Vue 基础(持续更新…)
el:挂载点
1、el的作用:设置Vue实例挂载/管理的元素 2、Vue会管理el命中的元素以及内部的后代元素 3、可以使用其他选择器,建议使用id选择器 4、可以使用其他的双标签,不能使用和
data:数据对象
Vue中用到的对象定义在data 中,data 中可以写简单类型、数组、复杂对象等
methods:方法对象
本地应用
v-text 指令 :
设置标签的内容,可以使用 {{ xx }}
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id="app">
{{ message + "拼接字符串1"}}<br/>
{{ message }}拼接字符串2
<br/>
<span>
{{ student.wechat}} : {{ student.name }} : {{ student.age }}
</span>
<ul>
<li>{{ address[0] }}</li>
<li>{{ address[1] }}</li>
</ul>
<h2 v-text="message + '拼接字符串3'">
123(无效、不显示123)
</h2>
</div>
<script>
var app = new Vue({
el:"#app",
data:{
message:"hello,姚",
student:{
name:"yaoyu",
age:18,
wechat:"microddyy"
},
address:["shanghai","北京"]
}
})
</script>
</body>
</html>
v-html 指令 :
用于设置元素的innerHTML 解析纯文本用v-text ,需要解析html时使用v-html
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id='app'>
<p v-html="content"></p>
<p v-text="content"></p>
</div>
<script>
var app = new Vue({
el:'#app',
data:{
content:"<a href='http://www.baidu.com'>点我</a>"
}
})
</script>
</body>
</html>
v-on 指令 :
为元素绑定事件
注意: 1、事件名不加on 2、v-on 可以简写为:@ 3、绑定的方法写在methods属性中 4、方法内通过this 关键字可以访问data中的数据
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id='app'>
<input type="button" value="单机事件绑定1" v-on:click="doSome"/>
<input type="button" value="双击事件绑定2" v-on:dblclick="doSome"/>
<input type="button" value="单机事件绑定3" @click="doSome"/>
<h2 type="button" @click="change">{{ food }}</h2>
</div>
<script>
var app = new Vue({
el:'#app',
methods:{
doSome:function(){
alert(123);
},
change:function(){
this.food += "potato";
}
},
data:{
food:"西红柿"
}
})
</script>
</body>
</html>
小例子:计数器
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id="app">
<div class="input_nunm">
<button @click="down">
-
</button>
<span>{{ num }}</span>
<button @click="up">
+
</button>
</div>
</div>
<script>
var app = new Vue({
el:"#app",
data:{
num:1
},
methods:{
down:function(){
if (this.num > 0){
this.num--;
} else{
alert("不能减小了!");
}
},
up:function(){
if (this.num < 5){
this.num++;
} else{
alert("不能增大了!");
}
}
}
})
</script>
</body>
</html>
结果:
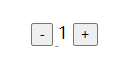
点击 + 计数加一,点击 - 计数减一。减到0弹窗提示不能再减了,加到5弹窗提示不能再加了。
v-show 指令:
根据表达式的真假,切换元素的显示和隐藏。本质是通过修改元素的 display 属性来实现显示或者隐藏
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id="app">
<img src="http://www.itheima.com/images/logo.png" alt="图片加载失败提示文字" title="鼠标悬停图片上提示文件" v-show="true"/>
<img src="http://www.itheima.com/images/logo.png" alt="图片加载失败提示文字" title="鼠标悬停图片上提示文件" v-show="isShow"/>
<img src="http://www.itheima.com/images/logo.png" alt="图片加载失败提示文字" title="鼠标悬停图片上提示文件" v-show="age>18"/>
</div>
<script>
var app = new Vue({
el:"#app",
data:{
isShow:false,
age:19
}
})
</script>
</body>
</html>
v-if 指令:
根据表达式的真假,切换元素的显示与隐藏(操作的是dom元素,表达式为false就直接移除标签,表达式为true时在dom树中添加标签。v-show修饰的元素一直都在html中,只是修改了元素的display属性)
频繁变换的数据使用 v-show,否则使用 v-if(操作dom元素更消耗性能)
<img src="http://www.itheima.com/images/logo.png" alt="图片加载失败提示文字" title="鼠标悬停图片上提示文件" v-if="true"/>
<img src="http://www.itheima.com/images/logo.png" alt="图片加载失败提示文字" title="鼠标悬停图片上提示文件" v-if="isShow"/>
<img src="http://www.itheima.com/images/logo.png" alt="图片加载失败提示文字" title="鼠标悬停图片上提示文件" v-if="age>18"/>
v-bind 指令:
设置元素的属性,如:class 、src 、title 等
注意:v-bind可以省略;设置class时建议使用对象的方式
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
<style>
.active{
border: 1px solid red;
}
</style>
</head>
<body>
<div id="app">
<img v-bind:src="imgSrc" />
<img :src="imgSrc" :title="imgSrc+'123'"/>
<input type="button" value="change" @click="toggleActive"/>
<img :src="imgSrc" :class="isActive?'active':''" />
<img :src="imgSrc" :class="{active:isActive}" />
</div>
<script>
var app = new Vue({
el:"#app",
data:{
imgSrc:"http://www.itheima.com/images/logo.png",
isActive:false
},
methods:{
toggleActive:function(){
this.isActive = !this.isActive;
}
}
})
</script>
</body>
</html>
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-PVWpnedy-1628002748861)(C:\Users\yao\AppData\Roaming\Typora\typora-user-images\image-20210802155313769.png)]](https://img-blog.csdnimg.cn/ac99857a30e642c9a5abe22e33be00fd.png)
小例子:图片切换
分析:将图片保存到数组中 → 为每个图片设置一个索引 → 绑定src属性 → 写切换图片的逻辑 → 显示状态切换
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
<link rel="stylesheet" href="./css/index.css" />
</head>
<body>
<div id="mask">
<div class="center">
<h2 class="title">
<img src="./images/logo.png" alt=""> xxxx校区环境
</h2>
<img :src="imageAddress[index]"/ alt="">
<a href="javascript:void(0)" class="left">
<img src="./images/prev.png" alt="" @click="prev"/ v-show="index!=0">
</a>
<a href="javascript:void(0)" class="right">
<img src="./images/next.png" alt="" @click="next" v-show="index<imageAddress.length-1"/>
</a>
</div>
</div>
<script>
var app = new Vue({
el:"#mask",
data:{
imageAddress:[
"./images/00.jpg",
"./images/01.jpg",
"./images/02.jpg",
"./images/03.jpg",
"./images/04.jpg",
"./images/05.jpg",
"./images/06.jpg",
"./images/07.jpg",
"./images/08.jpg",
"./images/09.jpg",
"./images/10.jpg",
],
index:0
},
methods:{
prev:function(){
this.index--;
},
next:function(){
this.index++;
}
}
})
</script>
</body>
</html>
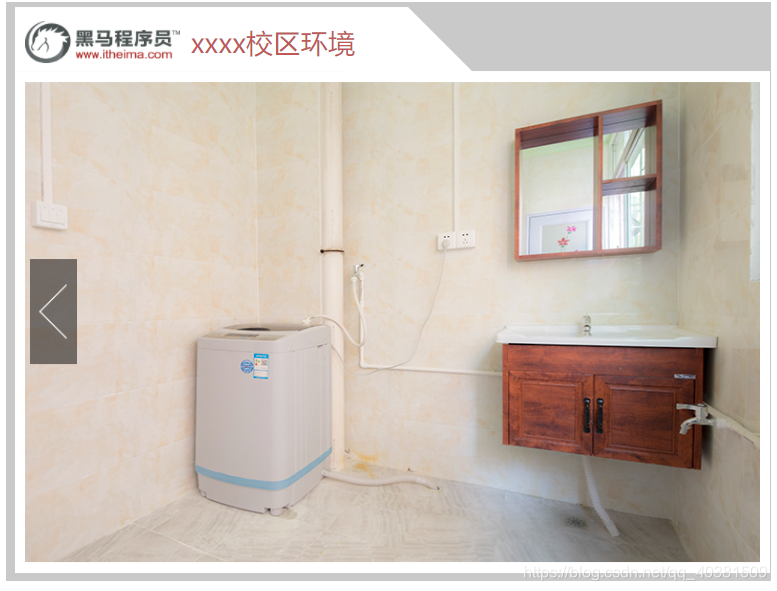
v-for 指令:
遍历数组、对象等数据,动态【展示、删除、添加】页面元素。
<!DOCTYPE html>
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id="app">
<ul>
<li v-for="(item,index) in arr" v-bind:title="item">
{{ index+1 }}:生成的列表:{{ item }}
</li>
</ul>
<h2 v-for="it in objArr">{{ it }}</h2>
<h2 v-for="it in objArr">{{ it.name }}</h2>
<input type="button" value="添加" @click="add" />
<input type="button" value="删除" @click="remove" />
</div>
</div>
<script>
var app = new Vue({
el: "#app",
data: {
arr: ["bj", "sh", "gz", "sz"],
objArr: [
{name: "zs"},
{name: "ls"},
]
},
methods:{
add:function(){
this.objArr.push({name:"ww"});
this.arr.push("qq");
},
remove:function(){
this.objArr.shift();
}
}
})
</script>
</body>
</html>
v-on 指令补充:
1、传递自定义参数
2、事件修饰符
<!DOCTYPE html>
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id="app">
<input type="button" value="click" @click="getNum(2)"/>
<input type="text" v-on:keyup.enter="doSome" />
</div>
</div>
<script>
var app = new Vue({
el: "#app",
methods:{
getNum:function(num){
console.log(num);
},
doSome:function(){
alert("!!!");
}
}
})
</script>
</body>
</html>
v-model 指令:
获取用户的输入。 获取和设置表单元素的值(也叫:双向数据绑定),绑定的数据 和 表单元素的值 相关联
<!DOCTYPE html>
<html>
<head>
<title>vue test</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id="app">
<input type="button" value="click" @click="changeM"/>
<input type="text" v-model="message" v-on:keyup.enter="doSome" />
<h2>{{ message }}</h2>
</div>
</div>
<script>
var app = new Vue({
el: "#app",
data:{
message:"xxx"
},
methods:{
doSome:function(){
alert("!!!");
},
changeM:function(){
this.message="yyy";
}
}
})
</script>
</body>
</html>
小例子:记事本
功能:
? 1新增: ? 生成列表结构:v-for ? 获取用户输入:v-model ? 回车,新增数据:v-on.enter
? 2删除: ? 点击 × 删除指定记录
? 3统计: ? 自动更改数据条数
? 4清空: ? 清除所有数据
? 5隐藏: ? 没有数据时,隐藏底部:v-show / v-if
<!DOCTYPE html>
<html>
<head>
<title>notebook</title>
<link rel="stylesheet" type="text/css" href="./css/index2.css" />
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<section id="todoapp">
<header class="header">
<h1>小黑记事本</h1>
<input autofocus="autofocus" autocomplete="off" placeholder="请输入任务" class="new-todo" v-model="inputValue" v-on:keyup.enter="generateNote"/>
</header>
<section class="main">
<ul class="todo-list">
<li class="todo" v-for="(item,index) in arr">
<div class="view" >
<span class="index">{{ index+1 }}</span>
<label>{{ item }}</label>
<button class="destroy" @click="deleteEle(index)"></button>
</div>
</li>
</ul>
</section>
<footer class="footer" >
<span class="todo-count" v-show="arr.length!=0">
<strong>{{ arr.length }}</strong> items left
</span>
<button class="clear-completed" @click="clearEle" v-if="arr.length!=0">
Clear
</button>
</footer>
</section>
<footer class="info"></footer>
<script>
var app = new Vue({
el:"#todoapp",
data:{
arr:["吃饭", "睡觉", "学习"],
inputValue:"xxxxx"
},
methods:{
generateNote:function(){
this.arr.push(this.inputValue);
},
deleteEle:function(index){
this.arr.splice(index,1);
},
clearEle:function(){
this.arr=[];
}
},
})
</script>
</body>
</html>
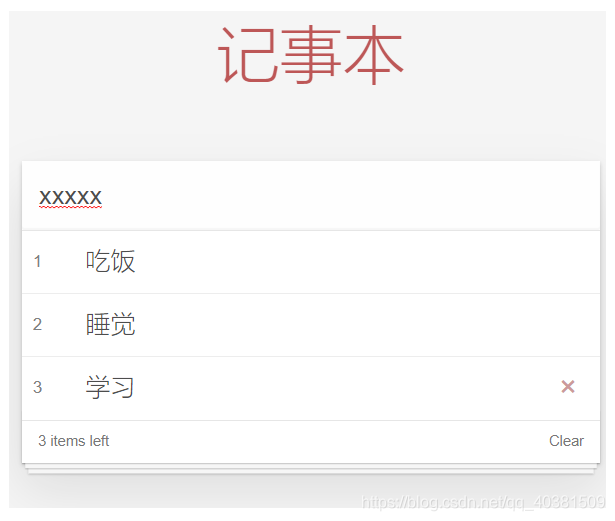
|