一、原生js实现轮播图
(1)实现功能
- 1.能够自动平滑的循环轮播。
- 2.点击导航圆点控制轮播。
(2)html示例代码
<div id="outer">
<ul id="imgList">
<li>
<img src="img/1.webp" alt="壁纸">
</li>
<li>
<img src="img/2.webp" alt="壁纸">
</li>
<li>
<img src="img/3.webp" alt="壁纸">
</li>
<li>
<img src="img/4.webp" alt="壁纸">
</li>
<li>
<img src="img/5.webp" alt="壁纸">
</li>
<li>
<img src="img/6.webp" alt="壁纸">
</li>
<li>
<img src="img/1.webp" alt="壁纸">
</li>
</ul>
<div id="navDiv">
<a href="javascript:;"></a>
<a href="javascript:;"></a>
<a href="javascript:;"></a>
<a href="javascript:;"></a>
<a href="javascript:;"></a>
<a href="javascript:;"></a>
</div>
</div>
(3)css示例代码
<style type="text/css">
*{
margin:0;
padding:0;
list-style:none;
}
body {
-moz-user-select: none;
-webkit-user-select: none;
-ms-user-select: none;
-khtml-user-select: none;
user-select: none;
}
#outer{
width:533px;
height:300px;
background-color:skyblue;
margin:50px auto;
padding:10px 0;
position:relative;
overflow:hidden;
}
#imgList{
height: 300px;
position:absolute;
}
#imgList li{
float:left;
}
#navDiv{
position:absolute;
bottom:20px;
}
#navDiv a{
float:left;
width:20px;
height:20px;
margin:0 10px;
border-radius:50%;
background-color:rgba(187, 255, 170,.3);
}
#navDiv a:hover{
background-color: skyblue;
}
</style>
(3)js示例代码
<!-- 引入自定义js动画工具 -->
<script type="text/javascript" src="js/tools.js"></script>
<script type="text/javascript">
window.onload = function(){
var imgList = document.getElementById("imgList");
var imgArr = document.getElementsByTagName("img");
imgList.style.width = 533*imgArr.length + "px";
var navDiv = document.getElementById("navDiv");
var outer = document.getElementById("outer");
navDiv.style.left = (outer.offsetWidth - navDiv.offsetWidth)/2 + "px";
var allA = document.getElementsByTagName("a");
var index = 0;
allA[index].style.backgroundColor = "skyblue";
for(var i=0;i<allA.length;i++){
allA[i].num = i;
allA[i].onclick = function(){
clearInterval(timer);
index = this.num;
setA();
move(imgList,"left",-533*index,10,function(){
autoChange();
});
};
}
autoChange();
function setA(){
if(index >= imgArr.length - 1){
index = 0;
imgList.style.left = 0;
}
for(var i=0;i<allA.length;i++){
allA[i].style.backgroundColor = "";
}
allA[index].style.backgroundColor = "skyblue";
}
var timer;
function autoChange(){
timer = setInterval(function(){
index++;
if(index>=imgArr.length){
index = 0;
}
move(imgList,"left",-533*index,10,function(){
setA();
});
},3000);
}
};
</script>
(4)说明
这里使用到了一个我自己封装的js工具库,有需要的可以去我的github主页下载。
自封装js工具库
(5)效果演示
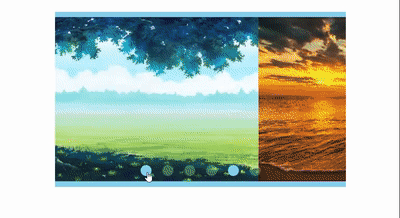
二、jQuery实现轮播图
(1)实现功能
- 1.点击向右(向左)的图标,平滑切换到下(上)一页
- 2.无限循环切换,第一页的上一页为最后一页,最后一页的下一页是第一页
- 3.每隔三秒自动滑动到下一页
- 4.当鼠标进入图片区域时,自动切换停止,当鼠标离开后,开始自动切换
- 5.切换页面时,下面的原点也同步更新
- 6.点击圆点图标切换到对应的页
- 7.解决快速点击出现空白bug
(2)html示例代码
<div class="wrapper">
<ul class="banner">
<li>
<img src="img/5.jpg" alt="">
</li>
<li>
<img src="img/1.jpg" alt="">
</li>
<li>
<img src="img/2.jpg" alt="">
</li>
<li>
<img src="img/3.jpg" alt="">
</li>
<li>
<img src="img/4.jpg" alt="">
</li>
<li>
<img src="img/5.jpg" alt="">
</li>
<li>
<img src="img/1.jpg" alt="">
</li>
</ul>
<div class="pointer">
<span class="display"></span>
<span></span>
<span></span>
<span></span>
<span></span>
</div>
<div class="last"><</div>
<div class="next">></div>
</div>
(3)css示例代码
<style type="text/css">
* {
margin: 0;
padding: 0;
list-style: none;
}
body {
-moz-user-select: none;
-webkit-user-select: none;
-ms-user-select: none;
-khtml-user-select: none;
user-select: none;
}
.wrapper{
width:1202px;
height:676px;
margin:0 auto;
position:relative;
overflow: hidden;
}
.banner {
height: 676px;
position: absolute;
font-size: 0;
}
.banner li {
float: left;
}
.pointer{
position:absolute;
bottom:20px;
}
.display{
background-color:red;
}
span{
display: block;
cursor: pointer;
width:30px;
height:30px;
margin:0 20px;
float:left;
background-color: skyblue;
border-radius: 50%;
}
.last,.next{
width:40px;
height:80px;
color:#fff;
cursor:pointer;
font:bolder 16px "楷体";
text-align: center;
line-height:80px;
position:absolute;
top:45%;
background-color: rgba(83, 82, 82, 0.3);
}
.last:hover,.next:hover{
color:skyblue;
font-size:30px;
background-color: rgba(83, 82, 82, 0.8);
}
.last{
left:0;
}
.next{
right:0;
}
</style>
(4)js示例代码
$(function () {
var $wrapper = $(".wrapper");
var $banner = $(".banner");
var $imgList = $(".banner > li");
var $pointer = $(".pointer");
var $pointerSpan = $(".pointer > span");
var $last = $(".last");
var $next = $(".next");
var $wrapperWidth = $wrapper.width();
var $pointerWidth = $pointer.width();
var $imgLength = $imgList.length;
var $pointerLength = $pointerSpan.length;
var index = 0;
var move = false;
var TIME = 600;
var ITEM_TIME = 20;
$banner.css({
width: $wrapperWidth * $imgLength,
left: -$wrapperWidth
});
$pointer.css({
left: ($wrapperWidth - $pointerWidth) / 2
});
$next.click(function () {
nextPage(true)
});
$last.click(function () {
nextPage(false)
});
var timer = setInterval(function () {
nextPage(true);
}, 3000);
$wrapper
.mouseenter(function () {
console.log("hello");
clearInterval(timer);
})
.mouseleave(function () {
timer = setInterval(function () {
nextPage(true);
}, 3000);
});
function updatePointer(flag) {
var targetIndex = 0;
if (typeof flag === "boolean") {
if (flag) {
targetIndex = index + 1;
if (targetIndex === $pointerLength) {
targetIndex = 0;
}
} else {
targetIndex = index - 1;
if (targetIndex === -1) {
targetIndex = $pointerLength - 1;
}
}
}else {
targetIndex = flag;
}
$pointerSpan.eq(index).removeClass("display");
$pointerSpan.eq(targetIndex).addClass("display");
index = targetIndex;
}
$pointerSpan.click(function () {
var targetIndex =$(this).index();
if(targetIndex != index){
nextPage(targetIndex);
}
});
function nextPage(flag) {
if(move){
return;
}
move = true;
var offset = 0;
if (typeof flag === "boolean") {
offset = flag ? -$wrapper.width() : $wrapper.width();
} else {
offset = -(flag - index) * $wrapperWidth;
}
var itemOffset = offset / (TIME / ITEM_TIME);
var currLeft = $banner.position().left;
var targetLeft = currLeft + offset;
var timer = setInterval(function () {
currLeft += itemOffset;
if (Math.round(currLeft) === Math.round(targetLeft)) {
clearInterval(timer);
move =false;
if (Math.round(currLeft) === Math.round(-($pointerLength + 1) * $wrapperWidth)) {
currLeft = Math.round(-$wrapperWidth);
} else if (Math.round(currLeft) === 0) {
currLeft = Math.round(-$pointerLength * $wrapperWidth);
}
}
$banner.css({
left: currLeft
});
}, ITEM_TIME);
updatePointer(flag);
}
});
(5)效果展示
??视频在线展示
三、最后要说的话
轮播图是网页中必不可少的一种功能,希望这个例子能对大家有所帮助。
|