很多时候我们需要改变父组件向子组件传递的props,但是这个数据流是单向的。要想使父子之间props双向绑定就要借助自定义事件。下面来举个例子:
子组件中有一个盒子和一个按钮,父组件通过变量visible控制子组件是否显示,同时子组件现在想要通过按钮来改变父组件中visible的值,从而控制子组件的显示与否。
<template>
<div>
<Zi v-show="visible" :visible="visible" @update="update($event)"/>
</div>
</template>
<script>
import Zi from "./Zi";
export default {
name: "Fu",
data() {
return {
visible:true
}
},
components: {
Zi,
},
methods: {
update($event){
console.log($event);
}
},
};
</script>
<template>
<div>
<div class="box"></div>
<br />
<button @click="update">切换</button>
</div>
</template>
<script>
export default {
name: "Zi",
props: ["visible"],
methods: {
update() {
this.$emit("update", !this.visible);
},
},
};
</script>
<style lang="css" scoped>
.box {
width: 100px;
height: 100px;
background: cornflowerblue;
}
</style>
点一下按钮测试一下,可以接收到子组件传递过来的值
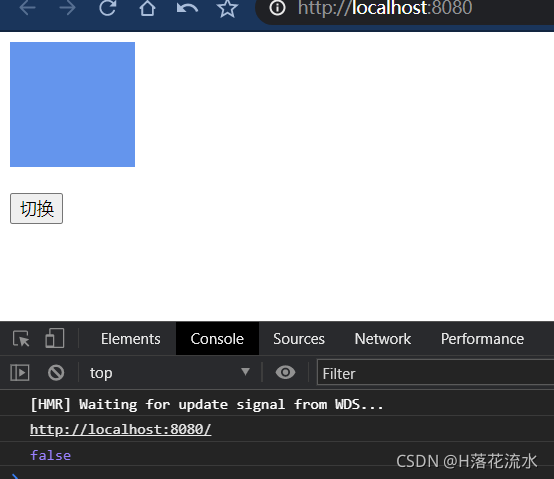 接下来将子组件传过来的值赋值给父组件的visible,并简化一下代码
<template>
<div>
<Zi v-show="visible" :visible="visible" @update="visible = $event" />
</div>
</template>
<script>
import Zi from "./Zi";
export default {
name: "Fu",
data() {
return {
visible: true,
};
},
components: {
Zi,
},
};
</script>
此时已完成了props双向绑定,针对这种情况,vue利用.sync修饰符做了简化。此时代码可修改为
<template>
<div>
<Zi v-show="visible" :visible.sync="visible"></Zi>
</div>
</template>
<script>
import Zi from "./Zi";
export default {
name: "Fu",
data() {
return {
visible: true,
};
},
components: {
Zi,
},
};
</script>
<template>
<div>
<div class="box"></div>
<br />
<button @click="update">切换</button>
</div>
</template>
<script>
export default {
name: "Zi",
props: ["visible"],
methods: {
update() {
this.$emit("update:visible", !this.visible);
},
},
};
</script>
|