1.概述
Vuex 是一个专为 Vue.js 应用程序开发的状态管理模式 。它采用集中式存储管理 应用的所有组件的状态,并以相应的规则保证状态以一种可预测的方式 发生变化。
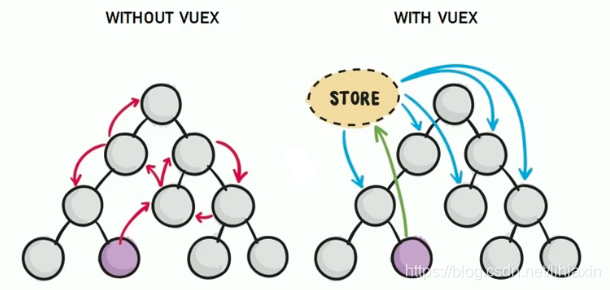
当紫色的组件需要向箭头方向的组件共享数据:
- 在没有Vuex的情况下,它需要通过链条的形式向他的父组件共享数据,父组件再向他的父组件再共享数据…这个过程需要很多的步骤才能将紫色组件的数据向兄弟组件共享。
- 在使用Vuex的情况下,紫色组件只需要通过共享数据状态对象
store 向兄弟组件共享数据。
Vuex的工作流程: 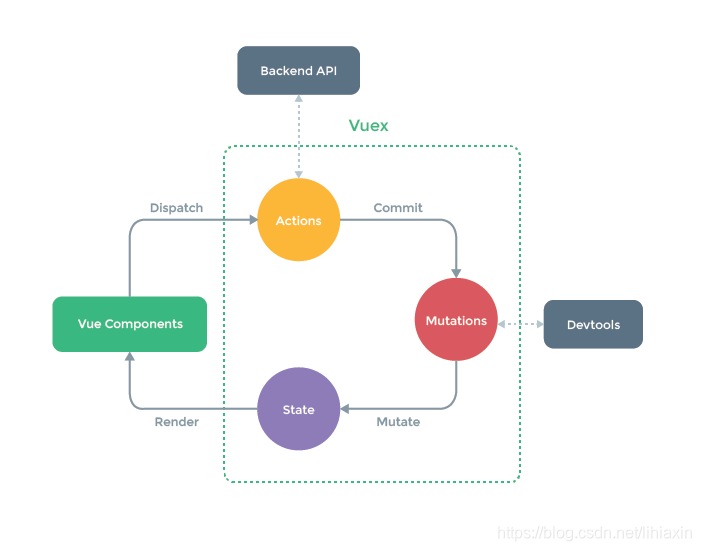
1.1 安装方法
npm i vuex -s
安装后,项目的文件夹应为: 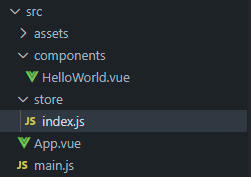 store.js中的代码为: 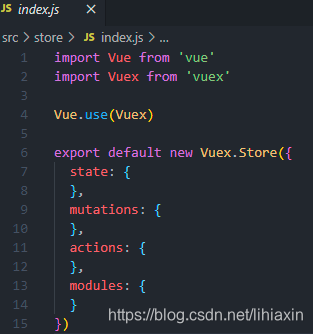
1.2 基本对象
- state:存放状态
- mutations:state成员操作
- getters:加工state成员给外界
- actions:异步操作
- modules:模块化状态管理
2.核心概念
2.1 State
State 提供唯一的公共数据源,所有共享的数据都要统一放到Store的State中进行存储。
const store = new vuex.store ({
state: { count: 0 }
})
(1)组件访问State中数据的第一种方式
this.$store.state.全局数据名称
比如:this.$store.state.count
(2)组件访问State中数据的第二种方式
import { mapstate } from 'vuex'
通过刚才导入的mapState函数,将当前组件需要的全局数据,映射为当前组件的computed计算属性:
export default{
computed: {
...mapState(['count'])
}
2.2 Getter
Getter 用于对Store中的数据进行加工处理 形成新的数据 。
①Getter 可以对Store中已有的数据加工处理之后形成新的数据,类似Vue的计算属性。
②Store 中数据发生变化,Getter 的数据也会跟着变化。
const store = new vuex.store ({
state: { count: 0 },
getters: {
share(state){
return 'count 的最新值为:'+state.count
}
}
})
(1)组件使用getters的第一种方式
this.$store.getters.名称
比如:this.$store.getters.count
(2)组件使用getters的第二种方式
import { mapGetters } from 'vuex'
通过刚才导入的mapGetters函数,将当前组件需要的全局数据,映射为当前组件的computed计算属性:
export default{
computed: {
...mapGetters (['share'])
}
2.3 Mutation
Mutation用于变更Store中的数据。
①只能通过mutation变更Store数据,不可以直接操作Store中的数据。
②通过这种方式虽然操作起来稍微繁琐一些,但是可以集中监控所有数据的变化。
const store = new vuex.store ({
state: { count: 0 },
mutation: {
add(state){
state.count ++
},
addN(state,step){
state.count +=step
}
}
})
(1)组件触发mutation的第一种方式
export default {
methods:{
addcount(){
this.$store.commit('add')
},
addNcount(){
this.$store.commit('addN',2)
},
}
}
(2)组件触发mutation的第二种方式
import { mapMutation } from 'vuex'
通过刚才导入的mapMutations函数,将需要的mutations函数,映射为当前组件的methods方法:
methods: {
...mapMutations (['add','addN'])
}
2.4 Action
由于直接在mutation方法中进行异步操作,将会引起数据失效。所以提供了Actions来专门进行异步操作,最终提交mutation方法。
const store = new Vuex. store ({
mutation: {
add(state){
state.count ++
},
addN(state,step){
state.count +=step
}
}
actions: {
addAsync (context) {
setT imeout( () => {
context.commit('add')
},1000)
},
addNAsync (context,step) {
setT imeout( () => {
context.commit('addN',step)
},1000)
}
})
(1)组件触发action的第一种方式
methods: {
handle() {
this.$store.dispatch('addAsync')
this.$store.dispatch('addNAsync', 5)
}
}
(2)组件触发mutation的第二种方式
import { mapActions } from 'vuex'
通过刚才导入的mapActions函数,将需要的actions函数,映射为当前组件的methods方法:
methods: {
...mapActions([ 'addASync','addNASync'])
}
2.5 Module
由于使用单一状态树,应用的所有状态会集中到一个比较大的对象。当应用变得非常复杂时,store 对象就有可能变得相当臃肿。
为了解决以上问题,Vuex 允许我们将 store 分割成模块(module)。每个模块拥有自己的 state、mutation、action、getter、甚至是嵌套子模块——从上至下进行同样方式的分割:
const moduleA = {
state: () => ({ ... }),
mutations: { ... },
actions: { ... },
getters: { ... }
}
const moduleB = {
state: () => ({ ... }),
mutations: { ... },
actions: { ... }
}
const store = new Vuex.Store({
modules: {
a: moduleA,
b: moduleB
}
})
store.state.a
store.state.b
(1)模块的局部状态 对于模块内部的 mutation 和 getter,接收的第一个参数是模块的局部状态对象。
const moduleA = {
state: () => ({
count: 0
}),
mutations: {
increment (state) {
state.count++
}
},
getters: {
doubleCount (state) {
return state.count * 2
}
}
}
同样,对于模块内部的 action,局部状态通过 context.state 暴露出来,根节点状态则为 context.rootState:
const moduleA = {
actions: {
incrementIfOddOnRootSum ({ state, commit, rootState }) {
if ((state.count + rootState.count) % 2 === 1) {
commit('increment')
}
}
}
}
对于模块内部的 getter,根节点状态会作为第三个参数暴露出来:
const moduleA = {
getters: {
sumWithRootCount (state, getters, rootState) {
return state.count + rootState.count
}
}
}
|