1.JavaScript的概述
JavaScript是web上?种功能强?的编程语?,?于开发交互式的web??。它不需要进?编译,?是直接嵌?在HTML??中,由浏览器执?。
- JavaScript被设计?来向HTML??添加交互?为。
- JavaScript是?种脚本语?(脚本语?是?种轻量级的编程语?)。
- JavaScript由数?可执?计算机代码组成。
- JavaScript通常被直接嵌?HTML??。
- JavaScript是?种解释性语?(就是说,代码执?不进?预编译)。
JavaScript的组成:
- 核?(ECMAScript)
- ?档对象模型(DOM)
- 浏览器对象模型(BOM)
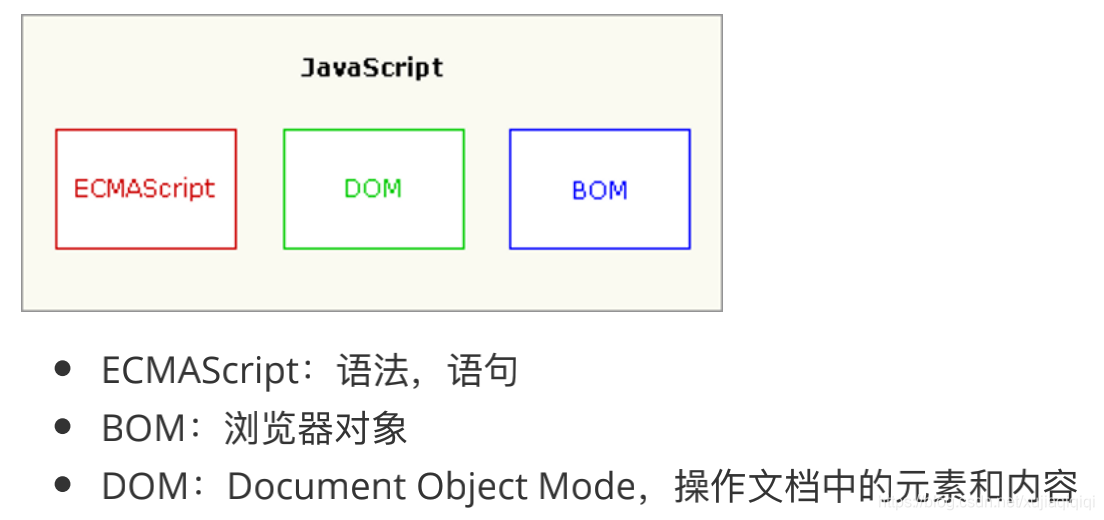
JavaScript的作?: 使?JavaScript添加??动画效果,提供?户操作体验。主要应?有:嵌?动态?本于HTML??、对浏览器事件做出相应、读写HTML元素、验证提交数据、检测访客的浏览器信息等。
JavaScript的引?: 在HTML?件中引?JavaScript有两种?式,?种是在HTML?档直接嵌?JavaScript脚本,称为内嵌式,另?种是链接外部JavaScript脚本?件,称为外联式。对他们的具体讲解如下:
<script type="text/javascript">
</script>
<script src="1.js" type="text/javascript" charset="UTF-8"></script>
2.基本语法
变量 在使?JavaScript时,需要遵循以下命名规范:
- 必须以字?或下划线开头,中间可以是数字、字符或下划线
- 变量名不能包含空格等符号
- 不能使?JavaScript关键字作为变量名,如:function
- JavaScript严格区分??写
- 变量的声明
var 变量名;
var 变量名 = 值;
数据类型 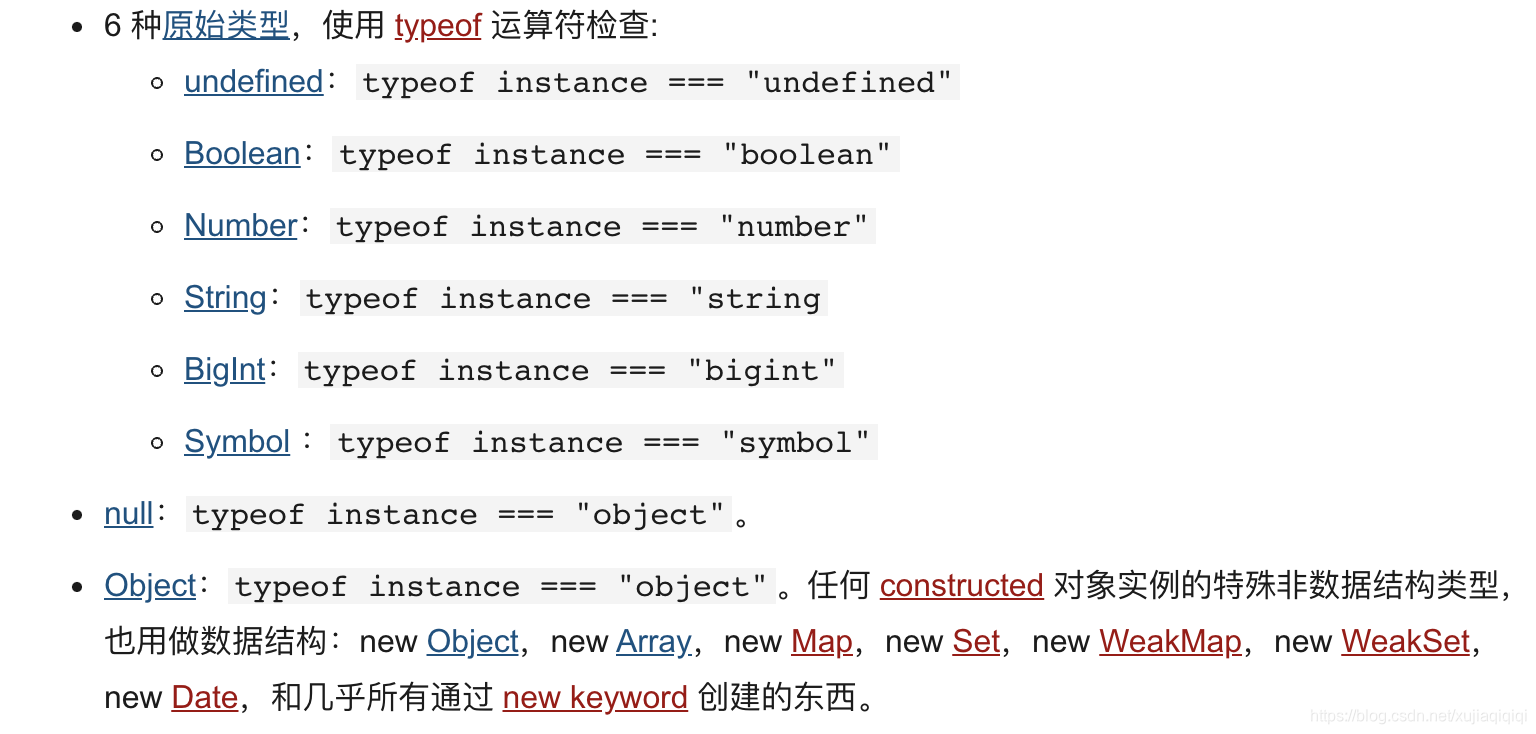 运算符
3.基础操作(案例)
案例1:完成注册??的校验
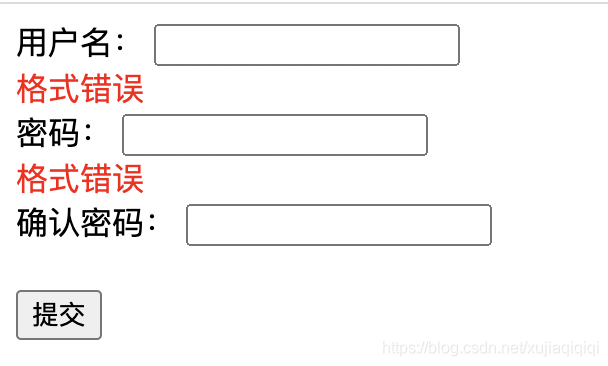
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script>
window.onload = function(){
usernameInput = document.getElementById("username");
passwordInput = document.getElementById("password");
password_repeInput = document.getElementById("password_repe");
usernameInput.onblur = checkUsername;
passwordInput.onblur = checkPassword;
password_repeInput.onblur = checkPassword_repeInput;
}
function checkUsername(){
var reg = /^[a-zA-Z]\w{5,17}$/;
var username = usernameInput.value;
var spanMsg1 = document.getElementById("msg1");
if(!reg.test(username)){
spanMsg1.innerHTML = "<font color='red'>格式错误</font>";
return false;
}else{
spanMsg1.innerHTML = '';
return true;
}
}
function checkPassword(){
var reg = /^\w{6,15}$/;
var password = passwordInput.value;
var spanMsg2 = document.getElementById("msg2");
if(!reg.test(password)){
spanMsg2.innerHTML = "<font color='red'>格式错误</font>";
return false;
}else{
spanMsg2.innerHTML = '';
return true;
}
}
function checkPassword_repeInput(){
var password_repe = password_repeInput.value;
var spanMsg3 = document.getElementById("msg3");
if(!password_repe.match(passwordInput.value)){
spanMsg3.innerHTML = "<font color='red'>格式错误</font>";
return false;
}else{
spanMsg3.innerHTML = '';
return true;
}
}
function check(){
return checkUsername() && checkPassword() && checkPassword_repeInput()
}
</script>
</head>
<body>
<form action="#" onsubmit="return check()">
用户名:
<input type="text" name="username" id="username" >
<br>
<span id="msg1"></span><br>
密码:
<input type="password" name="password" id="password" />
<br>
<span id="msg2"></span><br>
确认密码:
<input type="password" name="password_repe" id="password_repe" />
<br>
<span id="msg3"></span><br>
<input type="submit">
</form>
</body>
</html>
案例2:轮播图
定时器 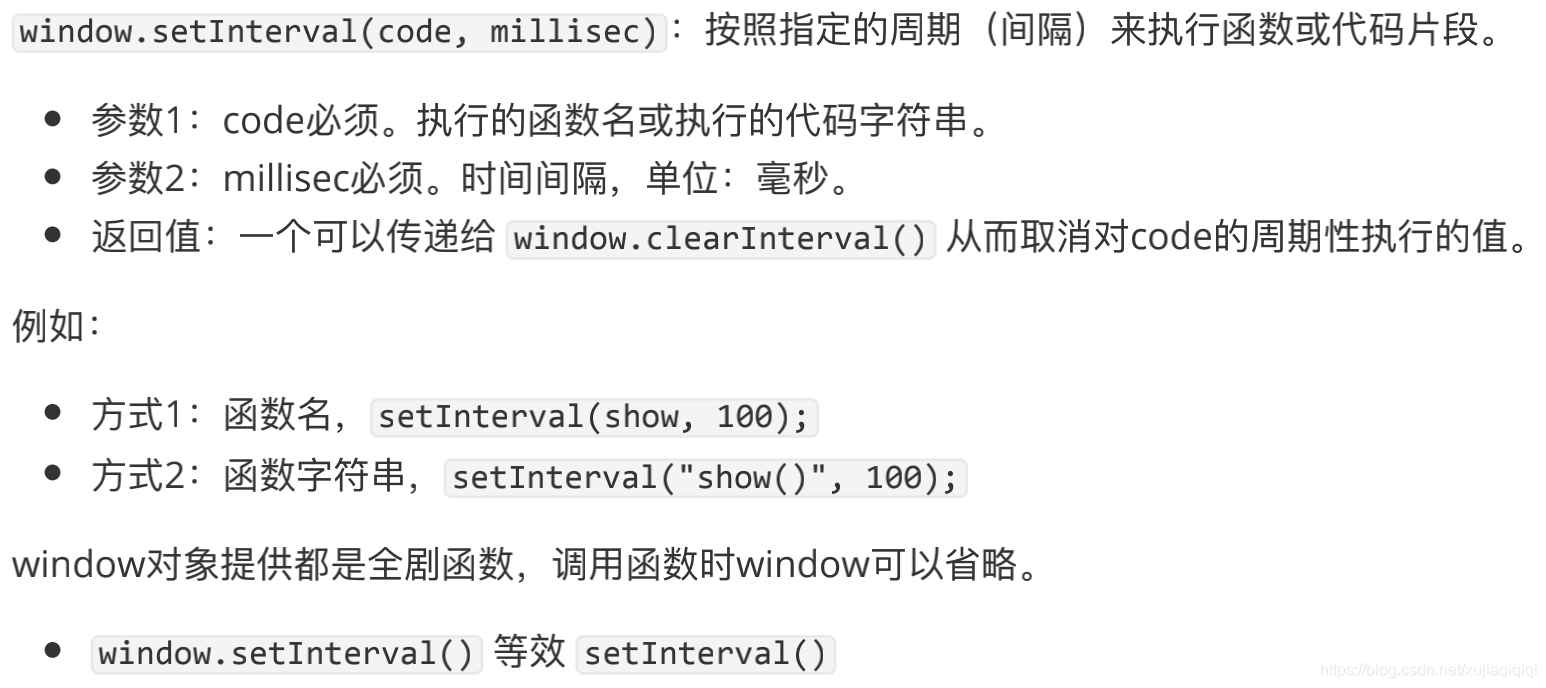
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>轮播</title>
<script>
window.onload = function(){
startLunbo();
}
var imgarr = ["../image/products/c_0001.jpg","../image/products/c_0002.jpg","../image/products/c_0003.jpg"];
var i =0;
function lunbo(){
var img = document.getElementById("img");
img.src = imgarr[i++%3];
}
function startLunbo(){
intervalId=setInterval("lunbo()",1000);
}
function stopLunbo(){
clearInterval(intervalId);
}
</script>
</head>
<body>
<img src="../image/products/c_0001.jpg" alt="" id ="img" onmouseout="startLunbo()" onmouseover="stopLunbo()">
</body>
</html>
案例3:定时弹?告
setTimeout 使用方法同上 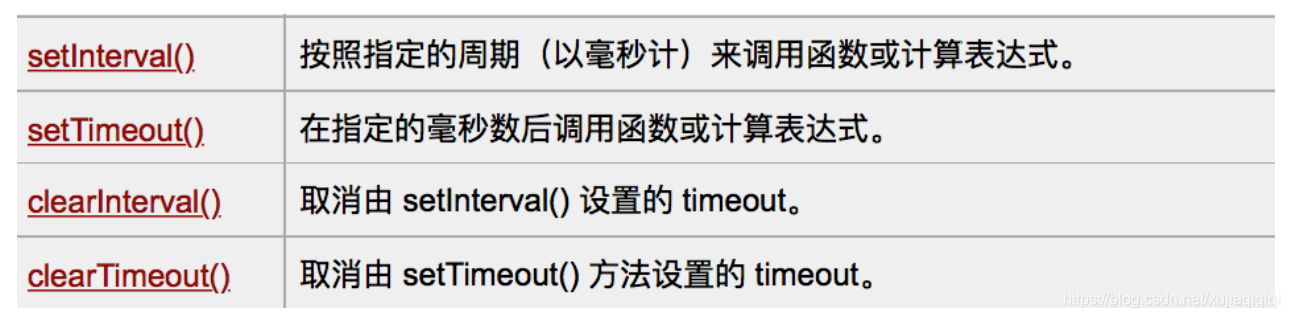
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script>
onload = function(){
setTimeout("showAd()",3000);
}
function showAd(){
var ad =document.getElementById("img");
ad.style.display = "block";
setTimeout("hideAd()",3000);
}
function hideAd(){
var ad =document.getElementById("img");
ad.style.display = "none";
}
</script>
</head>
<body>
<img src="../image/image/2.jpg" alt="" id="img" style="display: none;" width="100%">
</body>
</html>
4.进阶操作(案例)
案例1:表格隔?换?以及鼠标高亮
- 在函数内部this表示:当前操作的元素。
- this.setAttribute(name, value):给当前元素设置属性
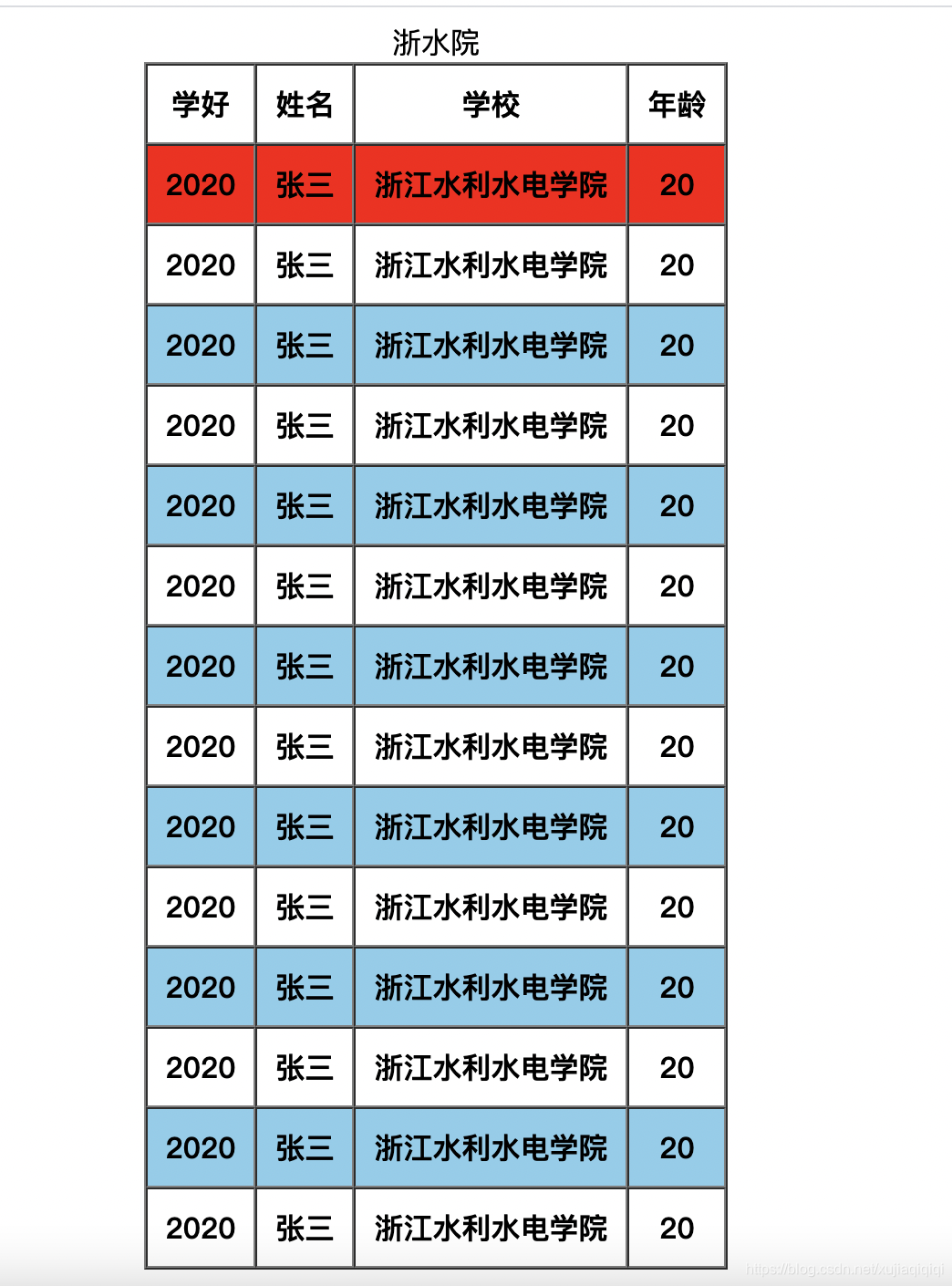
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>表格隔?换?以及鼠标高亮</title>
<script type="text/javascript">
onload = function(){
var tab = document.getElementById("table");
var tBodies = tab.tBodies;
index=0;
for(var i = 0;i<tBodies.length;i++){
var ros = tBodies[i].rows;
for(var j = 0;j<ros.length;j++){
if(index++ %2==0){
ros[j].style.backgroundColor = "skyblue";
}
ros[j].onmouseover = function(){
this.setAttribute("color",this.style.backgroundColor)
this.style.backgroundColor = "red";
}
ros[j].onmouseout = function(){
this.style.backgroundColor = this.getAttribute("color");
}
}
}
}
</script>
</head>
<body>
<table border="1" cellspacing="0" cellpadding="10" align="center" id = "table">
<caption>浙水院</caption>
<thead>
<tr class="cls1">
<th>学好</th>
<th>姓名</th>
<th>学校</th>
<th>年龄</th>
</tr>
</thead>
<tbody>
<tr class="cls1">
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
</tbody>
<tbody>
<tr>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
</tbody>
</table>
</body>
</html>
案例2:复选框全选/全不选
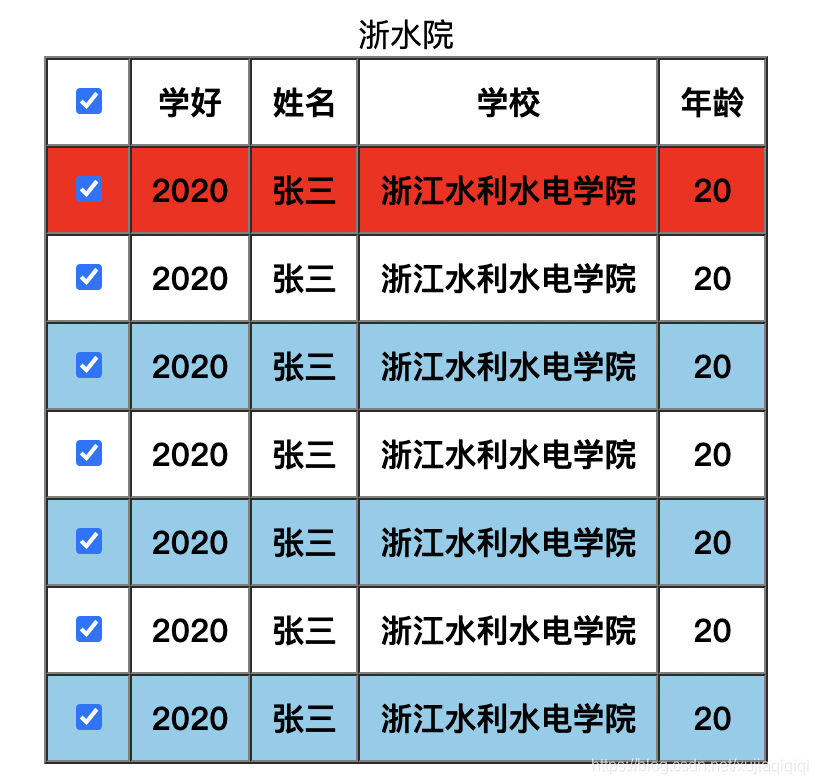
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>各行换色</title>
<script type="text/javascript">
onload = function(){
var tab = document.getElementById("table");
var tBodies = tab.tBodies;
index=0;
for(var i = 0;i<tBodies.length;i++){
var ros = tBodies[i].rows;
for(var j = 0;j<ros.length;j++){
if(index++ %2==0){
ros[j].style.backgroundColor = "skyblue";
}
ros[j].onmouseover = function(){
this.setAttribute("color",this.style.backgroundColor)
this.style.backgroundColor = "red";
}
ros[j].onmouseout = function(){
this.style.backgroundColor = this.getAttribute("color");
}
}
}
document.getElementById("AllCheck").onclick = function(){
var inputs = document.getElementsByTagName("input");
for(var i = 0;i<inputs.length ;i++){
inputs[i].checked = this.checked;
}
}
}
</script>
</head>
<body>
<table border="1" cellspacing="0" cellpadding="10" align="center" id = "table">
<caption>浙水院</caption>
<thead>
<tr class="cls1">
<th><input type="checkbox" name="" id="AllCheck" value="" /></th>
<th>学好</th>
<th>姓名</th>
<th>学校</th>
<th>年龄</th>
</tr>
</thead>
<tbody>
<tr class="cls1">
<th><input type="checkbox" name="" id="" value="" /></th>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th><input type="checkbox" name="" id="" value="" /></th>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th><input type="checkbox" name="" id="" value="" /></th>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th><input type="checkbox" name="" id="" value="" /></th>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th><input type="checkbox" name="" id="" value="" /></th>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th><input type="checkbox" name="" id="" value="" /></th>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
<tr class="cls1">
<th><input type="checkbox" name="" id="" value="" /></th>
<th>2020</th>
<th>张三</th>
<th>浙江水利水电学院</th>
<th>20</th>
</tr>
</tbody>
</table>
</body>
</html>
案例3:省市?级联动
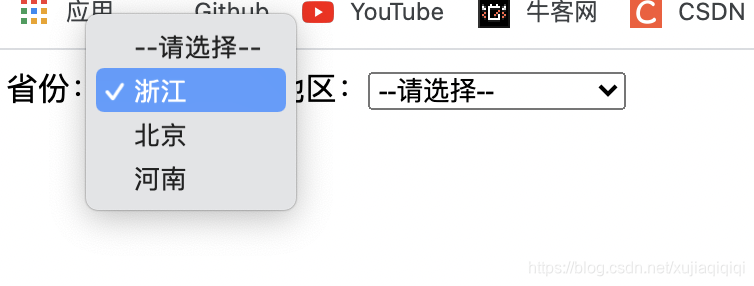 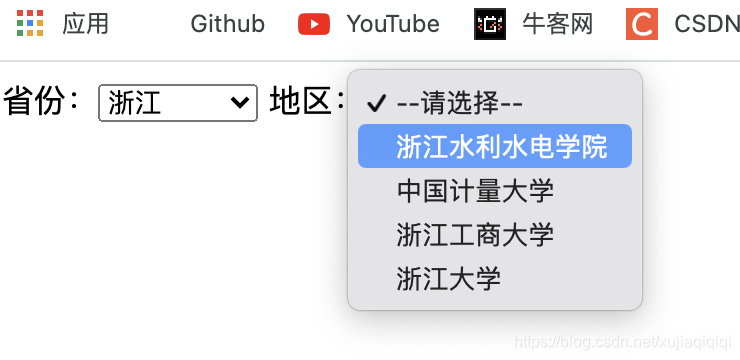
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>二级省份选择</title>
<script>
onload = function (){
}
cities = [
["浙江水利水电学院","中国计量大学","浙江工商大学","浙江大学"],
["北京大学","清华大学"],
["中国科技大学"]
];
function changeCity(obj){
var citySelect = document.getElementById("city");
var citiys = cities[obj.value];
citySelect.innerHTML="<option >--请选择--</option>"
if(citiys){
for(var i = 0 ; i < citiys.length;i++){
var cityOption = document.createElement("option");
cityOption.innerHTML = citiys[i];
citySelect.appendChild(cityOption)
}
}
}
</script>
</head>
<body>
省份:<select onchange="changeCity(this)">
<option>--请选择--</option>
<option value="0">浙江</option>
<option value="1">北京</option>
<option value="2">河南</option>
</select>
地区:<select name="" id="city">
<option value="">--请选择--</option>
</select>
</body>
</html>
|