环境
-
node:v14.17.4 -
npm: 6.14.14 -
配置cnpm
0.如果在使用npm安装的时候出现了 “Missing write Acess”,执行下面这个给权限
sudo chown -R $USER /usr/local/lib/node_modules```
1.下载中国镜像版npm ,最后保存在了/usr/local/lib/node_modules/cnpm/bin/cnpm
打开方式 command + shift + g 该文件路径
npm install -g cnpm --registry=https://registry.npm.taobao.org
2.为了避免出现npm和cnpm下载冲突,看网上说是cnpm使用软链接,导致npm安装后就会更新cnpm包,从而导致之前的cnpm会gg,因此先设置一个使用谁
npm install -g nrm
nrm use cnpm
3.如果项目已经使用了cnpm的话
cnpm i --by=npm
cnpm install vue-cli -g
查看基于哪些模板创建vue应用程序,通常使用 webpack
vue list
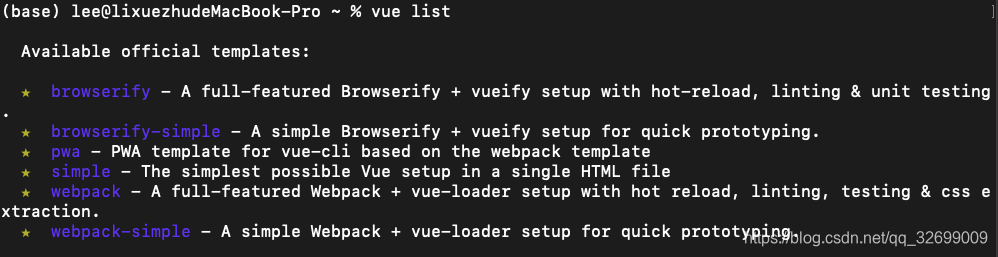
vue init webpack myvue
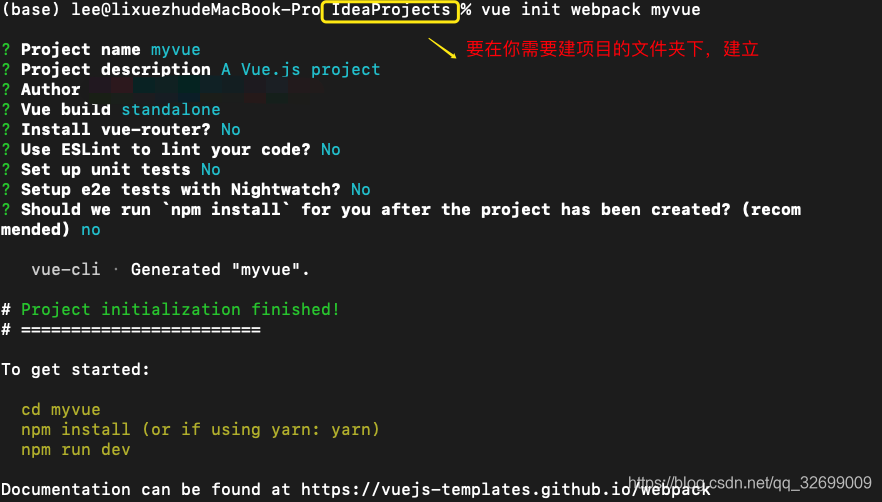
cd myvue
cnpm install
cnpm install webpack -g
cnpm install webpack-cli -g
测试安装成功没有
webpack -v
安装成功显示: 
使用webpack
官方文档说的是webpack是一个module budler(模块打包器)
为啥要打包?
如果原本的html页面需要引入不同js文件的话,那么就需要在页面中用script标签去引入
// index.html
<!doctype html>
<html>
<head><link href="main.css" rel="stylesheet"></head>
<body>
<div>hello world</div>
<script type="text/javascript" src="a.js"></script>
<script type="text/javascript" src="b.js"></script>
<script type="text/javascript" src="c.js"></script>
</body>
</html>
因为存在3个js文件,那么浏览器就需要发送三次http请求来获取这是哪个文件,然后获取到了在执行其中的代码,如果有一个文件因为网络问题延误了,会导致整个文件延误。
那么在项目很大的情况下,问题会变得更严重
因此是不是把所有的js文件合成一个文件然后再传输就好了?
显然在开发中合并这些是不合理的,也不利于维护,所以用打包的方式解决
打包内容:模块
在js中可以把模块看作是 一堆代码,这堆代码是可以复用的,【在我理解是一个工具类?】
需要执行具体的某个操作,就可以调用被暴露的(exports)模块功能给外面的用【在我理解就是这个方法被public了?】
打包是webpack最核心的功能,webpack其它所有的功能都是为了让打包这个功能更好。我们从一个简单的html页面介绍了通过webpack对模块进行打包,既保留了单个模块的可维护性,又减少了页面的http请求,减少了页面加载时间,从而增加了页面的显示速度,让整个应用的体验更好
因此结合上面所述,将这些众多的模块打包到bundle.js,bundle的中文意思也就是捆
那么捆好了自然就放到所以要渲染的页面中使用即可,下面是流程
流程1:exports&require
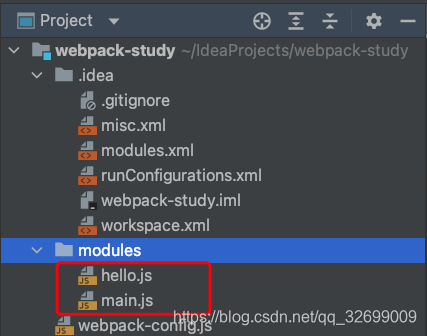 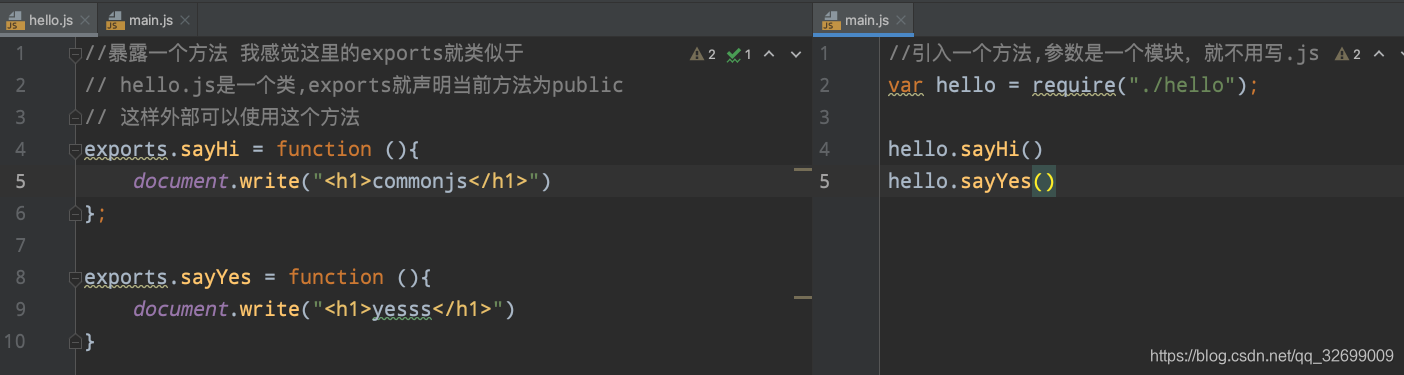
流程2: module打包
是一个js应用程序的静态模块打包器,当webpack处理应用程序时,它就会递归地构建一个依赖关系图,这个关系图包含了程序需要的模块,然后将这些模块打包成bundle
打包的文件名 webpack.config.js
module.exports = {
entry: './modules/main.js',
output:{
filename: "./js/bundle.js"
}
};
然后在终端输入打包命令
webpack
打包成功 
然后会看见目录下生成一个dist里面有一个bundle.js,里面就存放了我们打包压缩的东西
流程3:使用bundle.js
在需要的页面引入bundle,js文件即可
<script src="dist/js/bundle.js"></script>
Vue Router
主要要局部安装,在myvue文件下的终端里安装
意思就是 安装vuerouter 放在dev下
cnpm install vue-router --save-dev
使用router
npm run dev
index一般代表主配置,里面包含了路由跳转信息
这里需要引入配好vue-router的组件、app、以及你需要的组件!
import Vue from "vue";
import VueRouter from "vue-router";
import Content from "../components/Content";
import Main from "../components/Main";
Vue.use(VueRouter);
export default new VueRouter({
routes: [
{
path: '/content',
name: 'content',
component: Content
},
{
path: '/main',
name: 'content',
component: Main
}
]
})
<template>
<div id="app">
<h1>Vue-Router-Test</h1>
<router-link to="/main">首页</router-link>
<router-link to="/content">内容页</router-link>
<router-view></router-view>
</div>
</template>
<script>
export default {
name: 'App',
components: {
},
}
</script>
<style>
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
这里是配置的单服务页面
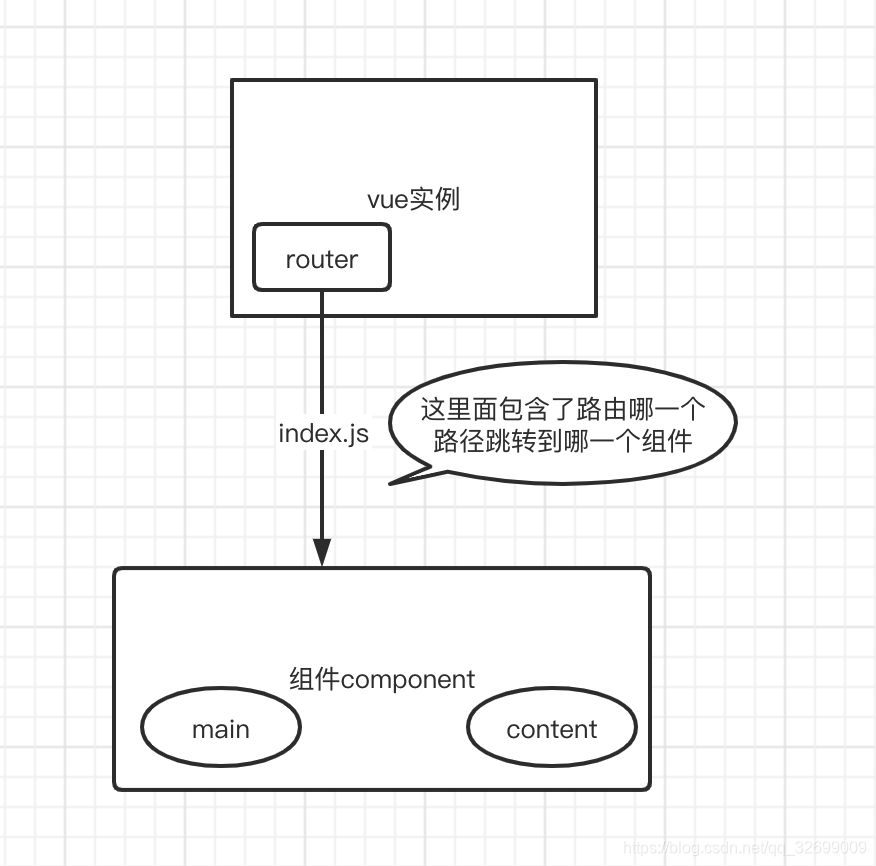
Vue + Element
初始化项目
1.初始化一个hello-vue的项目
vue init webpack hello-vue
2.进入这个文件夹
cd hello-vue
3.下载element-ui在当前项目下
cnpm i element-ui -S
4.安装依赖
cnpm install
5.安装sass加载器
cnpm install sass-loader node-sass --sava-dev
6.启动测试
npm run dev
登陆页
<template>
<div>
<el-form ref="loginForm" :model="form" :rules="rules" label-width="80px" class="login-box">
<h3 class="login-title">欢迎登录</h3>
<el-form-item label="账号" prop="username">
<el-input type="text" placeholder="请输入账号" v-model="form.username"/>
</el-form-item>
<el-form-item label="密码" prop="password">
<el-input type="password" placeholder="请输入密码" v-model="form.password"/>
</el-form-item>
<el-form-item>
<el-button type="primary" v-on:click="onSubmit('loginForm')">登录</el-button>
</el-form-item>
</el-form>
<el-dialog
title="温馨提示"
:visible.sync="dialogVisible"
width="30%"
:before-close="handleClose">
<span>请输入账号和密码</span>
<span slot="footer" class="dialog-footer">
<el-button type="primary" @click="dialogVisible = false">确 定</el-button>
</span>
</el-dialog>
</div>
</template>
<script>
export default {
name: "Login",
data() {
return {
form: {
username: '',
password: ''
},
rules: {
username: [
{required: true, message: '账号不可为空', trigger: 'blur'}
],
password: [
{required: true, message: '密码不可为空', trigger: 'blur'}
]
},
dialogVisible: false
}
},
methods: {
onSubmit(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
this.$router.push("/main");
} else {
this.dialogVisible = true;
return false;
}
});
}
}
}
</script>
<style lang="scss" scoped>
.login-box {
border: 1px solid #DCDFE6;
width: 350px;
margin: 180px auto;
padding: 35px 35px 15px 35px;
border-radius: 5px;
-webkit-border-radius: 5px;
-moz-border-radius: 5px;
box-shadow: 0 0 25px #909399;
}
.login-title {
text-align: center;
margin: 0 auto 40px auto;
color: #303133;
}
</style>
引入element
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
import App from './App.vue';
Vue.use(ElementUI);
new Vue({
el: '#app',
render: h => h(App)
});
以上代码便完成了 Element 的引入。需要注意的是,样式文件需要单独引入。
降sass版本
打开package.json ,这两个要配起来,只改一个貌似不得行
"node-sass": "^4.13.0",
"sass-loader": "^7.3.1",
嵌套路由
嵌套路由可以在整体视图不变的情况下部分内容的变化。
相当于在视图不变的情况下,profile变成了post,而user没有变 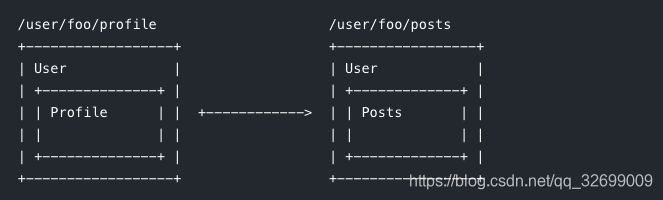 将要跳转的子页面放到index.js中
然后将子页面写入到children中,代表是嵌套路由
import Vue from 'vue'
import Router from 'vue-router'
import Login from "../views/Login";
import Main from "../views/Main";
import list from "../views/user/List";
import profile from "../views/user/Profile";
Vue.use(Router)
export default new Router({
routes: [
{
path: '/login',
component: Login
},
{
path:'/main',
component: Main,
children:[
{
path:'/user/List',
component: list
},
{
path:'/user/Profile',
component: profile
}
]
}
]
})
传递参数
前端传,router接受,然后router找到对应的页面展示
两个传参的方法
使用axios
- 安装
npm install --save axios vue-axios
- 将代码加入到入口文件,这里就是main.js
import Vue from 'vue'
import axios from 'axios'
import VueAxios from 'vue-axios'
Vue.use(VueAxios, axios)
到这儿我炸了。。。环境没了
|