1、vuex4简介
全局状态管理 可以使用组合式API(compose API) 单向数据流理念 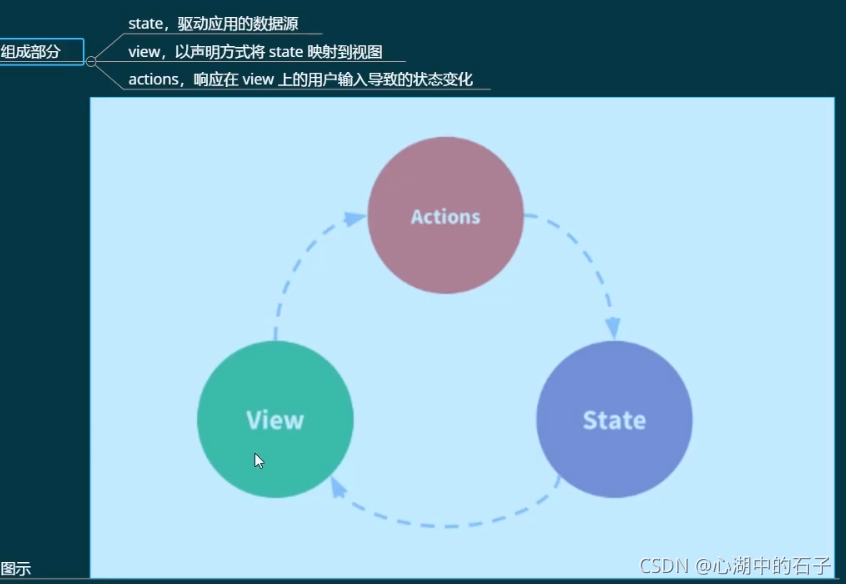 vuex把组件共享状态抽取出来,以一个全局单例模式管理,在这种模式下,项目中的组件树构成了一个巨大的‘视图’,不管在树的哪个位置,任何组件都能够获取状态或触发行为。
vuex是什么 vuex是专为vue.js应用程序开发的状态管理模式,她采用集中式存储管理应用的所有组件的状态,并以响应的规则保证状态以一种可预测的方式发生变化 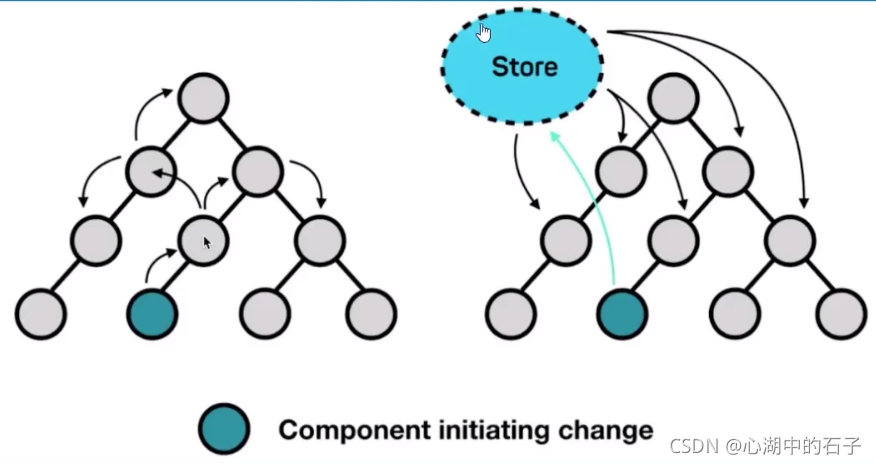 一句话表述:
vuex相当于一个数据银行,对vue应用中多个组件的共享状态进行集中式的管理(读/写) 但是,如果不打算开发大型单页应用,使用vuex可能是繁琐荣誉的 大型SPA(单页面应用)项目存在多个状态在多个界面共享的问题 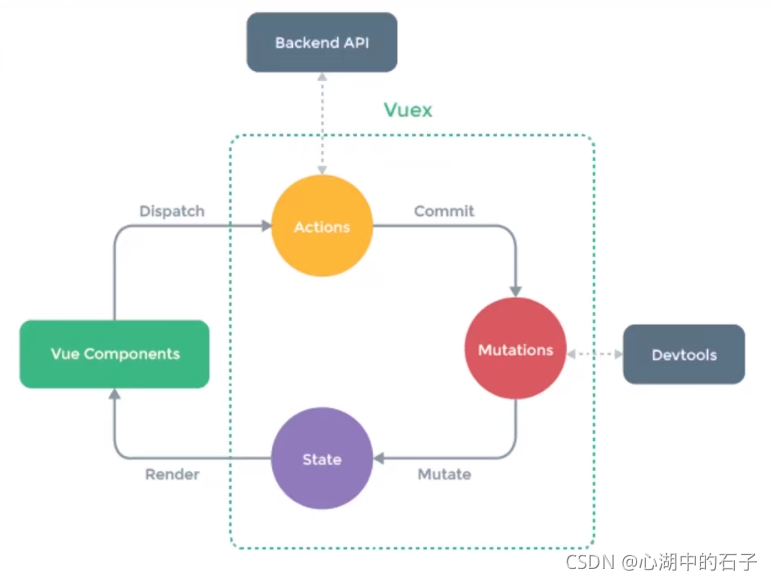
2、vuex4集成
vuex的组成部分 state getters mutations actions mapState mapActions mapMutations Modules
3、vuex4获取和共享状态
先安装vuex4
npm install vuex@next --save
创建src/store/index.js index.js
import {createStore} from 'vuex'
const store=createStore({
state:{
counter:0
},
getters:{
},
mutations:{
},
actions:{
}
})
export default store
main.js中引入这个index.js main.js
import { createApp } from 'vue'
import App from './App.vue'
import store from './store/index.js'
const app=createApp(App);
app.use(store)
app.mount('#app')
app.vue
<template>
<div>
<h3>首页的状态:{{$store.state.counter}}</h3>
<hello-world></hello-world>
</div>
</template>
<script >
export default {
components:{
HelloWorld
},
setup(){
}
}
</script>
<style>
</style>
4、vuex4数据多页面共享
src/store/index.js
import {createStore} from 'vuex'
const store=createStore({
state:{
counter:0
},
getters:{
},
mutations:{
},
actions:{
}
})
export default store
main.js
import { createApp } from 'vue'
import App from './App.vue'
import store from './store/index.js'
const app=createApp(App);
app.use(store)
app.mount('#app')
src/components/Helloworld.vue
<template>
<div>
<p>********其他页面******</p>
<h3>其他页面{{$store.state.counter}}</h3>
<p>********其他页面*****</p>
hello world
</div>
</template>
<script setup>
import {defineProps,reactive} from 'vue'
defineProps({
msg:String
})
const state=reactive({count:0})
</script>
<style scoped>
</style>
App.vue
<template>
<div>
<h3>首页的状态:{{$store.state.counter}}</h3>
<hello-world></hello-world>
</div>
</template>
<script >
import HelloWorld from './Components/HelloWorld.vue'
export default {
components:{
HelloWorld
},
setup(){
}
}
</script>
<style>
</style>
运行结果 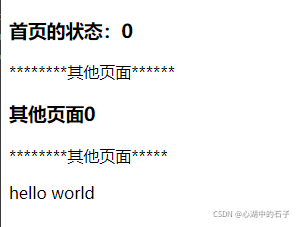
5、vuex4修改共享状态
实际要经过一下流程,组件中的具体方法dispath一个actions里的小写方法名(是个名字就可以),再从actions里的小写方法名commit一个mutations的大写方法名,在mutations的大写方法中修改state状态里面的具体变量 实际流程如下: 组件界面(按钮触发动作函数(回调函数)) ↓ dispatch一个actions的方法(小写方法名) ↓ commit一个mutations的方法(大写方法名) ↓ mutations的方法修改state状态内的具体变量
代码如下: index.js
import {createStore} from 'vuex'
const store=createStore({
state:{
counter:0
},
getters:{
},
mutations:{
INCREMENT(state){
state.counter++
},
DECREMENT(state){
state.counter--
}
},
actions:{
increment({commit}){
commit('INCREMENT')
},
decrement({commit}){
commit('DECREMENT')
}
}
})
export default store
App.vue
<template>
<div>
<h3>首页的状态:{{$store.state.counter}}</h3>
<hello-world></hello-world>
<button @click="add()">加一</button>
<button @click="jian()">减一</button>
</div>
</template>
<script >
import HelloWorld from './Components/HelloWorld.vue'
import {useStore} from 'vuex'
export default {
components:{
HelloWorld
},
setup(){
const store=useStore()
const add=()=>{
store.commit('INCREMENT')
}
const jian=()=>{
store.commit('DECREMENT')
}
return {
add,
jian
}
}
}
</script>
<style>
</style>
6、vuex4异步状态
src/store/index.js index.js
<template>
<div>
<h3>首页的状态:{{$store.state.counter}}</h3>
<hello-world></hello-world>
<button @click="add()">加一</button>
<button @click="jian()">减一</button>
<button @click="add1999(1999)">两秒异步加1999</button>
</div>
</template>
<script >
import HelloWorld from './Components/HelloWorld.vue'
import {useStore} from 'vuex'
export default {
components:{
HelloWorld
},
setup(){
const store=useStore()
const add=()=>{
store.dispatch('increment')
}
const jian=()=>{
store.dispatch('decrement')
}
const add1999=(num)=>{
store.dispatch('increment_1999',num)
}
return {
add,
jian,
add1999
}
}
}
</script>
<style>
</style>
App.vue
<template>
<div>
<h3>首页的状态:{{$store.state.counter}}</h3>
<hello-world></hello-world>
<button @click="add()">加一</button>
<button @click="jian()">减一</button>
<button @click="add1999(1999)">两秒异步加1999</button>
</div>
</template>
<script >
import HelloWorld from './Components/HelloWorld.vue'
import {useStore} from 'vuex'
export default {
components:{
HelloWorld
},
setup(){
const store=useStore()
const add=()=>{
store.dispatch('increment')
}
const jian=()=>{
store.dispatch('decrement')
}
const add1999=(num)=>{
store.dispatch('increment_1999',num)
}
return {
add,
jian,
add1999
}
}
}
</script>
<style>
</style>
运行结果: 两秒后加1999 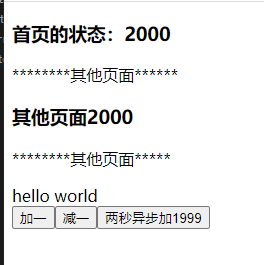
7、vuex4改进用法
上例的index.js文件不变 App.vue
<template>
<div>
<h3>首页的状态:{{counter}}</h3>
<hello-world></hello-world>
<button @click="increment()">加一</button>
<button @click="decrement()">减一</button>
<button @click="increment_1999(1999)">两秒异步加1999</button>
</div>
</template>
<script >
import HelloWorld from './Components/HelloWorld.vue'
import {useStore} from 'vuex'
import {computed} from 'vue'
export default {
components:{
HelloWorld
},
setup(){
const store=useStore()
return {
counter:computed(()=>store.state.counter),
increment:()=>store.dispatch('increment'),
decrement:()=>store.dispatch('decrement'),
increment_1999:(num)=>store.dispatch('increment_1999',num),
}
}
}
</script>
<style>
</style>
8、vuex4日历案例
略
9、vuex4模块分割
解决文件对象过大的问题 略 完结
|