下面的总结都将按照这个模型图去设计
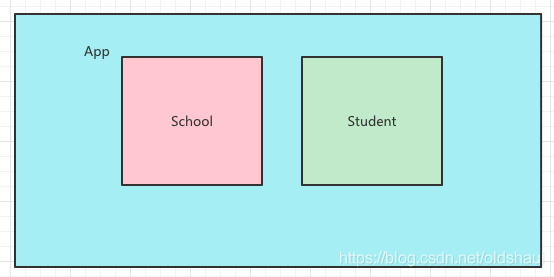
?
父传子的方式 :(Props)
App组件:
<template>
<div>
<Student name="carry" :age=26 sex="男"/>
</div>
</template>
<script>
import Student from './components/Student';
export default {
name:'App',
components: {Student},
}
</script>
<style lang='scss' scoped>
</style>
Student组件
<template>
<div>
<h1>{{ msg }}</h1>
<h2>学生姓名:{{ name }}</h2>
<h2>学生性别:{{ sex }}</h2>
<h2>学生年龄:{{ myAge + 1 }}</h2>
<button @click="updateAge">尝试修改收到的年龄</button>
</div>
</template>
<script>
export default {
data() {
return {
msg: "我是NewEgg",
myAge: this.age,
};
},
// 简单的声明接收
//props:['name','age','sex'],
//接收的同时,对数据类型进行限制
// props:{
// name:String,
// age: Number,
// sex: String
// },
//接收的同时,对数据类型限制+默认值的指定+必要性的限制
props: {
name: {
type: String, //name的类型是字符串
required: true, //name是必要的
},
age: {
type: Number,
default: 99, //默认值
},
sex: {
type: String,
required: true,
},
},
components: {},
computed: {},
methods: {
updateAge() {
this.myAge++;
},
},
};
</script>
<style lang='scss' scoped>
</style>
总结:
父组件传给子组件的值,子组件利用Props去接收(几种情况可以看代码)
子组件给父组件传值Props方式
子组件给父组件传值消息总线方式
子组件给父组件传递消息自定义事件的方式
兄弟组件传递消息总线方式
School组件代码
<template>
<div class="school">
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="sendAddrToStu">把学校的地址给学生</button>
</div>
</template>
<script>
export default {
name:'School',
data() {
return {
name:'南阳理工',
address:'南阳',
}
},
mounted() {
this.$bus.$on('hello', (data) => {
console.log('我是school组件,w我收到了Student数据', data)
})
},
methods:{
sendAddrToStu() {
this.$bus.$emit('addr', this.address)
}
}
}
</script>
<style scoped>
.school{
background-color: skyblue;
padding: 5px;
}
</style>
Student组件代码
<template>
<div class="student">
<h2>学生姓名:{{name}}</h2>
<h2>学生性别:{{sex}}</h2>
<button @click="sendStudentName">把学生名给School组件</button>
</div>
</template>
<script>
export default {
name:'Student',
data() {
return {
name:'张三',
sex:'男',
number:0
}
},
methods:{
sendStudentName() {
this.$bus.$emit('hello', this.name);
}
},
mounted() {
this.$bus.$on('addr',(data) => {
console.log('我是stu组件,我收到了我兄弟组件传来的数据:',data)
})
}
}
</script>
<style scoped>
.student{
background-color: pink;
padding: 5px;
margin-top: 30px;
}
</style>
App
<!-- -->
<template>
<div>
<h1>{{ msg }},学生姓名是:{{ studentName }}</h1>
<School/>
<Student/>
</div>
</template>
<script>
import Student from "./components/Student";
import School from "./components/School";
export default {
name: "App",
components: { Student, School },
data() {
return {
msg: "你好啊!",
studentName: "",
};
},
};
</script>
<style scoped>
</style>
main.js
// 引入vue
import Vue from 'vue'
// 引入app
import App from './App.vue'
//关闭Vue的生产提示
Vue.config.productionTip = false;
//创VM
new Vue({
el:"#app",
render: h=>h(App),
beforeCreate() {
Vue.prototype.$bus= this // 安装全局事件总线
}
})
未完待续.......
|