1、结果界面 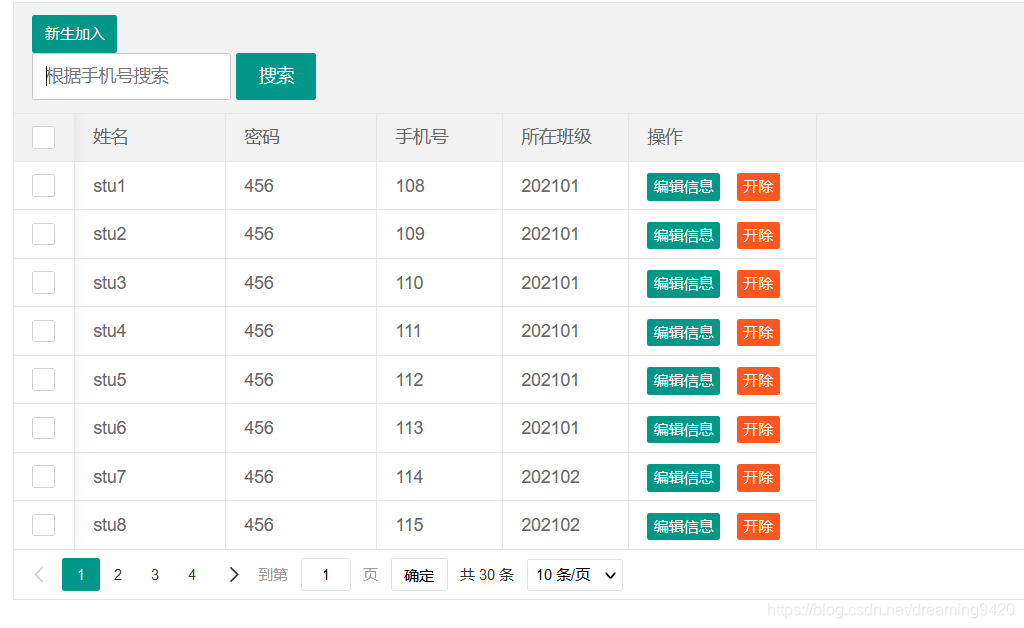 2、 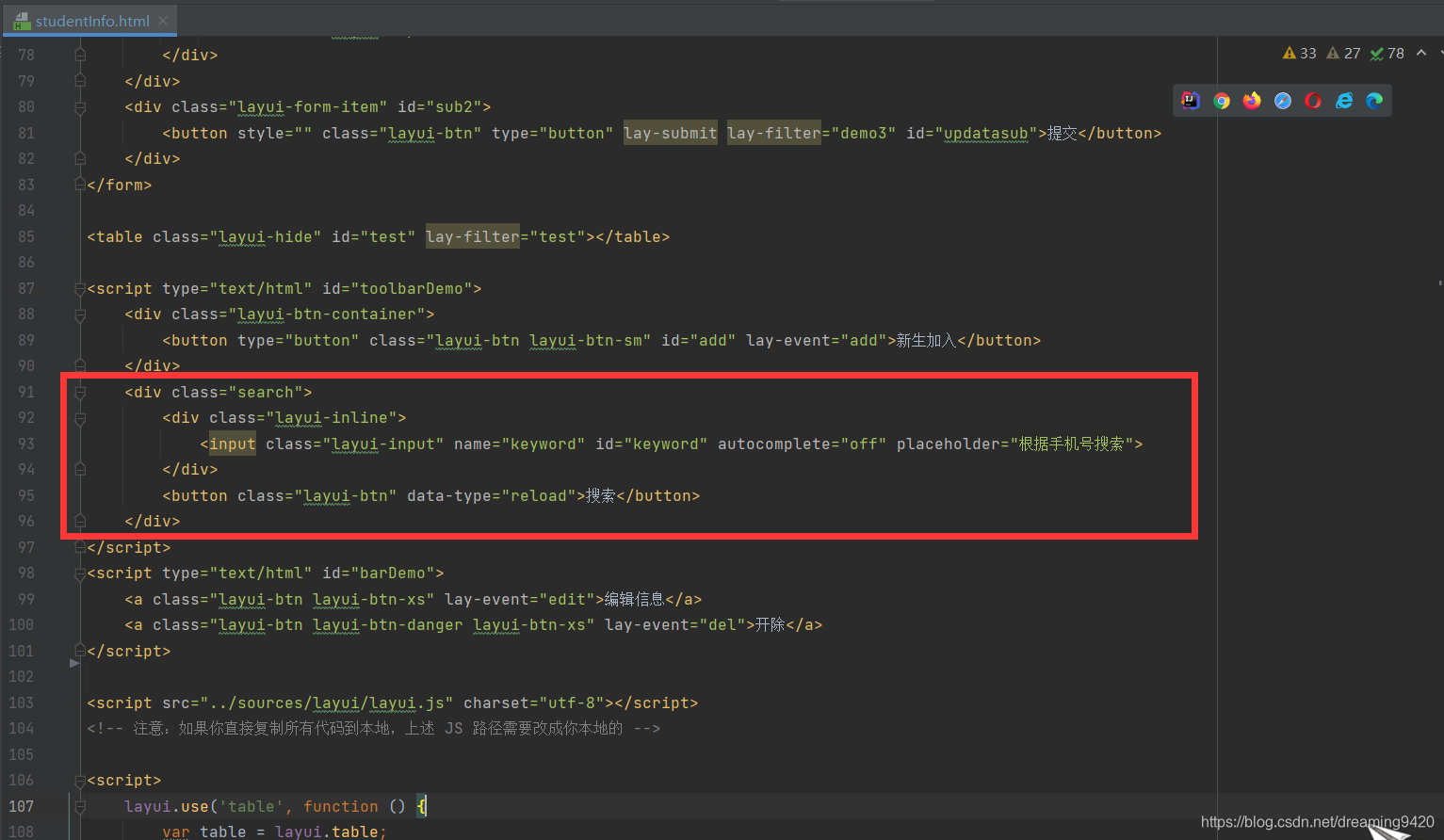
<div class="search">
<div class="layui-inline">
<input class="layui-input" name="keyword" id="keyword" autocomplete="off" placeholder="根据手机号搜索">
</div>
<button class="layui-btn" data-type="reload">搜索</button>
</div>
3、 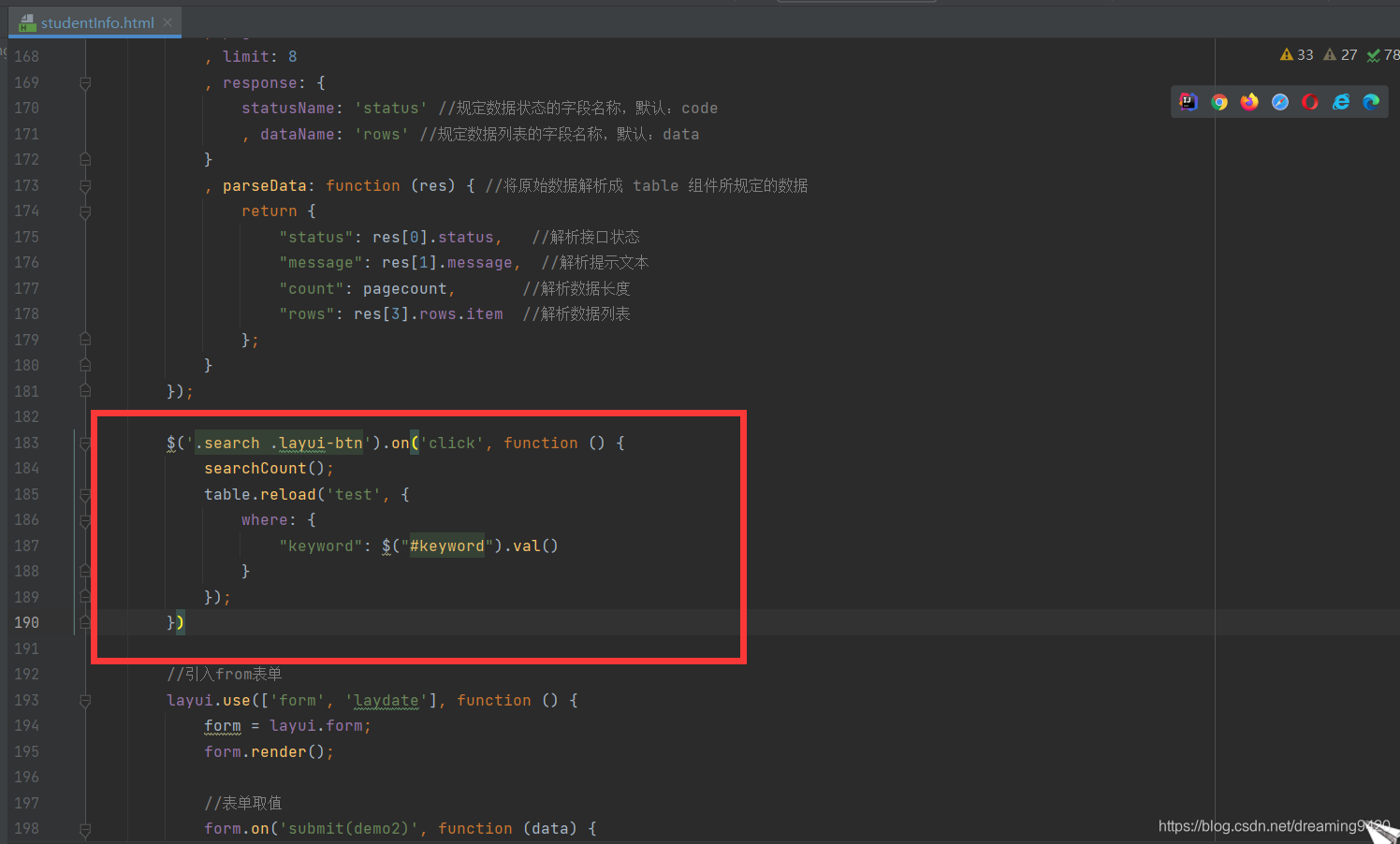
$('.search .layui-btn').on('click', function () {
searchCount();
table.reload('test', {
where: {
"keyword": $("#keyword").val()
}
});
})
4、 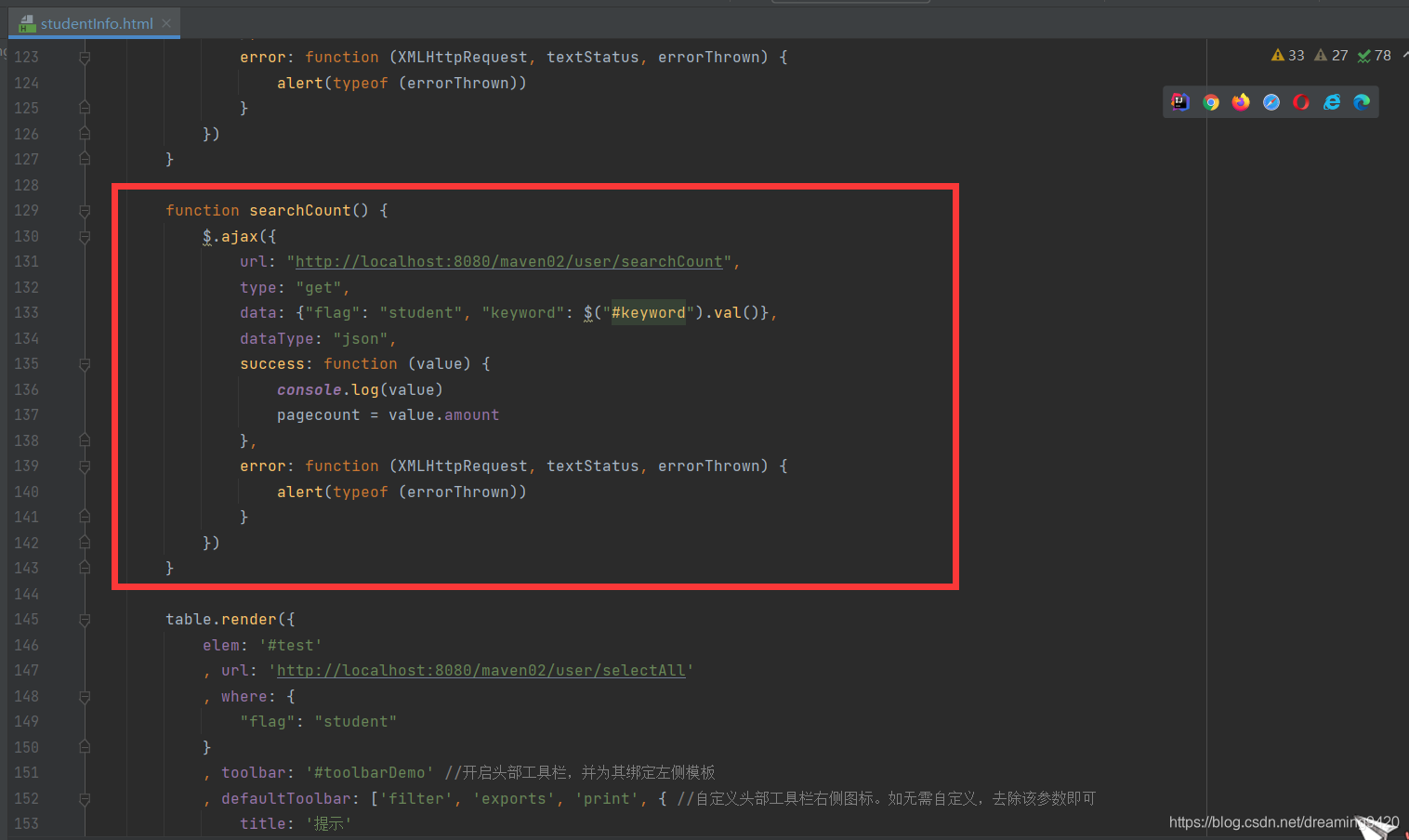
function searchCount() {
$.ajax({
url: "http://localhost:8080/maven02/user/searchCount",
type: "get",
data: {"flag": "student", "keyword": $("#keyword").val()},
dataType: "json",
success: function (value) {
console.log(value)
pagecount = value.amount
},
error: function (XMLHttpRequest, textStatus, errorThrown) {
alert(typeof (errorThrown))
}
})
}
5、mapper
<select id="searchByPhone" resultMap="BaseResultMap">
select * from user
<where>
<if test="keyword!=null and keyword!=''">
and phone like '%${keyword}%'
</if>
<if test="flag!=null and flag!=''">
and flag=
</if>
</where>
limit
</select>
<select id="searchCount" resultType="java.lang.Integer">
select count(*) from user
<where>
<if test="keyword!=null and keyword!=''">
and phone like '%${keyword}%'
</if>
<if test="flag!=null and flag!=''">
and flag=
</if>
</where>
</select>
6、dao
List<User> searchByPhone(@Param("keyword") Integer keyword,@Param("flag") String flag,
@Param("offset") int offset, @Param("limit") int limit);
Integer searchCount(@Param("keyword") Integer keyword,@Param("flag") String flag);
7、service
List<User> searchByPhone(Integer keyword, String flag, int offset, int limit);
Integer searchCount(Integer keyword, String flag);
8、serviceImpl
public List<User> searchByPhone(Integer keyword, String flag, int offset, int limit) {
return this.userDao.searchByPhone(keyword, flag, offset, limit);
}
public Integer searchCount(Integer keyword, String flag) {
return this.userDao.searchCount(keyword, flag);
}
9、controller
@ResponseBody
@RequestMapping(value = "/searchCount", method = RequestMethod.GET)
public String searchCount(HttpServletRequest request) {
String key = request.getParameter("keyword");
Integer keyword = Integer.valueOf(key);
String flag = request.getParameter("flag");
Integer countInteger = userService.searchCount(keyword, flag);
String count = String.valueOf(countInteger);
String data = "{\"amount\":" + count + "}";
return data;
}
@ResponseBody
@RequestMapping(value = "/selectAll", method = RequestMethod.GET)
public String selectAll(@RequestParam(value = "keyword", required = false) String keyword,
HttpServletRequest request) throws Exception {
String flag = request.getParameter("flag");
String offsetString = request.getParameter("page");
String limitString = request.getParameter("limit");
Integer limit = Integer.valueOf(limitString);
Integer offset = (Integer.valueOf(offsetString) - 1) * limit;
if (keyword == null) {
List<User> users = userService.queryAllByLimit(flag, offset, limit);
String[] columns = {"id", "username", "password", "phoneNumber", "classnum", "flag"};
String data = ObjtoLayJson.ListtoJson(users, columns);
return data;
} else {
Integer key = Integer.valueOf(keyword);
List<User> users = userService.searchByPhone(key, flag, offset, limit);
String[] columns = {"id", "username", "password", "phoneNumber", "classnum", "flag"};
String data = ObjtoLayJson.ListtoJson(users, columns);
return data;
}
}
|