##回调地狱
多层回调函数的相互嵌套,就形成了回调地狱
缺点:1.代码耦合性强,难以维护 2.冗余代码相互嵌套,代码可读性变差
##promise的基本概念
①promise是一个构造函数(实例对象代表一个异步操作)
②promise.prototype上包含一个.then()方法
③.then()方法用来预先指定成功和失败的回调函数
1.利用then-fs读取文件npm i then-fs
import thenFs from 'then-fs'
//普通promise调用,没有顺序
thenFs.readFile('./files/1.txt','utf8').then(res=>{
console.log('普通promise调用,没有顺序:',res);
})
thenFs.readFile('./files/2.txt','utf8').then(res=>{
console.log('普通promise调用,没有顺序:',res);
})
thenFs.readFile('./files/3.txt','utf8').then(res=>{
console.log('普通promise调用,没有顺序:',res);
})
// 按顺序读取文件内容promise链式调用
thenFs
.readFile('./files/1.txt','utf8')
.then(res1=>{
console.log('按顺序读取文件内容promise链式调用:',res1);
return thenFs.readFile('./files/2.txt','utf8')
})
.then(res2=>{
console.log('按顺序读取文件内容promise链式调用:',res2);
return thenFs.readFile('./files/3.txt','utf8')
})
.then(res3=>{
console.log('按顺序读取文件内容promise链式调用:',res3);
})
.catch(err=>{
console.log(err);
})
const promiseArr=[
thenFs.readFile('./files/1.txt','utf8'),
thenFs.readFile('./files/2.txt','utf8'),
thenFs.readFile('./files/3.txt','utf8')
]
//所有的异步操作全部结束后才执行下一步
Promise.all(promiseArr).then(res=>{
console.log('promise.all():',res);
})
.catch(err=>{
console.log(err);
})
//只要任何一个异步操作完成,就立即执行下一步的.then操作(看谁跑得快)
Promise.race(promiseArr).then(res=>{
console.log('promise.race():',res);
})
.catch(err=>{
console.log(err);
})
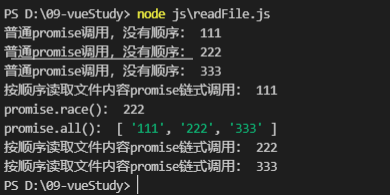
2.基于Promise封装读文件的方法
- 方法名要定义为getFile
- 方法接收一个形参fpath,表示要读取的文件路径
- 方法的返回值为promise实例对象
|