Redux 是 JavaScript 状态容器,提供可预测化的状态管理。可以让你构建一致化的应用,运行于不同的环境(客户端、服务器、原生应用),并且易于测试。不仅于此,它还提供 超爽的开发体验,比如有一个时间旅行调试器可以编辑后实时预览。
Redux基本用法
一、Redux的npm下载
npm install --save redux
注意:不是全局安装,要安装在具体项目
二、引入Redux具体内容
在官方提供的react脚手架src目录中创建一个文件夹store 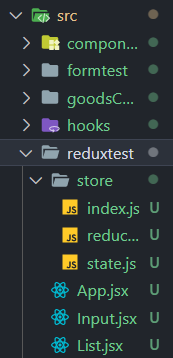 在store文件夹中创建三个文件,分别是index.js,reducer.js,state.js,分别代表的是引入模块,reducer操作函数和state数据。
1、首先打开index.js,(确保redux已经下载成功了),复制以下代码引入目录,并将store抛出。
import { createStore } from "redux";
import reducer from "./reducer";
import state from "./state"
const store = createStore(reducer,state)
export default store
2、打开state.js文件,建立一个空对象,并将state抛出。
const state = {
}
export default state
3、打开reducer.js文件,建议一个空函数,并将reducer抛出
const reducer = (state,action)=>{
return state
}
export default reducer;
到这里,我们前期的准备工作就基本完成了,下面说一下具体的操作
三、Redux在组件内的的具体操作
我这里有一个简易版todolist的小案例,点击Edite是弹出框编辑本条,点击del是删除本条,最终效果如下图所示 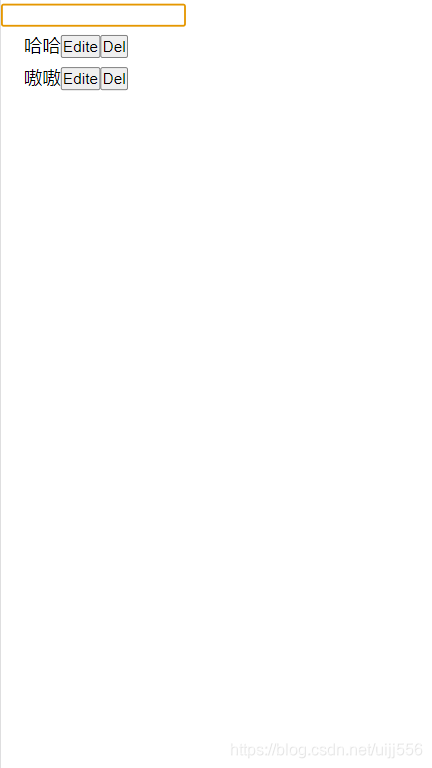 学过Vuex的小伙伴应该都知道$store.state吧,在组件内调取store内部的数据的方法,同样redux跟vuex其实差不多是一个东西,都是状态管理,所以,简单介绍一下在react的组件中调取和操作store中的数据的方法
* store对象
* store.getState() 获取store中的state数据
* store.dispatch(action) 向store中reducer派发新的action对象
* store.subscribe(()=>{}) 监听store数据的变化
1、首先要在组件中引入store
import store from './store'
2、直接在组件中这样写就可以拿到store state中的数据
render(){
return(
{store.getState."在state中定义的键名"}
)
}
不过我们并不推荐这么写,因为这样每次组件都会去请求store,会浪费性能,所以我们采取下面这个方法:
constructor(){
super()
this.state = store.getState()
store.subscribe(()=>{
this.setState(store.getState())
})
}
前面介绍到的redux在组件内的方法,监听也是放在constructor中是最好的,获取到值立马开始监控。
3、添加一个点击提交的回车事件(这里暂时只看onKeyUp) 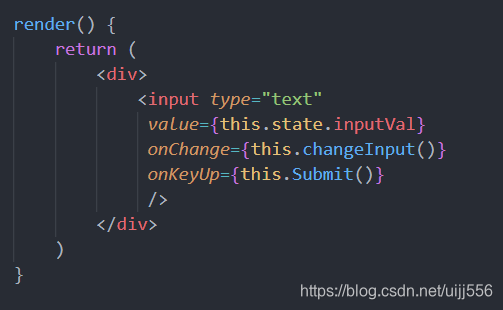 然后我们写一个回车事件Submit
Submit = ()=>{
return (e)=>{
if(e.keyCode === 13){
let action = {
type:"submit_input",
value:this.state.inputVal
}
store.dispatch(action)
}
}
}
可以观察到,
let action = {
type:"submit_input",
value:this.state.inputVal
}
store.dispatch(action)
这里就是传递action的地方,就是在这里将组件内定义好的action传递到reducer函数中的,
type:type参数必须要有,这是reducer判断你要干什么事所必需的
value:这是自定义的参数名,从这里开始,你可以定义很多参数名去传递数据
store.dispatch(action) :这是将action抛给reducer函数的方法
四、Redux在store内reduce操作函数的方法
以刚刚的回车事件提交函数为例,在store.dispatch将action抛给reducer函数后,先if判断一下传过来的type是什么,为什么这么做?因为在一个reducer函数中,可以传递很多 “if(type??){}” 进来,这样判断后reducer才能知道你传递的action到底是给哪个if用的。
为了不影响原本的数据和外部数据,先设置一个变量tempstate深复制原本的state数据,然后将tempstate抛出去赋值给state就大功告成了
const reducer = (state,action)=>{
if(action.type === 'submit_input'){
let tempstate = JSON.parse(JSON.stringify(state));
tempstate.inputValue = action.value;
tempstate.list.push(action.value)
tempstate.inputValue = ""
return tempstate;
}
return state
}
export default reducer;
|