内置对象
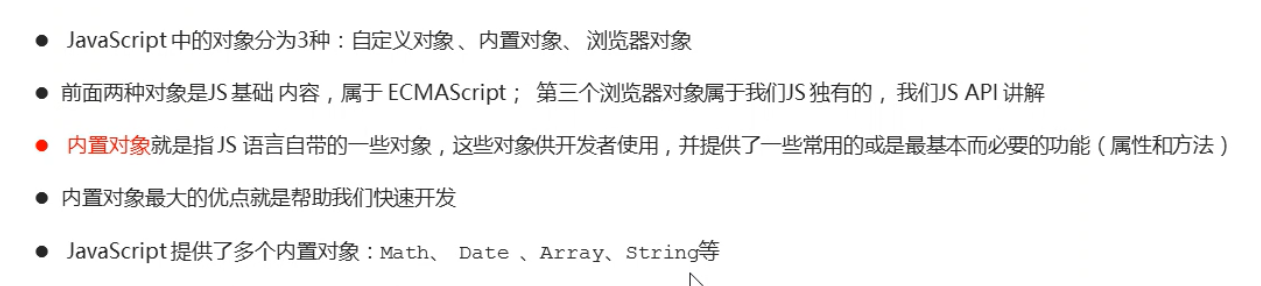
查阅MDN文档
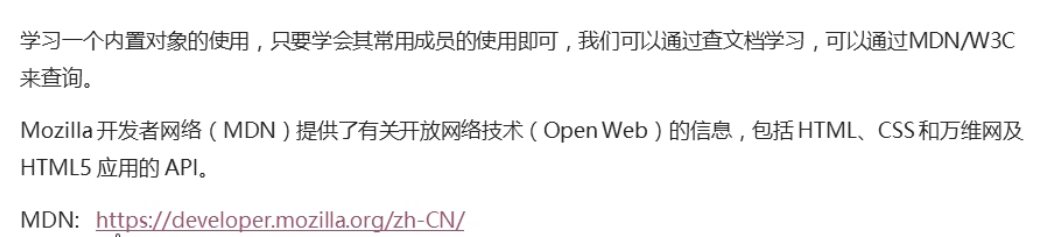 MDN 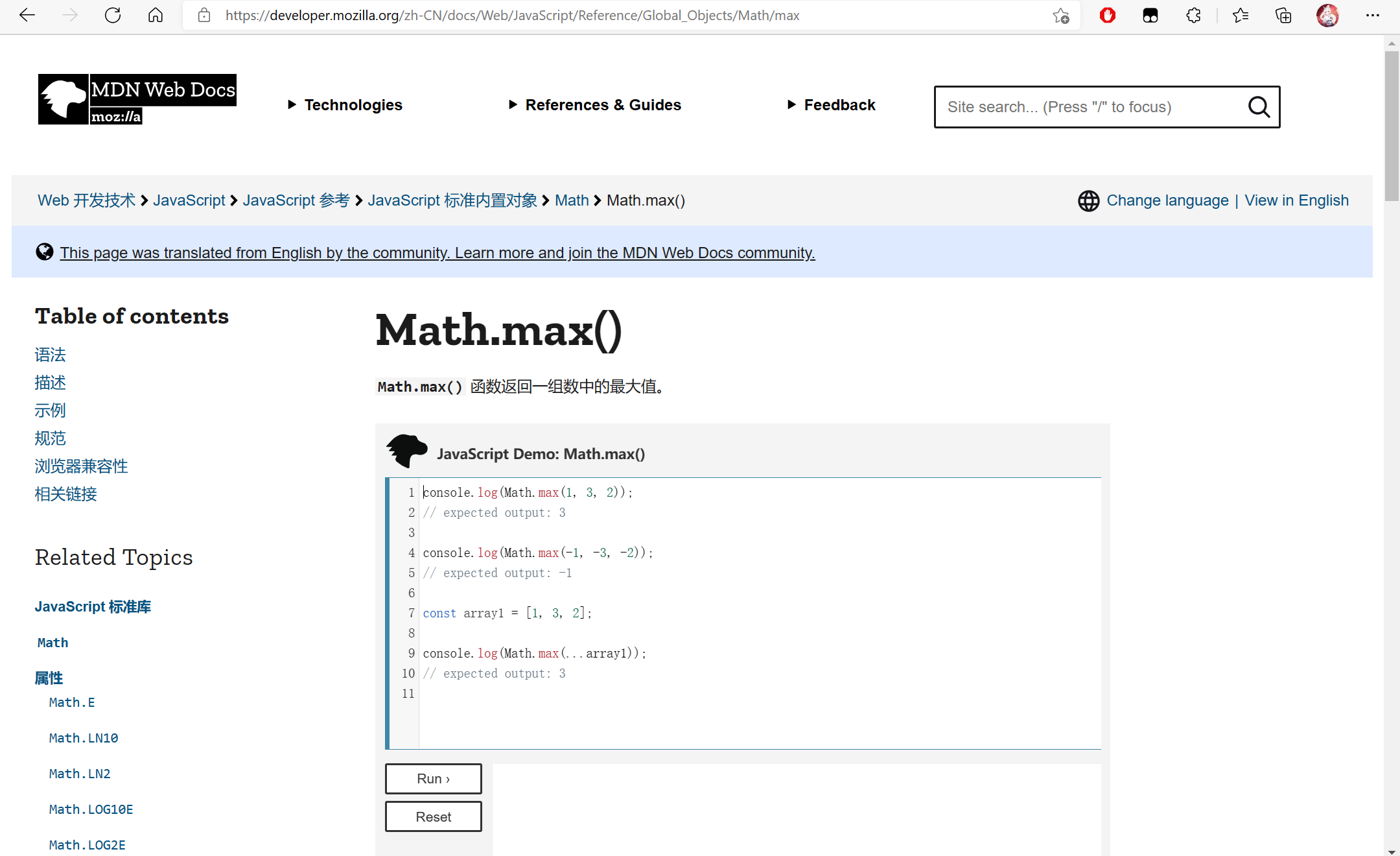 学习对象中的方法 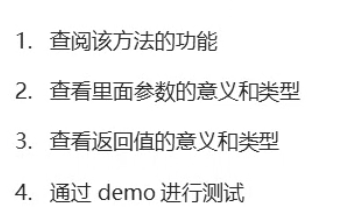
数学对象Math
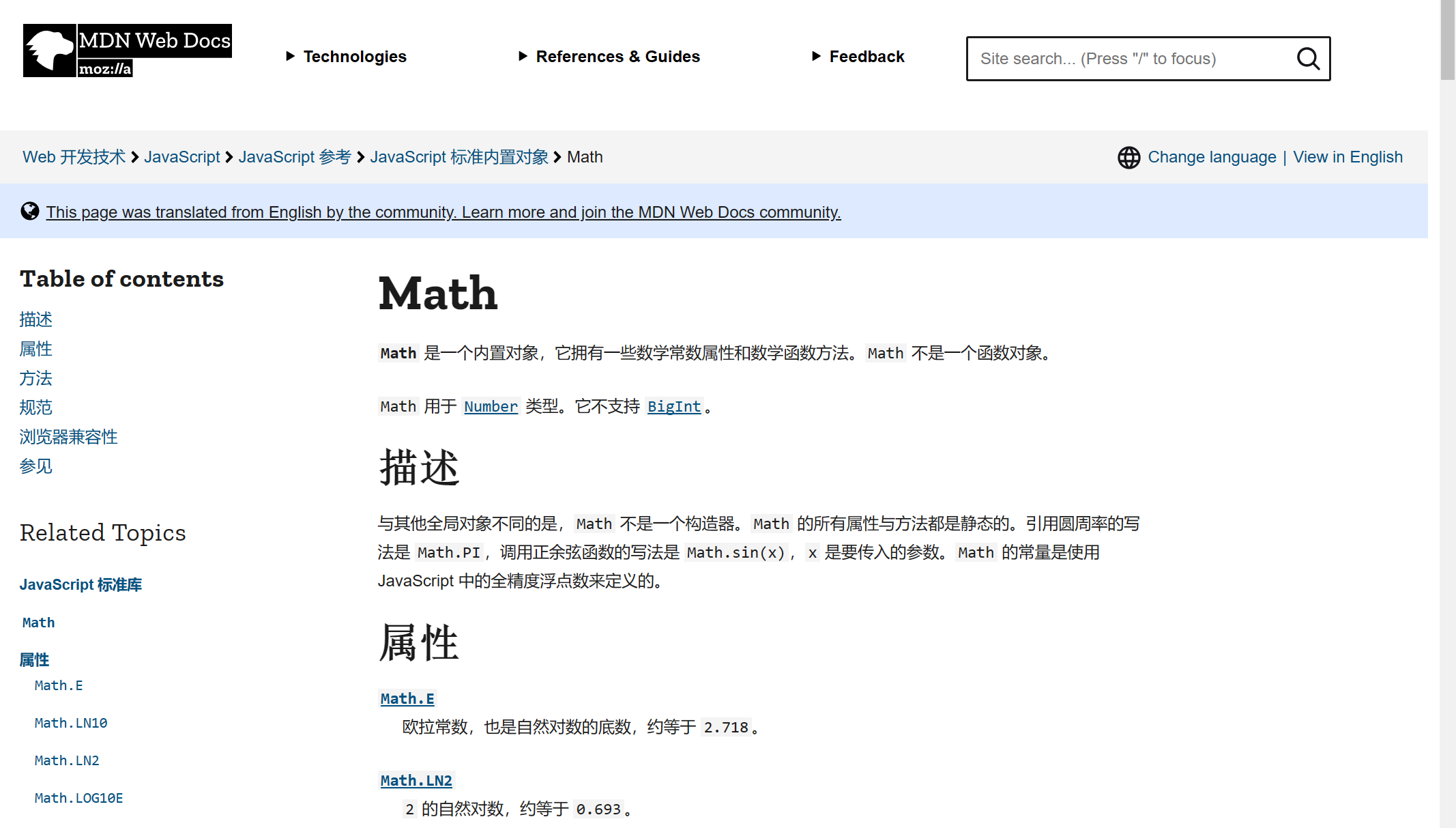
封装自己的数学对象
里面有PI 最大值和最小值
<script>
var myMath = {
PI:3.141592653589,
max:function() {
var max = arguments[0];
for(var i = 1;i < arguments.length;i++){
if(arguments[i] > max){
max = arguments[i];
}
}
return max;
},
min:function(){
var min = arguments[0];
for(var i = 1;i < arguments.length;i++){
if(arguments[i] < min){
min = arguments[i];
}
}
return min;
}
}
console.log(myMath.PI);
console.log(myMath.max(2,6,5,3));
console.log(myMath.min(2,6,5,3));
</script>
math绝对值和取整
绝对值方法
<script>
console.log(Math.abs[1]);
console.log(Math.abs[-1]);
console.log(Math.abs['-1']);
</script>
三个都是输出1 第三个隐式转换,,把字符型-1转换为数字型
取整方法
<script>
console.log(Math.floor(1.1));
console.log(Math.ceil(1.1));
console.log(Math.round(1.1));
console.log(Math.round(1.5));
</script>
Math.round(-1.5)结果是-1 其他数字都是四舍五入,只有.5是往大了取
math随机数
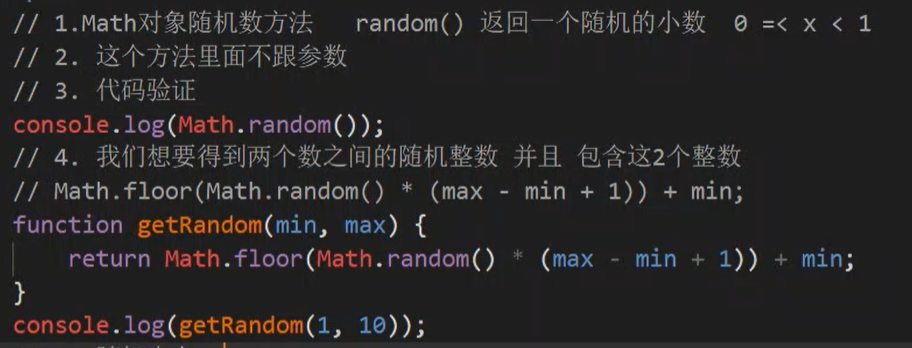  4可以换成arr.length - 1
猜数字游戏
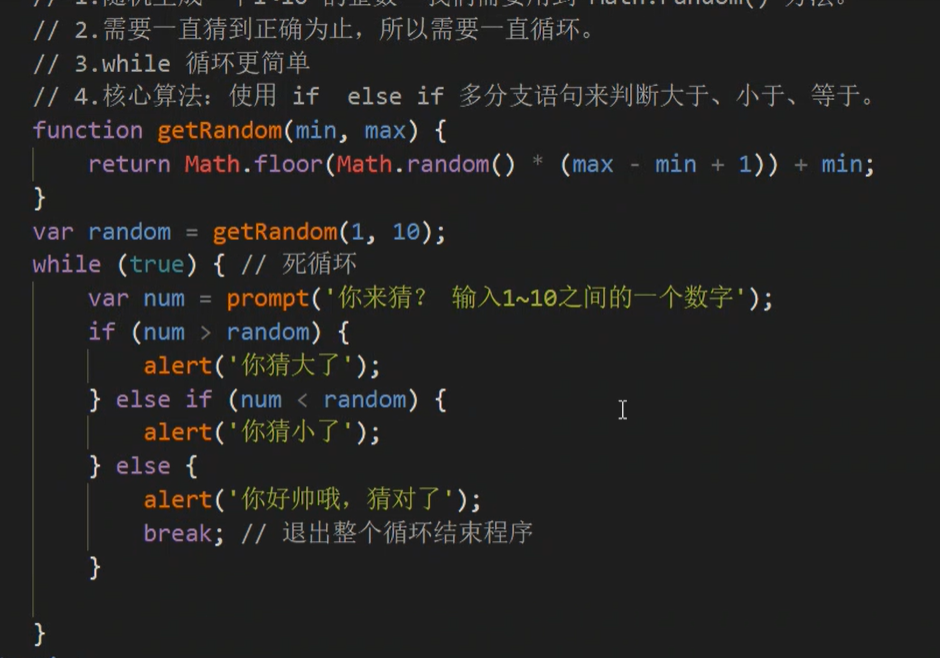
Date日期对象的使用
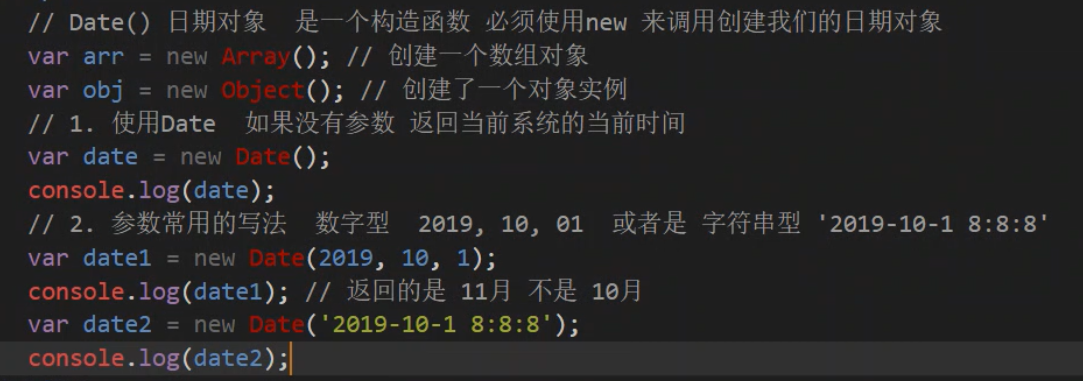 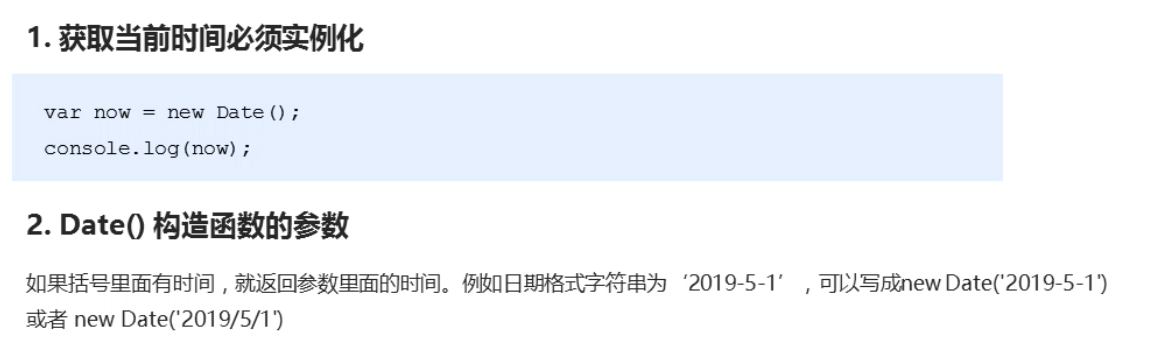
格式化日期
年月日星期
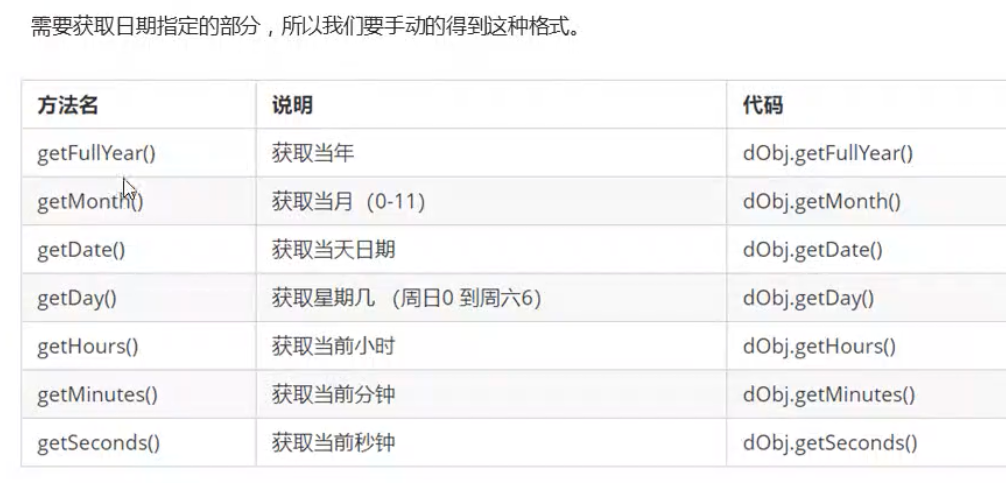
<script>
var date = new Date();
console.log(date.getFullYear());
console.log(date.getMonth() + 1);
console.log(date.getDate());
console.log(date.getDay());
</script>
<script>
var date = new Date();
var year = date.getFullYear();
var month = date.getMonth() + 1;
var date = date.getDate();
var arr = ['星期日','星期一','星期二','星期三·','星期四','星期五','星期六'];
var day = date.getDay();
console.log('今天是:'+ year + '年' + month + '月' + date + '日 ' + arr[day]);
</script>
时分秒
<script>
var date = new Date();
console.log(date.getHours());
console.log(date.getMinutes());
console.log(date.getSeconds());
function getTime(){
var time = new Date();
var h = time.getHours();
h = h < 10 ? '0' + h : h;
var m = time.getMinutes();
m = m < 10 ? '0' + m : m;
var s = time.getSeconds();
s = s < 10 ? '0' + s : s;
return h + ':' + m + ':' + s;
}
console.log(getTime());
</script>
Date总的毫秒(时间戳)
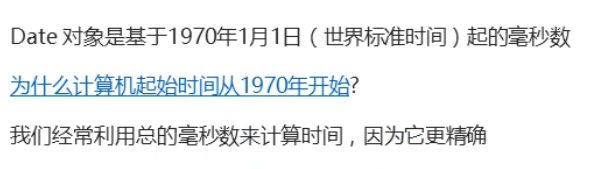 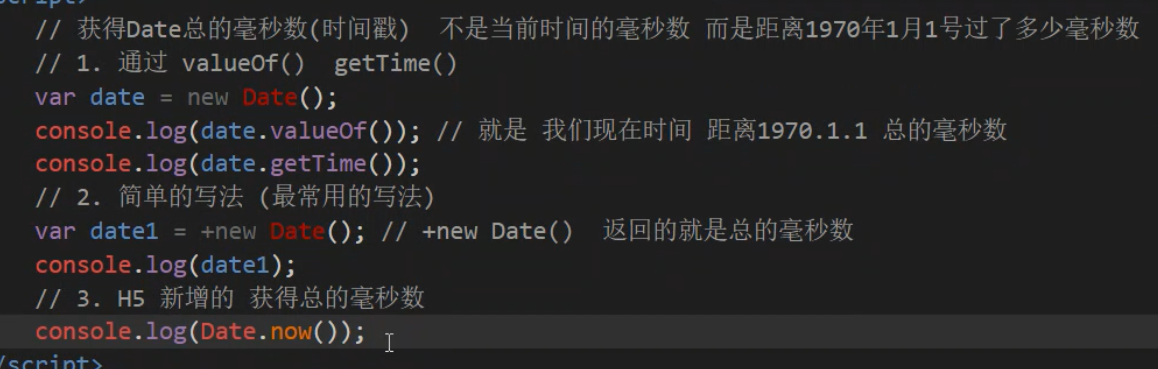
倒计时
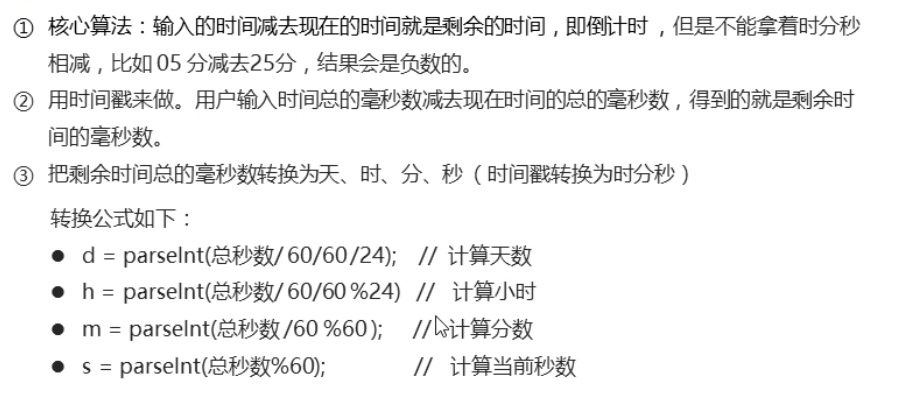
<script>
function countDown(time){
var nowTime = +new Date();
var inputTime = +new Date(time);
var times = (inputTime - nowTime) / 1000;
var d = parseInt(times / 60 / 60 / 24);
d = d < 10 ? '0'+ d : d;
var h = parseInt(times / 60 / 60 % 24);
h = h < 10 ? '0'+ h : h;
var m = parseInt(times / 60 % 60);
m = m < 10 ? '0'+ m : m;
var s = parseInt(times % 60);
s = s < 10 ? '0'+ s : s;
return d + '天' + h + '时' + m + '分' + s +'秒';
}
console.log(countDown('2021-8-29 22:00:00'));
</script>
|