注意:针对类式组件(不考虑hooks)
第一属性:state
state的初始化
首先:不初始化的话就是如下: 初始化: 使用构造器进行初始化
<script type="text/babel">
class Weather extends React.Component{
constructor(props){
super(props)
this.state={isHot:false,wind:'大风'}
}
render(){
console.log(this)
return <h1>今天天气很{this.state.isHot?'炎热':'凉爽'}</h1>
}
}
ReactDOM.render(<Weather/>,document.getElementById('test'))
</script>
React中的事件绑定
复习:普通的 事件绑定 方式 三种方式: React事件绑定: 请用第三种(避免直接操作DOM)
<script type="text/babel">
class Weather extends React.Component{
constructor(props){
super(props)
this.state={isHot:false,wind:'大风'}
}
render(){
console.log(this)
return <h1 onClick={demo}>今天天气很{this.state.isHot?'炎热':'凉爽'}</h1>
}
}
ReactDOM.render(<Weather/>,document.getElementById('test'))
function demo(){
alert('按钮被点击了')
}
</script>
事件函数中this的使用问题
错误:试图在onClick回调函数中通过this找到state中的元素 结论: 需要通过实例调用才能获取到指向REACT组件实例的this
解决: 如何在onClick回调函数changeWeather中得到指向REACT组件实例的this?
从原型上获取方法,然后挂在实例自身(知识点:bind函数)
<script type="text/babel">
class Weather extends React.Component{
constructor(props){
super(props)
this.state={isHot:false,wind:'大风'}
this.changeWeather=this.changeWeather.bind(this)
}
render(){
console.log(this)
return <h1 onClick={this.changeWeather}>今天天气很{this.state.isHot?'炎热':'凉爽'}</h1>
}
changeWeather(){
console.log(this.state.isHot)
}
}
ReactDOM.render(<Weather/>,document.getElementById('test'))
</script>
运行结果: 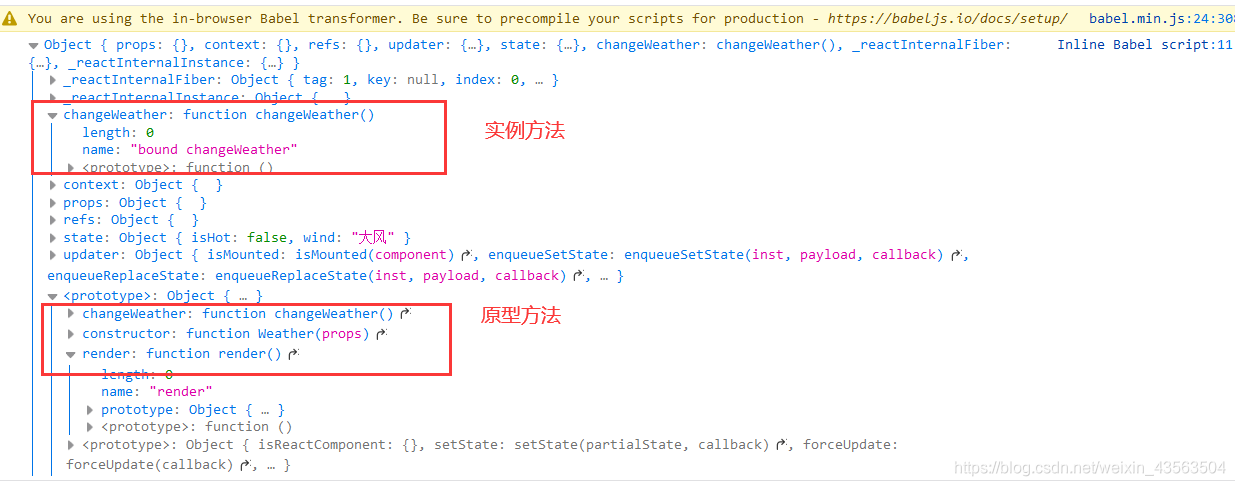
更改状态
<script type="text/babel">
class Weather extends React.Component{
constructor(props){
super(props)
this.state={isHot:false,wind:'大风'}
this.changeWeather=this.changeWeather.bind(this)
}
render(){
console.log(this)
return <h1 onClick={this.changeWeather}>今天天气很{this.state.isHot?'炎热':'凉爽'}</h1>
}
changeWeather(){
const isHot=this.state.isHot
this.setState({isHot:!isHot})
}
}
ReactDOM.render(<Weather/>,document.getElementById('test'))
</script>
简化
|