一、新增页面书籍类别下拉框加载
1、查询所有类型的方法(CategoryDao)
package com.xly.dao;
import java.util.List;
import com.xly.entity.Category;
import com.zking.util.BaseDao;
import com.zking.util.PageBean;
public class CategoryDao extends BaseDao<Category>{
public List<Category> list(Category category, PageBean pageBean) throws Exception {
String sql="select * from t_easyui_category where 1=1";
long id = category.getId();
if(id!=0) {
sql+=" and id ="+id;
}
return super.executeQuery(sql, Category.class, pageBean);
}
// select b.*,c.name from t_easyui_book b, t_easyui_category c where b.cid=c.id
}
2、子控制器(CategoryAction)
package com.xly.web;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.xly.dao.CategoryDao;
import com.xly.entity.Category;
import com.zking.framework.ActionSupport;
import com.zking.framework.ModelDriver;
import com.zking.util.ResponseUtil;
public class CategoryAction extends ActionSupport implements ModelDriver<Category>{
private Category category=new Category();
private CategoryDao categoryDao=new CategoryDao();
public Category getModel() {
return category;
}
/**
* 加载数据类别下拉框
* @param req
* @param resp
* @return
*/
public String combobox(HttpServletRequest req, HttpServletResponse resp) {
try {
List<Category> list = categoryDao.list(category, null);
ResponseUtil.writeJson(resp, list);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
3、在点击菜单栏需弹出一个增加的提示窗口
$(function(){
$("#bookMenus").tree({
url:$("#ctx").val()+"/permission.action?methodName=tree",
// 给菜单栏一个点击
onClick: function(node){
// 判断面板是否存在
var exists=$("#bookTabs").tabs('exists',node.text);
if(exists){
$("#bookTabs").tabs('select',node.text);
}else{
$('#bookTabs').tabs('add',{
title:node.text,
content:'<iframe width="100%" height="100%" src="'+$("#ctx").val()+node.attributes.self.url+'" />',
closable:true
});
}
}
});
})
4、添加api事件
?<input id="cid" name="cid" value="" label="类别" >
二、书籍上架下架新增功能
(一)、新增功能
1、完成新增功能实体类
package com.xly.entity;
import java.util.Date;
import com.fasterxml.jackson.annotation.JsonFormat;
public class Book {
private long id;
private String name;
private String pinyin;
private long cid;
private String author;
private float price;
private String image;
private String publishing;
private String description;
private int state;
@JsonFormat(pattern="yyyy-MM-dd HH:mm:ss",timezone = "GMT+8")
private Date deployTime;
private int sales;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPinyin() {
return pinyin;
}
public void setPinyin(String pinyin) {
this.pinyin = pinyin;
}
public long getCid() {
return cid;
}
public void setCid(long cid) {
this.cid = cid;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public float getPrice() {
return price;
}
public void setPrice(float price) {
this.price = price;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
public String getPublishing() {
return publishing;
}
public void setPublishing(String publishing) {
this.publishing = publishing;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public int getState() {
return state;
}
public void setState(int state) {
this.state = state;
}
public Date getDeployTime() {
return deployTime;
}
public void setDeployTime(Date deployTime) {
this.deployTime = deployTime;
}
public int getSales() {
return sales;
}
public void setSales(int sales) {
this.sales = sales;
}
@Override
public String toString() {
return "Book [id=" + id + ", name=" + name + ", pinyin=" + pinyin + ", cid=" + cid + ", author=" + author
+ ", price=" + price + ", image=" + image + ", publishing=" + publishing + ", description="
+ description + ", state=" + state + ", deployTime=" + deployTime + ", sales=" + sales + "]";
}
}
?2、写方法BookDao?
public void add( Book t) throws Exception {
// 转化拼音
t.setPinyin(PinYinUtil.getAllPingYin(t.getName()));
t.setDeployTime(new Date());
String sql="insert into t_easyui_book(name,pinyin,cid,author,price,image,publishing,description,state,deployTime,sales) values(?,?,?,?,?,?,?,?,?,?,?)";
super.executeUpdate(sql,
t, new String[] {"name","pinyin","cid","author","price","image","publishing","description","state","deployTime","sales"});
}
3、写子控制器(BookAction)
public void add(HttpServletRequest req, HttpServletResponse resp) {
try {
bd.add(book);
ResponseUtil.writeJson(resp, 1);
} catch (Exception e) {
e.printStackTrace();
try {
ResponseUtil.writeJson(resp, 0);
} catch (Exception e1) {
e1.printStackTrace();
}
}
}
4、获取数据,提交表单
/* 通过form控件提交 */
function submitForm() {
$('#ff').form('submit', {
url:'${pageContext.request.contextPath}/book.action?methodName=add',
success:function(data){
if(data==1){
$('#ff').form('clear');
}
}
});
}
/* 刷新 */
function clearForm() {
$('#ff').form('clear');
}
5.1、效果显示
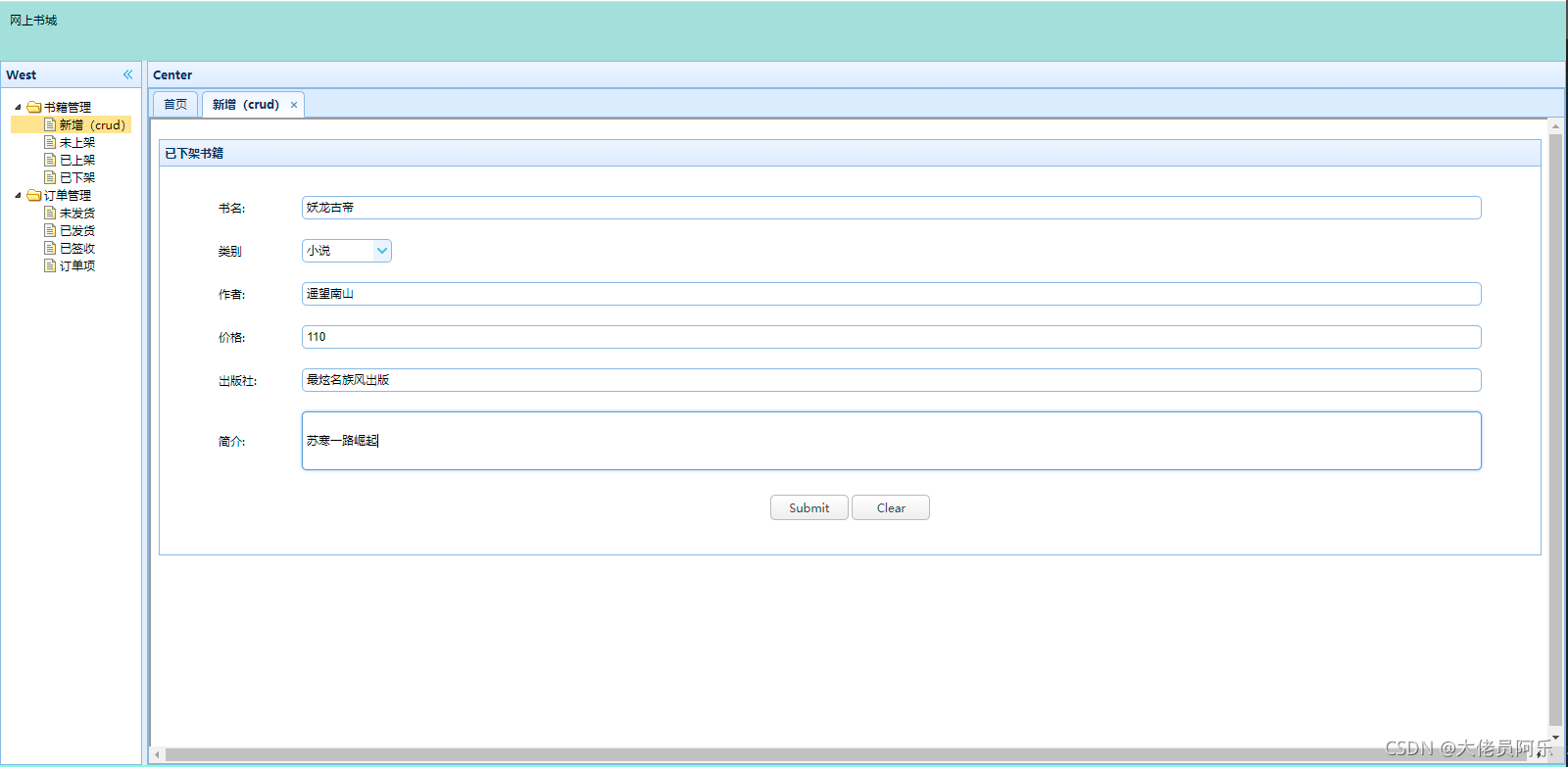
5.2、新增后效果(未上架页面显示?)
(二)、上架与下架
1、上架方法
package com.xly.dao;
import java.util.Date;
import java.util.List;
import com.xly.entity.Book;
import com.zking.util.BaseDao;
import com.zking.util.PageBean;
import com.zking.util.PinYinUtil;
import com.zking.util.StringUtils;
public class BookDao extends BaseDao<Book> {
public List<Book> list(Book book, PageBean pageBean) throws Exception {
String sql="select * from t_easyui_book where 1=1";
String name=book.getName();
int state = book.getState();
if(StringUtils.isNotBlank(name)) {
sql+=" and name like '%"+ name +"%'";
}
if(state!=0) {
sql+=" and state="+state;
}
return super.executeQuery(sql, Book.class, pageBean);
}
public void edit(Book t) throws Exception {
super.executeUpdate("update t_easyui_book set name=?,pinyin=?,cid=?,image=?,state=?,sales=? where id=?", t, new String[] {
"name","pinyin","cid","image","state","sales","id"});
}
public void add(Book t) throws Exception {
t.setPinyin(PinYinUtil.getAllPingYin(t.getName()));
t.setDeployTime(new Date());
super.executeUpdate("insert into t_easyui_book(name,pinyin,cid,author,price,image,publishing,description,state,deployTime,sales) values(?,?,?,?,?,?,?,?,?,?,?)",t,
new String[] {"name","pinyin","cid","author","price","image","publishing","description","state","deployTime","sales"});
}
public void editStatus(Book t) throws Exception {
super.executeUpdate("update t_easyui_book set state=? where id = ? ", t, new String[] {"state","id"});
}
}
2、子控制器
public void list(HttpServletRequest req, HttpServletResponse resp) {
PageBean pageBean=new PageBean();
pageBean.setRequest(req);
try {
List<Book> list = bd.list(book, pageBean);
ResponseUtil.writeJson(resp, new R().data("total", pageBean.getTotal())
.data("rows", list));
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
// 如果上架,书籍的状态改为2
// 如果下架,书籍的状态改为3
public void editStatus(HttpServletRequest req, HttpServletResponse resp) {
try {
bd.editStatus(book);
ResponseUtil.writeJson(resp, 1);
} catch (Exception e) {
e.printStackTrace();
try {
ResponseUtil.writeJson(resp, 0);
} catch (Exception e1) {
e1.printStackTrace();
}
}
3、js方法
function shangjia() {
$.messager.confirm('确认','您确认想要上架此书籍吗?',function(r){
if (r){
var row = $('#dg').datagrid('getSelected');
if (row){
$.ajax({
url:'${pageContext.request.contextPath}/book.action?methodName=editStatus&state=2&id=' + row.id,
success:function (data) {
}
})
}
}
});
}
根据状态的不同,改变上下架
function xiajia() {
$.messager.confirm('确认','您确认想要下架此书籍吗?',function(r){
if (r){
var row = $('#dg').datagrid('getSelected');
if (row){
$.ajax({
url:'${pageContext.request.contextPath}/book.action?methodName=editStatus&state=3&id=' + row.id,
success:function (data) {
$('#dg').datagrid('reload'); // 重新载入当前页面数据
}
})
}
}
});
}
4.1、展示效果(上架)
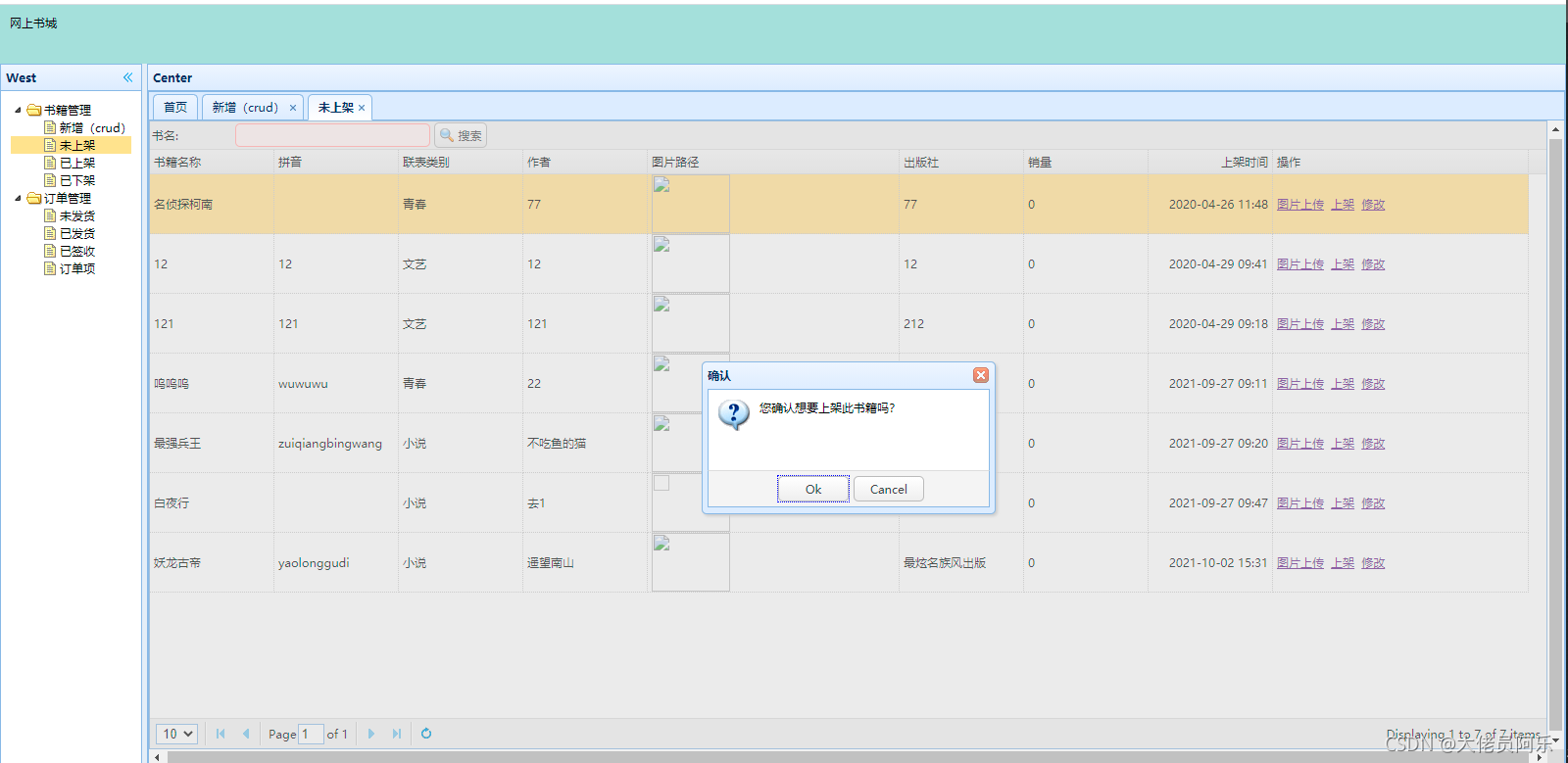
上架后上架页面显示
?
已上架页面加载显示
?
4.2、下架 ;点击下架
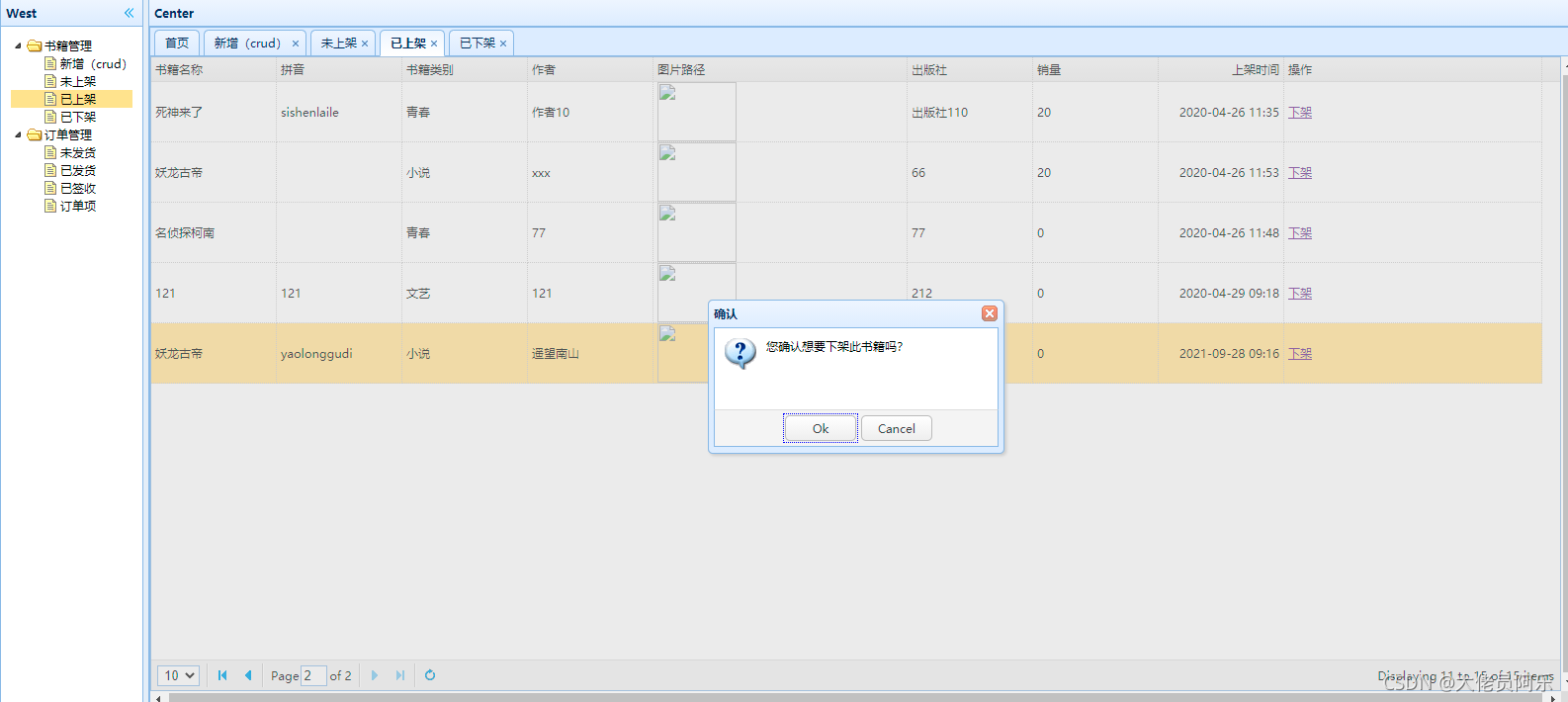
?
已下架页面显示
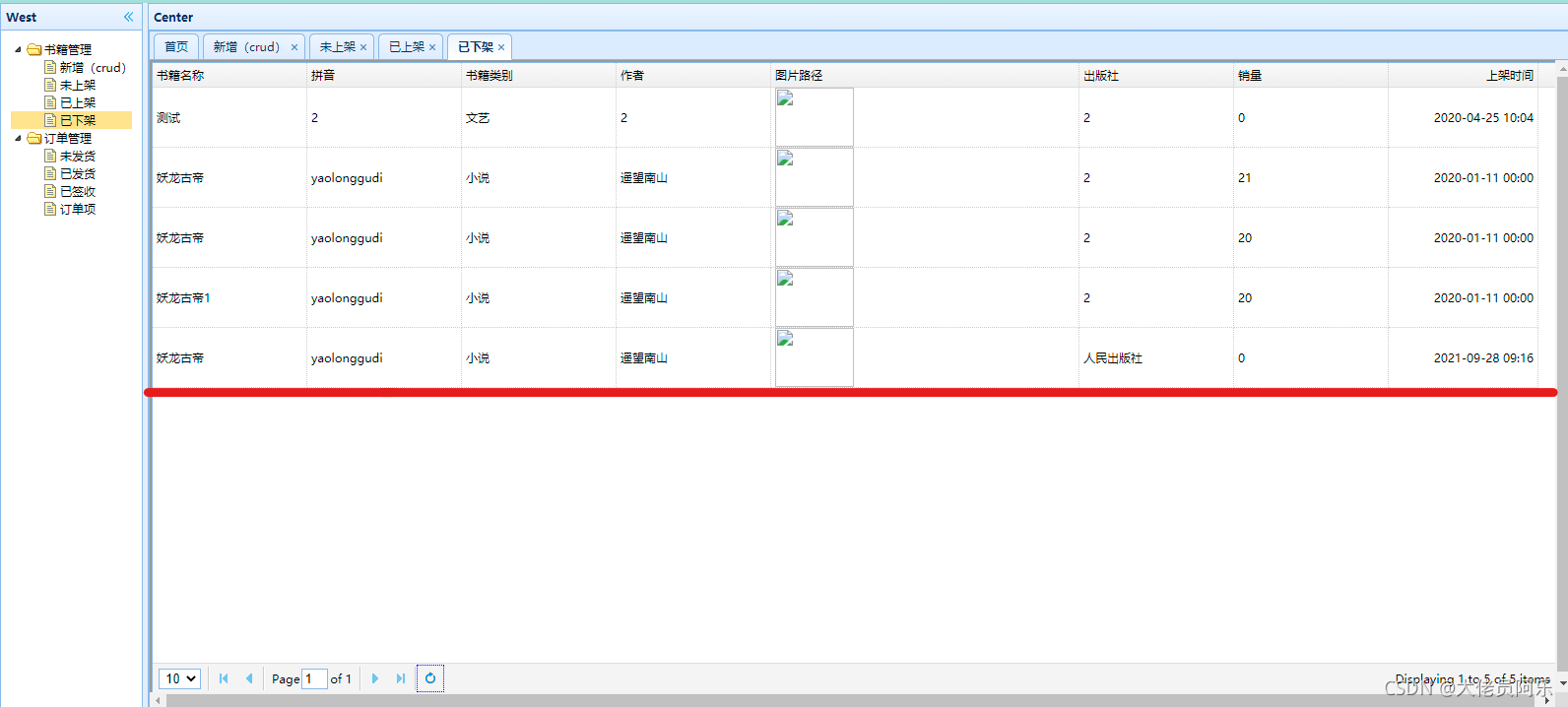
功能显示完成!!!!!
|