation、action、getter;方便模块之间的区分 官网文档:https://vuex.vuejs.org/guide/modules.html
首先创建modulesA 、modulesB 、modulesC 三个模块

然后引入到store文件下的index.js中
import Vue from 'vue'
import Vuex from 'vuex'
import modulesA from './modulesA.js'
import modulesB from './modulesB.js'
import modulesC from './modulesC.js'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
name:'我是index'
},
mutations: {
add(state){
state.name = "我是index模块+++"
},
},
actions: { },
modules: {
a:modulesA,
b:modulesB,
c:modulesC
}
})
modulesA.js 文件
export default{
state:{
name:'我是aaa模块'
},
mutations:{
add(state){
state.name = "我是aaa模块+++"
}
},
actions:{},
getters:{}
}
modulesB.js 文件
export default{
state:{
name:'我是bbb模块'
},
mutations:{
add(state){
state.name = "我是bbb模块+++"
}
},
actions:{},
getters:{}
}
modulesC.js 文件 使用namespaced: true . 当模块被注册时,它的所有 getter、actions 和 mutations 都将根据模块注册的路径自动命名空间;这样模块更加独立或可重用
export default{
namespaced: true,
state:{
name:'我是ccc模块'
},
mutations:{
add(state){
state.name = "我是ccc模块+++"
}
},
actions:{},
getters:{}
}
最后调用和引用modules中的方法和数据内容
调用state中数据: $store.state.a.name`
调用所有mutations中同名的方法: this.$store.commit(‘add’)
调用独立模块的方法: this.$store.commit(‘c/add’)
$store.state.模块.名称 ===某个模块的数据内容
this.$store.commit('方法名') ====所有模块的,如果方法名一样,全部调用
this.$store.commit('模块名/方法名') ====调用独立模块的方法
App.vue
<template>
<div id="app">
<h2>模块</h2>
<p>渲染内容{{ $store.state.name }}</p>
<p>渲染内容{{ $store.state.a.name }}</p>
<button @click="handle">点击我!可调用所有非独立模块的相同名字的方法</button>
<button @click="handle2">点击我!可调用独立模块的方法</button>
</div>
</template>
<script>
export default {
name: 'App',
methods:{
handle(){
this.$store.commit('add')
},
handle2(){
this.$store.commit('c/add')
}
}
}
</script>
参考学习视频1:https://www.bilibili.com/video/BV1h7411N7bg?t=161&p=14 参考学习视频2:https://www.bilibili.com/video/BV1Xa4y1L7SV?t=1208
|