1.前后端调用
1.1请求类型说明
分组:
?????????get/delete? 用法一致
???????? post/put? ??? 用法一致
1.2 delete请求
前段页面编辑
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>前后端调用delete</title>
</head>
<body>
<script src="../js/axios.js"></script>
<script>
// delete 测试1:需求:删除ID=232的数据
let id=
let url ="http://localhost:8090/axios/deleteById?id=${233}"
axios.delete(url).then(promise => {
console.log(promise.data)
})
</script>
</body>
</html>
后端controller层
@DeleteMapping("deleteById")
public String delete(Integer id){
userService.deleteById(id);
return "删除成功";
}
业务层Service
@Override
public void deleteById(Integer id) {
userMapper.deleteById(id);
}
执行结果
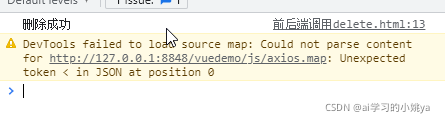
1.3 post请求
1.3.1 新增用户请求
url:请求地址:http://localhost:8090/axios/saveUser
请求参数的结构:是一个JSON串
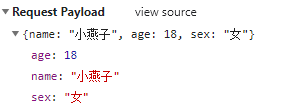
?难点:
1.user对象可以转换成json串? @RestController中的@ResponseBody带的方法
2.json转换为user对象 @Requestbody? 自能加在对象前
controller层代码
@PostMapping("saveUser")
public String saveUser(@RequestBody User user){
userService.saveUser(user);
return "添加成功";
}
?service业务层代码:
@Override
public void saveUser(User user) {
userMapper.insert(user);
}
前段页面编辑:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>post请求</title>
</head>
<body>
<h1>前后端调用 -POST请求测试</h1>
<script src="../js/axios.js"></script>
<script>
/*
需求:实现用户新增操作
语法:axious.post(url,新增参数)
规则:如果post/put 传递对象则直接赋值
*/
let user={
name:'小燕子',
age:18,
sex:'女'
}
let url="http://localhost:8090/axios/saveUser"
axios.post(url,user)//{param : user}只适用于get与delete
.then(promise =>{
console.log(promise.data)
})
</script>
</body>
</html>
post请求结构:
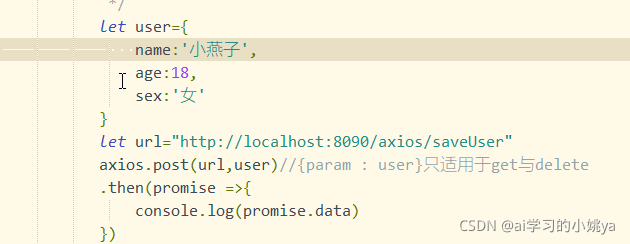
1.4 put请求
2 vue-Axios案例
2.1需求说明
1.当展现页面时,用户发起请求 http://localhost:8090/vue/findAll,获取所有的user数据
2.通过Vue.js要求在页面上展现数据,以表格的形式展现
3.为每行数据添加 修改/删除的按钮
4.在一个新的div中? 编辑3个文本框 name/age/sex通过提交按钮实现新增
5.如果用户点击修改按钮,则在全新的div中,回显数据。
6.用户修改完数据后,通过提交按钮 实现数据的修改。
?7.当用户点击删除按钮时,要求实现数据的删除的操作。
2.2 知识点梳理
1.vue页面初始化时,如何发起ajax请求
methods:{
getUserList(){
alert("查询数据!")
}
},created(){ //利用声明周期函数触发ajax
alert("声明周期函数")
this.getUserList()
}
2. vue定义axios请求的前缀
axios.defaults.baseURL ="http://localhost:8090/vue"
3.axios与vue数据结合
说明:当通过axios获取服务器数据之后,应该将该数据传给vue.data的属性
4.用户输入双向绑定
说明:在vue.js中一般情况下,如果遇到了用户输入的情况下需要设置双向数据绑定
3.案例(总结)
1.查询demo_user表中所有的表记录?? get(url)
前段代码步骤
a.导入jar包 vue.js??? ajax.js
b.在<script></scrpit>标签中加入??? axios.defaults.baseURL="http://localhost:8090/vue" 可以简化url的写法,不用每个操作都添加这部分地址
c.使用循环进行遍历,将结果赋予表格中? v-for(user in userList)??? v-text("user.属性")
2.增加记录? post(url,user)
3.删除记录 delete(url+user.id)? 通过id删除
4.修改记录 put(url+新user)?
前段代码如下:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<div id="app">
<!-- 新增 -->
<div align="center">
<h3>用户新增</h3>
姓名:<input type="text" v-model="addUser.name"/>
年龄:<input type="text" v-model="addUser.age"/>
性别:<input type="text" v-model="addUser.sex"/>
<button @click="addUserBtn()">添加</button>
</div>
<!-- 修改 -->
<div align="center" >
<h3>用户修改</h3>
编号: <input type="text" v-model="newUser.id" />
姓名:<input type="text" v-model="newUser.name"/>
年龄:<input type="text" v-model="newUser.age"/>
性别:<input type="text" v-model="newUser.sex"/>
<button @click="update2()">修改</button>
</div>
<div>
<table border="1px" width="80%" align="center" style="margin-top: 20px;">
<tr align="center">
<th colspan="5"><h3>用户列表</h3></th>
</tr>
<tr align="center">
<th>编号</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>操作</th>
</tr>
<tr align="center" v-for="user in userList">
<th v-text="user.id"></th>
<th v-text="user.name"></th>
<th v-text="user.age"></th>
<th v-text="user.sex"></th>
<th>
<button @click="update1(user)">修改</button>
<button @click="deleteUser(user)">删除</button>
</th>
</tr>
</table>
</div>
</div>
<script src="../js/axios.js"></script>
<script src="../js/vue.js"></script>
<script>
axios.defaults.baseURL="http://localhost:8090/vue"
new Vue({
el:"#app",
data:{
userList:[],
addUser:{
name:'',
age:'',
sex:''
},
newUser:{
id:'',
name:'',
age:'',
sex:''
}
},
methods:{
getUserList(){
alert("用户表如下:")
axios.get("findAll").then(promise =>{
this.userList= promise.data
})
},addUserBtn(){
axios.post("saveUser",this.addUser).then(promise =>{
let c = promise.data
alert(c)
this.getUserList()
this.addUser={}
})
},deleteUser(user){
axios.delete("deleteUserById?id="+user.id).then(promise =>{
let a = promise.data
alert(a)
this.getUserList()
})
},update1(user){
this.newUser=user
},update2(){
axios.put("updateById",this.newUser).then(promise =>{
let c = promise.data
alert(c)
this.getUserList()
this.newUser={}
})
}
},
created() {
this.getUserList()
}
})
</script>
</body>
</html>
后端代码:
controller层
package com.jt.controller;
import com.jt.Service.UserService;
import com.jt.pojo.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@CrossOrigin
@RequestMapping("vue")
public class ProController {
@Autowired
private UserService userService;
@GetMapping("findAll")
public List<User> findAll(){
return userService.findAll();
}
@PostMapping("saveUser")
public String addUser(@RequestBody User user){
userService.addUser(user);
return "添加成功";
}
@DeleteMapping("deleteUserById")
public String deleteUserById(Integer id){
userService.deleteById(id);
return "删除成功";
}
@PutMapping("updateById")
public String updateById(@RequestBody User user){
userService.updateById(user);
return "修改成功!!";
}
}
service层
@Override
public List<User> findAll() {
return userMapper.selectList(null);
}
@Override
public void addUser(User user) {
userMapper.insert(user);
}
@Override
public void deleteById(Integer id) {
userMapper.deleteById(id);
}
@Override
public void updateById(User user) {
userMapper.updateById(user);
}
运行结果:
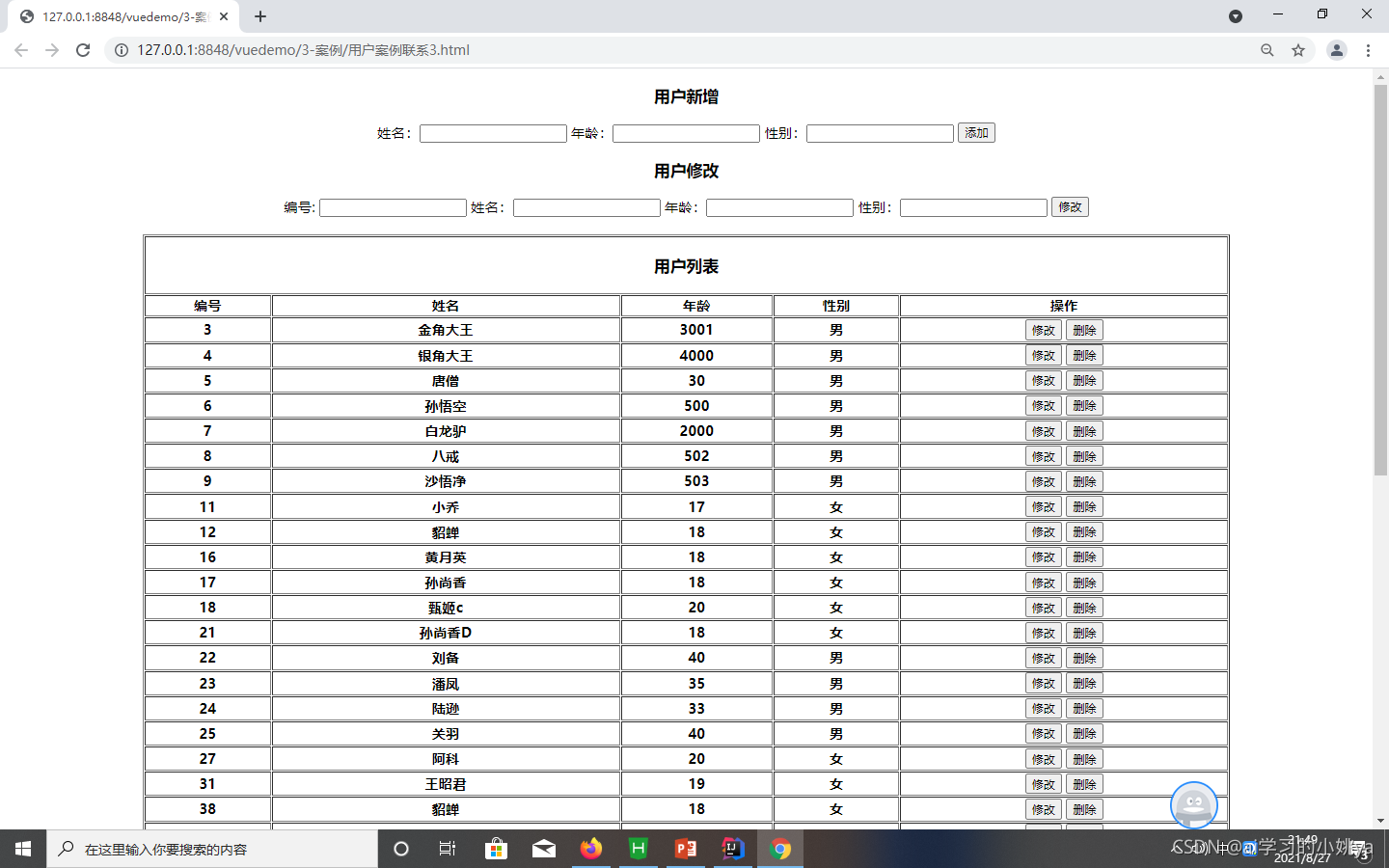
?新增:
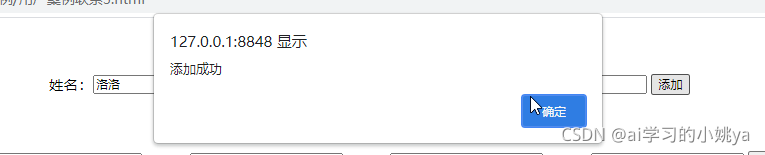
删除:
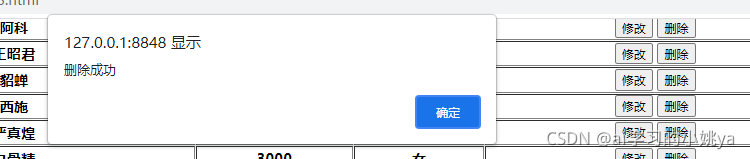
修改:
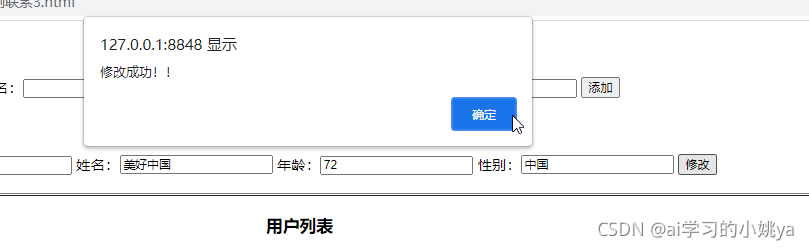
?
?
|