? ????????????????????????????????Vue之购物车功能

?我们都知道vue是数据驱动和组件化的模式。意思就是只要绑定的数据改变其他的变化由vue去完成,无须再去操作dom。
我们来看看简单的购物车功能怎么实现:
1.先完成数据绑定
代码:
html:
<div id="test">
<table id="mytable">
<tr>
<th><input type="checkBox" @click="selectProduct(isSelectAll)" v-bind:checked="isSelectAll" />全选</th>
<th>商品</th>
<th>数量</th>
<th>单价(元)</th>
<th>金额(元)</th>
<th>操作</th>
</tr>
<tr v-for="(item,index) in productList">
<td><input type="checkBox" v-bind:checked="item.isSelect" @click="item.isSelect=!item.isSelect"/></td>
<td>{{item.name}}</td>
<td><span>
<a href="#" @click="item.number>0?item.number--:''">-</a>
</span><strong>{{item.number}}</strong><span>
<a href="#" @click="item.number++">+</a>
</span></td>
<td>{{item.price}}</td>
<td>{{item.price*item.number}}</td>
<td><a href="#" @click="deletePro(index)">删除</a></td>
</tr>
</table>
<div class="checkPro">
<div class="leftConent">
<span><a href="#" @click="deleteProduct">删除所选产品</a></span>
</div>
<div class="rightConent">
<span>{{getTotal.totalNum}}件商品总计:¥{{getTotal.totalPrice}}</span>
</div>
</div>
</div>
js:
new Vue({
el: "#test",
data: {
productList: [],
},
created() { // 当 vm 实例 的 data 和 methods 初始化完毕后,vm实例会自动执行created 这个生命周期函数
this.getAllList();
},
methods: {
getAllList() {
// 由于已经导入了 Vue-resource这个包,所以 ,可以直接通过 this.$http 来发起数据请求
this.$http.get("{:url('admin/shopping/create')}").then(result => {
// 注意: 通过 $http 获取到的数据,都在 result.body 中放着
// console.log(result.data)
var result = result.body
if (result.code === 0) {
// 成功了
this.productList = result.data
console.log(result.data)
} else {
// 失败了
alert('获取数据失败!')
}
})
},
}
});
我们先绑定好data对应的数据对应每一列。
2.实现+和-数量功能,其实很简单就是操作proNum的加和减。
<td><span>
<a href="#" @click="item.number>0?item.number--:''">-</a>
</span><strong>{{item.number}}</strong><span>
<a href="#" @click="item.number++">+</a>
</span></td>
我们只需要对proNum进行加减,则后面的金额会自动改变,因我们绑定了
{{item.price*item.number}}
3.删除功能。我们在遍历data集合的时候带上下标index,然后点击删除时我们再通过传递下标的方式进行删除集合的元素。
效果图:
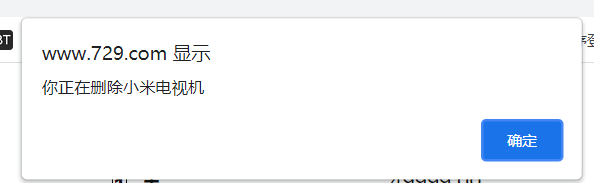
vue代码:?
deletePro : function(index){
// console.log(this.productList[index]);
alert("你正在删除"+this.productList[index].name);
this.productList.splice(index,1);
},
4.设置选中功能。实际上选中功能不会存到数据库,数据库也不会给我们传递是否选中。所以选中功能需要我们自己来创建。这里用vue的mounted来设置选中功能
vue代码:
mounted:function () {
var _this=this;
//为productList添加select(是否选中)字段,初始值为true
this.productList.map(function (item) {
_this.$set(item, 'select', true);
})
}
为productList挂载isSelect属性。
5.总商品数量和总价格。我们只需要在计算属性中实现getTotal并返回总数量和总价格即可
vue代码:
getTotal:function(){
var prolist = this.productList.filter(function (val) { return val.isSelect}),
totalPri = 0;
for (var i = 0,len = prolist.length; i < len; i++) {
totalPri+=prolist[i].price*prolist[i].number;
}
return {totalNum:prolist.length,totalPrice:totalPri}
},
我们遍历商品中选中的商品进行计算总数量和价格。
6.删除所选产品。过滤掉集合选中的元素即可
vue代码:
deleteProduct:function () {
this.productList=this.productList.filter(function (item) {return !item.isSelect})
},
7.全选功能。首先我们要在computed里面写一个检测是否全选的方法
isSelectAll:function(){
// console.log(this.productList.every(function (val) { return val.isSelect}))
//如果productList中每一条数据的isSelect都为true,返回true,否则返回false;
return this.productList.every(function (val) { return val.isSelect});
},
然后在写好选择商品的方法,其实全选功能就是为所有商品都设置选中或者反选。所以我们只需要为选择商品的方法传一个true或者false即可
selectProduct:function(_isSelect){
//遍历productList,全部取反
for (var i = 0, len = this.productList.length; i < len; i++) {
this.productList[i].isSelect = !_isSelect;
}
},
我们这里的选择框都是用input的checkbox,所以我们需要绑定checked是否选中。用v-bind:checked来设置checkbox是否选中。
最终页面代码:
第一步先要引入
<link href="/static/lib/layui/css/layui.css" type="text/css" rel="stylesheet">
<script src="https://cdn.staticfile.org/vue/2.4.2/vue.min.js"></script>
<script src="https://cdn.staticfile.org/axios/0.18.0/axios.min.js"></script>
<script src="https://cdn.staticfile.org/vue-resource/1.5.1/vue-resource.min.js"></script>
<!-- 引入样式 -->
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
<!-- 引入组件库 -->
<script src="https://unpkg.com/element-ui/lib/index.js"></script>
第二步
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<link href="/static/lib/layui/css/layui.css" type="text/css" rel="stylesheet">
<script src="https://cdn.staticfile.org/vue/2.4.2/vue.min.js"></script>
<script src="https://cdn.staticfile.org/axios/0.18.0/axios.min.js"></script>
<script src="https://cdn.staticfile.org/vue-resource/1.5.1/vue-resource.min.js"></script>
<!-- 引入样式 -->
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
<!-- 引入组件库 -->
<script src="https://unpkg.com/element-ui/lib/index.js"></script>
</head>
<style type="text/css">
table{width: 1200px;}
table th{width: 100px}
table td{width: 200px;text-align: center;}
a{text-decoration:none;color: black}
span{font-size: 20px;margin: 10px 10px}
strong{border: 1px solid;}
.checkPro{
background-color: gray;
}
.leftConent {
float: left;
}
.rightConent{
float: right;
}
</style>
<body>
<div id="test">
<table id="mytable">
<tr>
<th><input type="checkBox" @click="selectProduct(isSelectAll)" v-bind:checked="isSelectAll" />全选</th>
<th>商品</th>
<th>数量</th>
<th>单价(元)</th>
<th>金额(元)</th>
<th>操作</th>
</tr>
<tr v-for="(item,index) in productList">
<td><input type="checkBox" v-bind:checked="item.isSelect" @click="item.isSelect=!item.isSelect"/></td>
<td>{{item.name}}</td>
<td><span>
<a href="#" @click="item.number>0?item.number--:''">-</a>
</span><strong>{{item.number}}</strong><span>
<a href="#" @click="item.number++">+</a>
</span></td>
<td>{{item.price}}</td>
<td>{{item.price*item.number}}</td>
<td><a href="#" @click="deletePro(index)">删除</a></td>
</tr>
</table>
<div class="checkPro">
<div class="leftConent">
<span><a href="#" @click="deleteProduct">删除所选产品</a></span>
</div>
<div class="rightConent">
<span>{{getTotal.totalNum}}件商品总计:¥{{getTotal.totalPrice}}</span>
</div>
</div>
</div>
</body>
<script type = "text/javascript">
new Vue({
el : "#test",
data : {
productList:[],
},
created() { // 当 vm 实例 的 data 和 methods 初始化完毕后,vm实例会自动执行created 这个生命周期函数
this.getAllList();
},
methods:{
getAllList() {
// 由于已经导入了 Vue-resource这个包,所以 ,可以直接通过 this.$http 来发起数据请求
this.$http.get("{:url('admin/shopping/create')}").then(result => {
// 注意: 通过 $http 获取到的数据,都在 result.body 中放着
// console.log(result.data)
var result = result.body
if (result.code === 0) {
// 成功了
this.productList = result.data
console.log(result.data)
} else {
// 失败了
alert('获取数据失败!')
}
})
},
selectProduct:function(_isSelect){
//遍历productList,全部取反
for (var i = 0, len = this.productList.length; i < len; i++) {
this.productList[i].isSelect = !_isSelect;
}
},
//删除功能
deletePro : function(index){
// console.log(this.productList[index]);
alert("你正在删除"+this.productList[index].name);
this.productList.splice(index,1);
},
//删除已经选中(isSelect=true)的产品
deleteProduct:function () {
this.productList=this.productList.filter(function (item) {return !item.isSelect})
},
},
computed:{
//检测是否全选
isSelectAll:function(){
// console.log(this.productList.every(function (val) { return val.isSelect}))
//如果productList中每一条数据的isSelect都为true,返回true,否则返回false;
return this.productList.every(function (val) { return val.isSelect});
},
//总商品数量和总价格
getTotal:function(){
var prolist = this.productList.filter(function (val) { return val.isSelect}),
totalPri = 0;
for (var i = 0,len = prolist.length; i < len; i++) {
totalPri+=prolist[i].price*prolist[i].number;
}
return {totalNum:prolist.length,totalPrice:totalPri}
},
},
//设置选中功能
mounted:function () {
var _this=this;
//为productList添加select(是否选中)字段,初始值为true
this.productList.map(function (item) {
_this.$set(item, 'select', true);
})
}
})
</script>
</html>
|