一、是什么
Vuex 是一个专为 Vue.js 应用程序开发的状态管理模式。它采用集中式存储管理应用的所有组件的状态,并以相应的规则保证状态以一种可预测的方式发生变化。每一个 Vuex 应用的核心就是 store (仓库)。store 基本上就是一个容器,它包含着你的应用中大部分的状态 (state )。 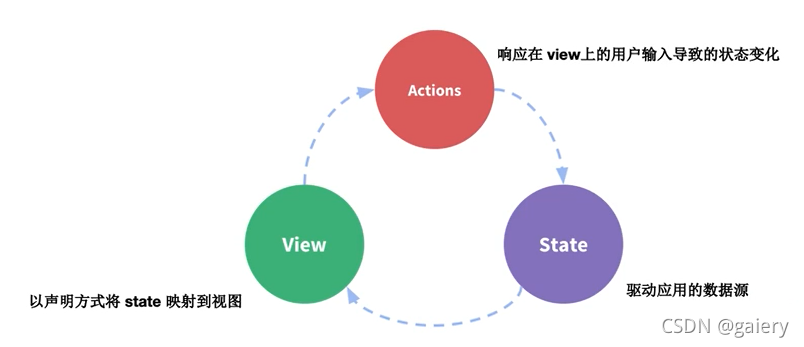
二、安装
npm install vuex --save
在一个模块化的打包系统中,必须显式地通过 Vue.use() 来安装 Vuex: store.js 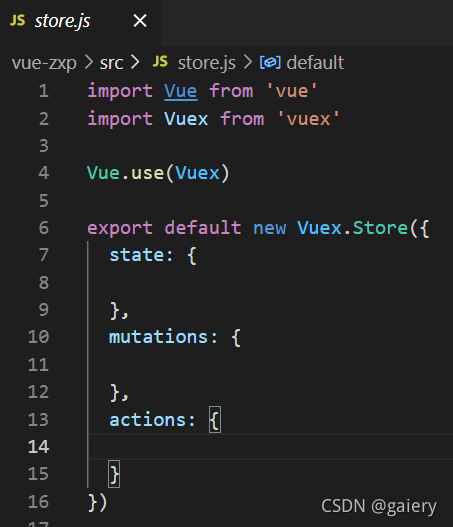 main.js 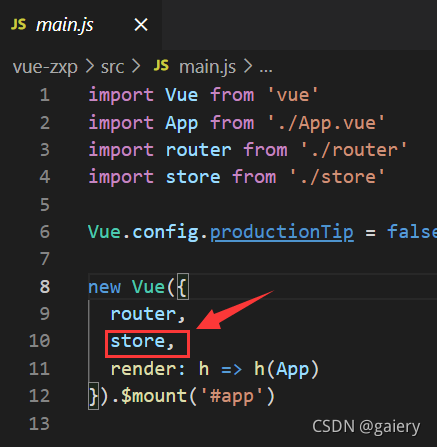
三、使用
app.vue
<template>
<div id="app">
<div>{{countGet}}</div>
<div>{{$store.getters.doubleCount}}</div>
<button @click="$store.commit('increment',5)">count++</button>
<button @click="$store.dispatch('increment2')">count++异步操作</button>
</div>
</template>
<script>
export default {
name:'app',
computed:{
countGet(){
return this.$store.state.count
}
}
}
</script>
store.js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
count:0
},
mutations: {
increment(state,n){
state.count+=n
}
},
actions: {
increment2({state}){
setTimeout(() => {
state.count++
}, 3000);
}
},
getters: {
doubleCount(state){
return state.count*2
}
}
})
四、vuex核心概念及底层原理
核心概念
- state:this.$store.state.xxx取值
- getter:this.$store.getters.xxx取值
- mutation:this.$store.commint(“xxx”)赋值
- actions:this.$store.dispatch(“xxx”)赋值
- modules
底层原理
- state:提供一个响应式数据
- getter:借助vue的计算属性computed来实现缓存
- mutation:更改state的方法
- actions:触发mutation的方法
- modules:vue.set动态添加state到响应式数据中
1、 src下创建min-vuex.js
import Vue from "vue";
const Store = function store(options = {}){
const {state={},mutations={}} = options
this._vm = new Vue({
data:{
$$state: state
}
})
this._mutations = mutations
}
Store.prototype.commit = function(type,payload){
if(this._mutations[type]){
this._mutations[type](this.state,payload)
}
}
Object.defineProperties(Store.prototype, {
state : {
get:function(){
return this._vm._data.$$state
}
}
})
export default {Store}
2、store.js中引入的vuex改为从min-vuex中引入
import Vue from 'vue'
import Vuex from './min-vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
count:0
},
mutations: {
increment(state,n){
state.count += n
}
},
})
3、main.js中就访问不到vue原来默认的store了,我们可以在原型上设置为我们自己写store
import Vue from 'vue'
import App from './App.vue'
import router from './router'
import store from './store'
Vue.config.productionTip = false
Vue.prototype.$store=store;
new Vue({
router,
render: h => h(App)
}).$mount('#app')
4、使用方法
<div id="app">
<div id="nav">
</div>
<div>{{countGet}}</div>
<button @click="$store.commit('increment',5)">count++</button>
<router-view/>
</div>
至此,我们自己写的vuex的state和commit功能就可以了,原来的count++依旧好用。
|