vue中d3的click事件怎么获取对应数据?? vue @click 绑定的函数,如何同时传入事件对象和自定义参数??vue中第三方插件的click事件,传回来第一个参数是vue的事件、第二个参数才是插件本身的内容。.on(‘click’, function ($event, e) {})
https://blog.csdn.net/little_kid_pea/article/details/89736282
- html 文件中 d3 的 click 事件返回的
.on('click', function (evt) {
console.log('this',this);
console.log(evt)
})
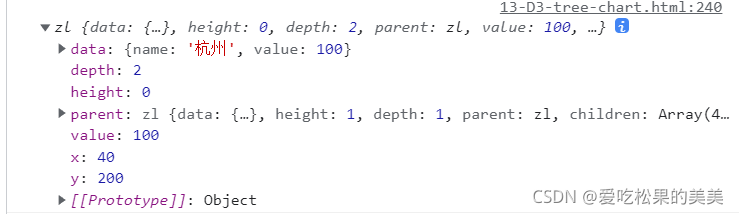
- vue 项目中同样的想要获取组件上的数据的话需要这样写
.on('click', function ($event, e) {
console.log($event, e)
})

- 完整代码
点击对应框框回调对应数据 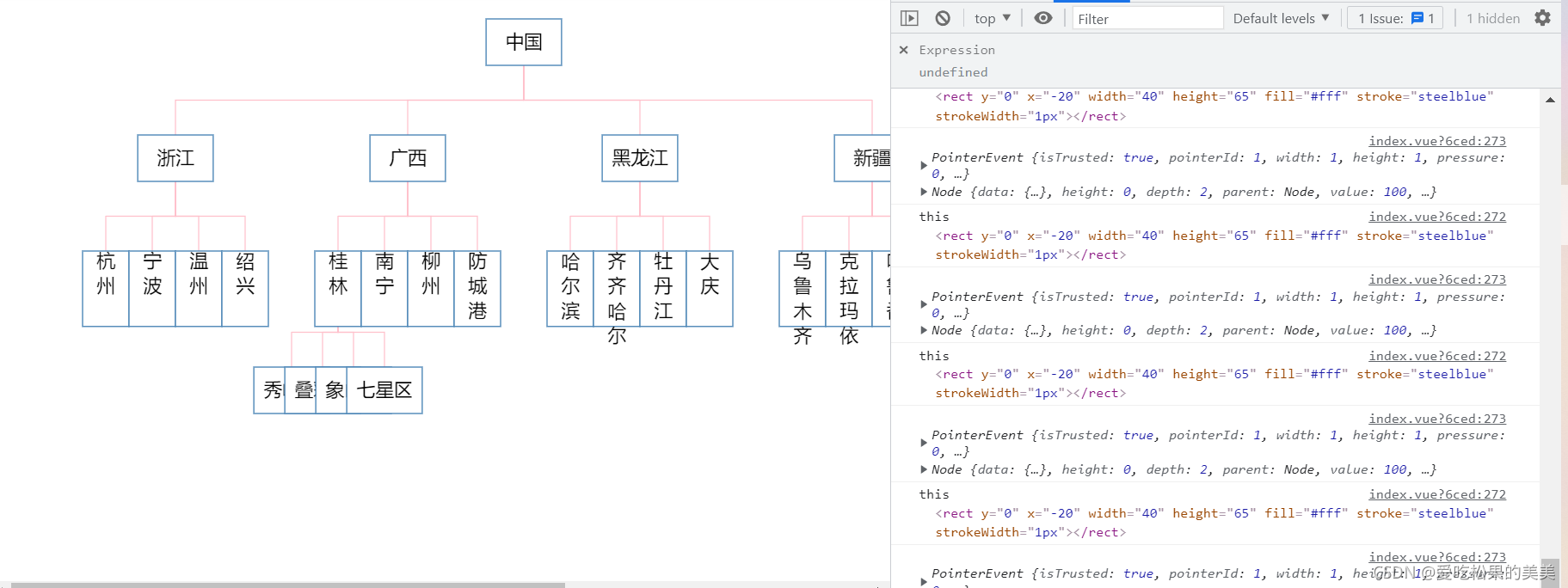
<template>
<div class="page-main">
<svg width="1200" height="500"></svg>
</div>
</template>
<script>
import * as d3 from 'd3'
export default {
name: 'districtManagement',
mounted() {
var data
data = {
name: '中国',
children: [
{
name: '浙江',
children: [
{ name: '杭州', value: 100 },
{ name: '宁波', value: 100 },
{ name: '温州', value: 100 },
{ name: '绍兴', value: 100 },
],
},
{
name: '广西',
children: [
{
name: '桂林',
children: [
{ name: '秀峰区', value: 100 },
{ name: '叠彩区', value: 100 },
{ name: '象山区', value: 100 },
{ name: '七星区', value: 100 },
],
},
{ name: '南宁', value: 100 },
{ name: '柳州', value: 100 },
{ name: '防城港', value: 100 },
],
},
{
name: '黑龙江',
children: [
{ name: '哈尔滨', value: 100 },
{ name: '齐齐哈尔', value: 100 },
{ name: '牡丹江', value: 100 },
{ name: '大庆', value: 100 },
],
},
{
name: '新疆',
children: [{ name: '乌鲁木齐' }, { name: '克拉玛依' }, { name: '吐鲁番' }, { name: '哈密' }],
},
],
}
var margin = 60
var svg = d3.select('.page-main').select('svg')
var width = svg.attr('width')
var height = svg.attr('height')
var boxWidth = 65
var boxHeight = 40
var g = svg.append('g').attr('transform', 'translate(' + margin + ',' + margin + ')')
var hierarchyData = d3.hierarchy(data).sum(function (d, i) {
return d.value
})
console.log(hierarchyData)
var tree = d3
.tree()
.size([width - 400, height - 200])
.separation(function (a, b) {
return (a.parent == b.parent ? 1 : 2) / a.depth
})
var treeData = tree(hierarchyData)
console.log(treeData)
var nodes = treeData.descendants()
var links = treeData.links()
console.log(nodes)
console.log(links)
var link = d3
.linkVertical()
.x(function (d) {
return d.x
})
.y(function (d) {
return d.y
})
function elbow(d) {
let sourceX = d.source.x,
sourceY = d.source.y + boxHeight,
targetX = d.target.x,
targetY = d.target.y
return 'M' + sourceX + ',' + sourceY + 'V' + ((targetY - sourceY) / 2 + sourceY) + 'H' + targetX + 'V' + targetY
}
g.append('g')
.selectAll('path')
.data(links)
.enter()
.append('path')
.attr('d', elbow)
.attr('stroke', 'pink')
.attr('stroke-width', 1)
.attr('fill', 'none')
var gs = g
.append('g')
.selectAll('.node')
.data(nodes)
.enter()
.append('g')
.attr('class', 'node')
.attr('strokeWidth', 100)
.attr('transform', function (d, i) {
return 'translate(' + d.x + ',' + d.y + ')'
})
gs.append('rect')
.attr('y', 0)
.attr('x', function (d) {
return d.depth !== 2 ? -(boxWidth / 2) : -(boxHeight / 2)
})
.attr('width', function (d) {
return d.depth !== 2 ? boxWidth : boxHeight
})
.attr('height', function (d) {
return d.depth !== 2 ? boxHeight : boxWidth
})
.attr('fill', '#fff')
.attr('stroke', 'steelblue')
.attr('strokeWidth', '1px')
.on('click', function ($event, e) {
console.log('this',this);
console.log($event, e)
})
gs.append('text')
.attr('y', function (d) {
return d.depth !== 2 ? boxHeight / 2 + 5 : 0
})
.attr('style', function (d) {
return d.depth !== 2 ? '' : 'writing-mode: tb;letter-spacing:0px'
})
.attr('text-anchor', function (d) {
return d.depth !== 2 ? 'middle' : 'start'
})
.text(function (d) {
return d.data.name
})
},
}
</script>
|