bootstrap-table官方教程 为了有更好的代入感,所以先看截图
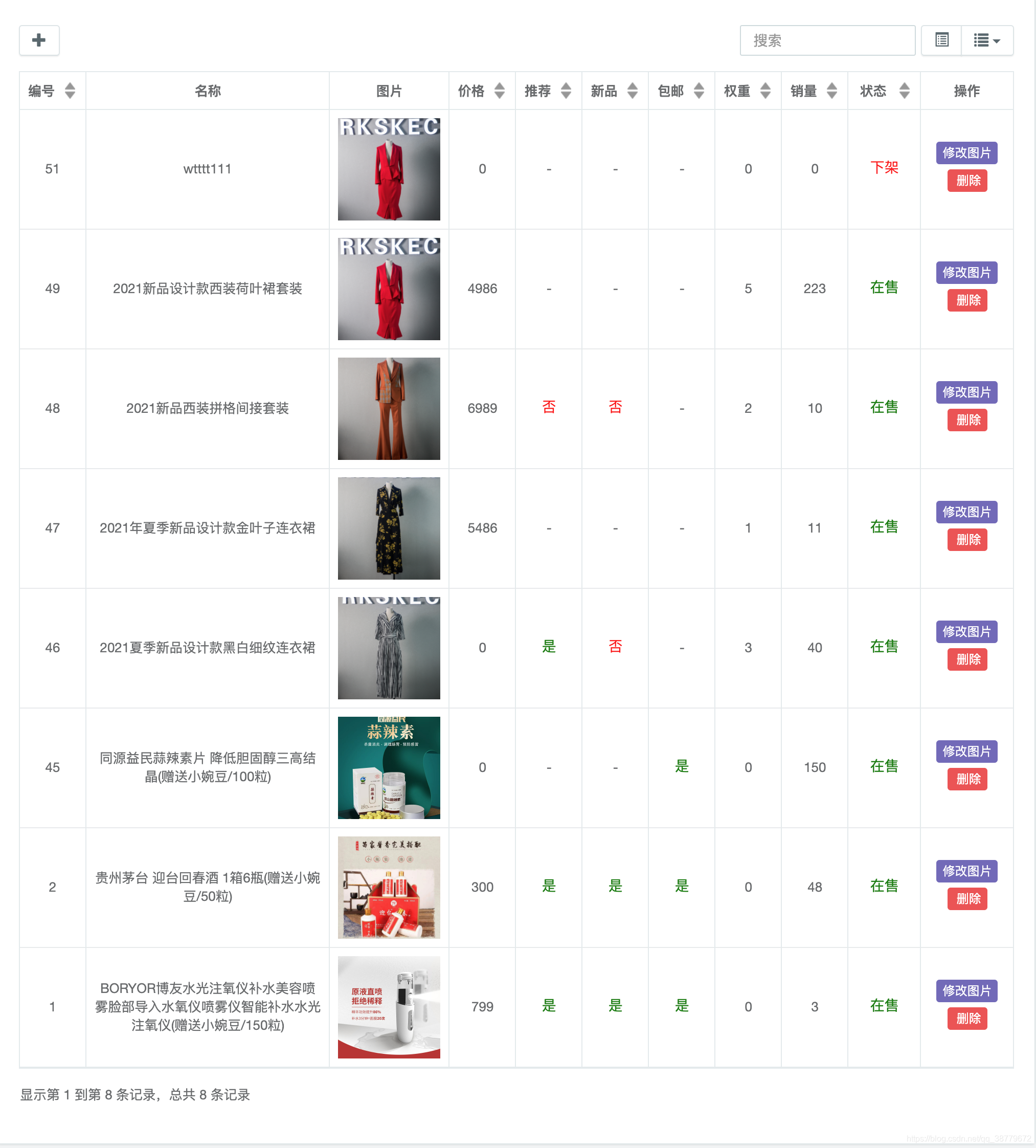
一、初始化
table.html
<table id="table" data-mobile-responsive="false">
table.js
(function() {
init();
$('#table').bootstrapTable({
search: true,
cache:false,
clickToSelect:true,
pageSize: 15,
pageNumber: 1,
pagination: true,
showToggle: true,
showColumns: true,
iconSize: 'outline',
toolbar: '#sliderToolbar',
icons: {
refresh: 'glyphicon-repeat',
toggle: 'glyphicon-list-alt',
columns: 'glyphicon-list'
},
columns: [{
field: 'id',
title: '编号',
align: 'center',
sortable: true,
}, {
field: 'name',
title: '名称',
align: 'center'
}, {
field: 'cover',
title: '图片',
align: 'center',
formatter:function(value,row,index){
if(value.indexOf("http") != -1){
return '<img src="' + value + '" height="100px" width="100px"/>';
} else {
return '<span>' + value + '</span>';
}
}
}, {
field: 'price',
title: '价格',
align: 'center',
sortable: true,
}, {
field: 'is_rec',
title: '推荐',
align: 'center',
sortable: true,
formatter:function(value,row,index){
if(value == 1){
return '<a class="btn" style="color: green">是</a>';
}
if(value == 2){
return '<a class="btn" style="color: red">否</a>';
}
}
}, {
field: 'is_new',
title: '新品',
align: 'center',
sortable: true,
formatter:function(value,row,index){
if(value == 1){
return '<a class="btn" style="color: green">是</a>';
}
if(value == 2){
return '<a class="btn" style="color: red">否</a>';
}
}
}, {
field: 'is_post',
title: '包邮',
align: 'center',
sortable: true,
formatter:function(value,row,index){
if(value == 1){
return '<a class="btn" style="color: green">是</a>';
}
if(value == 2){
return '<a class="btn" style="color: red">否</a>';
}
}
}, {
field: 'sort',
title: '权重',
align: 'center',
sortable: true,
}, {
field: 'sales',
title: '销量',
align: 'center',
sortable: true,
}, {
field: 'status',
title: '状态',
align: 'center',
sortable: true,
formatter:function(value,row,index){
if(value == 1){
return '<a class="btn" style="color: green">在售</a>';
}
if(value == 2){
return '<a class="btn" style="color: red">下架</a>';
}
}
}, {
field: 'operate',
title: '操作',
align: 'center',
formatter:function(value,row,index){
return '\
<a href="check_good.html" class="btn btn-xs btn-primary J_menuItem"><i class="fa fa-delete"></i>修改图片</a>\
<a id="deleteRow" class="btn btn-xs btn-danger"><i class="fa fa-delete"></i> 删除</a>\
';
},
events: {
'click #deleteRow': function (e, value, row, index) {
deleteRow(row.id)
},
}
}],
onClickCell: function(field, value, row, $element) {
if (field == "name" || field == "price" || field == "sort") {
$element.attr('contenteditable', true);
$element.blur(function() {
let index = $element.parent().data('index');
let tdValue = $element.html();
saveData(index, field, tdValue, row);
})
}
}
});
})();
二、使用请求后接口数据
全局变量
var goodList;
init()
function init(){
"use strict";
$('#table').bootstrapTable("showLoading");
var datas = JSON.stringify({"cur_page" : 1, "page_size" : page_size});
$.ajax({
url: baseUrl+"/admin/shop/list",
method: "post",
data: datas,
success: function(rs){
goodList = rs.data.datas
$('#table').bootstrapTable('load', goodList);
$('#table').bootstrapTable('refresh');
$('#table').bootstrapTable("hideLoading");
},
error:function(d){
console.log(d)
}
})
};
三、行操作
1. 请求完接口,前端过滤删除的row
deleteRow()
function deleteGood(id){
var datas = JSON.stringify({"id" : id});
$.ajax({
url: baseUrl+"/admin/shop/del",
method: "post",
data: datas,
success: function(rs){
if(rs.code == 0){
var newGoodList = goodList.filter((item, i) => item['id'] != id)
$('#table').bootstrapTable('load', newGoodList);
$('#table').bootstrapTable('refresh');
$('#table').bootstrapTable("hideLoading");
}
},
error:function(d){
console.log(d)
}
})
}
2. 请求完接口,前端手动修改row
saveData()
function saveData(index, field, value, row) {
"use strict";
$('#table').bootstrapTable('updateCell', {
index: index,
field: field,
value: value
})
var map = {"id" : row.id};
if(field == "price" || field == "sort"){
value = Number(value);
}
map[field] = value;
var datas = JSON.stringify(map);
$.ajax({
url: baseUrl+"/admin/shop/edit",
method: "post",
data: datas,
success: function(rs){
if(rs.code != 0){
alert("修改失败!"+rs.data)
}
},
error:function(d){
console.log(d)
}
})
}
四、总结
1. 解决BUG: 开启sort后不显示箭头符号
直接引用即可
.fixed-table-container thead th .sortable {
background-image: url('img/sort_sort.png');
background-size: 10px 16px;
cursor: pointer;
background-position: right;
background-repeat: no-repeat;
padding-right: 20px;
margin-right: 10px;
}
.fixed-table-container thead th .asc {
background-image: url('img/sort_asc.png');
background-size: 10px 16px;
cursor: pointer;
background-position: right;
background-repeat: no-repeat;
padding-right: 20px;
margin-right: 10px;
}
.fixed-table-container thead th .desc {
background-image: url('img/sort_desc.png');
background-size: 10px 16px;
cursor: pointer;
background-position: right;
background-repeat: no-repeat;
padding-right: 20px;
margin-right: 10px;
}
  
2. 监听每个cell点击事件
onClickCell: function(field, value, row, $element) {
if (field == "name" || field == "price" || field == "sort") {
$element.attr('contenteditable', true);
$element.blur(function() {
let index = $element.parent().data('index');
let tdValue = $element.html();
saveData(index, field, tdValue, row);
})
}
}
3. 更新某cell数据
$('#table').bootstrapTable('updateCell', {
index: index,
field: field,
value: value
})
4. 更新某行数据
$('#table').bootstrapTable('updateRow', {
index: index,
row: row
})
5. 自定义列样式
{
field: 'is_post',
title: '包邮',
align: 'center',
sortable: true,
formatter:function(value, row, index){
if(value == 1){
return '<a class="btn" style="color: green">是</a>';
}
if(value == 2){
return '<a class="btn" style="color: red">否</a>';
}
}
}
6. 重新加载数据
$('#table').bootstrapTable('load', []);
$('#table').bootstrapTable('refresh');
7. 显示或隐藏加载状态
$('#table').bootstrapTable("showLoading");
$('#table').bootstrapTable("hideLoading");
|