Vue.directive
自定义指令的五个钩子函数
下面展示一些 内联代码片 。
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Document</title>
</head>
<body>
<div id="app">
<input type="text" v-focus="msg" v-model="msg">
</div>
<script src="./vue.js"></script>
<script>
Vue.directive('focus', {
bind() {
console.log('bind')
},
inserted() {
console.log('inserted')
},
update() {
console.log('update')
},
componentUpdated() {
console.log('componentUpdated')
},
unbind() {
console.log('unbind')
}
})
const vm = new Vue({
el: '#app',
data: {
msg: 'hello vue'
}
})
</script>
</body>
</html>
钩子函数的参数
钩子函数有俩个参数 参数1: el 指令所在的元素 参数2: binding 指令相关的信息的对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Document</title>
</head>
<body>
<div id="app">
<p v-demo:aa.bb.cc.dd="msg">{{ msg }}</p>
</div>
<script src="./vue.js"></script>
<script>
Vue.directive('demo', {
bind(el, binding) {
console.log(el)
console.log(binding)
}
})
const vm = new Vue({
el: '#app',
data: {
msg: 'hello vue'
}
})
</script>
</body>
</html>
下面是对应参数 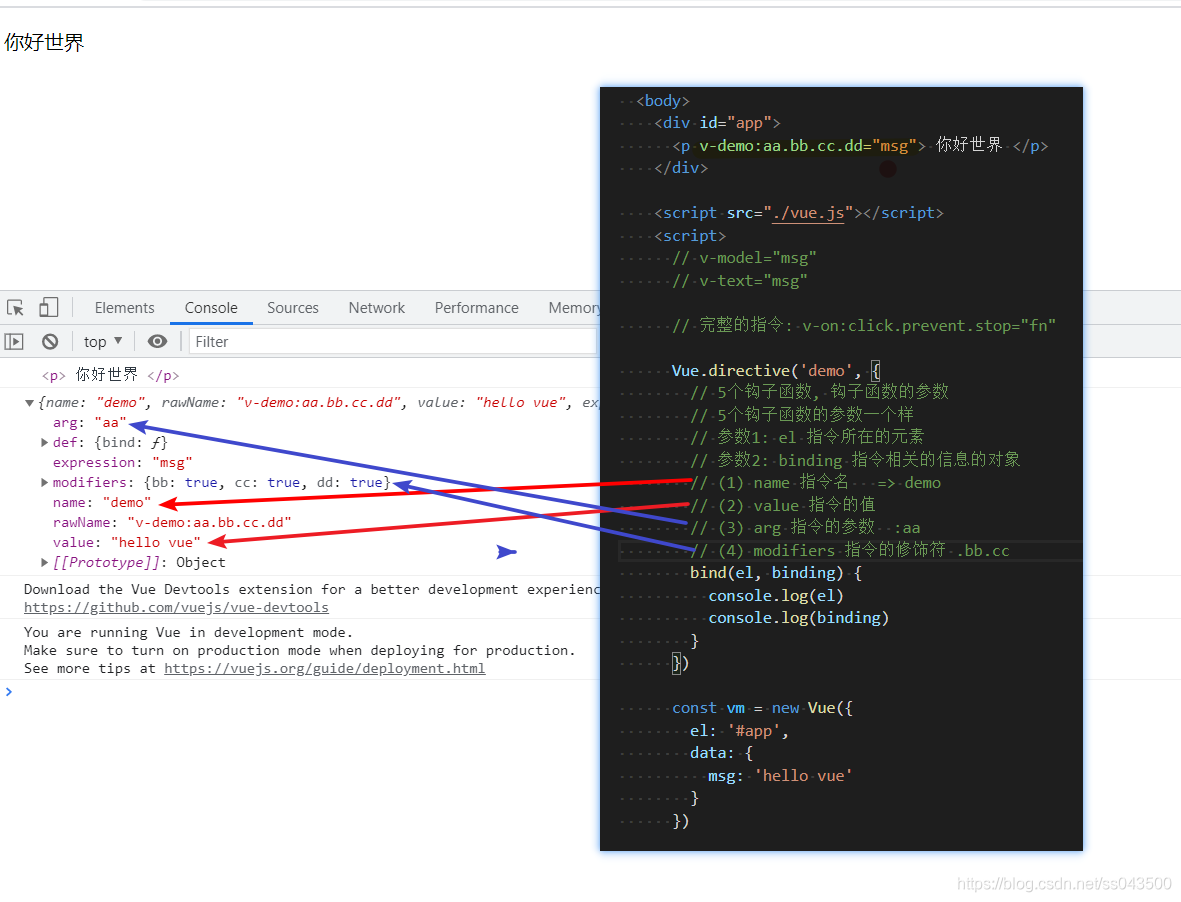 下面是自定义指令的用法
- value 的使用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Document</title>
</head>
<body>
<div id="app">
<h1 v-red>{{ msg }}</h1>
<h2 v-color="color">你好哇</h2>
<hr>
<p v-text="msg"></p>
<p v-mytext="msg"></p>
</div>
<script src="./vue.js"></script>
<script>
Vue.directive('red', {
bind(el, binding) {
el.style.color = 'red'
}
})
Vue.directive('color', {
bind(el, binding) {
console.log(binding);
el.style.color = binding.value
},
update(el, binding) {
el.style.color = binding.value
}
})
Vue.directive('mytext', {
bind(el, binding) {
el.innerText = binding.value
},
update(el, binding) {
el.innerText = binding.value
}
})
const vm = new Vue({
el: '#app',
data: {
msg: 'hello vue',
color: 'green'
}
})
</script>
</body>
</html>
- arg 的使用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Document</title>
</head>
<body>
<div id="app">
<h1 v-bind:title="msg" v-bind:str="str">{{ msg }}</h1>
<h1 v-mybind:title="msg" v-mybind:str="str">{{ msg }}</h1>
<hr>
<p v-color:bg="bgColor">我是文本, 我希望设置背景颜色</p>
<p v-color:font="fontColor">我是文本, 我希望设置字体颜色</p>
</div>
<script src="./vue.js"></script>
<script>
Vue.directive('mybind', {
bind(el, binding) {
console.log(binding.arg)
el.setAttribute(binding.arg, binding.value)
},
update(el, binding) {
el.setAttribute(binding.arg, binding.value)
}
})
Vue.directive('color', {
bind(el, binding) {
if (binding.arg === 'bg') {
el.style.backgroundColor = binding.value
}
if (binding.arg === 'font') {
el.style.color = binding.value
}
},
update(el, binding) {
if (binding.arg === 'bg') {
el.style.backgroundColor = binding.value
}
if (binding.arg === 'font') {
el.style.color = binding.value
}
}
})
const vm = new Vue({
el: '#app',
data: {
msg: 'hello vue',
str: '你好哇',
bgColor: 'pink',
fontColor: 'red'
}
})
</script>
</body>
</html>
- modifiers 的使用(修饰符)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Document</title>
</head>
<body>
<div id="app">
<p v-color:bg.bold.italic="bgColor">我是加粗的文本, 我希望设置背景颜色</p>
<p v-color:font.italic="fontColor">我是倾斜的文本, 我希望设置字体颜色</p>
</div>
<script src="./vue.js"></script>
<script>
Vue.directive('color', {
bind(el, binding) {
if (binding.arg === 'bg') {
el.style.backgroundColor = binding.value
}
if (binding.arg === 'font') {
el.style.color = binding.value
}
console.log(binding.modifiers)
if (binding.modifiers.bold) {
el.style.fontWeight = 700
}
if (binding.modifiers.italic) {
el.style.fontStyle = 'italic'
}
},
update(el, binding) {
if (binding.arg === 'bg') {
el.style.backgroundColor = binding.value
}
if (binding.arg === 'font') {
el.style.color = binding.value
}
}
})
const vm = new Vue({
el: '#app',
data: {
msg: 'hello vue',
str: '你好哇',
bgColor: 'pink',
fontColor: 'red'
}
})
</script>
</body>
</html>
自定义指令小技巧 当bind和updata 逻辑一样是可以简写
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Document</title>
</head>
<body>
<div id="app">
<h1 v-color="color">{{ msg }}</h1>
</div>
<script src="./vue.js"></script>
<script>
Vue.directive('color', (el, binding) => {
el.style.color = binding.value
})
const vm = new Vue({
el: '#app',
data: {
msg: 'hello vue',
color: 'red'
}
})
</script>
</body>
</html>
|