Vue 整合 Axios
提示:本篇基于【小项目】Axios 实现前后端交互
一、需求说明
- 用户发起请求 http://localhost:8080/vue/findAll,获取所有的 student 数据
- 通过 Vue.js 在页面中以表格的形式展现
- 为每行数据添加修改、删除的按钮
- 在一个新的 div 中,编辑 3 个文本框 name/age/sex 通过提交按钮实现新增
- 如果用户点击修改按钮,则在用户修改的 div 中回显数据
- 用户修改完成后,通过提交按钮实现数据的修改
- 当用户点击删除按钮时,要求实现数据的删除
二、数据库
把 student 表清空即可
三、前端
文件名:StudentTable.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>学生列表</title>
<script src="../js/axios.js"></script>
<script src="../js/vue.js"></script>
</head>
<body>
<div id="app">
<div align="center">
<h3>学生新增</h3>
姓名:<input type="text" v-model="addStu.name">
邮箱:<input type="text" v-model="addStu.email">
年龄:<input type="text" v-model="addStu.age">
<button @click="addStuBtn">新增</button>
</div>
<div align="center">
<h3>学生修改</h3>
<p>
编号:<input type="text" v-model="updateStu.id" disabled>
姓名:<input type="text" v-model="updateStu.name">
</p>
<p>
邮箱:<input type="text" v-model="updateStu.email">
年龄:<input type="text" v-model="updateStu.age">
</p>
<button @click="submitBtn">提交修改</button>
</div>
<table align="center" border="1px" width="80%" style="margin-top: 10px">
<tr align="center">
<th colspan="5"><h3>学生列表</h3></th>
</tr>
<tr>
<th>编号</th>
<th>姓名</th>
<th>邮箱</th>
<th>年龄</th>
<th>操作</th>
</tr>
<tr v-cloak v-for="student in studentList" align="center">
<th v-text="student.id"></th>
<th v-text="student.name"></th>
<th v-text="student.email"></th>
<th v-text="student.age"></th>
<th width="20%">
<button @click="updateStuBtn(student)">修改</button>
<button @click="deleteStuBtn(student)">删除</button>
</th>
</tr>
</table>
</div>
</body>
</html>
<script>
axios.defaults.baseURL = "http://localhost:8080/Vue"
const app = new Vue({
el:"#app",
data:{
studentList : [],
addStu : {
name : '',
email : '',
age : ''
},
updateStu : {
id : '',
name : '',
email : '',
age : ''
}
},
methods:{
getStuList(){
axios.get("/findAll").then(promise => {
this.studentList = promise.data
})
},
addStuBtn(){
axios.post("/addStu", this.addStu).then(promise => {
alert(promise.data)
this.getStuList()
this.addStu = {}
})
},
deleteStuBtn(student){
axios.delete("/deleteStu?id=" + student.id).then(promise => {
this.getStuList()
alert(promise.data)
})
},
updateStuBtn(student){
let temp = JSON.parse(JSON.stringify(student))
this.updateStu = temp
},
submitBtn(){
axios.put("/updateStu", this.updateStu).then(promise => {
alert(promise.data)
this.updateStu = {}
this.getStuList()
})
}
},
created(){
this.getStuList()
}
})
</script>
四、后端
1.StudentTableService.java
package com.sisyphus.studentssm.service;
import com.baomidou.mybatisplus.core.conditions.Wrapper;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.conditions.update.UpdateWrapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.baomidou.mybatisplus.extension.service.IService;
import com.sisyphus.studentssm.mapper.StudentMapper;
import com.sisyphus.studentssm.pojo.Student;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
@Service
public class StudentTableService implements IService<Student> {
@Autowired
private StudentMapper studentMapper;
public List<Student> findAll(){
QueryWrapper<Student> queryWrapper = new QueryWrapper<>();
queryWrapper
.select("id","name","email","age");
return studentMapper.selectList(queryWrapper);
}
public void addStu(Student student){
studentMapper.insert(student);
}
public void deleteStu(Integer whereId){
studentMapper.deleteById(whereId);
}
public void updateStu(Student student){
UpdateWrapper<Student> updateWrapper = new UpdateWrapper<>();
updateWrapper
.eq(true, "id", student.getId())
.set(true, "name",student.getName())
.set(true, "email",student.getEmail())
.set(true, "age",student.getAge());
studentMapper.update(student, updateWrapper);
}
@Override
public boolean saveBatch(Collection<Student> entityList, int batchSize) {
return false;
}
@Override
public boolean saveOrUpdateBatch(Collection<Student> entityList, int batchSize) {
return false;
}
@Override
public boolean updateBatchById(Collection<Student> entityList, int batchSize) {
return false;
}
@Override
public boolean saveOrUpdate(Student entity) {
return false;
}
@Override
public Student getOne(Wrapper<Student> queryWrapper, boolean throwEx) {
return null;
}
@Override
public Map<String, Object> getMap(Wrapper<Student> queryWrapper) {
return null;
}
@Override
public <V> V getObj(Wrapper<Student> queryWrapper, Function<? super Object, V> mapper) {
return null;
}
@Override
public BaseMapper<Student> getBaseMapper() {
return null;
}
@Override
public Class<Student> getEntityClass() {
return null;
}
}
2.StudentTableController.java
package com.sisyphus.studentssm.controller;
import com.sisyphus.studentssm.pojo.Student;
import com.sisyphus.studentssm.service.StudentTableService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("Vue")
@CrossOrigin
public class StudentTableController {
@Autowired
private StudentTableService studentTableService;
@GetMapping("findAll")
public List<Student> findAll(){
return studentTableService.findAll();
}
@PostMapping("addStu")
public String addStu(@RequestBody Student student){
studentTableService.addStu(student);
return "新增成功";
}
@DeleteMapping("deleteStu")
public String deleteStu(@RequestParam("id") Integer whereId){
studentTableService.deleteStu(whereId);
return "删除成功";
}
@PutMapping("updateStu")
public String updateStu(@RequestBody Student student){
studentTableService.updateStu(student);
return "修改成功";
}
}
五、测试
1.新增
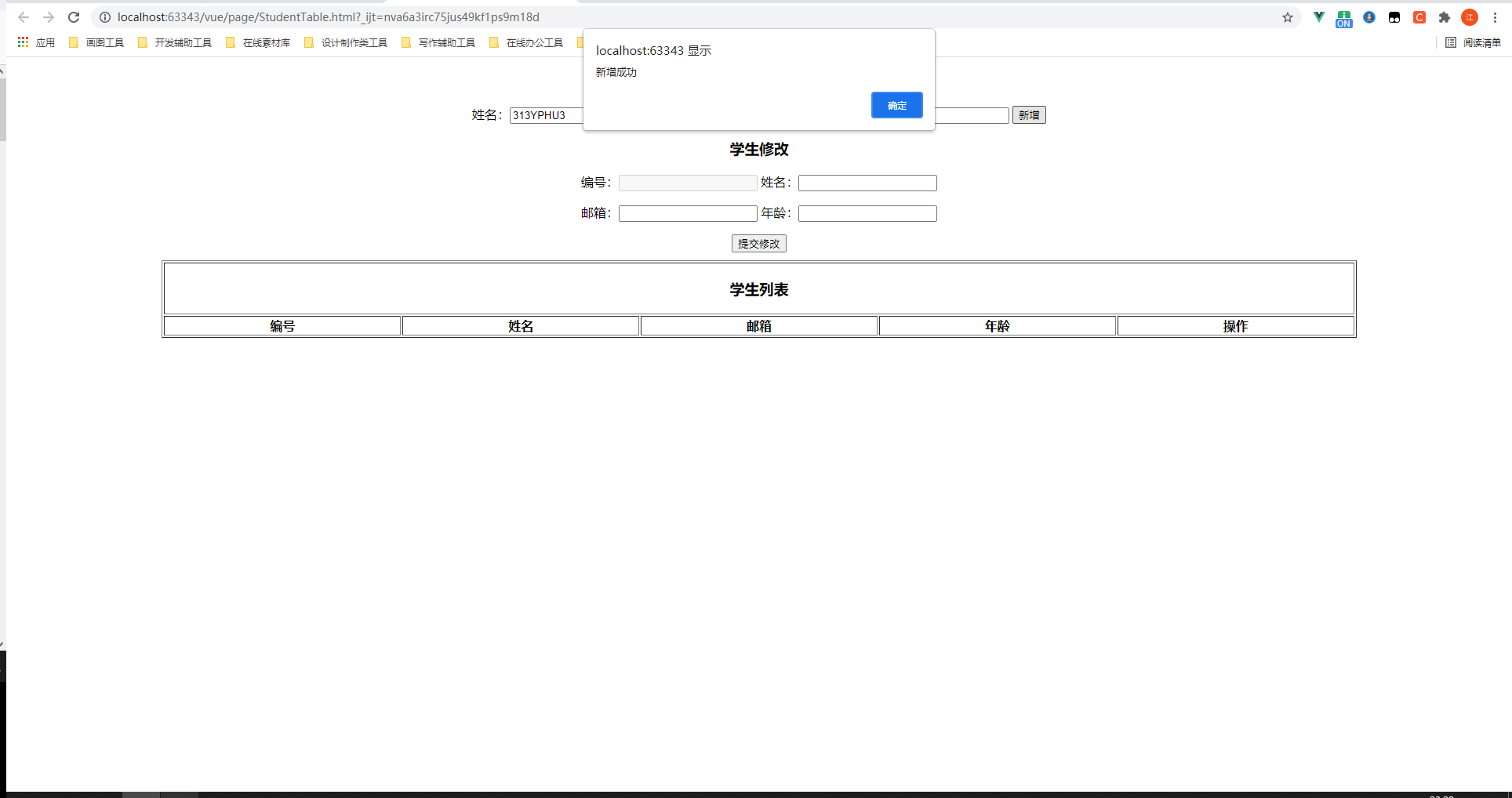
2.修改
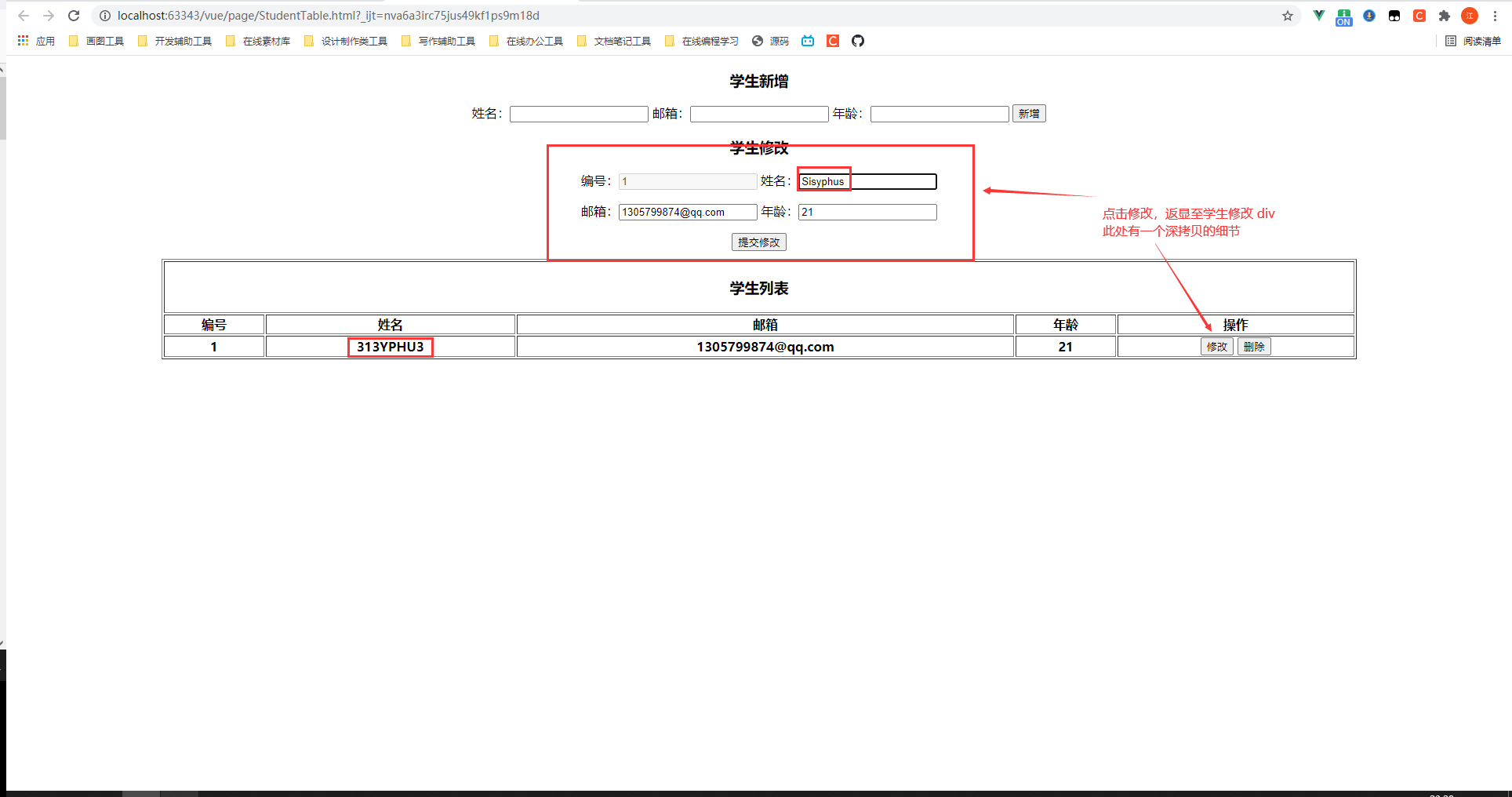
3.删除
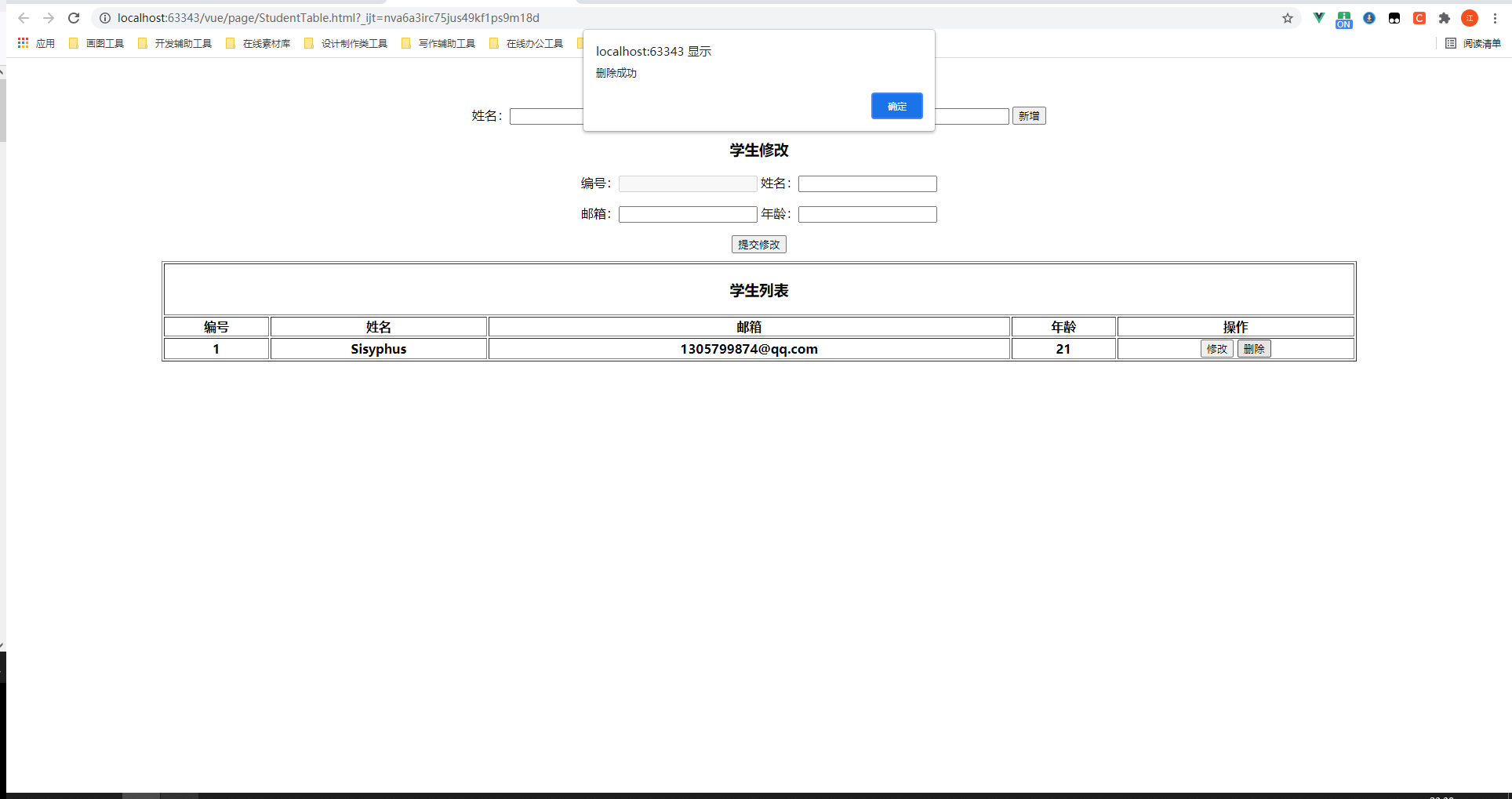 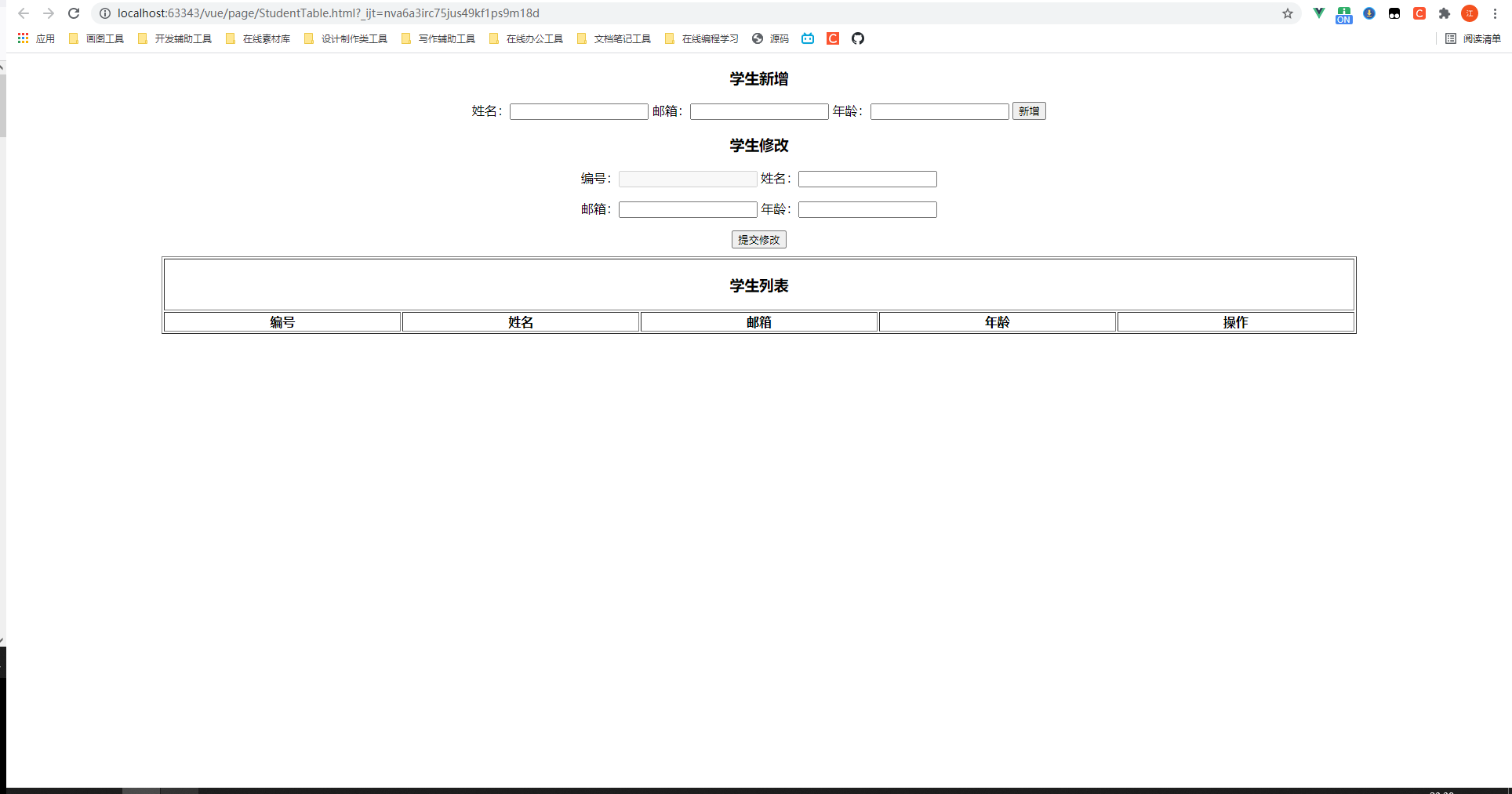
|