一文梳理React的核心概念
前言
React 是一个用于构建用户界面的 JavaScript 库
一、React的安装和配置
1.点击网站进入安装Node.js(为了运行 create-react-app) 安装Node.js 2.在终端打开Node.js的根目录并且运行
npx create-react-app moz-todo-react
这句命令创建了一个名为 moz-todo-react 的文件夹, 并在此文件夹里做了如下工作:
- 为你的应用程序安装了一些 npm 包;
- 写入 react 应用启动所需要的脚本文件;
- 创建一系列结构化的子文件夹和文件,奠定应用程序的基础架构;
- 如果你的电脑上安装了 git 的话,顺便帮你把 git 仓库也建好。
3.处理完成之后,你可以 cd 到 moz-todo-react 文件夹下,然后键入 npm start 命令并回车,先前由 create-react-app 创建的脚本会启动一个地服务 localhost:3000,并打开你的默认浏览器来访问这个服务。成功启动浏览器的话,你的浏览器上会显示如下画面:
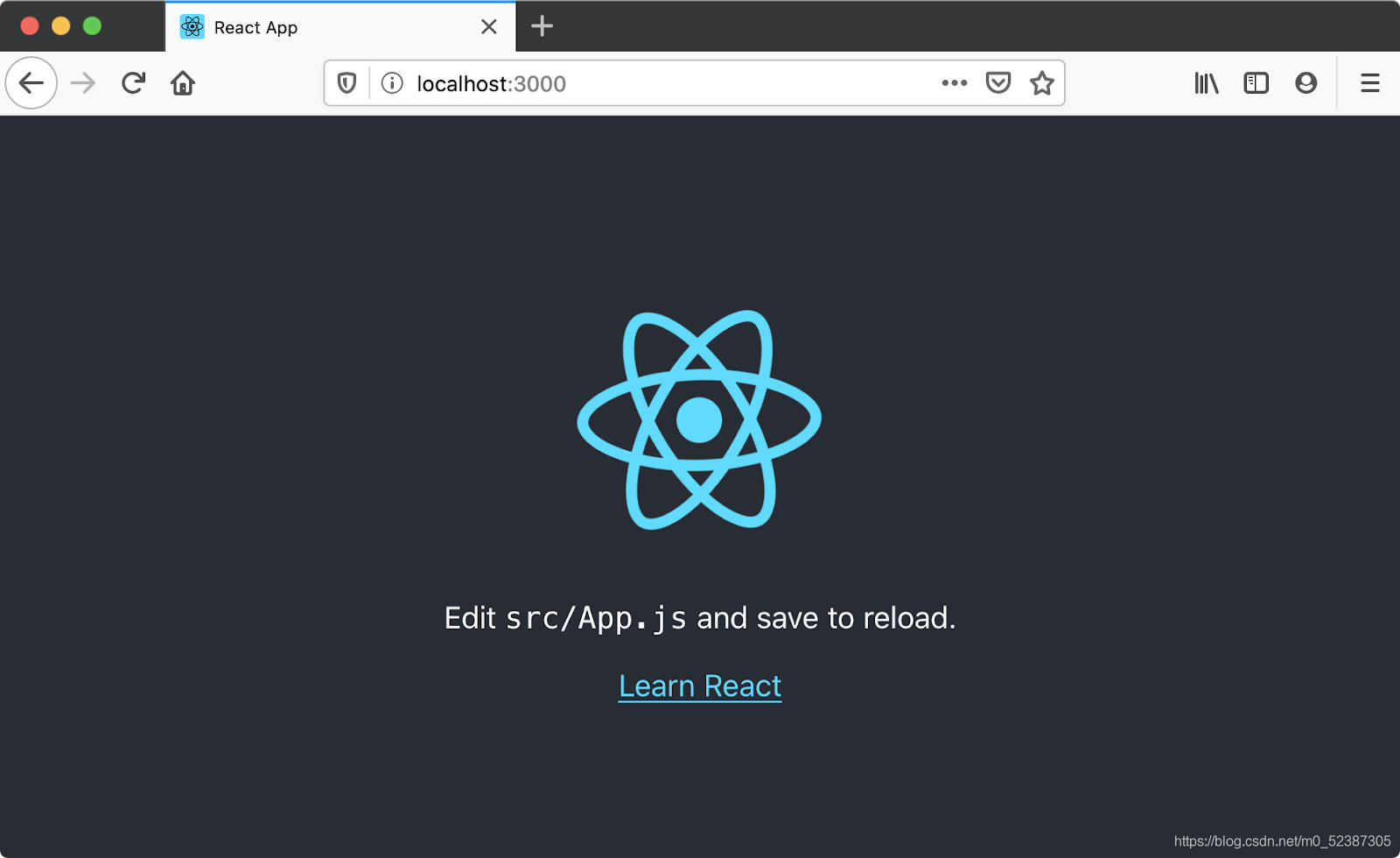 这样,React就安装好啦!
接下来,我会用一个项目来介绍 React的核心概念,在这个过程中可以对React的用法有更深的理解,不过前提是你必须有Web的基础:HTML、CSS、JavaScript
二、项目实战——体育用品仓库系统
1.设计UI
React提供了组件化的设计方式,因此,我们需要将实现的功能进行组件化,将每部分看作是一个组件,并对这一系列的组件进行设计和层次分级。 我们将要实现下图这样一个体育用品仓库系统 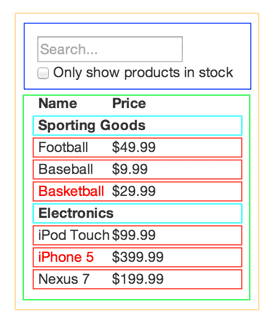 将各个组件进行标示: FilterableProductTable (橙色): 是整个示例应用的整体 SearchBar (蓝色): 接受所有的用户输入 ProductTable (绿色): 展示数据内容并根据用户输入筛选结果 ProductCategoryRow (天蓝色): 为每一个产品类别展示标题 ProductRow (红色): 每一行展示一个产品
根据功能进行层级划分:
- FilterableProductTable
- SearchBar
- ProductTable
- ProductCategoryRow
- ProductRow
2.用React创建一个静态版本
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Sporting Goods</title>
<script src="https://cdn.staticfile.org/react/16.4.0/umd/react.development.js"></script>
<script src="https://cdn.staticfile.org/react-dom/16.4.0/umd/react-dom.development.js"></script>
<script src="https://cdn.staticfile.org/babel-standalone/6.26.0/babel.min.js"></script>
</head>
<style>
</style>
<body>
<div id='root'></div>
<script type='text/babel'>
class ProductRow extends React.Component{
render(){
const product = this.props.product;
const name = product.stocked?product.name:<span style={{color:'red'}}>{product.name}</span>;
return(
<tr>
<td>{name}</td>
<td>{product.price}</td>
</tr>
);
}
}
class ProductCategoryRow extends React.Component{
render(){
const category = this.props.category;
return(
<tr>
<th>{category}</th>
</tr>
)
}
}
class ProductTable extends React.Component{
render(){
const rows = [];
let lastcategoty = null;
this.props.products.forEach((product) => {
if(product.category !==lastcategoty)
{
rows.push(<ProductCategoryRow category={product.category} key={product.category}/>);
}
rows.push(<ProductRow product={product} key={product.name}/>);
lastcategoty = product.category;
})
return(
<table>
<thead>
<tr>
<th >Name</th> <th>Price</th>
</tr>
</thead>
<tbody>{rows}</tbody>
</table>
)
}
}
class SearchBar extends React.Component{
render(){
return(
<form>
<input type='text' placeholder = " Enter to search..."/>
<p>
<input type='checkbox'/>
Only show products in stock
</p>
</form>
)
}
}
class FilterableProductTable extends React.Component{
render(){
return(
<div>
<SearchBar/>
<ProductTable products={this.props.products}/>
</div>
);
}
}
const PRODUCTS =[
{category: 'Sporting Goods', price: '$49.99', stocked: true, name: 'Football'},
{category: 'Sporting Goods', price: '$9.99', stocked: true, name: 'Baseball'},
{category: 'Sporting Goods', price: '$29.99', stocked: false, name: 'Basketball'},
{category: 'Electronics', price: '$99.99', stocked: true, name: 'iPod Touch'},
{category: 'Electronics', price: '$399.99', stocked: false, name: 'iPhone 5'},
{category: 'Electronics', price: '$199.99', stocked: true, name: 'Nexus 7'}
];
ReactDOM.render(
<FilterableProductTable products={PRODUCTS}/>,
document.getElementById('root')
);
</script>
</body>
</html>
3.加入动态元素
三、
总结
|