Vue
1. 指令
1.1 mustache
<div id="app">
<h2>{{message}}</h2>
<h2>{{message}},李银河!</h2>
<h2>{{firstName + lastName}}</h2>
<h2>{{firstName + ' ' +lastName}}</h2>
<h2>{{firstName}} {{lastName}}</h2>
<h2>{{counter * 2}}</h2>
</div>
<script src="../js/vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
message: '你好啊!',
firstName: 'kobe',
lastName: 'bryant',
counter:100,
}
})
</script>
1.2 v-once
v-once :
- 该指令后边不需要跟任何表达式
- 该指令表示元素和组件只渲染一次,不会随着数据的改变而改变
<div id="app">
<h2>{{message}}</h2>
<h2 v-once>{{message}}</h2>
</div>
<script src="../js/vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
message: '你好啊!'
}
})
</script>
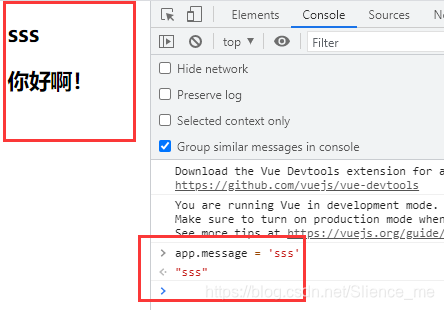
1.3 v-html
v-html :
- 该指令后边往往会跟上一个string类型
- 会将string的html解析出来并且渲染
<div id="app">
<h2>{{url}}</h2>
<h2 v-html="url"></h2>
</div>
<script src="../js/vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
message: '你好啊!',
url: '<a href="https://www.baidu.com">百度一下<a/>'
}
})
</script>

1.4 v-text
v-text :
- 该指令和Mustache比较相似:都是用于将数据显示在界面中
- 该指令通常情况下,接受一个string类型
- 相对不灵活,不容易拼接内容,一般不用
<div id="app">
<h2>{{message}},slience_me!</h2>
<h2 v-text="message">,slience_me!</h2>
</div>
<script src="../js/vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
message: '你好啊!'
}
})
</script>
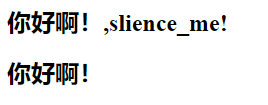
1.5 v-pre
v-pre :
- 该指令用于跳过这个元素和它的子元素的编译过程,用于显示原本的
Mustache 语法 - 原封不动的显示出来
<div id="app">
<h2>{{message}}</h2>
<h2 v-pre>{{message}}</h2>
</div>
<script src="../js/vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
message: '你好啊!'
}
})
</script>
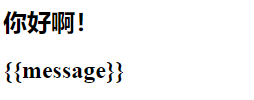
1.6 v-cloak
cloak:斗篷
v-cloak :
- 该指令防止不友好的{{message}}被看到
- 不会看到{{}}内容
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
[v-cloak] {
display: none;
}
</style>
</head>
<body>
<div id="app" v-cloak>
<h2>{{message}}</h2>
</div>
<script src="../js/vue.js"></script>
<script>
setTimeout(function () {
const app = new Vue({
el: '#app',
data: {
message: '你好啊!'
}
})
}, 1000)
</script>
</body>
</html>
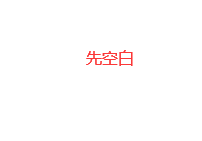 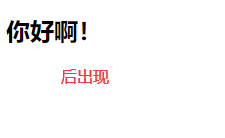
1.7 v-bind
1.7.1 基本使用
v-bind :
- 作用:动态绑定属性
- 缩写:
: - 预期:any(with argument) | Object (without argument)
- 参数:attrOrProp(optional)
- 例子:
<img :src="imgURL" alt="">
<div id="app">
<img v-bind:src="imgURL" alt="">
<a v-bind:href="aHref">百度一下</a>
<br>
<img :src="imgURL" alt="">
<a :href="aHref">百度一下</a>
</div>
<script src="../js/vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
message: '你好啊!',
imgURL:'https://cn.vuejs.org/images/logo.svg',
aHref: 'https://www.baidu.com'
}
})
</script>
1.7.2 动态绑定class
对象语法
<div id="app">
<h2 class="title" :class="getClasses()">{{message}}</h2>
<button v-on:click="btnClick">按钮</button>
</div>
<script src="../js/vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
message: '你好啊!',
isActive: true,
isLine: true
},
methods:{
btnClick:function (){
this.isActive = !this.isActive
},
getClasses:function () {
return {active: this.isActive,line: this.isLine}
}
}
})
</script>
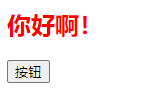 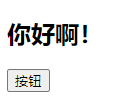
数组语法
<div id="app">
<h2 class="title" :class="['active', 'line']">{{message}}</h2>
<h2 class="title" :class="[active, line]">{{message}}</h2>
<h2 class="title" :class="getClasses()">{{message}}</h2>
</div>
<script src="../js/vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
message: '你好啊!',
active: 'aaaa',
line: 'bbbbbb'
},
methods: {
getClasses: function () {
return [this.active, this.line]
}
}
})
</script>
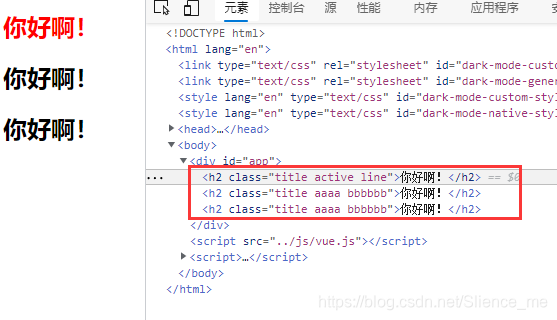
案例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
.active{
color: red;
}
</style>
</head>
<body>
<div id="app">
<ul>
<li v-for="(m, index) in movies" v-on:click=activeIndex=index :class={active:activeIndex===index}> {{index}}-{{m}}</li>
</ul>
</div>
<script src="../js/vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
movies:['海王','海尔兄弟','火影忍者','进击的巨人'],
activeIndex: 0
}
})
</script>
</body>
</html>
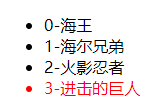 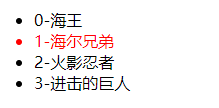
1.7.3 动态绑定style
对象绑定
数组绑定
|