效果: 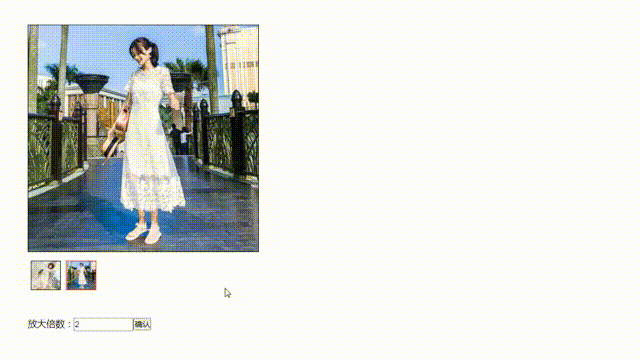 可对图片进行放大,可以自己随意放大倍数,无限制。 **
兄弟们都懂可以用来干什么把!
** 自己复制代码时,记得改成自己的图片路径和js路径。 完整代码如下: enlarge.html 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Enlarge</title>
<style>
*{margin: 0;padding: 0;}
.box{
width: 402px;
margin: 50px;
position: relative;
}
.middleBox{
width: 400px;
height: 400px;
border: 1px solid #000;
position: relative;
}
.middleBox>img{
width: 400px;
height: 400px;
}
.middleBox>.shade{
width: 200px;
height: 200px;
background-color: green;
opacity: .5;
position: absolute;
top: 0;
left: 0;
display: none;
}
.middleBox>.shade{
cursor: none;
}
.smallBox{
margin-top: 15px;
}
.smallBox>img{
width: 50px;
height: 50px;
border: 1px solid #000;
margin-left: 5px;
}
.smallBox>img.active{
border-color: red;
}
.bigBox{
width: 400px;
height: 400px;
border: 1px solid #000;
position: absolute;
top: 0;
left: 105%;
background-image: url(./images/big1.jpg);
background-repeat: no-repeat;
background-position: 0 0;
background-size: 800px 800px;
display: none;
}
input{
width: 100px;
height: 20px;
}
</style>
</head>
<body>
<div class="box">
<div class="middleBox">
<img src="./images/middle1.jpg">
<div class="shade"></div>
</div>
<div class="smallBox">
<img src="./images/small1.jpg" middleImg='./images/middle1.jpg' bigImg='./images/big1.jpg' class="active">
<img src="./images/small2.jpg" middleImg='./images/middle2.jpg' bigImg='./images/big2.jpg'>
</div>
<div class="bigBox"></div>
<br>
<br>
放大倍数:<input type="text" value="1"><button>确认</button>
</div>
</body>
</html>
<script src="./enlarge.js"></script>
js代码 enlarge.js
function Enlarge() {
this.box = document.querySelector('.box');
this.middleBox = document.querySelector('.middleBox');
this.middleImg = document.querySelector('.middleBox > img');
this.shade = document.querySelector('.shade');
this.bigBox = document.querySelector('.bigBox');
this.smallImgs = document.querySelectorAll('.smallBox > img');
this.input = document.querySelector('input')
this.button = document.querySelector('button')
}
Enlarge.prototype.bind = function () {
this.bigBox.style.backgroundSize = (Math.sqrt(this.input.value)*this.middleBox.clientWidth) + 'px' + ' ' + (Math.sqrt(this.input.value)*this.middleBox.clientWidth) + 'px'
for (let i = 0; i < this.smallImgs.length; i++) {
this.smallImgs[i].onclick = () => {
this.tab(this.smallImgs[i]);
}
}
this.middleBox.onmouseenter = () => {
let a = getStyle(this.bigBox, 'background-size');
let b = parseInt(a.split(' ')[0]);
this.shade.style.width = (this.middleBox.clientWidth * this.middleBox.clientWidth) / b + 'px'
this.shade.style.height = (this.middleBox.clientWidth * this.middleBox.clientWidth) / b + 'px'
this.shade.style.display = 'block';
this.bigBox.style.display = 'block';
this.middleBox.onmousemove = (e) => {
e = e || window.event;
this.move(e)
}
}
this.middleBox.onmouseleave = () => {
this.shade.style.display = 'none';
this.bigBox.style.display = 'none';
this.middleBox.onmousemove = null;
this.bigBox.style.backgroundPosition = `0px 0px`;
}
this.button.onclick = () => {
enlarge.bind()
}
}
Enlarge.prototype.tab = function (ele) {
for (let i = 0; i < this.smallImgs.length; i++) {
this.smallImgs[i].className = '';
}
ele.className = 'active';
let bigImg = ele.getAttribute('bigImg');
let middleImg = ele.getAttribute('middleImg');
this.middleImg.setAttribute('src', middleImg);
this.bigBox.style.backgroundImage = `url(${bigImg})`;
}
Enlarge.prototype.move = function (e) {
let x = e.pageX;
let y = e.pageY;
let shadeWidthBan = this.shade.clientWidth / 2;
let shadeHeightBan = this.shade.clientHeight / 2;
let boxLeft = this.box.offsetLeft;
let boxTop = this.box.offsetTop;
if (x < shadeWidthBan + boxLeft) {
x = shadeWidthBan + boxLeft;
}
if (y < shadeHeightBan + boxTop) {
y = shadeHeightBan + boxTop;
}
if (x > boxLeft + this.middleBox.clientWidth - shadeWidthBan) {
x = boxLeft + this.middleBox.clientWidth - shadeWidthBan;
}
if (y > boxTop + this.middleBox.clientHeight - shadeHeightBan) {
y = boxTop + this.middleBox.clientHeight - shadeHeightBan;
}
this.shade.style.left = x - boxLeft - shadeWidthBan + 'px';
this.shade.style.top = y - boxTop - shadeHeightBan + 'px';
this.bigImgMove()
}
Enlarge.prototype.bigImgMove = function () {
let shadeX = parseInt(getStyle(this.shade, 'left'));
let shadeY = parseInt(getStyle(this.shade, 'top'));
let bigImgSize = getStyle(this.bigBox, 'background-size');
let bigImgWidth = parseInt(bigImgSize.split(' ')[0]);
let bigImgHeight = parseInt(bigImgSize.split(' ')[1]);
let xPercent = shadeX / (this.middleBox.clientWidth - this.shade.clientWidth);
let yPercent = shadeY / (this.middleBox.clientHeight - this.shade.clientHeight);
let bigImgMoveX = (bigImgWidth - this.middleBox.clientWidth) * xPercent;
let bigImgMoveY = (bigImgHeight - this.middleBox.clientHeight) * yPercent;
this.bigBox.style.backgroundPosition = `-${bigImgMoveX}px -${bigImgMoveY}px`;
}
let enlarge = new Enlarge();
enlarge.bind()
function getStyle(ele, style) {
try {
return window.getComputedStyle(ele)[style];
} catch (error) {
return ele.currentStyle[style];
}
}
|