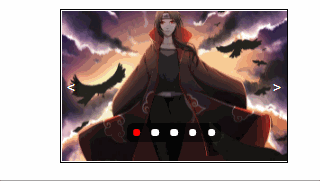
<div class="banner">
<ul></ul>
<ol></ol>
<div>
<a href="JavaScript:;" name="left"><</a>
<a href="JavaScript:;" name="right">></a>
</div>
</div>
* {
margin: 0;
padding: 0;
}
ul,
ol,
li {
list-style: none;
}
a,
a:hover,
a:active {
text-decoration: none;
}
img {
width: 100%;
height: 100%;
display: block;
}
.banner {
width: 600px;
height: 400px;
border: 5px solid #000;
position: relative;
margin: 50px auto;
overflow: hidden;
}
.banner>ul {
width: 500%;
height: 100%;
position: absolute;
top: 0;
left: 0;
}
.banner>ul>li {
float: left;
width: 600px;
height: 400px;
}
.banner>ol {
height: 50px;
position: absolute;
bottom: 50px;
left: 50%;
transform: translateX(-50%);
background: rgba(0, 0, 0, 0.5);
display: flex;
justify-content: center;
align-items: center;
border-radius: 15px;
}
.banner>ol>li {
width: 20px;
height: 20px;
border-radius: 50%;
background: #fff;
margin: 0 15px;
cursor: pointer;
}
.banner>ol>li.active {
background: red;
}
.banner>div {
width: 100%;
height: 50px;
position: absolute;
left: 0;
top: 50%;
transform: translateY(-50%);
display: flex;
justify-content: space-between;
align-items: center;
}
.banner>div>a {
display: flex;
justify-content: center;
align-items: center;
width: 50px;
height: 50px;
font-size: 40px;
color: #fff;
}
动态生成轮播图页面
当前我们自己定义一个数组 模拟一下
2, 根据数组动态生成页面内容生成 ul>li ol>li
定义变量 存储 ul>li字符串 ol>li字符串
循环遍历数组 每一个数组单元 对应一个 ul>li ol>li
第一个ol>li 有 class,active样式
将 ul>li字符串 ol>li字符串写入 ul ol 标签对象
获取 所有的 ul>li
克隆所有ul>li中的第一个li标签
克隆所有ul>li中的最后一个li标签
克隆的第一个ul>li标签写入到 ul标签的最后
克隆的最后一个ul>li标签写入到 ul标签的第一个
获取 ul>li 宽度
重新设定ul标签的宽度
(原始数组单元个数+2) * 一个li宽度 + 'px'
重新定位ul标签
向左定位一个 li宽度
function setPage() {
let ulStr = '';
let olStr = '';
bannerArr.forEach(function (item, key) {
ulStr += `<li><img src="./images/${item.name}"></li>`;
olStr += key === 0 ? `<li class="active" name="focus" num="${key}"></li>` :
`<li name="focus" num="${key}"></li>`;
})
oUl.innerHTML = ulStr;
oOl.innerHTML = olStr;
oUlLis = oUl.querySelectorAll('li');
oOlLis = oOl.querySelectorAll('li');
const cloneFirst = oUlLis[0].cloneNode(true);
const cloneLast = oUlLis[oUlLis.length - 1].cloneNode(true);
oUl.appendChild(cloneFirst);
oUl.insertBefore(cloneLast, oUlLis[0]);
liWidth = oUlLis[0].offsetWidth;
oUl.style.width = (length + 2) * liWidth + 'px';
oUl.style.left = -liWidth + 'px';
}

自动轮播
通过 ul标签 定位的改变 实现 显示内容不同的效果
ul标签 定位的改变 是 运动执行的效果
通过定义的运动函数实现
通过定时器 来 调用运动函数
实现 每间隔一段时间 ul做一次定位的改变
定时器的 时间间隔 必须要 大于 move() 运动函数的时间
function autoLoop() {
time = setInterval(function () {
index++;
console.log(index)
setFocusStyle();
move(oUl, {left: -index * liWidth}, loopEnd);
}, 4000)
}
焦点样式函数
清除所有ol>li标签焦点样式
给 显示的 ul>li 对应的 ol>li 标签添加焦点样式
ul>li索引下标数值 - 1 是 ol>li索引下标数值
也就是 index存储数值 -1 是 ol>li标签的索引下标
特殊情况
当 index 存储的索引下标是 最后一个li的索引下标时
index-1 没有对应的 ol>li标签
此时 应该是 所有 ol>li 中 索引下标是0的 第一个ol>li 标签 添加样式
当 index 存储的索引下标是 第一个li的索引下标时
index-1 没有对应的 ol>li标签
此时 应该是 所有 ol>li 中 索引下标是 最后一个 ol>li标签 添加样式
最后一个ul>li 给 第一个 ol>li 添加样式
第一个ul>li 给 最后一个 ol>li 添加样式
function setFocusStyle() {
oOlLis.forEach(function (item, key) {
item.classList.remove('active');
if (key === index - 1) {
item.classList.add('active');
}
})
console.log(index)
if (index === length + 1) {
oOlLis[0].classList.add('active');
} else if (index === 0) {
oOlLis[oOlLis.length - 1].classList.add('active');
}
}
轮播图运动结束函数
轮播图切换结束时 触发执行函数
判断 index的数值
如果 index 存储的是 最后一个li的索引下标
也就是 切换到 最后一个li , 也就是克隆的原始轮播图的第一张
给 index 赋值 1 同时立即切换轮播图 到 所有ul>li的第二个标签
如果 index 存储的是 第一个li的索引下标
也就是 切换到 第一个li , 也就是克隆的原始轮播图的最后一张
给 index 赋值 倒数第二个ul>li的索引下标 同时立即切换轮播图 到 所有 ul>li 的 倒数第二个标签
function loopEnd() {
if (index === length + 1) {
index = 1;
oUl.style.left = -index * liWidth + 'px';
} else if (index === 0) {
index = length;
oUl.style.left = -index * liWidth + 'px';
}
bool = true;
}
鼠标移入移出
鼠标移入:
停止轮播图运动
清除 自动轮播 定时器
function setMouse() {
oBanner.addEventListener('mouseenter', function () {
clearInterval(time);
console.log(111);
})
oBanner.addEventListener('mouseleave', function () {
autoLoop();
})
}
点击效果
左右按钮 焦点标签 都是动态生成的
使用事件委托语法 添加点击效果
1, 点击 左按钮
显示 上一个 ul>li 标签内容
变量index--
设定焦点标签css样式
根据 新的 index 定位 ul标签
动态完成切换效果
2, 点击 右按钮
显示 下一个 ul>li 标签内容
变量index++
设定焦点标签css样式
根据 新的 index 定位 ul标签
动态完成切换效果
3, 点击 焦点按钮
显示 点击的 ol>li 对应的 ul>li
ol>li标签索引下标+1 是 对应 ul>li索引下标
变量index 赋值 ol>li索引下标+1
设定焦点标签css样式
根据 新的 index 定位 ul标签
动态完成切换效果
function setClick() {
oBanner.addEventListener('click', function (e) {
if (e.target.getAttribute('name') === 'left') {
if (!bool) {
return;
}
index--;
setFocusStyle();
bool = false
move(oUl, {
left: -index * liWidth
}, loopEnd);
} else if (e.target.getAttribute('name') === 'right') {
if (!bool) {
return;
}
index++;
setFocusStyle();
bool = false
move(oUl, {left: -index * liWidth}, loopEnd);
} else if (e.target.getAttribute('name') === 'focus') {
if (!bool) {
return;
}
index = e.target.getAttribute('num') - 0 + 1;
setFocusStyle();
bool = false
move(oUl, {
left: -index * liWidth
}, loopEnd);
}
})
}
浏览器后台运行
浏览器最小化 或者 显示运行其他程序
浏览器不再是主要运行的程序 操作系统会切换到后台运行
再次 显示浏览器时 轮播图执行会出现混乱
当浏览器后台运行时 停止轮播图的自动轮播
浏览器显示时 再次触发轮播图的运行
给 document 添加 visibilitychange 浏览器显示状态 监听事件
浏览器显示状态改变时会触发函数程序
document.visibilityState 浏览器显示状态描述
hidden 隐藏 也就是 后台运行
清除 定时器 终止 轮播图的自动执行
visible 显示 也就是 正常运行浏览器
再次调用 自动轮播函数
function setHide() {
document.addEventListener('visibilitychange', function () {
if (document.visibilityState === 'hidden') {
clearInterval(time);
} else if (document.visibilityState === 'visible') {
autoLoop();
}
})
}
防止点击过快
本质是防止 上一个定时器没有执行结束 就触发下一个定时
也就是 防止同时有多个定时器触发执行
设定一个变量 存储原始值
判断变量 如果存储是原始值 变量赋值其他数值 执行之后的程序
判断变量 如果存储不是原始值 执行return 终止之后的程序
轮播图重点1:
定时器的嵌套
通过定时器A 每间隔一段时间 调用触发定时器B
定时器A 的间隔时间 一定要 大于 定时器B的执行时间
如果 定时器A的时间间隔短 就会 同时 有 多个定时器B 在同时执行
就是同时操作 同一个对象 造成显示混乱效果
轮播图重点2:
通过 ul标签定位的改变 执行效果是显示不同的li标签也就是显示不同的图片
通过 显示li标签的索引下标 * li标签的宽度 控制 ul标签的定位
定义变量 index 存储 索引下标数值
也就是 通过 控制 index 存储的数据数值 控制 ul标签的定位 控制显示内容
改变显示内容 一定要 先改变 index存储的数值
再 通过 index存储的数值 改变ul标签的定位
全部code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
ul,
ol,
li {
list-style: none;
}
a,
a:hover,
a:active {
text-decoration: none;
}
img {
width: 100%;
height: 100%;
display: block;
}
.banner {
width: 600px;
height: 400px;
border: 5px solid #000;
position: relative;
margin: 50px auto;
}
.banner>ul {
width: 500%;
height: 100%;
position: absolute;
top: 0;
left: 0;
}
.banner>ul>li {
float: left;
width: 600px;
height: 400px;
}
.banner>ol {
height: 50px;
position: absolute;
bottom: 50px;
left: 50%;
transform: translateX(-50%);
background: rgba(0, 0, 0, 0.5);
display: flex;
justify-content: center;
align-items: center;
border-radius: 15px;
}
.banner>ol>li {
width: 20px;
height: 20px;
border-radius: 50%;
background: #fff;
margin: 0 15px;
cursor: pointer;
}
.banner>ol>li.active {
background: red;
}
.banner>div {
width: 100%;
height: 50px;
position: absolute;
left: 0;
top: 50%;
transform: translateY(-50%);
display: flex;
justify-content: space-between;
align-items: center;
}
.banner>div>a {
display: flex;
justify-content: center;
align-items: center;
width: 50px;
height: 50px;
font-size: 40px;
color: #fff;
}
</style>
</head>
<body>
<div class="banner">
<ul></ul>
<ol></ol>
<div>
<a href="JavaScript:;" name="left"><</a>
<a href="JavaScript:;" name="right">></a>
</div>
</div>
<script src="move.js"></script>
<script>
var bannerArr = [{
id: 1,
width: 500,
height: 333,
size: 29.7,
name: '1.jpg'
},
{
id: 2,
width: 500,
height: 333,
size: 19.3,
name: '2.jpg'
},
{
id: 3,
width: 500,
height: 333,
size: 17.1,
name: '3.jpg'
},
{
id: 4,
width: 500,
height: 333,
size: 20.3,
name: '4.jpg'
},
{
id: 5,
width: 600,
height: 400,
size: 320,
name: '5.jpg'
},
];
const oBanner = document.querySelector('.banner');
const oUl = oBanner.querySelector('ul');
const oOl = oBanner.querySelector('ol');
let oUlLis;
let oOlLis;
let length = bannerArr.length;
let liWidth;
let index = 1;
let time;
let bool = true;
setPage();
autoLoop();
setMouse();
setClick();
setHide();
function setPage() {
let ulStr = '';
let olStr = '';
bannerArr.forEach(function (item, key) {
ulStr += `<li><img src="./images/${item.name}"></li>`;
olStr += key === 0 ? `<li class="active" name="focus" num="${key}"></li>` :
`<li name="focus" num="${key}"></li>`;
})
oUl.innerHTML = ulStr;
oOl.innerHTML = olStr;
oUlLis = oUl.querySelectorAll('li');
oOlLis = oOl.querySelectorAll('li');
const cloneFirst = oUlLis[0].cloneNode(true);
const cloneLast = oUlLis[oUlLis.length - 1].cloneNode(true);
oUl.appendChild(cloneFirst);
oUl.insertBefore(cloneLast, oUlLis[0]);
liWidth = oUlLis[0].offsetWidth;
oUl.style.width = (length + 2) * liWidth + 'px';
oUl.style.left = -liWidth + 'px';
}
function autoLoop() {
time = setInterval(function () {
index++;
console.log(index)
setFocusStyle();
move(oUl, {
left: -index * liWidth
}, loopEnd);
}, 4000)
}
function loopEnd() {
if (index === length + 1) {
index = 1;
oUl.style.left = -index * liWidth + 'px';
} else if (index === 0) {
index = length;
oUl.style.left = -index * liWidth + 'px';
}
bool = true;
}
function setFocusStyle() {
oOlLis.forEach(function (item, key) {
item.classList.remove('active');
if (key === index - 1) {
item.classList.add('active');
}
})
console.log(index)
if (index === length + 1) {
oOlLis[0].classList.add('active');
} else if (index === 0) {
oOlLis[oOlLis.length - 1].classList.add('active');
}
}
function setMouse() {
oBanner.addEventListener('mouseenter', function () {
clearInterval(time);
console.log(111);
})
oBanner.addEventListener('mouseleave', function () {
autoLoop();
})
}
function setClick() {
oBanner.addEventListener('click', function (e) {
if (e.target.getAttribute('name') === 'left') {
if (!bool) {
return;
}
index--;
setFocusStyle();
bool = false
move(oUl, {
left: -index * liWidth
}, loopEnd);
} else if (e.target.getAttribute('name') === 'right') {
if (!bool) {
return;
}
index++;
setFocusStyle();
bool = false
move(oUl, {left: -index * liWidth}, loopEnd);
} else if (e.target.getAttribute('name') === 'focus') {
if (!bool) {
return;
}
index = e.target.getAttribute('num') - 0 + 1;
setFocusStyle();
bool = false
move(oUl, {
left: -index * liWidth
}, loopEnd);
}
})
}
function setHide() {
document.addEventListener('visibilitychange', function () {
if (document.visibilityState === 'hidden') {
clearInterval(time);
} else if (document.visibilityState === 'visible') {
autoLoop();
}
})
}
</script>
</body>
</html>
|