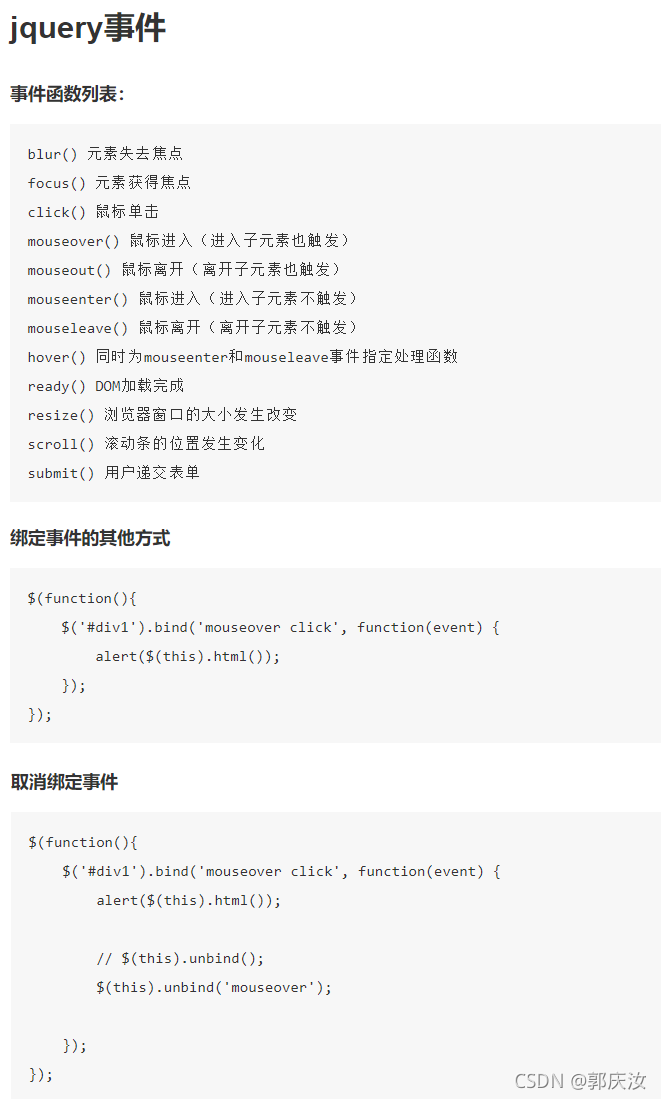
1、输入框获取焦点与失去焦点事件
blur() 元素失去焦点、focus() 元素获得焦点 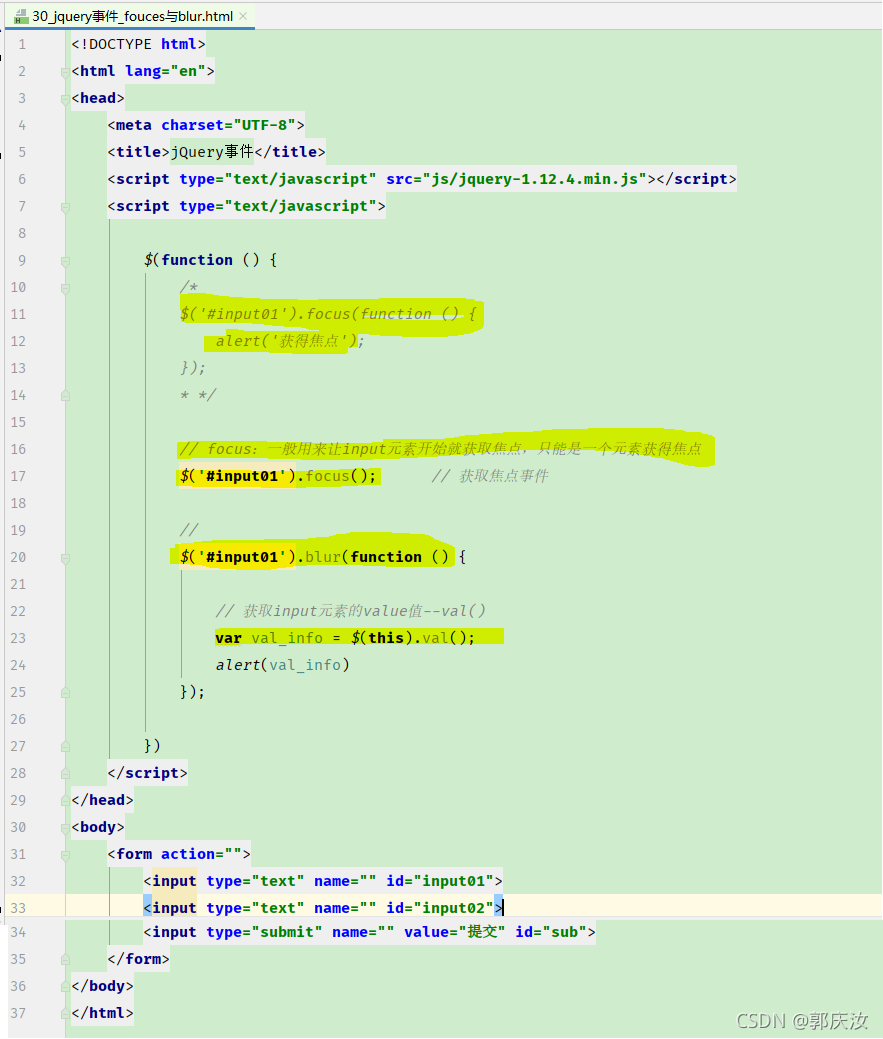
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>jQuery事件</title>
<script type="text/javascript" src="js/jquery-1.12.4.min.js"></script>
<script type="text/javascript">
$(function () {
$('#input01').focus();
$('#input01').blur(function () {
var val_info = $(this).val();
alert(val_info)
});
})
</script>
</head>
<body>
<form action="">
<input type="text" name="" id="input01">
<input type="text" name="" id="input02">
<input type="submit" name="" value="提交" id="sub">
</form>
</body>
</html>
2、鼠标移入与移出事件
mouseover() 鼠标进入(进入子元素也触发)、mouseout() 鼠标离开(离开子元素也触发) mouseenter() 鼠标进入(进入子元素不触发)、mouseleave() 鼠标离开(离开子元素不触发)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>鼠标移入移出事件(mouseover与mouseout)</title>
<script type="text/javascript" src="js/jquery-1.12.4.min.js"></script>
<script type="text/javascript">
$(function () {
$('.con,box').mouseover(function () {
alert("鼠标移入啦");
});
$('.con,box').mouseout(function () {
alert("鼠标移出啦")
});
$('.con1,box1').mouseenter(function () {
alert('mouseenter移入')
});
$('.con1,box1').mouseleave(function () {
alert('mouseleave移出')
});
})
</script>
<style type="text/css">
.con{
width: 200px;
height: 200px;
background-color: gold;
}
.box{
width: 100px;
height: 100px;
background-color: green;
}
.con1{
width: 200px;
height: 200px;
background-color: gold;
}
.box1{
width: 100px;
height: 100px;
background-color: green;
}
</style>
</head>
<body>
<div class="con">
<div class="box"></div>
</div>
<br>
<br>
<br>
<div class="con1">
<div class="box1"></div>
</div>
</body>
</html>
3、hover() 同时为mouseenter和mouseleave事件指定处理函数
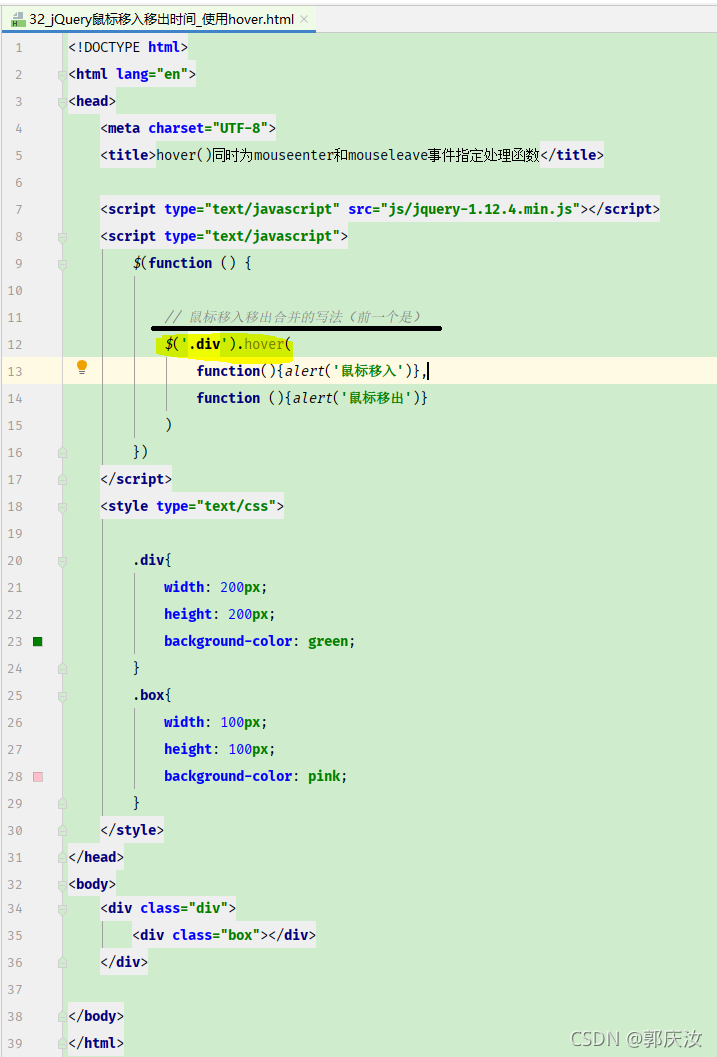
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>hover()同时为mouseenter和mouseleave事件指定处理函数</title>
<script type="text/javascript" src="js/jquery-1.12.4.min.js"></script>
<script type="text/javascript">
$(function () {
$('.div').hover(
function(){alert('鼠标移入')},
function (){alert('鼠标移出')}
)
})
</script>
<style type="text/css">
.div{
width: 200px;
height: 200px;
background-color: green;
}
.box{
width: 100px;
height: 100px;
background-color: pink;
}
</style>
</head>
<body>
<div class="div">
<div class="box"></div>
</div>
</body>
</html>
4、submit() 用户递交表单
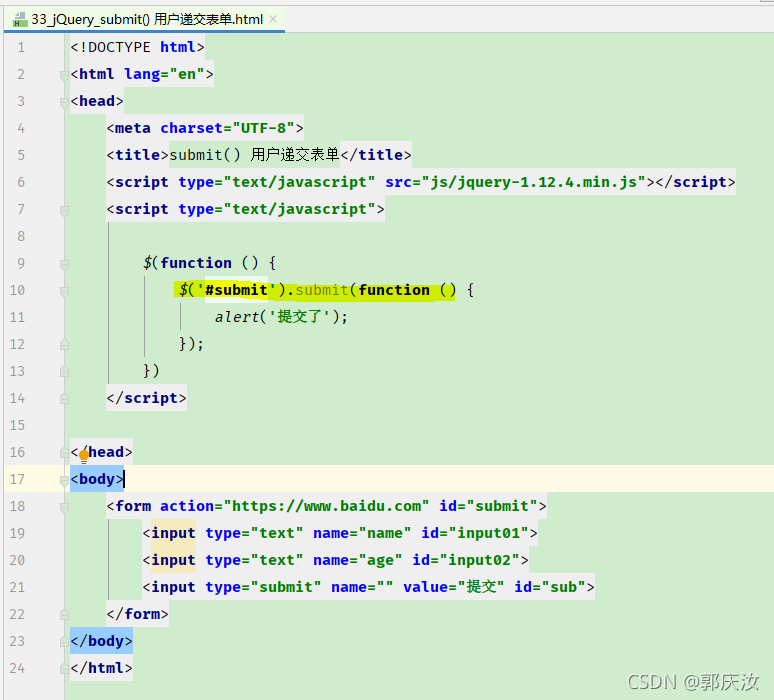 代码如下所示:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>submit() 用户递交表单</title>
<script type="text/javascript" src="js/jquery-1.12.4.min.js"></script>
<script type="text/javascript">
$(function () {
$('#submit').submit(function () {
alert('提交了');
});
})
</script>
</head>
<body>
<form action="https://www.baidu.com" id="submit">
<input type="text" name="name" id="input01">
<input type="text" name="age" id="input02">
<input type="submit" name="" value="提交" id="sub">
</form>
</body>
</html>
显示效果如下所示: 
5、resize() 浏览器窗口的大小发生改变
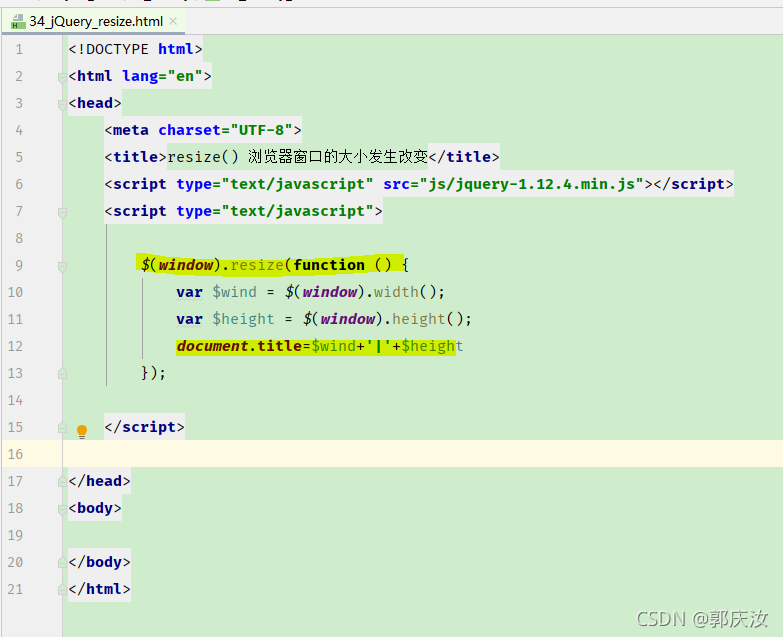 代码如下所示:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>resize() 浏览器窗口的大小发生改变</title>
<script type="text/javascript" src="js/jquery-1.12.4.min.js"></script>
<script type="text/javascript">
$(window).resize(function () {
var $wind = $(window).width();
var $height = $(window).height();
document.title=$wind+'|'+$height
});
</script>
</head>
<body>
</body>
</html>
显示效果如下: 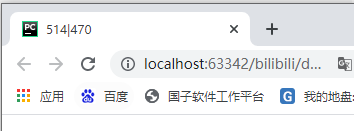
6、bind绑定事件和解绑
事件绑定:
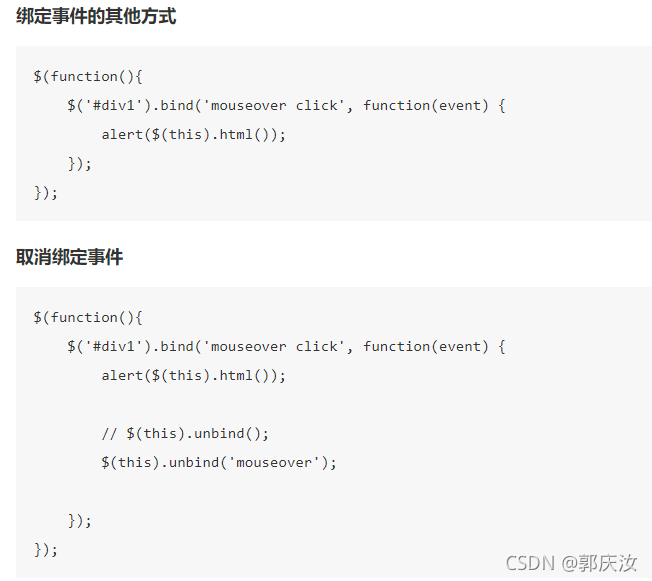 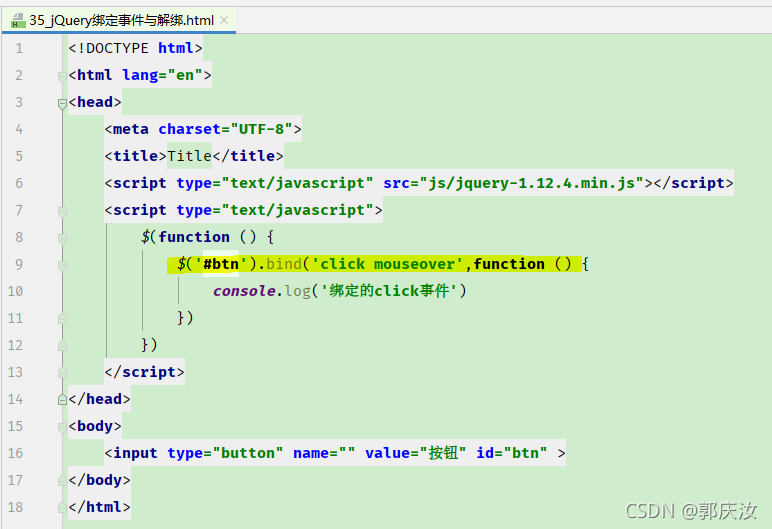 代码如下所示:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script type="text/javascript" src="js/jquery-1.12.4.min.js"></script>
<script type="text/javascript">
$(function () {
$('#btn').bind('click mouseover',function () {
console.log('绑定的click事件')
})
})
</script>
</head>
<body>
<input type="button" name="" value="按钮" id="btn" >
</body>
</html>
显示效果如下所示: 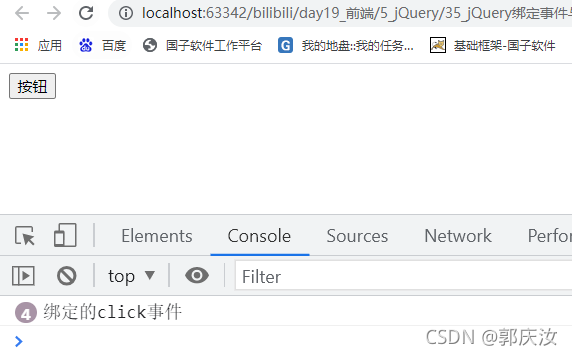
事件解绑:
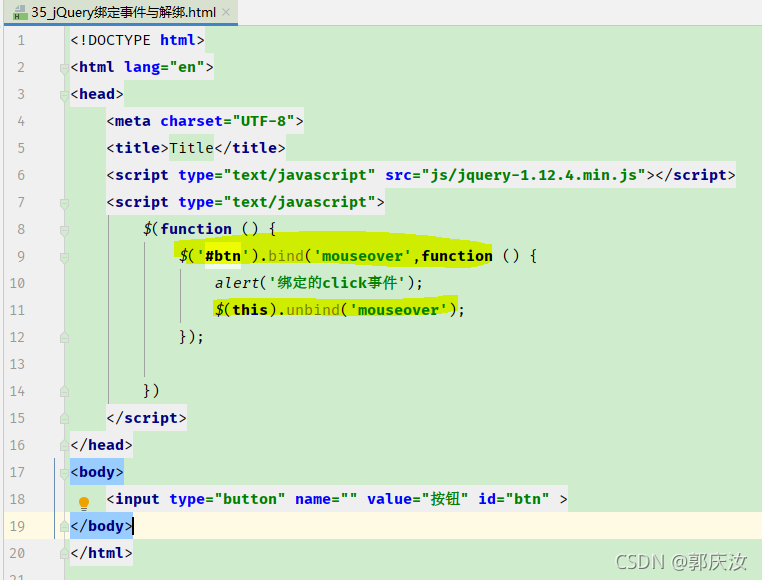
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script type="text/javascript" src="js/jquery-1.12.4.min.js"></script>
<script type="text/javascript">
$(function () {
$('#btn').bind('mouseover',function () {
alert('绑定的click事件');
$(this).unbind('mouseover');
});
})
</script>
</head>
<body>
<input type="button" name="" value="按钮" id="btn" >
</body>
</html>
|